JMeter Performance Testing: For Beginners to Experts
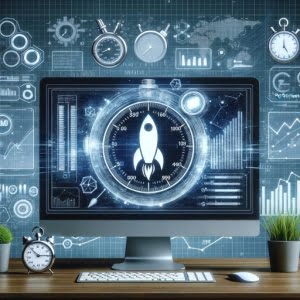
Are you feeling overwhelmed with performance testing? You’re not the only one. Many developers find it challenging to push their applications to their limits and ensure they can handle the stress.
Think of JMeter as your seasoned athlete, ready to help you in this task. With JMeter, you can conduct performance testing like a pro, ensuring your application can withstand high traffic and perform optimally under pressure.
In this guide, we will walk you through the basics of JMeter, a popular tool for performance testing. We will cover everything from installation, creating a test plan, to more advanced features. We will also discuss common issues you might encounter and how to solve them.
So, let’s dive in and start mastering JMeter!
TL;DR: How Do I Use JMeter for Performance Testing?
To use JMeter for performance testing, you first need to create a test plan, add thread groups, samplers, and listeners. You can start with a simple command like this:
jmeter -n -t [jmx file] -l [results file] -e -o [Path to output folder]
.
Here’s a simple example:
jmeter -n -t my_test_plan.jmx -l results.jtl -e -o /my/output/folder
In this example, we’re running JMeter in non-GUI mode (-n
), specifying a test plan (-t my_test_plan.jmx
), logging the results (-l results.jtl
), and generating an HTML report at the end (-e -o /my/output/folder
).
This is just a basic way to use JMeter for performance testing, but there’s much more to learn about creating and manipulating test plans in JMeter. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- JMeter Installation and Basic Usage
- Advanced JMeter Features: Timers, Assertions, and Configuration Elements
- Exploring Alternative Performance Testing Tools
- JMeter Troubleshooting: Common Issues and Solutions
- Optimizing JMeter Performance
- The Importance of Performance Testing
- The Role of JMeter in Performance Testing
- Understanding the Principles of Performance Testing
- The Relevance of Performance Testing in the Software Development Lifecycle
- Wrapping Up: Mastering JMeter for Efficient Performance Testing
JMeter Installation and Basic Usage
Before we can start performance testing with JMeter, we first need to install it. The installation process is straightforward. Here’s how you can install JMeter on a typical Linux system:
sudo apt-get update
sudo apt-get install jmeter
# Output:
# 'Reading package lists... Done'
# 'Building dependency tree... Done'
# 'jmeter is already the newest version (5.1.1+dfsg-3ubuntu1).'
# '0 upgraded, 0 newly installed, 0 to remove and 0 not upgraded.'
Once JMeter is installed, we can start creating a test plan. A test plan in JMeter is the place where you specify what tests you want to run on your application. Here are the steps to create a basic test plan:
- Launch JMeter: You can do this by running the command
jmeter
in your terminal. - Right-click on the Test Plan node and select Add > Threads (Users) > Thread Group.
- Right-click on the Thread Group node and select Add > Sampler > HTTP Request.
- Right-click on the HTTP Request node and select Add > Listener > View Results in Table.
This is a simple test plan that sends an HTTP request and views the results in a table. The ‘Thread Group’ defines the number of users that JMeter will simulate, the ‘HTTP Request’ is the request that each user will send, and the ‘View Results in Table’ listener will let you view the results of the test.
