Spring Bean Guide | Usage, Syntax, Examples
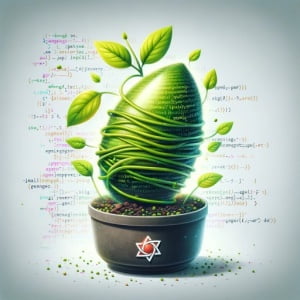
Are you finding it challenging to work with Spring Beans? You’re not alone. Many developers find themselves puzzled when it comes to understanding and managing Spring Beans, but we’re here to help.
Think of Spring Beans as the gears in a machine – they are the fundamental building blocks of any Spring application, powering the core functionality and features.
In this guide, we’ll take you through the journey of understanding, creating, and managing Spring Beans. We’ll cover everything from the basics of Spring Beans to more advanced techniques, as well as alternative approaches.
Let’s get started and master Spring Beans!
TL;DR: What is a Spring Bean?
A
Spring Bean
is an object that is instantiated, assembled, and managed by the Spring IoC (Inversion of Control) container, with the syntax<bean id='myBean' class='com.example.MyClass'/>
. It’s a fundamental part of the Spring Framework, acting as the building block of your application.
Here’s a simple example of a Spring Bean defined in an XML configuration file:
<bean id='myBean' class='com.example.MyClass'/>
In this example, we define a Spring Bean with the id ‘myBean’ and the class ‘com.example.MyClass’. This bean is now ready to be managed by the Spring IoC container, which takes care of its lifecycle and dependencies.
This is just the tip of the iceberg when it comes to Spring Beans. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Defining and Using Spring Beans: A Beginner’s Guide
One of the most common ways to define a Spring Bean is through XML-based configuration. This method is straightforward and widely used, making it an excellent starting point for beginners.
Let’s take a look at a simple example of defining a Spring Bean using XML configuration:
<beans xmlns='http://www.springframework.org/schema/beans'
xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance'
xsi:schemaLocation='http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd'>
<bean id='myBean' class='com.example.MyClass'/>
</beans>
In this XML file, we define a Spring Bean with the id ‘myBean’ and the class ‘com.example.MyClass’. The ‘id’ attribute is used to uniquely identify the bean in the Spring IoC container, while the ‘class’ attribute indicates the fully qualified name of the bean’s class.
Once the bean is defined, it can be used in your application like this:
ApplicationContext context = new ClassPathXmlApplicationContext('beans.xml');
MyClass myBean = (MyClass) context.getBean('myBean');
myBean.doSomething();
Here, we first create an ApplicationContext
by loading the beans.xml
file. Then, we retrieve our bean from the context using its id and cast it to the appropriate type (MyClass
in this case). Finally, we can call methods on our bean like doSomething()
.
This approach of defining and using Spring Beans offers several advantages. For one, it promotes loose coupling and high cohesion by managing object dependencies for you. It also provides a centralized way to configure your beans, making your code cleaner and easier to maintain.
However, it’s not without potential pitfalls. XML configuration can become verbose and difficult to manage as the number of beans grows. Also, since the wiring is done at runtime, errors in configuration may not be detected until the application is started.
Delving Deeper into Spring Beans
As you become more comfortable with Spring Beans, it’s time to explore some of the more complex aspects. Two vital concepts to grasp are bean scopes and bean lifecycle callbacks.
Understanding Bean Scopes
Bean scopes determine the lifecycle and visibility of a Spring Bean. Spring provides several scopes, but the most commonly used are ‘singleton’ and ‘prototype’.
- Singleton: This is the default scope. The Spring IoC container creates exactly one instance of the bean. It’s shared and used by all components that need it.
Prototype: A new instance is created every time the bean is requested.
Here’s how you can define the scope of a bean in XML configuration:
<bean id='myBean' class='com.example.MyClass' scope='prototype'/>
In this example, we specify that ‘myBean’ should have a ‘prototype’ scope, meaning a new instance will be created every time it’s requested.
