Python Datetime | Module Usage Guide (With Examples)
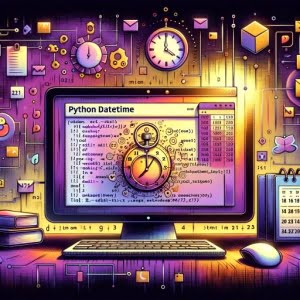
Ever found yourself wrestling with dates and times in Python? Well, you’re not alone. Handling time can be a mind-bending task, especially when dealing with different time zones, formats, and calculations. But fear not! Python’s datetime module is like your personal time machine, designed to help you navigate the complex world of dates, times, and intervals.
In this comprehensive guide, we’ll take a journey from the basics of using the datetime module, such as getting the current date and time, to more advanced techniques like calculating time differences and dealing with time zones. We’ll also discuss best practices to ensure your time travel journey is smooth and error-free.
So, if you’ve ever found yourself asking, ‘How can I handle dates and times in Python effectively?’, you’re in the right place. Let’s dive into the world of Python datetime!
TL;DR: How do I use the datetime module in Python?
To use the datetime module in Python, you first need to import it. Then, you can use its built-in functions to manipulate dates and times. Here’s a quick example to get the current date and time:
import datetime
now = datetime.datetime.now()
print(now)
# Output:
# 2022-12-28 14:30:22.123456 (The output will vary based on the current date and time.)
In this example, we’ve imported the datetime module, used the now()
function to get the current date and time, and printed it out. This is just the tip of the iceberg when it comes to Python’s datetime module capabilities.
If you’re intrigued and want to delve deeper into using datetime for more complex tasks, keep reading! We’re going to explore everything from basic usage to advanced techniques and best practices.
Table of Contents
- Python Datetime Basics: Getting Started
- Advanced Datetime: Calculate, Convert, and Parse
- Exploring Alternatives: Time, Calendar, and Dateutil
- Navigating Pitfalls: Troubleshooting Python’s Datetime
- Python Datetime Fundamentals: How Python Understands Time
- Expanding Horizons: Datetime in Real-world Projects
- Further Resources on Python Time Modules
- Python Datetime: A Comprehensive Recap
Python Datetime Basics: Getting Started
The datetime module in Python is packed with powerful features, but before we get into the more advanced stuff, let’s cover the basics. Here’s what you’ll learn in this section:
- How to get the current date and time
- How to create specific datetime objects
- How to format datetime objects into readable strings
Getting the Current Date and Time
The first thing you might want to do with Python’s datetime module is get the current date and time. Here’s how you do it:
import datetime
# Get the current date and time
now = datetime.datetime.now()
print(now)
# Output:
# 2022-12-28 14:30:22.123456 (The output will vary based on the current date and time.)
In this code block, we first import the datetime module. We then use the now()
function from the datetime class to get the current date and time. The now()
function returns a datetime object representing the current date and time.
Creating Specific Datetime Objects
Sometimes, you need to create a datetime object for a specific date and time. Here’s how you do it:
import datetime
# Create a datetime object for a specific date and time
birthday = datetime.datetime(1990, 5, 17, 12, 30)
print(birthday)
# Output:
# 1990-05-17 12:30:00
In this code block, we create a datetime object for a specific date and time. We pass the year, month, day, hour, and minute as arguments to the datetime constructor. The datetime object represents the specified date and time.
Formatting Datetime Objects into Readable Strings
Datetime objects are great for calculations, but they’re not very human-readable. Luckily, we can format datetime objects into strings. Here’s how you do it:
import datetime
# Get the current date and time
now = datetime.datetime.now()
# Format the datetime object into a string
formatted_now = now.strftime('%Y-%m-%d %H:%M:%S')
print(formatted_now)
# Output:
# '2022-12-28 14:30:22' (The output will vary based on the current date and time.)
In this code block, we use the strftime()
function to format the datetime object into a string. We pass a format string to the strftime()
function, which specifies the format of the output string. The %Y
represents the full year, %m
represents the month, %d
represents the day, %H
represents the hour, %M
represents the minute, and %S
represents the second.
