Bash ‘Else If’ Statement | Shell Scripting Conditionals
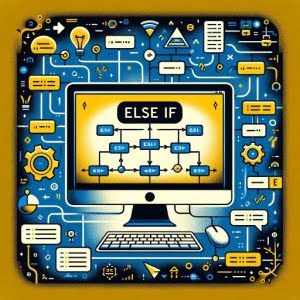
Are you finding it challenging to use ‘else if’ in bash scripting? You’re not alone. Many developers find themselves puzzled when it comes to handling ‘else if’ statements in bash scripting, but we’re here to help.
Think of ‘else if’ in bash scripting as a traffic cop, directing the flow of your code based on certain conditions. It’s a powerful tool that can make your scripts more flexible and responsive to various scenarios.
In this guide, we’ll walk you through the process of understanding and using ‘else if’ effectively in your bash scripts. We’ll cover everything from the basics of ‘else if’ to more advanced techniques, as well as alternative approaches.
Let’s get started and master ‘else if’ in bash scripting!
TL;DR: How Do I Use ‘Else If’ in Bash Scripting?
'Else if'
in bash scripting, often written as'elif'
, is used to check multiple conditions in your code. It is used with the syntax,if [firstStatement]; then... elif [secondCondition]; then... else
. It’s a powerful tool that allows your scripts to make decisions based on various scenarios.
Here’s a simple example:
a=10
b=20
if [ $a -eq $b ]; then
echo 'a is equal to b'
elif [ $a -gt $b ]; then
echo 'a is greater than b'
else
echo 'a is less than b'
fi
# Output:
# 'a is less than b'
In this example, we have two variables, ‘a’ and ‘b’. The script checks if ‘a’ is equal to ‘b’. If it’s not, it moves on to the ‘elif’ statement to check if ‘a’ is greater than ‘b’. If neither condition is met, it executes the ‘else’ statement, which in this case, prints ‘a is less than b’.
This is a basic way to use ‘else if’ in bash scripting, but there’s much more to learn about handling multiple conditions in your scripts. Continue reading for a deeper understanding and more complex usage scenarios.
Table of Contents
- Understanding ‘Else If’ in Bash Scripting
- Delving Deeper: Nested ‘Else If’ Statements
- Exploring Alternatives: The ‘Case’ Statement
- Handling Common Errors in Bash ‘Else If’
- Bash Scripting and Conditional Statements
- Expanding Horizons: ‘Else If’ in Larger Bash Scripts
- Wrapping Up: Mastering ‘Else If’ in Bash Scripting
Understanding ‘Else If’ in Bash Scripting
‘Else if’, or ‘elif’ as it’s often written in bash, is a fundamental part of conditional statements in bash scripting. It allows your scripts to check multiple conditions and execute different blocks of code based on the outcomes of these checks.
Syntax of ‘Else If’
The basic syntax of ‘else if’ in bash scripting is as follows:
if [ condition1 ]; then
# code to execute if condition1 is true
elif [ condition2 ]; then
# code to execute if condition1 is false and condition2 is true
else
# code to execute if both condition1 and condition2 are false
fi
Basic Example
Let’s look at a simple example to illustrate this:
a=5
b=10
c=15
if [ $a -gt $b ]; then
echo 'a is greater than b'
elif [ $a -gt $c ]; then
echo 'a is greater than c'
else
echo 'a is not greater than either b or c'
fi
# Output:
# 'a is not greater than either b or c'
In this example, the script first checks if ‘a’ is greater than ‘b’. If it’s not, it moves on to the ‘elif’ statement to check if ‘a’ is greater than ‘c’. If neither condition is met, it executes the ‘else’ statement, which in this case, prints ‘a is not greater than either b or c’.
Advantages and Pitfalls
The main advantage of using ‘else if’ in bash scripting is that it allows your scripts to handle multiple conditions, making them more flexible and powerful. However, it’s important to be aware of potential pitfalls. For instance, the order of your conditions matters. The script will execute the first condition that is met and ignore the rest, even if they are also true. So, always structure your conditions from the most specific to the most general.
Delving Deeper: Nested ‘Else If’ Statements
As you become more comfortable with using ‘else if’ in bash scripting, you can start exploring more complex usage scenarios. One such scenario is nested ‘else if’ statements.
Nested ‘Else If’ in Bash
Nested ‘else if’ statements are basically ‘if-elif-else’ statements within another ‘if-elif-else’ statement. This allows you to check multiple conditions within each condition of your script.
Here’s an example to illustrate this:
a=5
b=10
c=15
d=20
echo 'Enter a number:'
read number
if [ $number -eq $a ]; then
echo 'You entered 5'
elif [ $number -eq $b ]; then
echo 'You entered 10'
elif [ $number -eq $c ]; then
if [ $number -lt $d ]; then
echo 'You entered 15, which is less than 20'
else
echo 'You entered 15, which is not less than 20'
fi
else
echo 'You did not enter 5, 10, or 15'
fi
# Output:
# 'Enter a number:'
# 'You entered 15, which is less than 20'
In this example, we have a nested ‘if-else’ statement within the third ‘elif’ statement. If the user enters ’15’, the script checks if ’15’ is less than ’20’ and prints a message accordingly.
