Python strftime() | Master Date and Time Formatting
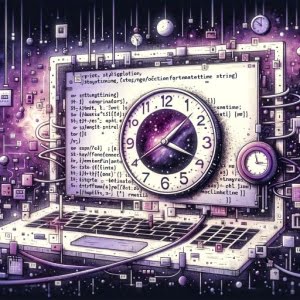
Are you grappling with date and time formatting in Python? Just like a proficient watchmaker, Python’s strftime function is your tool to mold date and time data into any format you require.
In this guide, we’ll navigate the intricacies of strftime. We’ll start from the basics, gradually moving to more advanced techniques. By the end of this guide, you’ll be able to wield strftime like a pro, turning raw date and time data into useful, formatted strings.
So, let’s dive in and start mastering strftime in Python together.
TL;DR: How Do I Use strftime in Python?
The strftime function in Python is your go-to tool for formatting date and time objects into strings. Here’s a simple example to illustrate:
from datetime import datetime
now = datetime.now()
formatted_date = now.strftime('%Y-%m-%d')
print(formatted_date)
# Output:
# '2022-01-01'
This code snippet grabs the current date and time using datetime.now()
, and then formats it into a string using strftime
. The format '%Y-%m-%d'
specifies that the date should be displayed in the format ‘Year-Month-Day’.
This is just a glimpse of what strftime can do. Keep reading for more in-depth examples and advanced usage tips.
Table of Contents
- strftime in Python: Basic Usage
- strftime in Python: Intermediate Techniques
- Exploring Alternatives to strftime in Python
- Troubleshooting strftime in Python
- Understanding Python’s Datetime Module and strftime
- Real-World Applications of strftime in Python
- Further Learning
- Wrapping Up: Mastering strftime in Python
strftime in Python: Basic Usage
The strftime function, which stands for ‘string format time’, is part of Python’s datetime module. It’s used to convert date and time objects into strings, allowing you to present them in a more human-readable format.
Let’s take a look at a basic example:
from datetime import datetime
current_time = datetime.now()
formatted_time = current_time.strftime('%H:%M:%S')
print(formatted_time)
# Output:
# '14:30:15'
In this code snippet, we first import the datetime module. We then use the datetime.now()
function to get the current date and time. The strftime
function is then used to format this datetime object into a string. The format '%H:%M:%S'
specifies that the time should be displayed in the format ‘Hour:Minute:Second’.
This basic application of strftime is incredibly useful for tasks such as logging or displaying timestamps in a user interface.
However, keep in mind that the strftime function uses specific format codes to represent date and time components (like %H for hour, %M for minute, and %S for second). Using an incorrect format code will result in an error, so it’s crucial to get familiar with the different format codes available in Python’s strftime.
strftime in Python: Intermediate Techniques
As you become more familiar with strftime, you can start to use it in more complex ways. For instance, strftime can handle different locales, which allows you to display dates and times according to the conventions of different countries or regions.
Let’s look at an example where we format the current date in a specific locale:
import locale
from datetime import datetime
# Set the locale to French
locale.setlocale(locale.LC_TIME, 'fr_FR')
current_time = datetime.now()
formatted_time = current_time.strftime('%A %d %B %Y')
print(formatted_time)
# Output:
# 'mardi 01 février 2022'
In this code snippet, we first import the locale module and set the locale to French using locale.setlocale(locale.LC_TIME, 'fr_FR')
. We then use strftime to format the current date into a string. The format '%A %d %B %Y'
specifies that the date should be displayed as ‘Weekday Day Month Year’. Because we’ve set the locale to French, strftime outputs the date in French.
Another advanced use of strftime is the ability to use custom format codes. For instance, you can use %j
to get the day of the year (from 001 to 366). Let’s see this in action:
from datetime import datetime
current_time = datetime.now()
formatted_time = current_time.strftime('Today is the %jth day of the year')
print(formatted_time)
# Output:
# 'Today is the 032nd day of the year'
In this example, we’ve used the format code %j
within a custom string to display the current day of the year. This demonstrates how strftime can be used to create custom date and time strings that suit your specific needs.
