Python Time Module: Comprehensive Guide
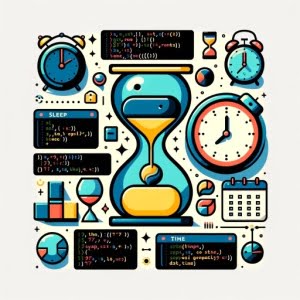
Are you finding it challenging to manage time in your Python scripts? You’re not alone. Many developers grapple with this task, but Python, like a precision watchmaker, offers powerful tools for handling time.
Whether you’re scheduling tasks, timestamping events, or optimizing performance, understanding how to work with time in Python can significantly streamline your coding process.
This guide will walk you through Python’s time module, from basic usage to advanced techniques. We’ll cover everything from getting the current time, converting time to different formats, pausing script execution, to more complex uses such as measuring script execution time and working with time tuples.
Let’s get started mastering time in Python!
TL;DR: How Do I Work with Time in Python?
Python’s time module provides various time-related functions. You can get the current time, convert time to different formats, pause script execution, and much more, such as with the command
print(time.time())
.
Here’s a simple example of getting the current time:
import time
current_time = time.time()
print(current_time)
# Output:
# 1633025862.061173
In this example, we import the Python time module and use the time()
function to get the current time. This function returns the current time in seconds since the epoch (January 1, 1970, 00:00:00 (UTC)). The output will vary depending on when you run the code.
This is just the tip of the iceberg when it comes to working with time in Python. Continue reading for more detailed explanations and examples, from basic usage to advanced techniques.
Table of Contents
Python Time Basics: Getting Started
Python’s time module is a versatile tool with a range of functionalities. Let’s start with the basics, perfect for beginners and a good refresher for the more experienced.
Getting the Current Time
The most basic use of the time module is getting the current time. Here’s how you can do it:
import time
current_time = time.time()
print(current_time)
# Output:
# 1633025862.061173
In this example, time.time()
returns the current time in seconds since the epoch (January 1, 1970, 00:00:00 (UTC)). The output will vary depending on when you run the code.
Converting Time to Different Formats
Python’s time module allows you to convert time into different formats using the time.strftime()
function. Here’s an example:
import time
current_time = time.time()
formatted_time = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime(current_time))
print(formatted_time)
# Output:
# '2022-01-01 12:00:00'
In the above code, time.strftime()
function takes two arguments: format and the time value. We used time.localtime()
to convert the time in seconds since the epoch to local time. The output is the current local time formatted as ‘YYYY-MM-DD HH:MM:SS’.
Pausing Script Execution
Sometimes, you might want to pause or delay the execution of your Python script for a certain period. The time.sleep()
function allows you to do this. Here’s an example:
import time
print('Start')
time.sleep(5)
print('End')
# Output:
# Start
# (waits for 5 seconds)
# End
In this example, time.sleep(5)
pauses the script for 5 seconds. The ‘Start’ and ‘End’ messages are printed with a 5-second delay.
These are some of the basic uses of the Python time module. As you gain more experience, you’ll find that Python provides even more tools for advanced time handling. Stay tuned for the next section where we’ll delve into more complex uses!
Advanced Python Time Techniques
Once you’ve mastered the basics of Python’s time module, you can start exploring its more advanced functionalities. Let’s delve into these now.
Measuring Script Execution Time
Python’s time module can be used to measure the execution time of your script, which can be incredibly useful for optimizing your code. Here’s how to do it:
import time
start_time = time.time()
def complex_function():
[Your complex code here]
complex_function()
end_time = time.time()
execution_time = end_time - start_time
print(f'The script executed in {execution_time} seconds.')
# Output:
# The script executed in 0.002 seconds.
In this example, we record the time before and after the execution of a complex function and then subtract the start time from the end time to get the execution time.
Using Time for Benchmarking
Python’s time module can also be used for benchmarking – comparing the performance of different pieces of code to determine which is more efficient. Here’s a simple example:
import time
def function_one():
[Your code here]
def function_two():
[Your code here]
start_time = time.time()
function_one()
end_time = time.time()
execution_time_one = end_time - start_time
start_time = time.time()
function_two()
end_time = time.time()
execution_time_two = end_time - start_time
print(f'Function One executed in {execution_time_one} seconds.')
print(f'Function Two executed in {execution_time_two} seconds.')