Best Practices
When using nested ‘else if’ statements, it’s crucial to keep your code organized and easy to read. Make sure to indent your code properly to indicate the hierarchy of your conditions. Also, while nested ‘else if’ statements can make your scripts more powerful, they can also make them more complex. Use them sparingly and only when necessary.
Exploring Alternatives: The ‘Case’ Statement
While ‘else if’ is a powerful tool for handling multiple conditions in bash scripting, it’s not the only one. An alternative approach is the ‘case’ statement. ‘Case’ statements can be more readable and easier to maintain than a long list of ‘elif’ conditions, especially when you’re dealing with many possible conditions.
Using ‘Case’ in Bash Scripting
A ‘case’ statement in bash scripting checks a variable or expression against a list of patterns. When a match is found, it executes the associated block of code.
Here’s the basic syntax of a ‘case’ statement:
case expression in
pattern1 )
# code to execute if pattern1 matches
;;
pattern2 )
# code to execute if pattern2 matches
;;
*
# code to execute if no pattern matches
esac
Example of ‘Case’ Statement
Let’s look at an example:
echo 'Enter a fruit (apple, banana, or cherry):'
read fruit
case $fruit in
apple )
echo 'You entered apple'
;;
banana )
echo 'You entered banana'
;;
cherry )
echo 'You entered cherry'
;;
* )
echo 'You did not enter apple, banana, or cherry'
esac
# Output:
# 'Enter a fruit (apple, banana, or cherry):'
# 'You entered banana'
In this example, the script checks the user’s input against three patterns: ‘apple’, ‘banana’, and ‘cherry’. If the user enters ‘banana’, the script prints ‘You entered banana’. If the user enters something other than these three fruits, the script prints ‘You did not enter apple, banana, or cherry’.
Benefits, Drawbacks, and Considerations
The main benefit of using ‘case’ statements is their readability and ease of maintenance when dealing with many conditions. However, they only work with pattern matching, not complex conditions. So, while ‘case’ statements are a great tool to have in your bash scripting toolbox, they’re not a replacement for ‘else if’. It’s important to understand the strengths and limitations of both and choose the right tool for the job.
Handling Common Errors in Bash ‘Else If’
While ‘else if’ is a powerful tool in bash scripting, it’s not without its challenges. Let’s go over some common errors you might encounter and how to solve them.
Error: Missing Spaces
One of the most common errors in bash scripting is forgetting to include spaces in the ‘if’ and ‘elif’ conditions. In bash, spaces are crucial. Forgetting them can lead to syntax errors.
a=5
b=10
# Incorrect syntax
if [$a -gt $b]; then
echo 'a is greater than b'
fi
# Output:
# ./script.sh: line 3: [5: command not found
In this example, the script fails because there are no spaces between the brackets and the variables. The correct syntax is if [ $a -gt $b ]; then
.
Error: Incorrect Condition
Another common error is using the wrong operator in the condition. For example, using ‘=’ instead of ‘-eq’ for numerical comparison can lead to unexpected results.
a=5
b=5
# Incorrect syntax
if [ $a = $b ]; then
echo 'a is equal to b'
fi
# Output:
# a is equal to b
In this example, the script works, but it’s not correct. The ‘=’ operator is for string comparison, not numerical comparison. For numerical comparison, you should use ‘-eq’. So, the correct syntax is if [ $a -eq $b ]; then
.
Best Practices and Optimization
To avoid these and other errors, here are some best practices:
- Always include spaces in your ‘if’ and ‘elif’ conditions.
- Use the correct operators for numerical and string comparisons.
- Indent your code properly to make it more readable.
- Test your scripts thoroughly to catch any errors.
- Use ‘case’ statements for pattern matching instead of long ‘elif’ chains.
Remember, the key to effective bash scripting is understanding the syntax and nuances of the language, as well as thorough testing.
Bash Scripting and Conditional Statements
To fully grasp the power of ‘else if’ in bash scripting, it’s essential to understand the fundamentals of bash scripting, conditional statements, and flow control.
What is Bash Scripting?
Bash (Bourne Again SHell) is a command-line interpreter, or shell, for the Linux operating system. A bash script is a file containing a series of commands that the bash shell can execute. These scripts can automate tasks, perform file manipulations, and much more.
Understanding Conditional Statements
Conditional statements are a fundamental part of any programming language, and bash scripting is no exception. They allow your scripts to make decisions based on certain conditions. The basic conditional statements in bash are ‘if’, ‘else’, and ‘elif’ (else if).
