Python Base64 Encode | b64encode() Function Guide
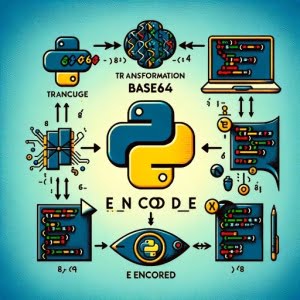
Ever found yourself puzzled over base64 encoding in Python? You’re not alone. Many developers find Python’s base64 encoding a bit of a mystery. Think of it as a secret language that Python can translate, allowing us to handle data in a unique way.
Base64 encoding is a powerful tool that enables us to convert binary data into a format that can be safely sent over systems designed to handle text. This encoding helps to ensure that the data remains intact without modification during transport.
In this guide, we’ll walk you through the process of base64 encoding in Python, from the basics to more advanced techniques. We’ll cover everything from simple encoding scenarios, handling different data types, to alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering base64 encoding in Python!
TL;DR: How Do I Encode Data Using Base64 in Python?
To encode data using base64 in Python, you can use the
base64
module’sb64encode()
function, likeprint(base64.b64encode(data))
. This function takes in binary data as input and returns the base64 encoded version of the input data.
Here’s a simple example:
import base64
data = b'Hello'
encoded_data = base64.b64encode(data)
print(encoded_data)
# Output:
# b'SGVsbG8='
In this example, we import the base64
module and define a byte string data
. We then use the b64encode()
function to encode the data. The function returns the base64 encoded version of ‘Hello’, which is printed out.
This is a basic way to use
b64encode()
for base64 encoding in Python, but there’s much more to learn about handling different data types, alternative approaches, and troubleshooting common issues. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Using b64encode() for Base64 Encoding in Python
- Handling Different Data Types in Base64 Encoding
- Exploring Alternative Methods for Base64 Encoding
- Navigating Python Base64 Encoding Challenges
- Understanding Python’s Base64 Module and Encoding
- Exploring the Impact of Base64 Encoding
- Wrapping Up: Mastering Base64 Encoding in Python
Using b64encode()
for Base64 Encoding in Python
In Python, the base64
module provides the b64encode()
function, which is a simple way to perform base64 encoding. This function accepts binary data as input and returns a base64 encoded byte string.
Here’s a basic example:
import base64
data = b'Hello, World!'
encoded_data = base64.b64encode(data)
print(encoded_data)
# Output:
# b'SGVsbG8sIFdvcmxkIQ=='
In this example, we first import the base64
module. We then define a byte string data
that we want to encode. We pass this data to the b64encode()
function, which returns the base64 encoded version of the data. This encoded data is then printed out.
The advantage of using the b64encode()
function is its simplicity and directness. It’s a straightforward way to perform base64 encoding in Python.
However, there are some potential pitfalls to be aware of. One such pitfall is that the b64encode()
function requires binary data as input. If you try to pass a regular string, you will encounter a TypeError
. This is because the function expects a bytes-like object.
To avoid this issue, you can convert your string to bytes using the encode()
method, like this:
import base64
string_data = 'Hello, World!'
byte_data = string_data.encode('utf-8')
encoded_data = base64.b64encode(byte_data)
print(encoded_data)
# Output:
# b'SGVsbG8sIFdvcmxkIQ=='
In this example, we first convert the string to bytes using the encode()
method, and then pass the resulting bytes to the b64encode()
function. This successfully encodes the string in base64.
Handling Different Data Types in Base64 Encoding
As you delve deeper into base64 encoding in Python, you’ll encounter situations where you need to encode data types other than simple strings. This section will guide you on how to handle different data types while encoding in base64.
Encoding an Image File
Let’s consider an example where we have an image file that we want to encode in base64. Here’s how you can do it:
import base64
with open('image.jpg', 'rb') as image_file:
encoded_string = base64.b64encode(image_file.read())
print(encoded_string)
# Output:
# b'/9j/4AAQSkZJRgABAQEAAAAAAAD/2wBDAAMCAgMCAgMDAwMEAwMEBQgFBQQEBQoHBwYIDAoMDAsKCwsNDhIQDQ4RDgsLEBYQERMUFRUVDA8XGBYUGBIUFRT/2wBDAQMEBAUEBQkFBQkUDQsNFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBQUFBT/wAARCAJYAyADAREAAhEBAxEB/8QAHwAAAQUBAQEBAQEAAAAAAAAAAAECAwQFBgcICQoL/8QAtRAAAgEDAwIEAwUFBAQAAAF9AQIDAAQRBRIhMUEGE1FhByJxFDKBkaEII0KxwRVS0fAkM2JyggkKFhcYGRolJicoKSo0NTY3ODk6Q0RFRkdISUpTVFVWV1hZWmNkZWZnaGlqc3R1dnd4eXqDhIWGh4iJipKTlJWWl5iZmqKjpKWmp6ipqrKztLW2t7i5usLDxMXGx8jJytLT1NXW19jZ2uHi4+Tl5ufo6erx8vP09fb3+Pn6/8QAHwEAAwEBAQEBAQEBAQAAAAAAAAECAwQFBgcICQoL/8QAtREAAgECBAQDBAcFBAQAAQJ3AAECAxEEBSExBhJBUQdhcRMiMoEIFEKRobHBCSMzUvAVYnLRChYkNOEl8RcYGRomJygpKjU2Nzg5OkNERUZHSElKU1RVVldYWVpjZGVmZ2hpanN0dXZ3eHl6goOEhYaHiImKkpOUlZaXmJmaoqOkpaanqKmqsrO0tba3uLm6wsPExcbHyMnK0tPU1dbX2Nna4uPk5ebn6Onq8vP09fb3+Pn6/9oADAMBAAIRAxEAPwD9/oooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiigAooooAKKKKACiiig
In this example, we open the image file in binary mode using the open()
function with ‘rb’ as the second argument. We then read the contents of the image file using the read()
method. The b64encode()
function is then used to encode the binary data of the image file. The result is a base64 encoded byte string representing the image data.