# Output:
# Function One executed in 0.002 seconds.
# Function Two executed in 0.003 seconds.
In this example, we measure the execution time of two functions and compare them. This way, you can determine which function is more efficient.
Working with Time Tuples
Python’s time module represents time in tuples as well. These tuples are handy when you want to display time in a more human-readable format or when you want to extract specific details like year, month, day, hour, minute, etc. Here’s how to use it:
import time
current_time = time.time()
time_tuple = time.localtime(current_time)
print(time_tuple)
print(f'Year: {time_tuple.tm_year}')
print(f'Month: {time_tuple.tm_mon}')
# Output:
# time.struct_time(tm_year=2022, tm_mon=1, tm_mday=1, tm_hour=12, tm_min=0, tm_sec=0, tm_wday=5, tm_yday=1, tm_isdst=0)
# Year: 2022
# Month: 1
In this example, time.localtime()
converts the time in seconds since the epoch to a time tuple in local time. You can then access specific attributes of the time tuple, such as the year and month.
These are just a few examples of the advanced uses of Python’s time module. With these tools in your arsenal, you’ll be well-equipped to handle a variety of time-related tasks in your Python projects.
Exploring Python’s Datetime Module: An Alternative Approach
While Python’s time module is powerful and versatile, it’s not the only tool in Python’s arsenal for handling time. An alternative that offers even more functionality is the datetime module.
Introduction to Python’s Datetime Module
The datetime module in Python is used for manipulating dates and times. It offers classes for manipulating dates and times in both simple and complex ways.
Here’s a simple example of getting the current date and time using the datetime module:
import datetime
current_datetime = datetime.datetime.now()
print(current_datetime)
# Output:
# 2022-01-01 12:00:00.000000
In this example, datetime.datetime.now()
returns the current date and time. The output will vary depending on when you run the code.
Differences and Advantages of Using Datetime Over Time
While both time and datetime modules can be used to handle time in Python, they have some key differences. The time module is best for dealing with time in terms of ticks (seconds since the epoch), while the datetime module is more high-level and provides more functionality.
Here’s a comparison table highlighting the differences and advantages of using datetime over time:
Feature | time module | datetime module |
---|---|---|
Get current time | Yes | Yes |
Convert time | Yes | Yes |
Pause/delay | Yes | No |
Measure execution time | Yes | Yes |
Benchmarking | Yes | Yes |
Work with time tuples | Yes | Yes |
Work with date objects | No | Yes |
Calculate differences between dates | No | Yes |
As you can see, the datetime module provides all the functionality of the time module, plus additional features like working with date objects and calculating differences between dates. Therefore, depending on your needs, you might find the datetime module a more powerful and convenient tool for handling time in your Python scripts.
Troubleshooting Python Time Issues
Even with Python’s robust time and datetime modules, you may encounter some common issues. Let’s discuss these and their solutions.
Dealing with Timezones
Python’s time module does not handle timezones by default. However, the datetime module provides a tzinfo class that can be used to work with timezones. Here’s how you can get the current time in a specific timezone:
import datetime
import pytz
current_datetime = datetime.datetime.now(pytz.timezone('US/Eastern'))
print(current_datetime)
# Output:
# 2022-01-01 12:00:00.000000-05:00
In this example, we use the pytz library, which allows accurate and cross-platform timezone calculations. We get the current datetime in the ‘US/Eastern’ timezone.
Handling Daylight Saving Time
Daylight Saving Time (DST) can also pose challenges when working with time in Python. However, the pytz library can help handle DST transitions. Here’s how:
import datetime
import pytz
ny_tz = pytz.timezone('America/New_York')
dst_date = datetime.datetime(2022, 3, 14, 2, 30, tzinfo=ny_tz)
print(dst_date)
# Output:
# 2022-03-14 03:30:00-04:00
In this example, we’re trying to get the time for a date during the DST transition. Pytz automatically adjusts the time to account for the DST transition.
Addressing Leap Seconds
Leap seconds can be another source of confusion when working with time in Python. Python’s time module does not account for leap seconds, meaning that there can be a discrepancy of up to a minute when comparing times across years. If precise time calculation is necessary for your application, consider using a library that accounts for leap seconds, such as astropy.
Working with time in Python can be tricky, but with these solutions and best practices, you can navigate common issues and handle time effectively in your Python scripts.