Here is a simple example of a JMeter test plan:
<jmeterTestPlan version="1.2" properties="5.0" jmeter="5.4.1">
<hashTree>
<TestPlan guiclass="TestPlanGui" testclass="TestPlan" testname="Test Plan" enabled="true">
<stringProp name="TestPlan.comments"></stringProp>
<boolProp name="TestPlan.functional_mode">false</boolProp>
<boolProp name="TestPlan.tearDown_on_shutdown">true</boolProp>
<boolProp name="TestPlan.serialize_threadgroups">false</boolProp>
<elementProp name="TestPlan.user_defined_variables" elementType="Arguments" guiclass="ArgumentsPanel" testclass="Arguments" testname="User Defined Variables" enabled="true">
<collectionProp name="Arguments.arguments"/>
</elementProp>
<stringProp name="TestPlan.user_define_classpath"></stringProp>
</TestPlan>
<hashTree>
<ThreadGroup guiclass="ThreadGroupGui" testclass="ThreadGroup" testname="Thread Group" enabled="true">
<stringProp name="ThreadGroup.on_sample_error">continue</stringProp>
<elementProp name="ThreadGroup.main_controller" elementType="LoopController" guiclass="LoopControlPanel" testclass="LoopController" testname="Loop Controller" enabled="true">
<boolProp name="LoopController.continue_forever">false</boolProp>
<stringProp name="LoopController.loops">1</stringProp>
</elementProp>
<stringProp name="ThreadGroup.num_threads">1</stringProp>
<stringProp name="ThreadGroup.ramp_time">1</stringProp>
<boolProp name="ThreadGroup.scheduler">false</boolProp>
<stringProp name="ThreadGroup.duration"></stringProp>
<stringProp name="ThreadGroup.delay"></stringProp>
</ThreadGroup>
<hashTree>
<HTTPSamplerProxy guiclass="HttpTestSampleGui" testclass="HTTPSamplerProxy" testname="HTTP Request" enabled="true">
<elementProp name="HTTPsampler.Arguments" elementType="Arguments" guiclass="HTTPArgumentsPanel" testclass="Arguments" testname="User Defined Variables" enabled="true">
<collectionProp name="Arguments.arguments"/>
</elementProp>
<stringProp name="HTTPSampler.domain"></stringProp>
<stringProp name="HTTPSampler.port"></stringProp>
<stringProp name="HTTPSampler.protocol"></stringProp>
<stringProp name="HTTPSampler.contentEncoding"></stringProp>
<stringProp name="HTTPSampler.path"></stringProp>
<stringProp name="HTTPSampler.method">GET</stringProp>
<boolProp name="HTTPSampler.follow_redirects">true</boolProp>
<boolProp name="HTTPSampler.auto_redirects">false</boolProp>
<boolProp name="HTTPSampler.use_keepalive">true</boolProp>
<boolProp name="HTTPSampler.DO_MULTIPART_POST">false</boolProp>
<stringProp name="HTTPSampler.embedded_url_re"></stringProp>
<stringProp name="HTTPSampler.connect_timeout"></stringProp>
<stringProp name="HTTPSampler.response_timeout"></stringProp>
</HTTPSamplerProxy>
<hashTree/>
</hashTree>
</hashTree>
</hashTree>
</jmeterTestPlan>
This XML code represents a simple JMeter test plan that sends a single HTTP GET request. You can save this as a .jmx
file and load it into JMeter or run it from the command line.
Advanced JMeter Features: Timers, Assertions, and Configuration Elements
As you get more comfortable with JMeter, you’ll want to explore its more advanced features. Let’s dive into these next.
JMeter Timers
Timers in JMeter allow you to introduce a delay between requests. This can be useful for simulating real-world user behavior, where users don’t send requests continuously but rather with some delay in between.
Here’s an example of how to add a timer to a JMeter test plan:
# Add a timer to the HTTP Request sampler
Right-click on the HTTP Request > Add > Timer > Constant Timer
This will add a Constant Timer to the HTTP Request. You can specify the delay in milliseconds in the ‘Thread Delay’ field.
JMeter Assertions
Assertions in JMeter allow you to validate the responses from your server. For example, you can use an assertion to check if a response contains a specific string or matches a regular expression.
Here’s an example of how to add an assertion to a JMeter test plan:
# Add an assertion to the HTTP Request sampler
Right-click on the HTTP Request > Add > Assertions > Response Assertion
This will add a Response Assertion to the HTTP Request. You can specify the pattern to test in the ‘Patterns to Test’ field.
JMeter Configuration Elements
Configuration elements in JMeter allow you to customize the samplers in your test plan. For example, you can use a HTTP Request Defaults configuration element to specify default values for all HTTP Request samplers in your test plan.
Here’s an example of how to add a configuration element to a JMeter test plan:
# Add a HTTP Request Defaults configuration element to the test plan
Right-click on the Test Plan > Add > Config Element > HTTP Request Defaults
This will add a HTTP Request Defaults configuration element to the test plan. You can specify the default values in the respective fields.
By understanding and utilizing these advanced features, you can create more complex and realistic test plans in JMeter. Remember, practice makes perfect, so don’t be afraid to experiment and try out different configurations!
Exploring Alternative Performance Testing Tools
While JMeter is a powerful tool for performance testing, it’s not the only one out there. Let’s take a look at a couple of other popular tools in the performance testing landscape: Gatling and LoadRunner.
Gatling: A High-Performance Alternative
Gatling is a high-performance load testing tool, particularly well-suited for testing applications that require real-time processing. It’s designed to be easy to use, with a DSL (domain-specific language) for test scripting that’s more concise and readable than JMeter’s XML-based test plans.
