Python XML Parser: Libraries and Modules Guide
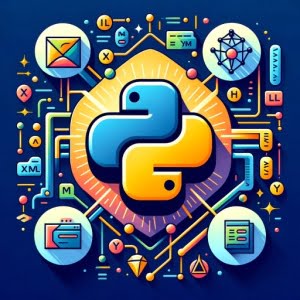
Are you finding it difficult to parse XML files in Python? You’re not alone. Many developers find themselves in a similar situation, but there’s a solution at hand.
Think of Python as a skilled linguist that can read and understand the language of XML. It can help you navigate the complexities of XML files and extract the data you need efficiently.
In this guide, we’ll walk you through how to parse XML files using Python. We’ll start with simple parsing tasks, like fetching data from XML documents, and gradually move into more complex arenas such as manipulating and transforming these files. Various strategies, alternative approaches and crucial best practices will also be discussed in detail.
So, let’s dive in and start mastering Python XML Parser!
TL;DR: How Do I Parse XML Files in Python?
To parse XML files in Python, you can use Python’s built-in
xml.etree.ElementTree
module. This module allows you to read XML files and extract the data you need.
Here’s a simple example:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
# Output:
# ('tag', {'attribute': 'value'})
In this example, we first import the xml.etree.ElementTree
module as ET
. We then parse the ‘example.xml’ file and get the root element of the XML document. Finally, we iterate over each child element in the root of the XML file and print the tag and attributes.
This is a basic way to parse XML files in Python, but there’s much more to learn about XML parsing. Continue reading for more detailed information and advanced usage examples.
Table of Contents
- Basic XML Parsing with Python
- Handling Complex XML Files with Python
- Exploring Alternative XML Parsing Methods
- Overcoming XML Parsing Challenges in Python
- Understanding XML and Its Structure
- The Relevance of XML Parsing in Python
- Further Resources for XML Parsing Mastery
- Wrapping Up: Mastering Python XML Parsing
Basic XML Parsing with Python
Python’s built-in xml.etree.ElementTree
module is a powerful tool for parsing XML files. This module treats an XML file as a tree of elements, hence the name ‘ElementTree’. Let’s delve into the basics of using this module.
The ElementTree Module
The xml.etree.ElementTree
module provides a simple and effective way to parse and create XML data. It’s a part of the Python Standard Library, so you don’t need to install anything to use it.
Here’s a basic example of how you might use the xml.etree.ElementTree
module to parse an XML file:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
# Output:
# ('tag1', {'attribute1': 'value1'})
# ('tag2', {'attribute2': 'value2'})
In this example, we first import the xml.etree.ElementTree
module as ET
. The ET.parse()
function reads the XML file and returns an ElementTree
object. We then get the root element of the XML document using the getroot()
method. Finally, we iterate over each child element in the root of the XML file and print the tag and attributes.
This is a simple and effective way to parse XML files in Python. However, it’s important to note that the xml.etree.ElementTree
module has its limitations. For instance, it doesn’t support XML namespaces, which can be a problem if you’re working with more complex XML files. In the next section, we will explore how to handle such complexities.
Handling Complex XML Files with Python
As you start working with more complex XML files, you’ll encounter new challenges. For instance, your XML file may include namespaces or mixed content. In this section, we’ll explore how to handle such complexities using Python’s xml.etree.ElementTree
module.
Parsing XML with Namespaces
XML namespaces are used to avoid element name conflicts in an XML file. They can be quite tricky to handle, but Python provides a way to deal with them.
Here’s an example of how you might parse an XML file with namespaces:
import xml.etree.ElementTree as ET
# Define the namespace
ns = {'real_person': 'http://people.example.com'}
# Parse the XML file
tree = ET.parse('example.xml')
root = tree.getroot()
# Find all 'neighbor' elements within the 'real_person' namespace
for neighbor in root.findall('real_person:neighbor', ns):
print(neighbor.attrib)
# Output:
# {'name': 'John Doe', 'house': '7'}
# {'name': 'Jane Doe', 'house': '8'}
In this example, we first define the namespace in a dictionary. We then parse the XML file and find all ‘neighbor’ elements within the ‘real_person’ namespace. The output is the attributes of each ‘neighbor’ element.
Parsing Mixed Content
Mixed content refers to XML elements that contain both text and child elements. Python’s xml.etree.ElementTree
module can handle mixed content, but it requires a bit more work.