Exploring Alternatives to strftime in Python
While strftime is a powerful tool for formatting dates and times in Python, it’s not the only option available. There are alternative approaches that can provide additional flexibility or simplicity, depending on your needs.
Using F-Strings for Date and Time Formatting
Python’s f-strings (formatted string literals) can be used to format datetime objects in a more intuitive way. Here’s how you can do it:
from datetime import datetime
current_time = datetime.now()
formatted_time = f'{current_time:%Y-%m-%d}'
print(formatted_time)
# Output:
# '2022-02-01'
In this example, we’ve used an f-string to format the current date. The format specifier %Y-%m-%d
is included directly in the string, making the code more readable.
Leveraging Third-Party Libraries
There are also third-party libraries like Arrow and Pendulum that provide more robust and user-friendly ways to handle date and time formatting. These libraries offer additional features such as timezone conversion, parsing strings into datetime objects, and more.
Here’s an example using Pendulum:
import pendulum
current_time = pendulum.now()
formatted_time = current_time.to_date_string()
print(formatted_time)
# Output:
# '2022-02-01'
In this snippet, we’ve used Pendulum’s to_date_string
method to format the current date. This method automatically formats the date in the ‘Year-Month-Day’ format, eliminating the need to remember format codes.
Choosing between strftime, f-strings, and third-party libraries depends on your specific needs. If you need more advanced features or find strftime’s format codes hard to remember, using a third-party library like Arrow or Pendulum might be a better choice. On the other hand, if you’re looking for a simple and straightforward way to format dates and times without installing additional libraries, using strftime or f-strings would be more suitable.
Troubleshooting strftime in Python
While strftime is a powerful tool, it’s not without its quirks. Here are some common issues you might encounter when using strftime, along with their solutions.
Dealing with Timezone-Aware and Naive Datetime Objects
One common issue arises when dealing with timezone-aware and naive datetime objects. A naive datetime object has no timezone information, while a timezone-aware datetime object does. If you try to use strftime on a timezone-aware datetime object without handling the timezone information, you could end up with unexpected results.
Here’s an example:
from datetime import datetime
import pytz
# Create a timezone-aware datetime object
ny_time = datetime.now(pytz.timezone('America/New_York'))
formatted_time = ny_time.strftime('%Y-%m-%d %H:%M:%S %Z%z')
print(formatted_time)
# Output:
# '2022-02-01 12:30:15 EST-0500'
In this code snippet, we’ve created a timezone-aware datetime object using the pytz library. The strftime format '%Y-%m-%d %H:%M:%S %Z%z'
includes %Z
for the timezone name and %z
for the UTC offset. This ensures that the timezone information is correctly displayed in the formatted string.
If you’re dealing with naive datetime objects and want to add timezone information, you can use the pytz
library to do so. Conversely, if you have a timezone-aware datetime object and want to make it naive (remove the timezone information), you can use the replace
method of the datetime object to set the tzinfo
attribute to None
.
By understanding these nuances of strftime and datetime objects in Python, you can avoid common pitfalls and ensure your date and time formatting is accurate and reliable.
Understanding Python’s Datetime Module and strftime
Before diving deeper into strftime, let’s take a step back and understand the fundamentals. At the heart of strftime is Python’s datetime module. This module supplies classes for manipulating dates and times in both simple and complex ways. It’s a built-in module, which means you don’t need to install anything to use it.
One of the most commonly used classes from the datetime module is the datetime class, which combines information about the date and time. You can create a datetime object that represents the current date and time using the datetime.now()
function, like so:
from datetime import datetime
current_time = datetime.now()
print(current_time)
# Output:
# 2022-02-01 14:30:15.123456
In this example, the datetime.now()
function returns a datetime object representing the current date and time down to the microsecond.