Here’s a simple Gatling test script:
import io.gatling.core.Predef._
import io.gatling.http.Predef._
import scala.concurrent.duration._
class BasicSimulation extends Simulation {
val httpProtocol = http
.baseUrl("https://computer-database.gatling.io")
.acceptHeader("text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8")
.doNotTrackHeader("1")
.acceptLanguageHeader("en-US,en;q=0.5")
.acceptEncodingHeader("gzip, deflate")
.userAgentHeader("Mozilla/5.0 (Macintosh; Intel Mac OS X 10.8; rv:16.0) Gecko/20100101 Firefox/16.0")
val scn = scenario("BasicSimulation")
.exec(http("request_1")
.get("/")
.check(status.is(200)))
setUp(scn.inject(atOnceUsers(1)).protocols(httpProtocol))
}
This script runs a single user sending a GET request to the specified URL and checks that the response status is 200.
LoadRunner: An Enterprise-Level Solution
LoadRunner is an enterprise-level performance testing tool from Micro Focus. It supports a wide range of protocols, making it suitable for testing all kinds of applications, from web apps to ERP systems.
LoadRunner uses a different scripting language, VuGen, which can be more complex than JMeter’s XML or Gatling’s DSL. However, it offers advanced features like end-to-end system monitoring and network virtualization.
Here’s an example of a LoadRunner VuGen script:
#include "lrun.h"
Action()
{
web_url("webtours",
"URL=http://127.0.0.1:1080/webtours/",
"Resource=0",
"RecContentType=text/html",
"Referer=",
"Snapshot=t1.inf",
"Mode=HTML",
LAST);
return 0;
}
This script sends a GET request to the specified URL, similar to the Gatling and JMeter examples.
Each of these tools has its own strengths and weaknesses. Gatling’s concise scripting and high performance make it a great choice for testing real-time applications, while LoadRunner’s advanced features and wide protocol support make it a powerful solution for enterprise-level testing. JMeter, with its easy-to-use GUI and flexibility, remains a reliable choice for a wide range of performance testing needs.
JMeter Troubleshooting: Common Issues and Solutions
While JMeter is a powerful tool, like any software, it’s not without its quirks. Here are some common issues that you might encounter when using JMeter and how to solve them.
OutOfMemoryError
One of the most common issues that users encounter when using JMeter is the OutOfMemoryError
. This error occurs when JMeter runs out of memory during a test. Here’s how you might see this error:
java.lang.OutOfMemoryError: Java heap space
This error means that JMeter has exhausted its allocated heap space. The heap space is where JMeter stores the objects it creates during a test, and if your test is too large or complex, it can fill up this space.
To solve this issue, you can increase the maximum heap size for JMeter. This can be done by editing the jmeter
startup script and changing the -Xmx
option. For example, to set the maximum heap size to 2 gigabytes, you would change the -Xmx
option to -Xmx2g
.
# Edit the jmeter startup script
nano /path/to/jmeter
# Change the -Xmx option
-Xmx2g
This change will allow JMeter to use up to 2 gigabytes of heap space, which should be sufficient for most tests.
Optimizing JMeter Performance
There are several ways you can optimize JMeter’s performance to get the most out of your tests. Here are a few tips:
- Use Non-GUI Mode: Running JMeter in non-GUI mode (
jmeter -n
) can significantly improve its performance, as it reduces the resources used by the GUI. - Reduce the Number of Samplers: Each sampler in a test plan consumes resources, so try to keep your test plans as simple as possible.
- Use Timers Wisely: While timers can be useful for simulating real-world user behavior, they can also slow down your tests. Use them judiciously.
- Disable or Limit Listeners: Listeners can consume a lot of resources, especially if they’re logging a lot of data. Disable any listeners you don’t need, and limit the amount of data they log.
By keeping these tips in mind, you can ensure that you’re using JMeter as efficiently as possible, allowing you to run larger and more complex tests without running into issues.
The Importance of Performance Testing
Performance testing is a crucial part of the software development process. It’s about ensuring your application can handle the expected load and perform well under stress. It’s about providing a smooth, seamless experience to your users, regardless of how many of them are using the application at the same time.
Imagine launching a new application, only to have it crash or slow down significantly when multiple users start using it. This could lead to a poor user experience, negative reviews, and ultimately, loss of users or revenue. Performance testing helps you avoid these scenarios.
The Role of JMeter in Performance Testing
This is where tools like JMeter come in. JMeter is a powerful, open-source tool designed for load testing and measuring performance. It can simulate multiple users sending requests to your application, allowing you to see how it performs under stress.
