Mockito Verify Function | Java Parameter Testing Guide
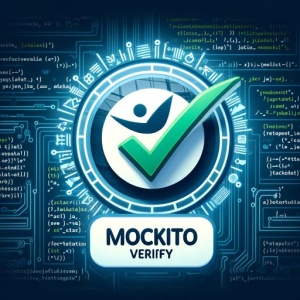
Are you finding it challenging to ensure your Java code behaves as expected? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a detective, Mockito verify is a handy utility that can seamlessly ensure your Java code behaves as expected. This tool is an essential part of Mockito, a popular mocking framework for unit tests in Java.
This guide will walk you through the ins and outs of using Mockito verify in your Java tests. We’ll explore Mockito verify’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Mockito verify!
TL;DR: How Do I Use Mockito Verify?
Mockito.verify()
is used to ensure a specific method was called with certain parameters. It is used with the syntax,Mockito.verify(mockObject, times(1)).methodToVerify();
It’s a powerful tool in Mockito’s arsenal that helps you validate your Java code’s behavior.
Here’s a simple example:
Mockito.verify(mockObject, times(1)).methodToVerify();
// Output:
// Ensures that methodToVerify() was called exactly once on mockObject.
In this example, we’re using Mockito’s verify method to ensure that methodToVerify()
was called exactly once on mockObject
. If the method was not called, or was called a different number of times, the test will fail.
This is just the tip of the iceberg when it comes to Mockito verify. Continue reading for a more detailed explanation, advanced usage scenarios, and troubleshooting tips.
Table of Contents
- Mockito Verify: Basic Use for Beginners
- Advanced Usage of Mockito Verify
- Exploring Alternative Approaches
- Troubleshooting Mockito Verify
- Understanding Behavior Verification in Testing
- The Role of Mockito in Java Testing
- Diving Deeper: Stubbing and Mocking
- Mockito Verify in Larger Testing Strategies
- Wrapping Up: Mastering Mockito.verify()
Mockito Verify: Basic Use for Beginners
Let’s start with the basics. Mockito verify is a method used in Mockito, a popular Java testing framework. Its primary function is to confirm that a specific method was called on a mock object during the test execution.
Here’s a simple example:
import static org.mockito.Mockito.*;
// Create a List mock
List<String> mockList = mock(List.class);
// Use the mock object
mockList.add("Mockito");
// Verify the behavior
verify(mockList).add("Mockito");
// Output:
// No output. The test passes silently as the method add() was called with the argument "Mockito".
In this example, we first create a mock object mockList
of type List
. Then, we use this mock object to perform an operation: adding the string “Mockito”. Finally, we use verify(mockList).add("Mockito");
to check that the add()
method was indeed called on mockList
with the argument “Mockito”.
If the method was not called with the specified argument, the test would fail. However, in this case, there’s no output because the test passes silently, indicating that our code behaved as expected.
This is a basic use of Mockito verify. It’s a powerful tool, but it has even more capabilities that we’ll explore in the next sections.
Advanced Usage of Mockito Verify
As you become more comfortable with Mockito verify, you can start to explore its more advanced features. It’s not just about verifying that a method was called; you can also confirm the number of times a method was invoked, or even check that it was called with specific parameters.
Verifying Method Calls
For instance, let’s say you want to ensure a method was called a certain number of times. Mockito verify can do that:
import static org.mockito.Mockito.*;
// Create a List mock
List<String> mockList = mock(List.class);
// Use the mock object
mockList.add("Mockito");
mockList.add("Mockito");
// Verify the behavior
verify(mockList, times(2)).add("Mockito");
// Output:
// No output. The test passes silently as the method add() was called twice with the argument "Mockito".
Here, we’re adding “Mockito” to our mockList
twice. Then, we use verify(mockList, times(2)).add("Mockito");
to confirm that add("Mockito")
was called exactly twice on mockList
. The test passes silently, which means our code behaved as expected.
Verifying Specific Parameters
You can also use Mockito verify to check that a method was called with specific parameters. Let’s look at an example:
import static org.mockito.Mockito.*;
// Create a Map mock
Map<String, String> mockMap = mock(Map.class);
// Use the mock object
mockMap.put("key", "value");
// Verify the behavior
verify(mockMap).put("key", "value");
// Output:
// No output. The test passes silently as the method put() was called with the arguments "key" and "value".