Keep in mind that the resulting base64 string can be quite long for larger images. This is normal, as the base64 encoding is designed to represent binary data as a text string.
Encoding a JSON Object
Another common use case is encoding a JSON object. Here’s an example:
import base64
import json
# Define a JSON object
json_obj = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
# Convert the JSON object to a string
json_string = json.dumps(json_obj)
# Convert the string to bytes
byte_data = json_string.encode('utf-8')
# Encode the bytes
encoded_data = base64.b64encode(byte_data)
print(encoded_data)
# Output:
# b'eyJuYW1lIjogIkpvaG4gRG9lIiwgImFnZSI6IDMwLCAiY2l0eSI6ICJOZXcgWW9yayJ9'
In this example, we first define a JSON object. We then convert the JSON object to a string using the dumps()
function from the json
module. This string is then converted to bytes and passed to the b64encode()
function to be encoded.
As you can see, base64 encoding in Python is quite flexible and can handle a variety of data types. Whether you’re encoding strings, image files, or JSON objects, Python’s base64
module has got you covered.
Exploring Alternative Methods for Base64 Encoding
Python’s base64
module provides additional methods for base64 encoding, one of which is the urlsafe_b64encode()
function. This function behaves similarly to b64encode()
, but uses a set of characters that are safe for inclusion in URLs.
Using urlsafe_b64encode()
Here’s an example of how to use this function:
import base64
data = b'Hello, World!'
encoded_data = base64.urlsafe_b64encode(data)
print(encoded_data)
# Output:
# b'SGVsbG8sIFdvcmxkIQ=='
In this example, the urlsafe_b64encode()
function returns a base64 encoded string that is safe for use in URLs. This is useful when you need to include binary data in a URL, such as in a query string or path segment.
Comparing b64encode()
and urlsafe_b64encode()
Both b64encode()
and urlsafe_b64encode()
provide base64 encoding functionality, but they differ in the set of characters they use. The b64encode()
function uses the standard base64 alphabet, while urlsafe_b64encode()
uses a URL and filename safe base64 alphabet.
Here’s a comparison table of the two methods:
Method | Description | Use Case |
---|---|---|
b64encode() | Uses the standard base64 alphabet | General purpose base64 encoding |
urlsafe_b64encode() | Uses a URL and filename safe base64 alphabet | Base64 encoding for use in URLs |
Both methods have their own advantages and use cases. The b64encode()
function is suitable for general purpose base64 encoding, while urlsafe_b64encode()
is ideal when you need to include the encoded data in a URL.
In conclusion, Python’s base64
module offers a variety of methods for base64 encoding to cater to different needs. Whether you’re working with general binary data or need to include binary data in a URL, there’s a method that fits your requirements.
While Python’s base64
module is straightforward to use, you might encounter some common issues during encoding. Let’s discuss these potential problems and their solutions.
‘TypeError’ During Encoding
One common issue you might face is a TypeError
when trying to encode a regular string using the b64encode()
function. This is because b64encode()
expects a bytes-like object, not a string.
Here’s an example of this error:
import base64
data = 'Hello, World!'
encoded_data = base64.b64encode(data)
print(encoded_data)
# Output:
# TypeError: a bytes-like object is required, not 'str'
To fix this issue, you need to convert the string to bytes before encoding. You can do this using the encode()
method, like this:
import base64
data = 'Hello, World!'
byte_data = data.encode('utf-8')
encoded_data = base64.b64encode(byte_data)
print(encoded_data)
# Output:
# b'SGVsbG8sIFdvcmxkIQ=='
In this corrected example, we first convert the string to bytes using the encode()
method, and then pass the resulting bytes to the b64encode()
function. This successfully encodes the string in base64.
Issues with Different Data Types
Another issue you might encounter is when trying to encode data types other than strings or bytes. For instance, if you try to encode a list or a dictionary directly, you will encounter a TypeError
.
In such cases, you need to convert your data to a string or bytes before encoding. If you’re working with a JSON object, you can convert it to a string using the json.dumps()
function. If you’re working with other data types, you might need to use the str()
function or similar methods to convert your data to a string or bytes.
