Java Spring Framework Mastery: A Detailed Usage Guide
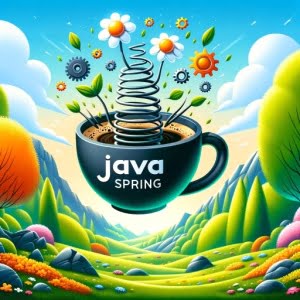
Are you finding it challenging to navigate the world of Java Spring? You’re not alone. Many developers find themselves in a maze when it comes to understanding and using this powerful framework. Think of Java Spring as a Swiss Army knife – a versatile tool that can simplify your Java development process and boost your productivity.
This guide will walk you through the basics to advanced usage of Java Spring, helping you to master this powerful tool. We’ll explore Java Spring’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java Spring!
TL;DR: What is Java Spring and Why is it Used?
Java Spring
is a powerful framework used for developing Java applications. It must be imported with,import org.springframework.boot.SpringApplication;
andimport org.springframework.boot.autoconfigure.SpringBootApplication;
It provides comprehensive infrastructure support, which reduces the need for manual configurations and boilerplate code. Here’s a simple example of a Spring application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
// Output:
// The application starts and listens for HTTP requests on port 8080.
In this example, we’ve created a basic Spring Boot application. The @SpringBootApplication
annotation tells Spring Boot that this is the main application class and the main
method uses Spring Boot’s SpringApplication.run()
method to launch the application.
This is just a basic introduction to Java Spring. There’s much more to learn about its capabilities, advanced features, and how to troubleshoot common issues. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Setting Up a Simple Java Spring Application
- Diving Deeper: Advanced Java Spring Usage
- Exploring Alternative Java Frameworks
- Navigating Java Spring: Common Issues and Solutions
- Understanding Java Spring and Its Architecture
- Expanding Horizons: Java Spring in Large-Scale Applications
- Wrapping Up: Java Spring Framework
Setting Up a Simple Java Spring Application
Let’s start with the basics. Setting up a simple Java Spring application is straightforward, especially with Spring Boot, which is a module that provides pre-configurations for rapid application development.
Here’s how you can set up a basic Java Spring application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
}
// Output:
// The application starts and listens for HTTP requests on port 8080.
In this example, the @SpringBootApplication
annotation is used. This is a convenience annotation that adds all of the following:
@Configuration
: Tags the class as a source of bean definitions for the application context.@EnableAutoConfiguration
: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.
The main
method uses Spring Boot’s SpringApplication.run()
method to launch the application. Once you run this application, it starts a server and listens for HTTP requests on port 8080.
Advantages of Using Java Spring
Java Spring simplifies the development process by providing a comprehensive programming and configuration model. It’s advantageous because it:
- Reduces the amount of boilerplate code.
- Provides a clean separation of concerns.
- Simplifies integration with other technologies.
Potential Pitfalls
While Java Spring is powerful, it’s not without its challenges. Some potential pitfalls include:
- It can be complex to learn due to its extensive features.
- Debugging can be difficult due to the high level of abstraction.
- It may be overkill for simple applications.
Despite these challenges, the benefits of Java Spring often outweigh the potential pitfalls, especially for larger, more complex applications.
Diving Deeper: Advanced Java Spring Usage
As you become more comfortable with Java Spring, you can start to leverage its more complex features. Let’s explore some of these advanced uses.
Spring Boot for Microservices
Spring Boot is a powerful tool for creating stand-alone, production-grade Spring-based applications. One of its many uses is in the creation of microservices. Here’s an example of how to create a simple microservice using Spring Boot:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class MicroserviceApplication {
@GetMapping("/hello")
public String hello() {
return "Hello from Microservice!";
}
public static void main(String[] args) {
SpringApplication.run(MicroserviceApplication.class, args);
}
}
// Output:
// When you access http://localhost:8080/hello, you see: 'Hello from Microservice!'
In this example, we’ve added a @RestController
annotation, which makes the class a controller able to handle HTTP requests. The @GetMapping("/hello")
annotation maps HTTP GET requests to the hello()
method. When you access the URL ‘http://localhost:8080/hello’, you will see the message ‘Hello from Microservice!’.
Spring Security for Authentication
Spring Security provides comprehensive security services for Java applications. It can help you add authentication and authorization to your Spring applications with minimal fuss.
Here’s an example of how to set up basic authentication with Spring Security:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@SpringBootApplication
@EnableWebSecurity
public class SecurityApplication extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
public static void main(String[] args) {
SpringApplication.run(SecurityApplication.class, args);
}
}
// Output:
// When you access the application, you are prompted for a username and password.
