Spring Cloud Gateway: From Setup to Advanced Use
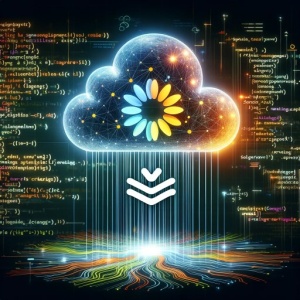
Are you finding it challenging to manage your microservices? You’re not alone. Many developers find themselves in a similar situation, but there’s a tool that can make this process a breeze.
Think of Spring Cloud Gateway as a skilled traffic controller, efficiently managing your microservices and ensuring smooth operation. It’s a powerful tool that can greatly simplify your work and improve your application’s performance.
This guide will walk you through the basics to advanced techniques of using Spring Cloud Gateway. We’ll explore its core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Spring Cloud Gateway!
TL;DR: What is Spring Cloud Gateway?
Spring Cloud Gateway
is a library provided by the Spring framework that acts as an API gateway for microservices. It can be ran with a simple line,SpringApplication.run(GatewayApplication.class, args);
, however its configuration can differ. It’s a powerful tool that helps manage and route requests in a microservices architecture.
Here’s a basic example of how to set it up:
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
In this example, we’ve only created a simple Spring Boot application. This is the first step in setting up your Spring Cloud Gateway.
But there’s much more to Spring Cloud Gateway than just setting it up. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Spring Cloud Gateway: Setting Up and Creating Routes
- Advanced Spring Cloud Gateway Configurations
- Exploring Alternative API Gateway Solutions
- Troubleshooting Common Spring Cloud Gateway Issues
- Understanding API Gateways and Microservices Architecture
- Spring Cloud Gateway: Beyond Basic Use
- Wrapping Up: Spring Cloud Gateway
Spring Cloud Gateway: Setting Up and Creating Routes
For beginners, setting up and creating routes in Spring Cloud Gateway is a crucial first step. Let’s start by setting up a basic Spring Cloud Gateway application.
@SpringBootApplication
@EnableDiscoveryClient
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
In this code snippet, we’re creating a simple Spring Boot application and enabling it as a Discovery Client. This is the foundation of our Spring Cloud Gateway application.
Creating Routes
Routes are the essence of Spring Cloud Gateway. They define the path that a request will take through your system. Here’s a simple example of how to create a route:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route(r -> r.path("/testpath/**")
.uri("http://localhost:8080"))
.build();
}
This code creates a route that directs all requests from /testpath/
to http://localhost:8080
.
Basic Configuration
Configuration in Spring Cloud Gateway is straightforward. You can define routes and filters directly in your application.yml
or application.properties
file. Here’s an example:
spring:
cloud:
gateway:
routes:
- id: test_route
uri: http://localhost:8080
predicates:
- Path=/testpath/**
This configuration does the same as our previous code example: it routes all requests from /testpath/
to http://localhost:8080
.
Using Spring Cloud Gateway offers several advantages. It simplifies the management of microservices, provides powerful routing capabilities, and integrates well with other Spring projects. However, it’s essential to understand its potential pitfalls, such as complexities in handling WebSocket routes, and the need for careful management of filters and predicates.
Advanced Spring Cloud Gateway Configurations
As you become more comfortable with Spring Cloud Gateway, you can start to explore its more advanced features. These include setting up filters, global filters, load balancing, and circuit breaking.
Setting Up Filters
Filters allow you to modify requests and responses before they are sent to or returned from the route destination. Below is an example of a simple filter that adds a request header:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route(r -> r.path("/testpath/**")
.filters(f -> f.addRequestHeader("Hello", "World"))
.uri("http://localhost:8080"))
.build();
}
In this code, we’ve added a filter that appends a ‘Hello: World’ header to all requests going to http://localhost:8080
.
Global Filters
Global filters apply to all routes in your application. They are useful for applying common behavior across all your routes. Here’s how to set up a global filter:
@Bean
public GlobalFilter customGlobalFilter() {
return (exchange, chain) -> {
exchange.getRequest().mutate().header("fake-header", "fake-value");
return chain.filter(exchange);
};
}
In this code, we’ve created a global filter that adds a ‘fake-header: fake-value’ to all requests.
Load Balancing and Circuit Breaking
Spring Cloud Gateway integrates well with Spring Cloud LoadBalancer for client-side load balancing and with Resilience4J for circuit breaking. Here’s a simple example of a load balanced route:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route(r -> r.path("/testpath/**")
.uri("lb://my-service"))
.build();
}
In this code, we’ve created a route that directs requests to the my-service
service using client-side load balancing.