In conclusion, while base64 encoding in Python is generally straightforward, you need to be aware of the data types you’re working with and ensure they’re compatible with the b64encode()
function. By understanding these potential issues and their solutions, you can avoid common pitfalls and make your encoding tasks smoother and more efficient.
Understanding Python’s Base64 Module and Encoding
To effectively use base64 encoding in Python, it’s important to understand the underlying principles and why this process is crucial in data handling.
What is Base64 Encoding?
Base64 is a binary-to-text encoding scheme that represents binary data in an ASCII string format. This encoding helps to ensure that the data remains intact without modification during transport. Base64 is commonly used when there is a need to encode binary data, especially when that data needs to be stored and transferred over media designed for text.
Python’s Base64 Module
Python provides the base64
module, which is a handy tool for performing base64 encoding and decoding. This module provides functions to encode binary data to base64 encoded format and decode such encodings back to binary data.
Here’s a simple example of using the base64
module:
import base64
data = b'Hello, World!'
encoded_data = base64.b64encode(data)
print(encoded_data)
# Output:
# b'SGVsbG8sIFdvcmxkIQ=='
In this example, we first import the base64
module. We then define a byte string data
that we want to encode. We pass this data to the b64encode()
function, which returns the base64 encoded version of the data. This encoded data is then printed out.
Importance and Use Cases of Base64 Encoding
Base64 encoding is widely used in a variety of applications. It is commonly used when there is a need to encode binary data, especially when that data needs to be sent over email or used in other text fields. It’s also used in many web and internet protocols, and for encoding digital signatures and certificates.
In conclusion, understanding the fundamentals of base64 encoding and Python’s base64
module is crucial for handling binary data in Python. Whether you’re sending data over a network, storing it in a database, or securing it for authentication, base64 encoding is an essential tool in your Python programming toolkit.
Exploring the Impact of Base64 Encoding
Base64 encoding is not just a coding technique; it has significant implications in various areas of data handling and transmission. Let’s explore how base64 encoding is relevant beyond the basics of Python programming.
Base64 Encoding in Data Transmission
One of the key uses of base64 encoding is in the transmission of data over the internet. When you send data over the internet, it travels through various networks, each of which may handle data differently. To ensure that your data remains intact without modification during transport, encoding it in base64 is a common practice.
import base64
# Assume `data` is the information you want to transmit
data = b'Some important data'
# Encoding the data
encoded_data = base64.b64encode(data)
print(f'Transmitting data: {encoded_data}')
# Output:
# Transmitting data: b'U29tZSBpbXBvcnRhbnQgZGF0YQ=='
In this code block, we are encoding some hypothetical ‘important data’ that we wish to transmit. The base64 encoding converts our data into a format that can be safely sent over networks designed to handle text, ensuring our data arrives as intended.
Base64 Encoding and File Handling
Base64 encoding is also widely used in file handling. For example, when you need to store binary data in a text file or a database that only accepts text, base64 encoding comes to the rescue.
import base64
# Assume `binary_data` is some binary data retrieved from a file
binary_data = b'[non displayable characters]'
# Encoding the binary data
encoded_data = base64.b64encode(binary_data)
print(f'Storing data: {encoded_data}')
# Output:
# Storing data: b'AAEC'
In this example, we are encoding binary data that we intend to store. By encoding it in base64, we can safely store this data in a text file or a text-based database.
Further Resources for Mastering Base64 Encoding
To delve deeper into the world of base64 encoding in Python and related concepts, here are some resources:
- IOFlood’s Tutorial on JSON in Python explores JSON data security and best practices for safe data exchange.
Efficient JSON Data Parsing in Python explains how to extract and process data from JSON structures using Python.
Efficient XML Parsing with Python – Learn how to work with XML documents and extract relevant information.
Python’s Official Documentation on the
base64
Module provides a comprehensive overview of the module and its functions.Reading and Writing Files in Python – This article from Real Python provides an in-depth guide to reading and writing files in Python.
Applications of JSON in Python – Explore the broad range of applications for JSON within Python programming.
Mastering base64 encoding in Python opens up a world of possibilities for handling and transmitting data. By understanding and applying this powerful tool, you can take your Python programming skills to the next level.
Wrapping Up: Mastering Base64 Encoding in Python
In this comprehensive guide, we’ve delved into the world of base64 encoding in Python, a powerful tool for converting binary data into a format that can be safely transported over systems designed for text.
We began with the basics, learning how to use Python’s base64
module and the b64encode()
function to perform base64 encoding. We then ventured into more advanced territory, dealing with different data types, and even explored alternative methods, such as the urlsafe_b64encode()
function.
Along the way, we tackled common issues you might encounter when using base64 encoding, such as ‘TypeError’ and problems with different data types, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Description | Use Case |
---|---|---|
b64encode() | Uses the standard base64 alphabet | General purpose base64 encoding |
urlsafe_b64encode() | Uses a URL and filename safe base64 alphabet | Base64 encoding for use in URLs |
Whether you’re just starting out with base64 encoding or you’re looking to level up your data handling skills, we hope this guide has given you a deeper understanding of base64 encoding in Python and its capabilities.
With its balance of flexibility, power, and ease of use, base64 encoding is a crucial tool for handling binary data in Python. Happy coding!