Python timedelta() DateTime | Usage and Examples Guide
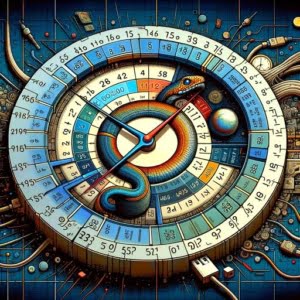
Working with date and time calculations in Python can often feel feel frustrating. But fear not: This is where Python’s timedelta comes in. This function, nestled within the datetime module, is like a Swiss Army knife for date and time manipulations.
Whether you’re calculating the difference between two dates, adding or subtracting time from a particular date, or even performing more complex time-based calculations, timedelta is your go-to tool.
By the end of this guide, you’ll have a firm grasp of timedelta’s core functionalities and how you can leverage its power in your Python programming journey. So, let’s unravel the complexities of Python’s timedelta together!
TL;DR: What is Python’s timedelta and how do I use it?
Python’s timedelta is a function within the datetime module used for calculating differences in dates and times. You can use it to add or subtract time from a particular date, calculate the difference between two dates, and more.
Here’s a simple example of using timedelta:
from datetime import datetime, timedelta
today = datetime.now()
future_date = today + timedelta(days=10)
print(future_date)
In this code, we’re getting the current date using datetime.now() and adding 10 days to it using timedelta(days=10).
Read on for more advanced methods, background, tips and tricks.
Table of Contents
Using timedelta: The basics
Python’s timedelta is a function within the datetime module. It represents a duration, the difference between two dates or times. It’s a versatile tool that can handle both positive and negative values, making it ideal for a wide range of time-based calculations.
Let’s kick things off with a simple example. Imagine you need to calculate the date 10 days from today. Here’s how you can utilize timedelta to accomplish that:
from datetime import datetime, timedelta
today = datetime.now()
future_date = today + timedelta(days=10)
print(future_date)
In this code, we’re importing the datetime and timedelta functions from the datetime module. We’re then getting the current date using datetime.now() and adding 10 days to it using timedelta(days=10).
Timedelta Parameters
Timedelta can handle weeks, hours, minutes, seconds, and even microseconds. This flexibility makes timedelta a powerful tool for a wide range of time-based calculations.
Parameter | Description | Example |
---|---|---|
weeks | Number of weeks | timedelta(weeks=1) |
days | Number of days | timedelta(days=1) |
hours | Number of hours | timedelta(hours=1) |
minutes | Number of minutes | timedelta(minutes=1) |
seconds | Number of seconds | timedelta(seconds=1) |
microseconds | Number of microseconds | timedelta(microseconds=1) |
For example, if you want to calculate the time 2 hours and 30 minutes from now, you can do it like this:
from datetime import datetime, timedelta
today = datetime.now()
future_time = today + timedelta(hours=2, minutes=30)
print(future_time)
Calculating time differences
One core application of timedelta is calculating the difference between two specific dates.
For instance, if you want to calculate the number of days between two dates, here’s how you can do it:
from datetime import datetime, timedelta
date1 = datetime(2022, 1, 1)
date2 = datetime(2022, 12, 31)
difference = date2 - date1
print(difference.days)
In this example, We do the following:
- Create two datetime objects, date1 and date2, representing the dates January 1, 2022, and December 31, 2022, respectively.
Subtract date1 from date2, which gives us a timedelta object.
Finally, we’re printing the number of days in that timedelta object.
Avoiding Common Pitfalls with Timedelta
While timedelta is a powerful tool, it does have its quirks. When it comes to leap years and daylight savings time, additional care and attention is warranted.
We’ll go over both of these problems along with their solutions below:
Leap Years
One common pitfall is the handling of leap years. When calculating the difference between two dates a year apart, timedelta might give you 365 days, even if a leap day is included in that range. To avoid this, you can use the date.toordinal() function, which correctly accounts for leap years.
