Learn Python: Convert datetime to string (With Examples)
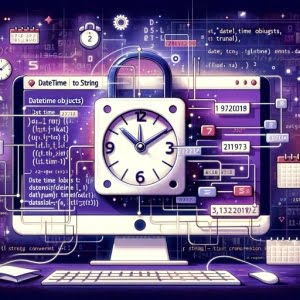
Ever felt like a time traveler lost in the realm of Python’s datetime objects? Struggling to convert these datetime objects into strings? Don’t worry, we’ve got you covered. Python provides us with easy-to-use functions to convert datetime objects into strings.
In this comprehensive guide, we will walk you through the process of converting Python datetime to string – from basic usage to more advanced techniques. So, buckle up and get ready to dive into the world of Python datetime conversions!
TL;DR: How Do I Convert Datetime to String in Python?
You can use the
strftime()
function to convert datetime to a string in Python. Here’s a simple example:
from datetime import datetime
now = datetime.now()
string = now.strftime('%Y-%m-%d %H:%M:%S')
print(string)
# Output:
# '2022-06-30 15:30:00'
In this example, we’ve imported the datetime
module, obtained the current date and time using datetime.now()
, and then converted it to a string using the strftime()
function. The format string ‘%Y-%m-%d %H:%M:%S’ specifies the output format, which in this case is ‘YYYY-MM-DD HH:MM:SS’.
This is just the tip of the iceberg! Keep reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of strftime()
Function in Python
In Python, the strftime()
function is a built-in method used to convert a datetime object into a string representing date and time under different formats.
The strftime()
stands for ‘String Format Time’. It belongs to the datetime and time modules, and it requires a format string to dictate the output format of the date and time.
Let’s see a basic example of how to use the strftime()
function:
from datetime import datetime
# current date and time
date_time = datetime.now()
# format specification
format = '%Y-%m-%d %H:%M:%S'
# applying strftime() to format the datetime
string = date_time.strftime(format)
print('Date and Time:', string)
# Output:
# Date and Time: 2022-06-30 15:30:00
In this code block, we first import the datetime module. Then, we get the current date and time using datetime.now()
. After that, we specify the format in which we want our date and time to be converted into a string. Finally, we use the strftime()
function to convert the datetime object into a string.
Handling Different Date and Time Formats
As you start to delve deeper into Python’s datetime conversions, you’ll find that there are numerous date and time formats to consider. The strftime()
function provides a wide range of format codes that allow you to handle different date and time formats.
Let’s take a look at an example where we use different format codes to represent the same datetime object:
from datetime import datetime
# current date and time
date_time = datetime.now()
# different format specifications
format1 = '%Y-%m-%d %H:%M:%S' # YYYY-MM-DD HH:MM:SS
format2 = '%d/%m/%Y, %H:%M:%S' # DD/MM/YYYY, HH:MM:SS
format3 = '%A, %d. %B %Y %I:%M%p' # Monday, 30. June 2022 03:30PM
# applying strftime() to format the datetime
string1 = date_time.strftime(format1)
string2 = date_time.strftime(format2)
string3 = date_time.strftime(format3)
print('Format 1:', string1)
print('Format 2:', string2)
print('Format 3:', string3)
# Output:
# Format 1: 2022-06-30 15:30:00
# Format 2: 30/06/2022, 15:30:00
# Format 3: Thursday, 30. June 2022 03:30PM
In this example, we’ve used three different format specifications for the same datetime object.
The first format is the standard ‘YYYY-MM-DD HH:MM:SS’. The second format changes the date to ‘DD/MM/YYYY’ and adds a comma between the date and time. The third format gives a more verbose output, including the day of the week, the date with a period after the day number, the full month name, the year, and the time in 12-hour format with AM/PM.
The strftime()
function provides a great deal of flexibility in handling different date and time formats. However, it’s crucial to use the correct format codes and to understand the output they produce.
Exploring Alternative Methods for Datetime Conversion
While strftime()
is a powerful tool for datetime conversion, Python offers alternative methods that can be more suitable in certain scenarios. Two of these methods are isoformat()
and __str__()
.
Converting Datetime to String Using isoformat()
The isoformat()
method returns a string representing the date in ISO 8601 format, which is ‘YYYY-MM-DDTHH:MM:SS’. Let’s see it in action:
from datetime import datetime
# current date and time
date_time = datetime.now()
# using isoformat()
string = date_time.isoformat()
print('ISO Format:', string)
# Output:
# ISO Format: 2022-06-30T15:30:00
In this example, we use isoformat()
to convert the datetime object into a string. Note the ‘T’ separator between the date and time in the output.
Converting Datetime to String Using __str__()
The __str__()
method is another way to convert datetime to string. It returns a string representing the date and time in the format ‘YYYY-MM-DD HH:MM:SS.ssssss’. Here’s how it works:
from datetime import datetime
# current date and time
date_time = datetime.now()
# using __str__()
string = date_time.__str__()
print('Str Format:', string)
# Output:
# Str Format: 2022-06-30 15:30:00.000000
In this example, we use __str__()
to convert the datetime object into a string. Note the inclusion of microseconds in the output.
While isoformat()
and __str__()
can be handy, they offer less flexibility compared to strftime()
. Both functions have fixed output formats and do not accept format codes. However, they can be more convenient for quick and simple conversions.