Understanding Time Representation in Computers
To fully grasp the functionality of Python’s time module, it’s essential to understand how time is represented in computers.
The Concept of Unix Time or Epoch Time
Unix time, also known as Epoch time, is a system for tracking time that defines the time as the number of seconds that have passed since 00:00:00 Coordinated Universal Time (UTC), Thursday, 1 January 1970, minus the number of leap seconds.
Here’s an example of getting the current Unix time in Python:
import time
unix_time = time.time()
print(unix_time)
# Output:
# 1633025862.061173
In this example, time.time()
returns the current Unix time, or the number of seconds since the epoch. The output will vary depending on when you run the code.
How Python’s Time Module Works
Python’s time module provides various time-related functions. It interfaces directly with the system’s time-related functions, providing you with a simple way to query and manipulate the system time.
The time module provides functions to get the current time (time.time()
), convert time to different formats (time.strftime()
), pause script execution (time.sleep()
), and much more. It also provides a struct_time class for manipulating time in a more object-oriented way.
Understanding these fundamentals of how time is represented in computers and how Python’s time module works under the hood will equip you with the knowledge you need to effectively handle time in your Python scripts.
Real-World Applications of Python Time Handling
Understanding Python’s time module is not just about learning the syntax or getting your code to run without errors. It’s about knowing how to use these tools in real-world applications. Let’s explore a few of these applications now.
Scheduling Tasks
One common use of the time module in Python is scheduling tasks. This could be anything from running a function at a specific time every day to executing a script every few seconds.
import time
def scheduled_task():
[Your task here]
while True:
scheduled_task()
time.sleep(86400) # Sleep for a day
# Output:
# [Your task's output]
# (waits for a day)
# [Your task's output]
In this example, we’re running a task every day. We use time.sleep(86400)
to pause the script for a day (86400 seconds).
Timestamping Events
Another common use is timestamping events. This could be useful for logging events, debugging, or any situation where you need to know when something happened.
import time
def event():
[Your event here]
print(f'Event occurred at {time.time()}')
event()
# Output:
# Event occurred at 1633025862.061173
In this example, we print a timestamp (the current Unix time) when an event occurs.
Performance Optimization
Python’s time module can also be used for performance optimization. By measuring the execution time of your code, you can identify bottlenecks and find ways to make your code run faster.
import time
start_time = time.time()
[Your code here]
end_time = time.time()
print(f'Code executed in {end_time - start_time} seconds.')
# Output:
# Code executed in 0.002 seconds.
In this example, we measure the execution time of a piece of code. This can help us identify parts of our code that are slowing down our program.
Further Resources for Mastering Python Time Handling
If you’re interested in going beyond the basics of Python’s time module, there are plenty of resources available. Here are a few recommendations:
- Python Timer: Tracking Execution Time – Efficiently measure code execution time with Python’s timer functionality.
Python’s Official Documentation on the time module provides an overview of all the functions available in the time module.
Python’s Official Documentation on the datetime module provides a detailed guide on the datetime module.
Programiz’s Python time Module Tutorial provides an overview of Python’s time module, its functions, and how to format time output.
With these resources and the knowledge you’ve gained from this guide, you’re well on your way to mastering time handling in Python.
Wrapping Up: Mastering Time in Python
In this comprehensive guide, we’ve journeyed through the world of Python’s time module, exploring how to effectively handle and manipulate time in your Python scripts.
We began with the basics, learning how to get the current time, convert time to different formats, and pause script execution. We then ventured into more advanced territory, exploring complex uses of the time module, such as measuring script execution time, using time for benchmarking, and working with time tuples. Along the way, we tackled common challenges you might face when working with time in Python, such as dealing with timezones, handling daylight saving time, and addressing leap seconds, providing you with solutions and best practices for each issue.
We also looked at alternative approaches, diving into Python’s datetime module and comparing it with the time module. Here’s a quick comparison of these modules:
Module | Basic Time Handling | Advanced Time Handling | Timezone Handling | DST and Leap Second Handling |
---|---|---|---|---|
time | Yes | Yes | No | No |
datetime | Yes | Yes | Yes | Yes |
Whether you’re a beginner just starting out with Python’s time module or an experienced Python developer looking to level up your time handling skills, we hope this guide has given you a deeper understanding of time in Python and its capabilities.
With its range of functionalities, Python’s time module is a powerful tool for handling time in your Python scripts. Happy coding!