Here’s an example that demonstrates the situation:
from datetime import datetime, timedelta
# Using timedelta
date1 = datetime(2020, 2, 28) # just before a leap day
date2 = datetime(2021, 2, 28)
delta = date2 - date1
print(delta.days) # outputs: 365
# Using date.toordinal()
ordinal_diff = date2.toordinal() - date1.toordinal()
print(ordinal_diff) # outputs: 366
In this example, there’s a leap day (Feb 29, 2020) between date1
and date2
. The timedelta only counts it as 365 days whereas date.toordinal()
correctly counts it as 366 days. So, when you’re dealing with dates spanning multiple years and leap year considerations matter, it is safer to use date.toordinal()
.
Daylight Savings Time:
Another potential pitfall is the handling of daylight saving time. If you’re adding or subtracting hours using timedelta, it might not correctly account for the one-hour shift in daylight saving time. To avoid this, you can use timezone-aware datetime objects.
This is a scenario that can be a little surprising if we are not aware of how daylight saving time works. Here is an example demonstrating the problem:
from datetime import datetime, timedelta
# Clocks spring forward on March 14, 2021
start = datetime(2021, 3, 14, 1, 30)
end = start + timedelta(hours=1)
print(f"Start: {start}")
print(f"End: {end}")
The output will be:
Start: 2021-03-14 01:30:00
End: 2021-03-14 02:30:00
This seems fine at first glance, but if you’re in a location that observes daylight saving time, the time actually goes from 01:59 straight to 03:00 on March 14, 2021. Therefore, 02:30 doesn’t actually exist on this day!
To correctly handle this, you can use timezone-aware datetime objects:
from datetime import datetime, timedelta, timezone
import pytz
# Specify timezone
tz = pytz.timezone("America/New_York")
# Specify the same start time but now with timezone
start = datetime(2021, 3, 14, 1, 30, tzinfo=tz)
end = start + timedelta(hours=1)
print(f"Start: {start}")
print(f"End: {end}")
the outputs would be displayed like below,
Start: 2021-03-14 01:30:00-04:56
End: 2021-03-14 03:30:00-04:56
Here, the timedelta correctly moves the time forward by one hour from 01:30 to 03:30, skipping the nonexistent 02:30.
Please note, working with dates and times can be tricky and is often very nuanced, so always verify that you are handling these concepts correctly in your own code. Pytz is a powerful library for this purpose.
Interactions Between Timedelta and Other Python Datetime Objects
Timedelta is just one part of Python’s datetime module. It’s often used in conjunction with other datetime objects, like datetime and time.
For example, you can add a timedelta to a datetime to get a new datetime, or subtract two datetimes to get a timedelta. Understanding the interaction between these objects is key to mastering date and time manipulations in Python.
Here’s an example demonstrating both adding timedelta to a datetime and subtracting two datetimes to get a timedelta:
from datetime import datetime, timedelta
# Define a datetime object
dt = datetime.now()
print(f"Current datetime: {dt}")
# Create a timedelta of 5 days
delta = timedelta(days=5)
# Add the timedelta to the datetime
future_dt = dt + delta
print(f"Datetime 5 days from now: {future_dt}")
# Subtract the original datetime from the future datetime
diff = future_dt - dt
print(f"Difference between the two datetimes: {diff}")
Here is the potential output:
Current datetime: 2022-01-04 16:00:00
Datetime 5 days from now: 2022-01-09 16:00:00
Difference between the two datetimes: 5 days, 0:00:00
Breaking down this example is the following steps:
- In this example, we are first defining a current datetime, then we are creating a timedelta of 5 days.
We add this delta to the current datetime to get a datetime object representing 5 days in the future.
Finally, when we subtract the original datetime object from the future datetime object.
Python then returns a timedelta representing the difference between these two dates, which is indeed 5 days.
Effective Usage of Timedelta: Tips and Tricks
Knowing a few tips and tricks can enhance your usage of timedelta.
Time Format
It’s important to remember that timedelta objects are normalized into days, seconds, and microseconds. This means that if you create a timedelta object with a number of hours, minutes, or weeks, they will be automatically converted into days, seconds, and microseconds.
Keep in mind that timedelta objects can be both positive and negative. This can be particularly useful when calculating differences in dates or times.