Circuit breaking, on the other hand, allows your application to elegantly handle failures. If a service is down or responding slowly, the circuit breaker can reroute requests to a fallback service or send an error response. This can be configured using Spring Cloud Circuit Breaker and Resilience4J.
These advanced features of Spring Cloud Gateway provide developers with powerful tools for managing microservices. By understanding and utilizing these features, you can build robust and efficient applications.
Exploring Alternative API Gateway Solutions
Spring Cloud Gateway is a powerful tool, but it’s not the only API gateway solution available. Two other popular options are Zuul and Nginx.
Zuul: A Legacy Choice
Zuul, like Spring Cloud Gateway, is a project from the Spring Cloud ecosystem. It was the original API gateway for Spring microservices. However, it’s no longer in active development and has been superseded by Spring Cloud Gateway.
@EnableZuulProxy
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
This is a simple setup for a Zuul API Gateway. As you can see, the setup is similar to that of Spring Cloud Gateway, but it lacks some of the more advanced features of its successor.
Nginx: A Powerful Option
Nginx is a popular open-source web server that can also function as an API gateway. It’s known for its high performance and flexibility.
server {
listen 80;
location / {
proxy_pass http://my_upstream;
}
}
This is a basic Nginx configuration that routes incoming requests to an upstream server. Nginx configurations can get quite complex, but they offer a great deal of flexibility.
Comparing the Options
Spring Cloud Gateway, Zuul, and Nginx each have their advantages and disadvantages.
- Spring Cloud Gateway is a modern, flexible, and feature-rich solution. It’s integrated with the Spring ecosystem, making it a great choice for Spring-based microservices.
Zuul is a legacy choice that’s no longer in active development. It’s simpler than Spring Cloud Gateway, but it lacks some features.
Nginx is a powerful and high-performance solution. It’s not specific to the Spring ecosystem, making it a versatile choice for any web application.
Choosing the right API gateway depends on your specific needs and the technologies you’re already using. Each of these options can be a good choice in the right circumstances.
Troubleshooting Common Spring Cloud Gateway Issues
Even with the best setup and configuration, you might encounter issues while using Spring Cloud Gateway. Let’s discuss some common problems and how to solve them.
Routing Issues
One of the most common issues with Spring Cloud Gateway is routing problems. For instance, you might have a misconfigured route that’s causing requests to go to the wrong place. Here’s an example of a misconfigured route:
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route(r -> r.path("/wrongpath/**")
.uri("http://localhost:8080"))
.build();
}
In this example, the route is configured to send requests from /wrongpath/
to http://localhost:8080
. If you intended to route from /testpath/
, this would be a problem. The solution is to correct the path in your route configuration.
Filter Problems
Another common issue is filter problems. Filters are a powerful feature of Spring Cloud Gateway, but they can also cause issues if not used correctly.
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route(r -> r.path("/testpath/**")
.filters(f -> f.addRequestHeader("Hello", ""))
.uri("http://localhost:8080"))
.build();
}
In this example, we’ve added a filter that’s supposed to add a ‘Hello’ header to all requests, but we’ve left the value empty. This could cause issues depending on how your application handles headers. The solution is to ensure that all filters are correctly configured and that they’re adding value to your application.
Other Considerations
There are other considerations to keep in mind when using Spring Cloud Gateway. For example, it’s important to understand that Spring Cloud Gateway operates on the application layer of the OSI model (Layer 7). This means it can handle any protocol that uses HTTP as a transport layer, but it might not be the best choice for lower-level protocols.
In conclusion, while Spring Cloud Gateway is a powerful tool for managing microservices, it’s essential to understand its common pitfalls and how to avoid them. With careful configuration and a good understanding of its features, you can avoid most issues and use Spring Cloud Gateway to its full potential.
Understanding API Gateways and Microservices Architecture
Before we delve deeper into the functionalities of Spring Cloud Gateway, it’s important to understand the concept of API gateways and microservices architecture.
The Concept of API Gateways
An API Gateway acts as a single entry point into a system allowing multiple APIs or microservices to act cohesively and provide a uniform experience to the user. It’s like a traffic cop, directing requests to the appropriate microservice.
# Example of a request going through an API Gateway
GET http://api.example.com/orders/12345
In this example, the request is made to the API Gateway, which then routes the request to the appropriate microservice.