Here’s an example:
import xml.etree.ElementTree as ET
tree = ET.parse('example.xml')
root = tree.getroot()
# Print the text content of each 'description' element
for description in root.iter('description'):
print(description.text)
# Output:
# 'This is a description.'
# 'This is another description.'
In this example, we parse the XML file and then use the iter()
method to find all ‘description’ elements. We then print the text content of each ‘description’ element.
While the xml.etree.ElementTree
module can handle complex XML files to some extent, there are other libraries available that offer more advanced features. We’ll explore some of these alternatives in the next section.
Exploring Alternative XML Parsing Methods
While Python’s built-in xml.etree.ElementTree
module is a powerful tool for parsing XML files, there are other libraries available that offer more advanced features. Two popular alternatives are the lxml
library and the xml.sax
module.
The lxml Library
The lxml
library is a third-party Python library that provides a more feature-rich and easy-to-use interface for XML parsing. It is compatible with both XPath 1.0 and XSLT 1.0, supports XML namespaces, and can handle large XML files efficiently.
Here’s an example of how you might use the lxml
library to parse an XML file:
from lxml import etree
tree = etree.parse('example.xml')
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
# Output:
# ('tag1', {'attribute1': 'value1'})
# ('tag2', {'attribute2': 'value2'})
In this example, we first import the etree
module from the lxml
library. The rest of the code is similar to the xml.etree.ElementTree
example, but lxml
provides additional features and better performance.
The xml.sax Module
The xml.sax
module is another part of the Python Standard Library that provides tools for parsing XML. Unlike xml.etree.ElementTree
, which uses a tree-based parsing approach, xml.sax
uses an event-driven parsing approach. This means it reads the XML file sequentially from start to end, triggering events as it encounters opening tags, closing tags, text content, and so on.
Here’s an example of how you might use the xml.sax
module to parse an XML file:
import xml.sax
class MyHandler(xml.sax.ContentHandler):
def startElement(self, name, attrs):
print('startElement', name)
def endElement(self, name):
print('endElement', name)
xml.sax.parse('example.xml', MyHandler())
# Output:
# startElement tag1
# endElement tag1
# startElement tag2
# endElement tag2
In this example, we first define a handler class that inherits from xml.sax.ContentHandler
and overrides the startElement
and endElement
methods. We then parse the XML file using xml.sax.parse()
, passing in the name of the XML file and an instance of our handler class.
Both lxml
and xml.sax
offer unique advantages and can be more suitable than xml.etree.ElementTree
depending on your needs. lxml
is feature-rich and efficient, but it’s a third-party library that needs to be installed separately. xml.sax
, on the other hand, is part of the Python Standard Library and uses an event-driven parsing approach that can be more efficient for large XML files.
Overcoming XML Parsing Challenges in Python
While Python offers several excellent tools for parsing XML files, you may still encounter some issues. In this section, we’ll discuss common problems such as ‘ParseError’ and namespace issues, and we’ll provide solutions and workarounds.
Handling ParseError
A ParseError
occurs when Python can’t parse the XML file. This could be due to a syntax error in the XML file or an encoding issue.
Here’s an example of how you might handle a ParseError
:
import xml.etree.ElementTree as ET
try:
tree = ET.parse('example.xml')
except ET.ParseError:
print('Failed to parse XML file')
# Output:
# Failed to parse XML file
In this example, we try to parse the XML file inside a try
block. If a ParseError
is raised, we catch it in the except
block and print a message.
Dealing with Namespace Issues
Namespaces can add complexity to XML files and make them more difficult to parse. However, Python provides ways to handle namespaces.
Here’s an example:
import xml.etree.ElementTree as ET
# Define the namespace
ns = {'real_person': 'http://people.example.com'}
# Parse the XML file
tree = ET.parse('example.xml')
root = tree.getroot()
# Find all 'neighbor' elements within the 'real_person' namespace
for neighbor in root.findall('real_person:neighbor', ns):
print(neighbor.attrib)
# Output:
# {'name': 'John Doe', 'house': '7'}
# {'name': 'Jane Doe', 'house': '8'}
In this example, we first define the namespace in a dictionary. We then parse the XML file and find all ‘neighbor’ elements within the ‘real_person’ namespace. The output is the attributes of each ‘neighbor’ element.