Here’s a basic ‘if’ statement in bash:
a=10
if [ $a -gt 5 ]; then
echo 'a is greater than 5'
fi
# Output:
# 'a is greater than 5'
In this example, the script checks if the variable ‘a’ is greater than 5. If the condition is true, it prints ‘a is greater than 5’.
Flow Control with ‘Else If’
‘Else if’, or ‘elif’, adds more flexibility to your conditional statements. It allows you to check multiple conditions in a single ‘if’ statement. The script executes the first condition that is true and ignores the rest.
Here’s an example of an ‘elif’ statement:
a=10
if [ $a -gt 20 ]; then
echo 'a is greater than 20'
elif [ $a -gt 15 ]; then
echo 'a is greater than 15'
elif [ $a -gt 10 ]; then
echo 'a is greater than 10'
else
echo 'a is not greater than 10, 15, or 20'
fi
# Output:
# 'a is not greater than 10, 15, or 20'
In this example, the script checks multiple conditions using ‘elif’. Since none of the conditions are met, it executes the ‘else’ statement and prints ‘a is not greater than 10, 15, or 20’.
Expanding Horizons: ‘Else If’ in Larger Bash Scripts
Understanding ‘else if’ statements is just the beginning. As you delve deeper into bash scripting, you’ll find that ‘else if’ is often used in conjunction with other elements like loops and functions to create more complex and powerful scripts.
‘Else If’ with Loops
In bash scripting, loops are used to repeat a block of code until a certain condition is met. When combined with ‘else if’, you can create scripts that handle a wide range of scenarios.
Here’s an example of a script that uses ‘else if’ within a ‘for’ loop:
for (( i=1; i<=10; i++ ))
do
if [ $i -eq 5 ]; then
echo 'i is equal to 5'
elif [ $i -eq 7 ]; then
echo 'i is equal to 7'
else
echo 'i is not equal to 5 or 7'
fi
done
# Output:
# 'i is not equal to 5 or 7'
# 'i is not equal to 5 or 7'
# 'i is not equal to 5 or 7'
# 'i is not equal to 5 or 7'
# 'i is equal to 5'
# 'i is not equal to 5 or 7'
# 'i is equal to 7'
# 'i is not equal to 5 or 7'
# 'i is not equal to 5 or 7'
# 'i is not equal to 5 or 7'
In this script, the ‘for’ loop iterates over the numbers 1 through 10. For each iteration, the ‘if-elif-else’ statement checks if the current number is equal to 5 or 7 and prints a message accordingly.
‘Else If’ with Functions
Functions are reusable blocks of code that perform a specific task. In bash scripting, you can use ‘else if’ within a function to make your scripts more modular and easier to maintain.
Here’s an example of a script that uses ‘else if’ within a function:
function checkNumber {
if [ $1 -eq 5 ]; then
echo 'The number is 5'
elif [ $1 -eq 10 ]; then
echo 'The number is 10'
else
echo 'The number is not 5 or 10'
fi
}
checkNumber 5
checkNumber 10
checkNumber 15
# Output:
# 'The number is 5'
# 'The number is 10'
# 'The number is not 5 or 10'
In this script, the function ‘checkNumber’ takes a number as an argument and uses an ‘if-elif-else’ statement to check if the number is equal to 5 or 10.
Further Resources for Bash Scripting Mastery
If you want to dive deeper into bash scripting, here are some resources that you might find helpful:
- Advanced Bash-Scripting Guide: This is a comprehensive guide to bash scripting that covers everything from the basics to more advanced topics.
- Bash Scripting Tutorial: This tutorial is a great starting point for beginners. It covers the basics of bash scripting in a clear and concise way.
- Bash Scripting else if Statement – GeeksforGeeks: This GeeksforGeeks article focuses on the else if statement in Bash scripting.
Wrapping Up: Mastering ‘Else If’ in Bash Scripting
In this comprehensive guide, we’ve delved into the use of ‘else if’ in bash scripting, a fundamental tool for managing multiple conditions in your scripts.
We began with the basics, exploring the syntax and basic use of ‘else if’ in bash scripting. We then moved into more advanced territory, examining nested ‘else if’ statements and the power they bring to your scripts. Along the way, we tackled common errors and pitfalls, providing solutions and strategies to keep your scripts running smoothly.
We also ventured beyond ‘else if’, looking at alternative approaches such as the ‘case’ statement. This exploration provided a broader perspective on handling multiple conditions in bash scripting, equipping you with the knowledge to choose the best tool for the job.
Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
‘Else If’ | High | Moderate | Multiple conditions with varying code blocks |
‘Case’ | Moderate | Low | Pattern matching with multiple possibilities |
Whether you’re a beginner just starting out with bash scripting or a seasoned programmer looking to deepen your understanding, we hope this guide has been a valuable resource in mastering ‘else if’ and its alternatives.
With this knowledge in your toolkit, you’re well-equipped to handle complex conditional logic in your bash scripts. Happy scripting!