Microservices Architecture: A Brief Overview
Microservices architecture is a design approach to build a single application as a suite of small services, each running in its own process and communicating with lightweight mechanisms, often an HTTP resource API. Each service is fully independent and can be updated, deployed, and scaled to meet demand for specific functions it provides.
# Example of services in a microservices architecture
GET http://orders.example.com/12345
GET http://users.example.com/67890
In this example, each request is made to a separate microservice, each with its own domain and purpose.
Spring Cloud Gateway in the Picture
Spring Cloud Gateway fits into this picture as an API Gateway implementation. It provides a simple, yet effective way to route traffic to the appropriate microservice. It’s built on Spring 5, Project Reactor, and Spring Boot 2, which means it benefits from the ecosystem’s non-blocking model.
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route(r -> r.path("/testpath/**")
.uri("http://localhost:8080"))
.build();
}
# Output:
# A route from /testpath/** to http://localhost:8080
In this example, Spring Cloud Gateway is set up to route traffic from /testpath/**
to http://localhost:8080
. This is a simple example of how Spring Cloud Gateway can manage traffic in a microservices architecture.
Understanding these fundamental concepts is key to mastering the use of Spring Cloud Gateway and effectively managing your microservices.
Spring Cloud Gateway: Beyond Basic Use
As you become more proficient with Spring Cloud Gateway, you might start to wonder about its relevance in large-scale applications, its role in cloud-native development, and its integration with other Spring Cloud components.
Spring Cloud Gateway in Large-Scale Applications
Spring Cloud Gateway shines in large-scale applications. Its non-blocking API and support for WebSockets make it a great choice for applications that need to handle a large number of concurrent connections. Plus, its integration with Spring Cloud’s DiscoveryClient means it can automatically update its routes as services are added or removed from your architecture.
Cloud-Native Development with Spring Cloud Gateway
Spring Cloud Gateway is a natural fit for cloud-native development. It’s built on the reactive stack, which is designed for building high-performance, scalable applications. With its support for route predicates and filters, it provides a powerful way to control how traffic flows through your application.
Integration with Other Spring Cloud Components
Spring Cloud Gateway integrates seamlessly with other Spring Cloud components. For example, with Spring Cloud DiscoveryClient, it can automatically discover services and create routes for them. It also integrates with Spring Cloud LoadBalancer for client-side load balancing and with Spring Cloud Circuit Breaker for resilience patterns.
Further Resources for Spring Cloud Gateways
To deepen your understanding of Spring Cloud Gateway, consider exploring these resources:
- Java Spring Boot Tutorial: Getting Started – Master Spring Boot’s embedded server capabilities.
Understanding Spring Bean Configuration – Learn to define beans for dependency injection
Exploring Java Spring Framework Components – Master Spring for robust and scalable applications.
Spring Cloud Gateway Reference Guide covers everything from basic setup to advanced features.
Baeldung’s Guide to Spring Cloud Gateway covers many aspects of using Spring Cloud Gateway, from setting up routes to handling errors.
Spring Cloud Gateway on GitHub – The official GitHub repository is a great place to find example code and see what’s coming in future releases.
By understanding these advanced topics and leveraging the resources available, you can truly master Spring Cloud Gateway and use it to its full potential in your applications.
Wrapping Up: Spring Cloud Gateway
In this comprehensive guide, we’ve navigated the complexities of Spring Cloud Gateway, a robust API gateway for microservices. We’ve explored its functionalities, from basic setup to advanced configurations, and discussed how it fits into the broader picture of microservices architecture.
We began with the basics, learning how to set up Spring Cloud Gateway and create routes. We then delved into more advanced topics, such as setting up filters, global filters, load balancing, and circuit breaking. Along the way, we tackled common challenges you might encounter when using Spring Cloud Gateway, such as routing issues and filter problems, providing you with solutions and workarounds for each issue.
We also considered alternative approaches to handle API gateway needs, comparing Spring Cloud Gateway with other popular options like Zuul and Nginx. Here’s a quick comparison of these options:
Gateway | Flexibility | Ecosystem Integration | Active Development |
---|---|---|---|
Spring Cloud Gateway | High | High | Yes |
Zuul | Moderate | High | No |
Nginx | High | Low | Yes |
Whether you’re just starting out with Spring Cloud Gateway or you’re looking to level up your microservices management skills, we hope this guide has given you a deeper understanding of Spring Cloud Gateway and its capabilities.
With its balance of flexibility, ecosystem integration, and active development, Spring Cloud Gateway is a powerful tool for managing microservices. Happy coding!