Limitations of timedelta
Timedelta cannot handle dates before the year 1 or after the year 9999. Also, it cannot handle time units larger than days, such as months or years, because these units have variable lengths.
To navigate these limitations, you can use other functions in the datetime module, such as date and datetime.
Here’s an example of using timedelta with limitations and workarounds:
Timedelta cannot handle dates before the year 1:
from datetime import datetime, timedelta
dt = datetime(year=1, month=1, day=1)
past = dt - timedelta(days=1) # throws an error
In the above example, subtracting a day from the first day of the year 1 will result in an error because it results in a date before the year 1.
Timedelta cannot represent a period of 1 month. This is because months have different numbers of days:
# This doesn't work
month=timedelta(months=1) # throws an error
As a workaround, datetime can be used:
from datetime import datetime
from dateutil.relativedelta import relativedelta
dt = datetime(year=2022, month=1, day=1)
dt_next_month = dt + relativedelta(months=1)
print(dt_next_month)
In this code sample, we’re taking a datetime representing January 1, 2022, and adding a month to it using relativedelta
. The result, dt_next_month
, represents February 1, 2022, as expected.
Please note,
relativedelta
is a function from thedateutil
module, notdatetime
. It’s a third-party module that provides powerful extensions todatetime
. You may need to install it withpip install python-dateutil
if it’s not already installed.
Diving Deeper: Python’s datetime Module
While timedelta is a powerful tool in its own right, it’s just one cog in a larger machine: Python’s datetime module. This module is a comprehensive toolkit for manipulating dates and times in Python.
It includes a variety of functions for parsing dates and times from strings, formatting dates and times into strings, handling timezones, and much more.
Exploring Other Functionalities of the datetime Module
The datetime module is packed with many other important functionalities. For example, the datetime function can be used to create datetime objects, which represent a specific point in time. The time function can be used to create time objects, which represent a time of day. The date function can be used to create date objects, which represent a date (year, month, day).
The datetime module also includes functions for handling timezones, such as tzinfo and timezone. These functions allow you to create timezone-aware datetime objects, which can accurately handle daylight saving time and other timezone-related complexities.
Additional Python Date and Time Tools
While timedelta is a powerful tool for manipulating dates and times, Python offers several other tools and libraries that provide additional functionalities.
For instance, the dateutil library extends the datetime module with additional features, like parsing dates from strings with fuzzy matching, computing relative deltas, and more. There’s also the pytz library, which provides a comprehensive set of timezone information.
Library | Unique Features | Use Cases |
---|---|---|
dateutil | Parsing dates from strings with fuzzy matching, computing relative deltas | When you need to parse dates from strings, compute relative deltas |
pytz | Provides a comprehensive set of timezone information | When you need to handle timezone-related complexities |
Further Resources for Python Time Modules
These additional materials will help your usage of time-related functions in Python:
- Python Time Explained: Core Concepts – Discover Python’s time module for efficient handling of time-related tasks in your projects.
Date and Time Formatting in Python – Explore Python’s strftime function for precise control over date and time formatting.
Python Timeit: Benchmarking Code Performance – Benchmark code performance accurately with Python’s timeit module.
Python Time Module Tutorial – A comprehensive guide to using Python’s native time module.
Getting Current Time in Python – Learn how to fetch and manipulate the current time in Python.
Python Date Usage – Master the usage of dates in Python programming with this easy-to-understand guide.
Immerse yourself in these educational materials to advance your understanding and add valuable tools to your Python programmer’s repertoire. Always remember, mastery comes with continuous learning.
Concluding Thoughts
Throughout this comprehensive guide, we’ve explored the functionalities of Python’s timedelta, a potent tool for manipulating dates and times.
We’ve delved into its basic functionalities, which range from simple operations like adding and subtracting days to more complex time-based calculations. We’ve also demonstrated its advanced applications and use cases, as well as alternatives and complementary libraries and functions.
We’ve explored the possible pitfalls of using timedelta. By understanding timedelta’s behavior and its limitations, you can fully leverage its capabilities and steer clear of common mistakes.
By mastering timedelta, you can augment your Python toolkit, write more efficient and effective code, and explore new possibilities in your Python programming journey.