In this example, we’ve extended WebSecurityConfigurerAdapter
and overridden the configure(HttpSecurity http)
method to set up basic authentication. Any request to the application will now require authentication.
Exploring Alternative Java Frameworks
While Java Spring is a powerful and versatile tool, it’s not the only Java framework available. Let’s explore a couple of alternatives: Struts and Hibernate.
Apache Struts: A Robust MVC Framework
Apache Struts is a free, open-source framework for creating enterprise-ready Java web applications. It uses a Model-View-Controller (MVC) architecture, which is helpful in designing large-scale applications due to its clear separation of concerns.
// A basic Struts action class
import com.opensymphony.xwork2.ActionSupport;
public class HelloWorldAction extends ActionSupport {
public String execute() {
return SUCCESS;
}
}
// Output:
// This action class will return a 'SUCCESS' result that maps to a view to be rendered.
In the above code, we’ve created a simple Struts action class. When the execute()
method is called, it returns a string that corresponds to a result in the Struts configuration file. This result maps to a view that will be rendered to the user.
Hibernate: Simplifying Database Operations
Hibernate is another Java framework that simplifies database operations. It’s a powerful Object-Relational Mapping (ORM) tool that lets you develop persistent classes following common Java idiom. Here’s a basic example of a Hibernate entity class:
@Entity
@Table(name = "EMPLOYEE")
public class Employee {
@Id @GeneratedValue
@Column(name = "id")
private int id;
@Column(name = "first_name")
private String firstName;
// getters and setters omitted for brevity
}
// Output:
// This class maps to the 'EMPLOYEE' table in the database.
In the above code, we’ve created a simple Hibernate entity class. The @Entity
annotation marks the class as a Hibernate entity (i.e., a persistent object). The @Table
annotation maps this entity to the ‘EMPLOYEE’ table in the database.
Comparing Struts, Hibernate, and Java Spring
While all three frameworks have their strengths, they each have different use cases:
- Struts is excellent for building large-scale applications thanks to its MVC architecture. However, it might be overkill for simple applications.
Hibernate shines when it comes to working with databases. It simplifies database operations, but it doesn’t provide the breadth of features that Spring does.
Java Spring is a versatile all-rounder. It can handle a wide range of tasks, from web application development to security, making it a one-stop-shop for many developers.
Choosing the right framework depends on your specific needs and the nature of your project.
While Java Spring is a powerful tool, it’s not without its challenges. Let’s explore some common issues you might encounter and their solutions.
Configuration Issues
One of the most common issues with Java Spring is configuration problems. These can occur due to incorrect or missing configuration settings. For example, you might forget to enable component scanning, causing Spring to overlook your beans.
@Configuration
@ComponentScan("com.example.myapp")
public class AppConfig {
}
// Output:
// Spring will scan the 'com.example.myapp' package for components.
In the above code, we’ve used the @ComponentScan
annotation to tell Spring to scan the ‘com.example.myapp’ package for components. Without this, Spring wouldn’t be aware of your components, leading to configuration issues.
Dependency Problems
Another common issue is problems with dependencies. This can happen if you have conflicting versions of the same dependency in your project. One way to troubleshoot this is to use the Maven Dependency Plugin to generate a dependency tree.
mvn dependency:tree
// Output:
// A tree of dependencies is printed to the console.
Running the above command will print a tree of dependencies to the console, allowing you to identify any conflicting versions.
Remember, troubleshooting is a common part of the development process. Don’t be discouraged by these challenges. Instead, view them as opportunities to deepen your understanding of Java Spring and improve your problem-solving skills.
Understanding Java Spring and Its Architecture
Java Spring is a powerful, feature-rich framework designed to streamline the development of Java applications. It provides a comprehensive infrastructure that simplifies many aspects of application development, allowing developers to focus on writing their business logic rather than boilerplate code.
Key Components of Java Spring
Java Spring is composed of several modules, each providing specific functionality. Here are a few key components:
- Spring MVC: This module provides a robust Model-View-Controller architecture for building web applications. It simplifies the development of web interfaces, handling everything from routing requests to rendering views.
Spring Boot: This is a convention-over-configuration solution for creating stand-alone, production-grade applications. It simplifies the setup process, allowing developers to get started quickly with minimal configuration.
Spring Security: This module provides comprehensive security features for Java applications, including authentication, authorization, and protection against common security threats.
Each of these components plays a crucial role in the functionality of the Spring framework and contributes to its versatility and power.
Here’s a simple example of a Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
// Output:
// The application starts and listens for HTTP requests on port 8080.