Method | Flexibility | Output Format |
---|---|---|
strftime() | High | Customizable |
isoformat() | Low | ‘YYYY-MM-DDTHH:MM:SS’ |
__str__() | Low | ‘YYYY-MM-DD HH:MM:SS.ssssss’ |
The method you choose for converting datetime to string in Python depends on your specific needs and the level of customization you require. If you need a specific format, strftime()
is the way to go. For standard formats, isoformat()
and __str__()
can save you some time.
Troubleshooting Common Issues in Datetime Conversion
Converting datetime to string in Python is not always a smooth process. You may encounter issues, especially when dealing with different date and time formats. Let’s discuss some common problems and their solutions.
Handling ‘ValueError’
One of the most common errors during datetime conversion is the ‘ValueError’. This error occurs when you use an incorrect format code in the strftime()
function. Here’s an example:
from datetime import datetime
# current date and time
date_time = datetime.now()
# incorrect format specification
format = '%Y-%m-%d %H:%M:%Q' # %Q is not a valid format code
# applying strftime() to format the datetime
try:
string = date_time.strftime(format)
except ValueError as e:
print('Error:', e)
# Output:
# Error: 'Q' is a bad directive in format '%Y-%m-%d %H:%M:%Q'
In this example, we’ve used ‘%Q’, which is not a valid format code, leading to a ‘ValueError’. The solution is simple: always ensure you’re using valid format codes with the strftime()
function.
Dealing with Different Formats
Another common issue is dealing with different date and time formats. For instance, if you’re working with a 12-hour format, you need to use ‘%I’ for hours instead of ‘%H’. Here’s an example that illustrates this:
from datetime import datetime
# current date and time
date_time = datetime.now()
# 12-hour format specification
format = '%Y-%m-%d %I:%M:%S %p' # %I is for 12-hour format, %p is for AM/PM
# applying strftime() to format the datetime
string = date_time.strftime(format)
print('Date and Time:', string)
# Output:
# Date and Time: 2022-06-30 03:30:00 PM
In this example, we’ve used ‘%I’ for hours and ‘%p’ for AM/PM to handle the 12-hour format. Always ensure you’re using the correct format codes for your specific needs.
Understanding Python’s Datetime Module and String Data Type
To fully grasp the conversion of datetime to string in Python, it’s crucial to understand the basics of Python’s datetime module and string data type.
Python’s Datetime Module
Python’s datetime module supplies classes to manipulate dates and times. The datetime class within this module is used to represent a point in time. Here’s a simple example of its usage:
from datetime import datetime
# current date and time
date_time = datetime.now()
print(date_time)
# Output:
# 2022-06-30 15:30:00.000000
In this example, we use the datetime.now()
function to get the current date and time. The output is a datetime object representing the current date and time.
Python’s String Data Type
In Python, strings are sequences of character data. The string type in Python is called ‘str’. String literals can be enclosed by either double or single quotes, although single quotes are more commonly used. Here’s a simple string assignment:
# a string
message = 'Hello, Python!'
print(message)
# Output:
# Hello, Python!
In this example, we assign a string to the variable ‘message’ and then print it. The output is the string ‘Hello, Python!’.
Date and Time Formats
When converting datetime to string, it’s important to understand different date and time formats. The strftime()
function uses format codes to specify the output format.
For instance, ‘%Y’ represents the full year, ‘%m’ represents the month, ‘%d’ represents the day, ‘%H’ represents the hour (24-hour format), ‘%M’ represents the minute, and ‘%S’ represents the second. You can combine these format codes to create a format that suits your needs.
By understanding Python’s datetime module, string data type, and different date and time formats, we can better understand the process of converting datetime to string in Python.
Exploring Related Concepts
Once you’ve mastered the conversion of datetime to string in Python, you might want to explore the following resources:
- Python Time: Tips and Techniques – Explore Python’s time module for tracking and managing time-based events in your applications.
Simplifying Date and Time Operations in Python – Master datetime manipulation in Python for various time-related tasks.
strftime in Python: Formatting Date and Time – Unleash the power of Python’s strftime function for date and time objects.
Handling Time Zones in Python – Python’s official documentation on managing time zones with the datetime module.
Performing Date and Time Arithmetic – Explore datetime arithmetic and timedelta objects in Python.
Working with Timestamps in Python – Understand how to use timestamps in the datetime module of Python.
Wrapping Up:
Throughout this guide, we’ve journeyed from the basics to the advanced aspects of converting datetime to string in Python. We’ve explored the strftime()
function’s usage, which offers high flexibility with customizable output formats. We’ve also discussed common issues like handling ‘ValueError’ and dealing with different date and time formats, providing solutions and workarounds for each.
We dove into alternative approaches such as isoformat()
and __str__()
, both offering less flexibility but proving convenient for quick conversions with standard formats. Here’s a quick comparison of these methods:
Method | Flexibility | Output Format |
---|---|---|
strftime() | High | Customizable |
isoformat() | Low | ‘YYYY-MM-DDTHH:MM:SS’ |
__str__() | Low | ‘YYYY-MM-DD HH:MM:SS.ssssss’ |
Remember, the method you choose depends on your specific needs and the level of customization you require. As always, happy coding and continue exploring the vast world of Python!