Remember, XML parsing can be complex, but Python provides powerful tools and libraries to simplify the process. Understanding these tools and how to troubleshoot common issues is key to becoming proficient in Python XML parsing.
Understanding XML and Its Structure
Before we delve deeper into Python XML parsing, it’s essential to understand XML and its structure. XML, or Extensible Markup Language, is a markup language used to encode data in a format that is both human-readable and machine-readable.
XML Tags and Attributes
XML documents are made up of elements, which are defined by tags. Each element can have attributes, which provide additional information about the element.
Here’s an example of an XML document:
<person>
<name type='first'>John</name>
<name type='last'>Doe</name>
<age>30</age>
<city>New York</city>
</person>
In this example, person
is an element defined by the person
tag. This element contains four child elements: name
, name
, age
, and city
. The name
elements have an attribute type
, which specifies whether the name is a first name or a last name.
XML Tree Structure
XML documents have a tree-like structure, with one root element and a number of child elements. This structure is why we often use tree-based parsers like xml.etree.ElementTree
to parse XML files.
Understanding the structure of XML documents is key to mastering XML parsing in Python. With this foundation, you can better understand how Python’s XML parsing libraries work and how to use them effectively.
The Relevance of XML Parsing in Python
XML parsing in Python is not just a standalone skill; it’s a crucial part of broader data extraction and web scraping techniques. When you master XML parsing, you can extract data from XML files or XML-based APIs, and use it in a wide range of applications.
XML Parsing in Data Extraction
Data extraction is the process of obtaining data from a database or SaaS platform so it can be replicated to a destination — such as a data warehouse — designed to support online analytical processing (OLAP). XML files are often used to store data in a structured manner, making XML parsing an essential skill for data extraction.
XML Parsing in Web Scraping
Web scraping is the process of extracting data from websites. Many websites use XML (or HTML, which has a similar structure to XML) to structure their data. By mastering XML parsing, you can extract this data and use it for a variety of purposes, from data analysis to machine learning.
Exploring Related Concepts
Once you’ve mastered XML parsing in Python, you might want to explore related concepts, such as HTML parsing and JSON parsing. HTML parsing allows you to extract data from websites, while JSON parsing allows you to work with JSON data, which is commonly used for APIs and config files.
Further Resources for XML Parsing Mastery
To amplify your proficiency with XML parsing, we highly encourage you to explore the following resources:
- Python JSON Best Practices – Explore JSON data loading and parsing from external sources.
Encoding Data in Python with base64 – A practical guide on base64 encoding and decoding in Python.
Python JSON Pretty Printing – Learn how to format and display JSON data beautifully in Python.
XML in Python – An introductory tutorial on DataCamp that provides a more in-depth look at XML parsing in Python.
Python XML with ElementTree: Beginner’s Guide walks you through the process of reading and writing XML files with Python.
XML Processing Modules – The official Python documentation provides information about the different XML processing modules in Python.
These resources are designed to bridge the gap between understanding and implementation, ultimately making you a more adept and efficient Python developer.
Wrapping Up: Mastering Python XML Parsing
In this comprehensive guide, we’ve explored the ins and outs of parsing XML files using Python. From the basics to more advanced techniques, we’ve covered a wide range of topics to help you understand and master Python XML parsing.
We started with the basics, learning how to use Python’s built-in xml.etree.ElementTree
module to parse XML files. We then moved on to more advanced topics, such as parsing XML files with namespaces and mixed content. We also explored how to handle common issues like ‘ParseError’ and namespace problems.
We didn’t stop there. We also looked at alternative methods for parsing XML files in Python, such as using the lxml
library and the xml.sax
module. These alternatives offer unique advantages and can be more suitable than xml.etree.ElementTree
depending on your needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
xml.etree.ElementTree | Simple, tree-based parsing | Doesn’t support XML namespaces |
lxml | Feature-rich, efficient | Third-party library, needs to be installed separately |
xml.sax | Event-driven parsing, efficient for large XML files | More complex to use |
Whether you’re a beginner just starting out with Python XML parsing or an experienced developer looking to deepen your knowledge, we hope this guide has been a valuable resource for you.
Python XML parsing is a powerful skill that can open up new possibilities for data extraction and processing. With the knowledge you’ve gained from this guide, you’re well-equipped to tackle any XML parsing task that comes your way. Happy coding!