In this example, the @SpringBootApplication
annotation tells Spring Boot that this is the main application class. The main
method uses Spring Boot’s SpringApplication.run()
method to launch the application.
The Principle of Dependency Injection
One of the core principles of Java Spring is Dependency Injection (DI). DI is a design pattern that allows for loose coupling between components. Instead of a component creating its dependencies, they are injected into it by an external entity (the Spring container in this case).
This approach has several benefits, including improved testability, better separation of concerns, and easier configuration. Here’s a simple example of DI in action:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
private final MyDependency myDependency;
@Autowired
public MyComponent(MyDependency myDependency) {
this.myDependency = myDependency;
}
}
// Output:
// Spring will automatically wire an instance of 'MyDependency' into 'MyComponent'.
In the above code, we’ve created a class MyComponent
that depends on MyDependency
. Instead of MyComponent
creating an instance of MyDependency
, it’s injected into it via the constructor. The @Autowired
annotation tells Spring to handle this injection.
Understanding these fundamentals of Java Spring is crucial to using the framework effectively. As you continue to explore Java Spring, you’ll discover even more powerful features and techniques.
Expanding Horizons: Java Spring in Large-Scale Applications
Java Spring isn’t just for small applications. It’s robust enough to handle large-scale applications and microservices, making it a versatile tool in any Java developer’s toolkit.
Java Spring and Microservices
Java Spring, and in particular Spring Boot, provides a robust foundation for building microservices. It offers features like easy database access, security, and auto-configuration, which simplifies the microservices development process.
Here’s an example of a simple microservice using Spring Boot:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class MicroserviceApplication {
@GetMapping("/hello")
public String hello() {
return "Hello from Microservice!";
}
public static void main(String[] args) {
SpringApplication.run(MicroserviceApplication.class, args);
}
}
// Output:
// When you access http://localhost:8080/hello, you see: 'Hello from Microservice!'
In this example, we’ve created a simple microservice that responds with a greeting when accessed at the ‘/hello’ endpoint.
Exploring Related Concepts: RESTful APIs and Spring Data
Java Spring also integrates well with other important concepts in modern web development, such as RESTful APIs and Spring Data.
RESTful APIs allow for easy communication between different parts of a web application or between different applications entirely. Spring’s @RestController
and @RequestMapping
annotations make building these APIs a breeze.
Spring Data simplifies database access, reducing the amount of boilerplate code you need to write. It offers powerful repository and custom query abstraction capabilities that can save you a significant amount of development time.
Further Resources for Mastering Java Spring
If you’re interested in learning more about Java Spring, here are a few resources that you might find helpful:
- Beginner’s Guide to Java Spring Boot – Explore Spring Boot’s auto-configuration feature.
Exploring Spring Cloud Gateway Features – Master configuration for resilient microservices systems
Understanding Spring Boot Starter in Depth – Master Spring Boot starter configuration for rapid development
Spring Framework Documentation is a comprehensive resource covering everything from the basics to advanced topics.
Baeldung’s Spring Guides offers a wide range of tutorials and guides on various Spring topics.
DigitalOcean’s Spring Boot Tutorial covers the basics of Spring Boot, making it a great starting point to the framework.
Remember, mastering a tool like Java Spring takes time and practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy coding!
Wrapping Up: Java Spring Framework
In this comprehensive guide, we’ve delved into the world of Java Spring, a robust and versatile framework for developing Java applications. From basic setup to advanced usage, we’ve covered the breadth of what Java Spring has to offer, providing you with the tools to master this powerful framework.
We began with setting up a simple Java Spring application, where we learned about the basics of the framework and its advantages. We then ventured into more advanced territory, exploring how to create microservices with Spring Boot and set up authentication with Spring Security. Along the way, we tackled common challenges that you might encounter when using Java Spring, such as configuration issues and dependency problems, offering solutions and workarounds for each issue.
We also looked at alternative approaches to Java development, comparing Java Spring with other frameworks like Struts and Hibernate. Here’s a quick comparison of these frameworks:
Framework | Versatility | Learning Curve | Use Case |
---|---|---|---|
Java Spring | High | Moderate | Comprehensive solution for many applications |
Apache Struts | Moderate | High | Best for large-scale applications with MVC architecture |
Hibernate | Moderate | Low | Ideal for database operations |
Whether you’re just starting out with Java Spring or you’re looking to level up your Java development skills, we hope this guide has given you a deeper understanding of Java Spring and its capabilities.
With its balance of versatility, power, and scalability, Java Spring is a valuable tool for any Java developer. Now, you’re well equipped to harness its potential. Happy coding!