Exploring Bean Lifecycle Callbacks
Spring Beans have a lifecycle that you can hook into to perform certain actions upon bean initialization and destruction. You can define init-method
and destroy-method
callbacks in your bean configuration like this:
<bean id='myBean' class='com.example.MyClass' init-method='init' destroy-method='cleanup'/>
In this example, the init
method will be called after the bean is instantiated and dependencies are injected, and the cleanup
method will be called before the bean is destroyed.
Here’s what the MyClass
might look like:
public class MyClass {
public void init() {
System.out.println('Bean is going through init.');
}
public void cleanup() {
System.out.println('Bean will be destroyed now.');
}
}
When you run your application, you’ll see the following output:
# Output:
# 'Bean is going through init.'
And when you close your application context (or shut down your application), you’ll see:
# Output:
# 'Bean will be destroyed now.'
Understanding bean scopes and lifecycle callbacks allows you to manage your Spring Beans more effectively and can lead to cleaner and more efficient code.
Alternative Ways to Define and Use Spring Beans
As you progress in your journey with Spring Beans, you’ll likely encounter situations where XML-based configuration might not be the most efficient or practical approach. Thankfully, Spring offers alternative methods to define and use Spring Beans, namely Java-based configuration and annotation-based configuration.
Java-Based Configuration
In Java-based configuration, you define your beans directly in your Java code. This approach is more type-safe and refactoring-friendly compared to XML configuration.
Here’s an example of how to define a Spring Bean using Java-based configuration:
@Configuration
public class AppConfig {
@Bean
public MyClass myBean() {
return new MyClass();
}
}
In this example, we use the @Configuration
annotation to indicate that this class will define beans. We then use the @Bean
annotation to define ‘myBean’.
Annotation-Based Configuration
Annotation-based configuration allows you to define beans directly in the classes that will be managed by the Spring IoC container. This approach can lead to more straightforward and self-contained code.
Here’s an example of how to define a Spring Bean using annotation-based configuration:
@Component
public class MyClass {
// ...
}
In this example, we use the @Component
annotation to indicate that this class is a bean and should be managed by the Spring IoC container.
Both Java-based and annotation-based configurations offer their own benefits and drawbacks. Java-based configuration is more explicit and clear but can become verbose for large applications. Annotation-based configuration is more concise and intuitive but can lead to scattered and hard-to-track configurations.
The decision of which approach to use ultimately depends on your specific use case and personal preference. It’s crucial to understand all these methods to make an informed decision and write efficient and maintainable Spring applications.
Troubleshooting Common Spring Bean Issues
While working with Spring Beans, you may encounter some common issues. Understanding these problems and their solutions can save you a lot of time and frustration. Let’s discuss some frequent challenges and their workarounds.
Bean Wiring Issues
One common problem is bean wiring issues, which occur when Spring is unable to resolve dependencies between beans. This can happen due to various reasons such as a missing bean definition or a typo in the bean id.
Here’s an example of a bean wiring issue:
ApplicationContext context = new ClassPathXmlApplicationContext('beans.xml');
MyClass myBean = (MyClass) context.getBean('nonExistentBean');
This code will throw a NoSuchBeanDefinitionException
because there’s no bean with the id ‘nonExistentBean’ defined in ‘beans.xml’.
To solve this issue, ensure that all your beans are properly defined and that you’re using the correct ids when retrieving them.
Scope-Related Problems
Another common issue is related to bean scopes. For example, if you define a bean as ‘prototype’ but use it as if it were a ‘singleton’, this can lead to unexpected behavior.
To avoid scope-related problems, make sure you understand the differences between ‘singleton’ and ‘prototype’ scopes, and use them appropriately.
Remember, ‘singleton’ beans are shared and reused, while ‘prototype’ beans are recreated every time they’re requested.
While these are some of the common issues, remember that every problem is an opportunity to learn more about Spring Beans and the Spring Framework. Happy troubleshooting!
Spring Framework and Inversion of Control (IoC)
To truly master Spring Beans, it’s crucial to understand the underlying principles of the Spring Framework and the concept of Inversion of Control (IoC).