In this example, we use verify(mockMap).put("key", "value");
to confirm that put("key", "value")
was called on mockMap
. Again, the test passes silently, indicating that the put()
method was called with the correct parameters.
These examples showcase some of the advanced uses of Mockito verify. As you can see, it’s a versatile tool that can greatly enhance your Java testing capabilities.
Exploring Alternative Approaches
While Mockito verify is a powerful tool, it’s not the only way to validate behavior in Mockito. There are other techniques, such as using argument captors or alternative testing frameworks, that can also be effective depending on your specific needs.
Argument Captors in Action
Argument captors are a feature of Mockito that allow you to capture arguments for further assertions. This can be particularly useful when you want to verify certain characteristics of objects passed to the method.
Here’s an example of how you might use an argument captor:
import static org.mockito.Mockito.*;
import org.mockito.ArgumentCaptor;
// Create a mock List
List<String> mockList = mock(List.class);
// Use the mock object
mockList.add("Mockito");
// Create an ArgumentCaptor
ArgumentCaptor<String> argumentCaptor = ArgumentCaptor.forClass(String.class);
// Use the ArgumentCaptor
verify(mockList).add(argumentCaptor.capture());
// Verify the captured argument
assertEquals("Mockito", argumentCaptor.getValue());
// Output:
// No output. The test passes silently as the captured argument matches the expected value.
In this example, we use an ArgumentCaptor to capture the argument passed to the add()
method. We then verify that the captured argument matches the expected value, “Mockito”.
Considering Alternative Testing Frameworks
While Mockito is a popular choice for Java testing, there are other frameworks you might consider. JUnit and TestNG, for instance, also offer robust testing capabilities. However, Mockito’s simplicity and focus on mocking make it a preferred choice for many developers.
In conclusion, while Mockito verify is an invaluable tool for validating behavior in your Java tests, it’s worth exploring other methods and frameworks to find the approach that best suits your needs.
Troubleshooting Mockito Verify
While Mockito verify is a powerful tool, like any other, it can sometimes throw up unexpected results. Let’s explore some common issues you might encounter when using Mockito verify, along with their solutions.
Unexpected Method Calls
One common issue is when a method is called more times than you expected. This can cause your test to fail. Here’s an example:
import static org.mockito.Mockito.*;
// Create a mock List
List<String> mockList = mock(List.class);
// Use the mock object
mockList.add("Mockito");
mockList.add("Mockito");
// Verify the behavior
verify(mockList, times(1)).add("Mockito");
// Output:
// org.mockito.exceptions.verification.TooManyActualInvocations:
// mockList.add("Mockito");
// Wanted 1 time but was 2
In this case, we expected add("Mockito")
to be called once, but it was actually called twice. Mockito throws a TooManyActualInvocations
exception, causing the test to fail.
To resolve this, we need to ensure our code aligns with our expectations. In this case, we either need to only call add("Mockito")
once or adjust our verification to expect two calls.
Incorrect Parameters
Another issue you might encounter is when a method is called with different parameters than you expected. Here’s an example:
import static org.mockito.Mockito.*;
// Create a mock List
List<String> mockList = mock(List.class);
// Use the mock object
mockList.add("Java");
// Verify the behavior
verify(mockList).add("Mockito");
// Output:
// org.mockito.exceptions.verification.junit.ArgumentsAreDifferent:
// Argument(s) are different! Wanted:
// mockList.add("Mockito");
// -> at ...
// Actual invocation has different arguments:
// mockList.add("Java");
// -> at ...
In this case, we expected add("Mockito")
to be called, but the actual call was add("Java")
. Mockito throws an ArgumentsAreDifferent
exception, causing the test to fail.
To resolve this, we need to ensure our code aligns with our expectations. In this case, we either need to call add("Mockito")
or adjust our verification to expect add("Java")
.
These are just a few examples of the issues you might encounter when using Mockito verify. By understanding these problems and their solutions, you can avoid common pitfalls and write more robust tests.
Understanding Behavior Verification in Testing
Before we delve deeper into Mockito verify, let’s take a step back and understand the principles of behavior verification in testing. Behavior verification is a testing approach that focuses on the behavior of a system rather than its state. It’s all about ensuring that your system behaves as expected under different circumstances.
Consider a simple example. Let’s say you’re testing a calculator application. Instead of checking the state of the calculator after performing an operation (like checking the value displayed on the screen after adding two numbers), you would verify that the correct methods were called when you clicked the ‘add’ button.