That covers the basics of using Python’s datetime module. But there’s much more to learn, so let’s dive into some more advanced topics!
Advanced Datetime: Calculate, Convert, and Parse
Now that you’re comfortable with the basics of Python’s datetime module, let’s dive into some more advanced topics. In this section, we’ll cover:
- Calculating time differences
- Working with time zones
- Parsing datetime strings
Calculating Time Differences
Calculating the difference between two points in time is a common task in Python. Here’s how you do it with the datetime module:
import datetime
# Create two datetime objects
start_time = datetime.datetime(2022, 1, 1, 12, 0)
end_time = datetime.datetime(2022, 1, 1, 14, 30)
# Calculate the difference between the two times
time_difference = end_time - start_time
print(time_difference)
# Output:
# 2:30:00
In this code block, we first create two datetime objects representing two points in time. We then subtract the start time from the end time to get a timedelta object representing the difference between the two times. This timedelta object can be printed out as a string in the format ‘hours:minutes:seconds’.
Working with Time Zones
Working with time zones can be tricky, but Python’s datetime module makes it easier. Here’s how you can create a datetime object in a specific time zone:
import datetime
import pytz
# Create a datetime object for the current time in UTC
now_utc = datetime.datetime.now(pytz.timezone('UTC'))
print(now_utc)
# Output:
# 2022-12-28 14:30:22.123456+00:00 (The output will vary based on the current date and time.)
In this code block, we first import the datetime and pytz modules. We then use the now()
function with the pytz.timezone('UTC')
argument to get the current date and time in UTC. The +00:00
at the end of the output indicates that the time is in the UTC time zone.
Parsing Datetime Strings
Sometimes, you’ll have a string representing a date and time, and you’ll need to convert this string into a datetime object. Here’s how you do it:
import datetime
# Create a string representing a date and time
datetime_string = '2022-12-28 14:30:22'
# Parse the string into a datetime object
parsed_datetime = datetime.datetime.strptime(datetime_string, '%Y-%m-%d %H:%M:%S')
print(parsed_datetime)
# Output:
# 2022-12-28 14:30:22
In this code block, we first create a string representing a date and time. We then use the strptime()
function to parse the string into a datetime object. The strptime()
function takes two arguments: the string to parse and the format of the string.
That’s a wrap on the intermediate-level uses of Python’s datetime module. But there’s still more to learn, so let’s keep going!
Exploring Alternatives: Time, Calendar, and Dateutil
While the datetime module provides a robust set of tools to work with dates and times in Python, it’s not the only game in town. In this section, we’ll explore alternative ways to handle dates and times, including the time and calendar modules, and the third-party library dateutil.
Python’s Time Module
Python’s time module provides functions to work with time. Here’s an example of getting the current time using the time module:
import time
# Get the current time
now = time.time()
print(now)
# Output:
# 1640698212.123456 (The output will vary based on the current time.)
In this code block, we first import the time module. We then use the time()
function to get the current time in seconds since the epoch (January 1, 1970).
Python’s Calendar Module
Python’s calendar module provides functions to work with dates. Here’s an example of getting the number of days in a month using the calendar module:
import calendar
# Get the number of days in February 2022
days = calendar.monthrange(2022, 2)[1]
print(days)
# Output:
# 28
In this code block, we first import the calendar module. We then use the monthrange()
function to get the number of days in February 2022.
The Dateutil Library
The dateutil library is a third-party library that provides powerful extensions to the standard datetime module. Here’s an example of parsing a datetime string in an arbitrary format using dateutil:
from dateutil.parser import parse
# Parse a datetime string in an arbitrary format
datetime = parse('2022-12-28T14:30:22Z')
print(datetime)
# Output:
# 2022-12-28 14:30:22+00:00
In this code block, we first import the parse function from dateutil.parser. We then use the parse()
function to parse a datetime string in an arbitrary format.