Here’s a simple example of using JMeter to send 100 users to your application:
jmeter -n -t my_test_plan.jmx -Jusers=100 -l results.jtl
# Output:
# 'Creating summariser <summary>'
# 'Created the tree successfully using my_test_plan.jmx'
# 'Starting the test @ Tue Mar 15 16:52:36 PDT 2022 (1647390756938)'
# 'Waiting for possible Shutdown/StopTestNow/HeapDump/ThreadDump message on port 4445'
# 'summary = 100 in 00:00:30 = 3.3/s Avg: 283 Min: 63 Max: 1023 Err: 0 (0.00%)'
# 'Tidying up ... @ Tue Mar 15 16:53:06 PDT 2022 (1647390786938)'
# '... end of run'
In this example, we’re running JMeter in non-GUI mode (-n), specifying a test plan (-t my_test_plan.jmx), setting the number of users to 100 (-Jusers=100), and logging the results (-l results.jtl). The output shows that JMeter successfully sent 100 users to the application in 30 seconds, with an average response time of 283 milliseconds.
Understanding the Principles of Performance Testing
Performance testing is based on a few key principles. First, it’s about simulating real-world conditions. This means sending requests to your application in a way that mimics how your users would. Second, it’s about measuring and analyzing the results. This involves looking at metrics like response time, error rate, and throughput. Finally, it’s about making improvements based on these results. This could involve optimizing your code, increasing your server resources, or making other changes to improve performance.
By understanding these principles and using tools like JMeter, you can ensure your application is ready to perform in the real world.
The Relevance of Performance Testing in the Software Development Lifecycle
Performance testing isn’t a one-time event; it’s an ongoing process that should be integrated into every stage of your software development lifecycle. From the initial design phase to the final deployment, performance testing provides valuable insights that can inform your decisions and help you build a better application.
Load Testing, Stress Testing, and Endurance Testing
While we’ve focused on performance testing in this guide, it’s worth noting that there are several related concepts that you might want to explore. These include load testing, stress testing, and endurance testing.
- Load Testing: This involves testing how your application performs under a specific load, such as a certain number of users or requests per second. It’s similar to performance testing, but the focus is on simulating a specific, expected load.
Stress Testing: This involves testing how your application performs under extreme conditions, such as a very high load or a sudden spike in traffic. The goal is to identify the breaking point of your application and ensure it fails gracefully.
Endurance Testing: This involves testing how your application performs over a long period of time, such as several hours or days. The goal is to identify any issues that might only appear after prolonged use, such as memory leaks.
Each of these tests provides a different perspective on your application’s performance, and together they can give you a comprehensive understanding of how your application behaves under various conditions.
Further Resources for JMeter and Performance Testing
If you’re interested in learning more about JMeter and performance testing, here are a few resources that you might find helpful:
- Java Code Testing Tutorial: Getting Started – Dive into test-driven development (TDD) principles and practices in Java.
Exploring Mockito Verify Features – Explore use cases of Mockito verify for method validating in Java tests.
Understanding Reflection in Java – Explore practical use cases for Java reflection, including dependency injection.
Apache JMeter User’s Manual covers everything from installation to advanced features.
JMeter Performance Testing by Guru99 offers a comprehensive tutorial on performance testing with Apache JMeter.
Web Performance Testing with Apache JMeter online course provides an introduction to performance testing with JMeter.
Remember, mastering JMeter and performance testing is a journey, not a destination. Keep learning, keep experimenting, and most importantly, have fun along the way!
Wrapping Up: Mastering JMeter for Efficient Performance Testing
In this comprehensive guide, we’ve delved into the world of JMeter, a robust tool for performance testing. We’ve explored how to get started with JMeter, how to create test plans, and how to use its advanced features to simulate real-world user behavior and validate server responses.
We began with the basics, discussing how to install JMeter and create a simple test plan. We then dived deeper, exploring JMeter’s advanced features like timers, assertions, and configuration elements. We also provided practical examples and code snippets to guide you along the way.
In addition to JMeter, we explored alternative tools for performance testing, such as Gatling and LoadRunner, comparing their strengths and weaknesses. We also addressed common issues you might encounter when using JMeter, such as ‘OutOfMemoryError’, and provided tips for optimizing JMeter’s performance.
Here’s a quick comparison of the tools we’ve discussed:
Tool | Pros | Cons |
---|---|---|
JMeter | Flexible, easy-to-use GUI | Can be resource-intensive |
Gatling | High performance, concise scripting | Less GUI support |
LoadRunner | Wide protocol support, advanced features | Complex scripting, less free options |
Whether you’re a beginner just starting out with JMeter or an experienced developer looking to up your performance testing game, we hope this guide has been a valuable resource. With the knowledge and skills you’ve gained, you’re now well-equipped to use JMeter to ensure your applications can handle the load and deliver a smooth user experience. Happy testing!