The strftime function is another important part of the datetime module. It’s a method of the datetime class that converts a datetime object into a string according to a specified format. The ‘str’ in strftime stands for ‘string’, while ‘f’ stands for ‘format’. The ‘time’ part indicates that it’s part of the time module.
The format is specified using format codes, which are special directives that get replaced by the corresponding value from the datetime object. For instance, %Y
gets replaced by the four-digit year, %m
by the two-digit month, and %d
by the two-digit day. There are many format codes available, allowing you to create date and time strings in virtually any format you need.
Understanding the role of the datetime module and the concept of format codes is crucial for mastering strftime in Python. With this foundation, you can use strftime to create custom date and time strings that suit your specific needs.
Real-World Applications of strftime in Python
The power of strftime isn’t limited to formatting dates and times in a Python shell or script. It has a wide range of applications in real-world scenarios.
Logging
Logging is a common use case of strftime. When you’re logging events in an application, you often need to timestamp each event. strftime allows you to format these timestamps in a way that’s easy to read and understand.
import logging
from datetime import datetime
logging.basicConfig(filename='app.log', level=logging.INFO)
def log_message(message):
logging.info(f'{datetime.now().strftime('%Y-%m-%d %H:%M:%S')}: {message}')
log_message('This is a log message.')
# Check the contents of app.log
# Output in app.log:
# 2022-02-01 14:30:15: This is a log message.
In this code snippet, we’ve defined a log_message
function that logs a message with a timestamp. The timestamp is formatted using strftime.
Data Analysis
strftime is also useful in data analysis. When you’re working with datasets that include dates and times, you often need to format these dates and times in a certain way to facilitate your analysis.
Web Development
In web development, strftime can be used to format dates and times for display on a web page. This allows you to present dates and times in a user-friendly format.
These are just a few examples of how strftime can be used in real-world scenarios. The possibilities are virtually endless.
Further Learning
If you’re interested in learning more about handling dates and times in Python, we have provided several related resources to explore.
- Python Time: Understanding Time Management – Explore Python’s time module for scheduling tasks, measuring time intervals, and more.
Python Datetime to String: Formatting Date and Time – Convert datetime objects to strings with the datetime module.
Python Timedelta: Calculating Time Differences – Explore Python’s timedelta module for easy manipulation of time intervals.
Python String to DateTime Conversion – Understand how to seamlessly convert strings into DateTime objects in Python.
Getting Current Time in Python – Learn to fetch the current time using Python’s datetime module.
Python Programming DateTime Guide on Programiz can help you understand and work with the datetime module in Python.
These topics would complement your understanding of strftime and broaden your skills in handling dates and times in Python.
Wrapping Up: Mastering strftime in Python
Throughout this guide, we’ve explored the power and flexibility of Python’s strftime function. We started with the basics, learning how to use strftime to convert datetime objects into formatted strings. We then delved into intermediate techniques, such as handling different locales and using custom format codes.
We also looked at common issues that can arise when using strftime, such as dealing with timezone-aware and naive datetime objects, and how to solve them. In addition, we explored alternative approaches for handling date and time formatting, including using f-strings and third-party libraries like Arrow and Pendulum.
Here’s a quick comparison of the methods we discussed:
Method | Advantages | Disadvantages |
---|---|---|
strftime | Built-in, powerful, customizable | Requires memorizing format codes |
f-strings | More readable, intuitive | Limited formatting options |
Third-party libraries (Arrow, Pendulum) | More features, user-friendly | Requires installation, may be overkill for simple tasks |
Remember, the best method depends on your specific needs. If you need a simple and straightforward solution, strftime or f-strings might be the best choice. If you need more advanced features, a third-party library could be the way to go.
By mastering strftime and understanding its alternatives, you’ll be well-equipped to handle any date and time formatting task in Python. Happy coding!