As you can see, Python provides several ways to handle dates and times, each with its own strengths and weaknesses. Depending on your specific needs, you might find one approach more suitable than others.
As powerful as Python’s datetime module is, it’s not without its quirks. In this section, we’ll discuss some common issues developers face when working with datetime, including dealing with leap years, daylight saving time, and time zone differences. We’ll also share solutions and best practices to help you avoid these pitfalls.
Dealing with Leap Years
Leap years can be a source of bugs in date calculations. Fortunately, Python’s datetime module handles leap years automatically. However, if you’re calculating dates manually, remember that a year is a leap year if it’s divisible by 4, but not by 100, unless it’s also divisible by 400.
Handling Daylight Saving Time
Daylight saving time can also cause issues. When converting between time zones, Python’s datetime module doesn’t automatically account for daylight saving time. You’ll need to use a library like pytz, which has a database of daylight saving time rules.
Here’s an example of converting a datetime from one time zone to another, accounting for daylight saving time:
import datetime
import pytz
# Create a datetime object in one time zone
start_time = datetime.datetime(2022, 3, 14, 12, 0, tzinfo=pytz.timezone('US/Eastern'))
# Convert the datetime to another time zone
end_time = start_time.astimezone(pytz.timezone('US/Pacific'))
print(end_time)
# Output:
# 2022-03-14 09:00:00-07:00
In this code block, we create a datetime object in the US/Eastern time zone. We then use the astimezone()
function to convert the datetime to the US/Pacific time zone. The output shows that the time is adjusted correctly for daylight saving time.
Time Zone Differences
Working with different time zones can be challenging, but Python’s datetime module, in combination with the pytz library, makes it manageable. Always be aware of the time zone of your datetime objects, and use the astimezone()
function to convert between time zones.
Remember, when it comes to handling dates and times in Python, understanding the tools at your disposal is half the battle. With these tips and best practices, you’ll be well-equipped to navigate the challenges of Python’s datetime module.
Python Datetime Fundamentals: How Python Understands Time
Before we delve further into Python’s datetime module, it’s crucial to understand the basics of how Python represents dates and times, and the design of the datetime module itself. This foundation will help you better understand and use the module’s features.
Python’s Representation of Dates and Times
In Python, dates and times are represented as datetime objects, which are instances of the datetime class in the datetime module. A datetime object consists of several components, including the year, month, day, hour, minute, second, and microsecond.
Here’s an example of creating a datetime object:
import datetime
# Create a datetime object
my_datetime = datetime.datetime(2022, 1, 1, 12, 0)
print(my_datetime)
# Output:
# 2022-01-01 12:00:00
In this code block, we import the datetime module and create a datetime object representing January 1, 2022, at 12:00 PM. The output shows the date and time in a human-readable format.
The Design of the Datetime Module
The datetime module is designed to be both simple and flexible. It provides several classes, including datetime, date, time, timedelta, and tzinfo, which allow you to work with dates, times, intervals, and time zones in various ways.
The datetime class is the most important and provides the most functionality. It includes methods to get the current date and time, create datetime objects, and manipulate and format them.
Time Zones and Daylight Saving Time
Python’s datetime module also provides tools to work with time zones and daylight saving time. However, these features are somewhat limited, and for more complex time zone operations, you might need to use a third-party library like pytz.
Here’s an example of creating a datetime object in a specific time zone:
import datetime
import pytz
# Create a datetime object in a specific time zone
my_datetime = datetime.datetime(2022, 1, 1, 12, 0, tzinfo=pytz.timezone('US/Eastern'))
print(my_datetime)
# Output:
# 2022-01-01 12:00:00-05:00
In this code block, we import the datetime and pytz modules and create a datetime object in the US/Eastern time zone. The output shows the date and time, along with the time zone offset (-05:00 for Eastern Standard Time).