The Role of Mockito in Java Testing
Now, where does Mockito fit into all this? Mockito is a mocking framework for unit tests in Java. It’s a tool that allows you to create and configure mock objects. With Mockito, you can simulate the behavior of complex, real objects and isolate the code under test.
Let’s illustrate this with a code snippet:
import static org.mockito.Mockito.*;
// Create a mock List
List<String> mockList = mock(List.class);
// Use the mock object
mockList.add("Mockito");
// Verify the behavior
verify(mockList).add("Mockito");
// Output:
// No output. The test passes silently as the method add() was called with the argument "Mockito".
In this example, we’re creating a mock List, using it to perform an operation, and then verifying the behavior. This is a fundamental use case of Mockito in Java testing.
Diving Deeper: Stubbing and Mocking
While we’ve focused on behavior verification, Mockito also supports other testing techniques like stubbing and mocking.
Stubbing involves creating ‘stubs’, or dummy objects, that return fixed values when their methods are called. This allows you to test the behavior of your code when it interacts with external systems.
Mocking, on the other hand, is about creating ‘mock’ objects that mimic the behavior of real objects. You can define what values the mock objects return when their methods are called and even verify if certain methods were called during the test execution.
In conclusion, Mockito verify is a powerful feature of Mockito that supports behavior verification in testing. By understanding the principles of behavior verification, as well as the role of Mockito in Java testing, you can write more effective tests and ensure your code behaves as expected.
Mockito Verify in Larger Testing Strategies
While Mockito verify is a powerful tool in its own right, it’s also an integral part of larger testing strategies. Let’s explore how Mockito verify fits into the bigger picture of test-driven development and integration testing.
Test-Driven Development and Mockito Verify
In test-driven development (TDD), you write tests before you write the actual code. The idea is to ensure your code meets its requirements and behaves as expected right from the start.
Mockito verify can play a crucial role in TDD. By using Mockito verify to write tests that confirm the behavior of your code, you can ensure that your code meets its requirements before you even start writing it.
Mockito Verify in Integration Testing
Integration testing is about testing how different parts of your system work together. Mockito verify can be useful here too.
For instance, you might use Mockito verify to confirm that a method was called when a certain action was performed in your system. This can help you ensure that different parts of your system are interacting as they should.
Further Resources for Mockito.verify() Mastery
If you’re interested in diving deeper into Mockito verify and its role in larger testing strategies, here are some resources you might find helpful:
- IOFlood’s Java Code Testing Article explores various testing techniques, including white-box and black-box testing.
Mockito Spy in Java – Understand Mockito’s spy, for creating partial mocks of objects in Java.
JMeter Overview – Explore Apache JMeter, a tool for performance and load testing web applications.
Mockito’s official documentation – A comprehensive resource on all things Mockito, including
verify
.Baeldung’s Guide to Mockito covers various aspects of Mockito, including advanced features.
JavaCodeGeeks’ Mockito Tutorial takes you through the basics of Mockito and Mockito verify.
By understanding how Mockito verify fits into larger testing strategies, you can leverage its full potential and write more effective tests.
Wrapping Up: Mastering Mockito.verify()
In this comprehensive guide, we’ve unlocked the secrets of Mockito verify, a powerful tool for behavior verification in Java testing. We’ve explored how Mockito verify can help ensure your code behaves as expected, enhancing the reliability of your software.
We began with the basics, learning how to use Mockito verify in simple scenarios. We then delved into more advanced uses, such as verifying method calls with specific parameters or a certain number of times. We also tackled common issues you might encounter when using Mockito verify, such as unexpected method calls or incorrect parameters, providing solutions to help you overcome these challenges.
Alongside Mockito verify, we explored alternative methods for verifying behavior in Mockito, such as using argument captors. We also considered other testing frameworks like JUnit and TestNG, giving you a broader perspective on Java testing tools. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Mockito Verify | High | Moderate | Verifying method calls |
Argument Captors | High | High | Verifying method arguments |
JUnit | Moderate | Low | General unit testing |
TestNG | High | High | Advanced testing scenarios |
Whether you’re just starting out with Mockito verify or you’re looking to level up your Java testing skills, we hope this guide has given you a deeper understanding of Mockito verify and its capabilities.
With its balance of flexibility and precision, Mockito verify is a powerful tool for behavior verification in Java testing. Happy coding!