Unraveling the Spring Framework
The Spring Framework is a comprehensive platform for building Java applications. It provides a wide array of features such as dependency injection, data access, transaction management, and more. At the heart of these features is the Spring IoC container, which manages the lifecycle and configuration of Spring Beans.
Demystifying Inversion of Control (IoC)
Inversion of Control is a design principle where the control flow of a program is inverted: instead of the programmer controlling the flow of a program, the external framework (in this case, Spring) takes control.
In the context of Spring, IoC means that the Spring Framework creates and manages beans. This is a departure from traditional Java development, where you’d instantiate and manage your own objects.
// Traditional Java development
MyClass myObject = new MyClass();
// Spring IoC
MyClass myBean = context.getBean(MyClass.class);
In the first example, you’re in control: you create and manage myObject
. In the second example, Spring’s IoC container is in control: it creates and manages myBean
.
Understanding the Spring Framework and IoC provides a solid foundation for working with Spring Beans. It helps you comprehend why beans are created and managed the way they are, leading to more effective and efficient Spring development.
The Role of Spring Beans in Larger Applications
Spring Beans are not isolated entities. They play a crucial role in larger Spring applications, including web applications and microservices.
Spring Beans in Web Applications
In a Spring web application, Spring Beans can be used to manage services, repositories, and controllers. They can be injected into other beans, such as controllers, to provide services like data access and business logic.
@Controller
public class MyController {
@Autowired
private MyService myService;
// ...
}
In this example, MyService
is a Spring Bean that is auto-wired into MyController
, another Spring Bean. This allows MyController
to use the services provided by MyService
.
Spring Beans in Microservices
In a microservice architecture, each microservice can be a separate Spring application with its own set of Spring Beans. These beans can be used to manage everything from business logic and data access to communication with other microservices.
Expanding Your Knowledge: Spring MVC, Spring Boot, and More
To fully leverage the power of Spring Beans, it’s beneficial to explore related concepts like Spring MVC and Spring Boot.
- Spring MVC: This is a framework for building web applications. It uses Spring Beans to manage controllers, services, and more.
Spring Boot: This is a platform that simplifies the setup of Spring applications. It provides default configurations and takes care of boilerplate code, allowing you to focus on your business logic (which can be managed by Spring Beans).
Further Resources for Mastering Spring Beans
Ready to delve deeper into Spring Beans and related concepts? Here are some resources that can help:
- Strengthening Your Java Spring Boot Skills – Reduce XML config with Spring Boot’s annotations.
Understanding the Differences: Spring vs Spring Boot – Learn about setup, configuration, and ease of use distinctions.
Spring Cloud Gateway: Overview – Discover Spring Cloud Gateway for API gateways.
Spring Framework Documentation: The official Spring Framework documentation.
Baeldung’s Guide to Spring Beans provides a detailed overview of Spring Beans, including their definition, configuration, scopes, and lifecycle.
DigitalOcean’s Spring Tutorial covers various aspects of Spring, including Spring Beans.
Wrapping Up: Spring Beans
In this comprehensive guide, we’ve explored the ins and outs of Spring Beans, the fundamental building blocks of any Spring application. From their basic use to more advanced techniques, we’ve covered the gamut of what you need to know about Spring Beans.
We began with the basics, understanding what a Spring Bean is and how to define and use it using XML-based configuration. We then delved into more complex aspects of Spring Beans, such as bean scopes and lifecycle callbacks. We also introduced alternative ways to define and use Spring Beans, including Java-based and annotation-based configurations.
Along the way, we tackled common issues you might encounter when working with Spring Beans, such as bean wiring issues and scope-related problems, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
XML-Based Configuration | Moderate | Low | Small to Medium Applications |
Java-Based Configuration | High | Medium | Medium to Large Applications |
Annotation-Based Configuration | High | High | Large Applications |
Whether you’re just starting out with Spring Beans or looking to level up your skills, we hope this guide has given you a deeper understanding of Spring Beans and their usage.
With their versatility and the power of the Spring Framework behind them, Spring Beans are a powerful tool for any Java developer. Now, you’re well equipped to harness that power. Happy coding!