By understanding these fundamentals, you’ll be better equipped to make full use of Python’s datetime module.
Expanding Horizons: Datetime in Real-world Projects
Python’s datetime module isn’t just for learning exercises or small scripts. It’s a powerful tool that can be used in larger projects, including data analysis, web development, and automation. Let’s explore a few scenarios where datetime really shines.
Datetime in Data Analysis
In data analysis, datetime can be used to manipulate and analyze time-series data, which are data points indexed (or listed or graphed) in time order. Here’s a simple example using Python’s pandas library:
import pandas as pd
import datetime
# Create a date range
dates = pd.date_range(start='1/1/2022', end='1/07/2022')
# Create a time series with the date range as index
time_series = pd.Series(range(len(dates)), index=dates)
print(time_series)
# Output:
# 2022-01-01 0
# 2022-01-02 1
# 2022-01-03 2
# 2022-01-04 3
# 2022-01-05 4
# 2022-01-06 5
# 2022-01-07 6
# Freq: D, dtype: int64
In this code block, we first import the pandas and datetime modules. We then create a date range and a time series with the date range as index. The output shows a time series with dates as indices and integers as values.
Datetime in Web Development
In web development, datetime can be used to handle user input, store data in databases, and display data to users. For example, a blog website might use datetime to store the time of each post and display it to users in their local time zone.
Datetime in Automation
In automation, datetime can be used to schedule tasks. For example, you might use datetime to run a script every day at a specific time. Python’s built-in sched module, along with datetime, can be used to accomplish this.
As you can see, Python’s datetime module is incredibly versatile and can be used in a wide range of projects. If you’re interested in learning more, consider exploring Python’s time and calendar modules, which provide additional tools to work with dates and times.
Further Resources on Python Time Modules
To help you advance your exploration of Time Modules in Python, we’ve gathered a list of notable resources:
- Python Time: A Quick Overview – Explore Python’s time module for measuring elapsed time and scheduling tasks.
Simplifying Date Retrieval in Python – Discover Python’s built-in functions for getting the current date with ease.
Converting Datetime Objects to Strings in Python – Learn how to represent datetime objects as strings in Python scripts.
Converting to 12-Hour Clock on Codecademy – Learn how to convert time to a 12-hour clock format in Python.
Understanding Strftime in Python on FreeCodeCamp – Explore how to use Python’s strftime function to format time and date.
Python DateTime Tutorial on DataQuest – A comprehensive guide to working with dates and times in Python.
Use these resources to solidify your foundation and keep expanding your Python Time Modules knowledge.
Python Datetime: A Comprehensive Recap
We’ve journeyed through the ins and outs of Python’s datetime module, starting from the basics and moving onto more advanced techniques. Here’s a quick recap of what we’ve covered:
We started with the basics of the datetime module, learning how to get the current date and time, create specific datetime objects, and format these objects into readable strings. We then ventured into more advanced territory, discussing how to calculate time differences, work with time zones, and parse datetime strings.
We explored common issues when working with datetime, such as dealing with leap years, daylight saving time, and time zone differences. We also shared solutions and best practices to help you navigate these challenges.
We looked at alternative approaches to handling dates and times, including Python’s time and calendar modules, and the third-party library dateutil. Each of these alternatives has its own strengths and weaknesses, and the best choice depends on your specific needs.
We delved into the fundamentals of how Python represents dates and times, and the design of the datetime module. Understanding these fundamentals can help you make better use of the datetime module’s features.
Finally, we discussed how the datetime module can be used in real-world projects, including data analysis, web development, and automation. The datetime module is incredibly versatile and can be used in a wide range of applications.
Whether you’re a beginner just starting out with Python, or an experienced developer looking to brush up on your skills, we hope this guide has been helpful in mastering Python’s datetime module. Remember, practice makes perfect, so don’t be afraid to get your hands dirty and experiment with datetime in your own projects!