Java Time: Your Complete Guide to Time Management
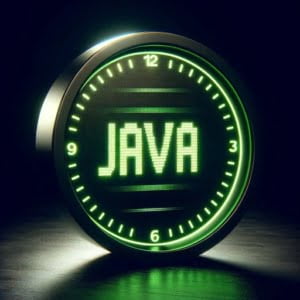
Are you finding it challenging to manage time in Java? You’re not alone. Many developers find themselves grappling with Java’s date and time APIs, but rest assured, there’s a way to make it simpler.
Think of Java’s time management tools as a precise clock – they offer robust and reliable ways to work with time, from getting the current time to formatting and manipulating time.
In this guide, we’ll walk you through the process of mastering time in Java, from the basics to more advanced techniques. We’ll cover everything from using the LocalTime
class and its methods, to more complex uses of Java’s date and time APIs, and even alternative approaches.
Let’s get started and become a master of time in Java!
TL;DR: How Do I Get the Current Time in Java?
To get the current time in Java, you can use the
LocalTime.now()
method from Java’sjava.time
package.
Here’s a simple example:
LocalTime now = LocalTime.now();
System.out.println(now);
# Output:
# Current time in the format: HH:MM:SS.SSS
In this example, we’re using the LocalTime.now()
method to fetch the current time. This method returns the current time from the system clock in the default time-zone. The System.out.println(now);
line then prints this time.
This is a basic way to get the current time in Java, but there’s much more to learn about managing date and time in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Harnessing LocalTime in Java
- Formatting Time with DateTimeFormatter
- Performing Operations with Duration and Period
- Exploring Alternative Date-Time APIs in Java
- Navigating Common Java Time Issues
- Java Time Handling: The Fundamentals
- Time Handling in Larger Java Applications
- Further Resources for Mastering Java Time
- Wrapping Up: Time in Java
Harnessing LocalTime in Java
Java provides a class named LocalTime
within the java.time
package, which is designed to represent the time of day. This class provides several methods to work with time in Java, making it a valuable tool for any developer.
Fetching the Current Time
To get the current time, you can use the now()
method of the LocalTime
class. Here’s a simple example:
LocalTime currentTime = LocalTime.now();
System.out.println('Current time is: ' + currentTime);
# Output:
# 'Current time is: ' followed by current time in the format: HH:MM:SS.SSS
In this example, the now()
method retrieves the current time from the system clock based on your default time-zone. The System.out.println
line then prints this time.
Advantages and Pitfalls
The LocalTime
class is a powerful tool for handling time in Java. It provides precise time information, down to the nanosecond, and it’s easy to use. However, it’s important to note that LocalTime
does not store or represent a date or time-zone. For date-related operations or if you need to work with time-zones, other classes in the java.time
package would be more appropriate.
Formatting Time with DateTimeFormatter
Java provides a class named DateTimeFormatter
for formatting and parsing date-time objects. This class allows you to convert a LocalTime
object to a string in a specific format.
Here’s an example:
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
LocalTime now = LocalTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern('HH:mm');
String formattedTime = now.format(formatter);
System.out.println(formattedTime);
# Output:
# Current time in the format: HH:mm
In this example, we’re creating a DateTimeFormatter
with a specific pattern (HH:mm
), and then using the format()
method to convert the LocalTime
object to a string in that format.
Performing Operations with Duration and Period
Java provides the Duration
and Period
classes for working with amounts of time. Duration
is used for time-based amounts (hours, minutes, seconds), while Period
is used for date-based amounts (years, months, days).
Here’s an example of using Duration
to calculate the difference between two times:
import java.time.LocalTime;
import java.time.Duration;
LocalTime startTime = LocalTime.of(10, 0); // 10:00
LocalTime endTime = LocalTime.of(15, 0); // 15:00
Duration duration = Duration.between(startTime, endTime);
System.out.println(duration);
# Output:
# PT5H
In this example, we’re using the Duration.between()
method to calculate the duration between startTime
and endTime
. The output is in the ISO-8601 duration format (PT5H
means a period of time of 5 hours).
Exploring Alternative Date-Time APIs in Java
While java.time
is a robust and comprehensive tool for handling time in Java, it’s not the only one. Java also provides other date-time APIs, such as Date
and Calendar
, that you might find useful depending on your specific needs.
The Date Class
The Date
class, part of Java’s core since its first version, represents a specific instant in time. Here’s a simple example of how to get the current date and time using Date
:
import java.util.Date;
Date date = new Date();
System.out.println(date);
# Output:
# Current date and time in the format: Mon Feb 24 00:00:00 GMT 2020
In this example, we’re creating a new Date
object, which gets initialized with the current date and time. However, Date
is not recommended for new code because of its many shortcomings, such as lack of support for time zones and locales.
The Calendar Class
The Calendar
class, introduced in Java 1.1, provides a more complete and flexible solution for date and time manipulation. Here’s an example of how to get the current time using Calendar
:
import java.util.Calendar;
Calendar calendar = Calendar.getInstance();
System.out.println(calendar.getTime());
# Output:
# Current date and time in the format: Mon Feb 24 00:00:00 GMT 2020
In this example, we’re using Calendar.getInstance()
to get a Calendar
object representing the current date and time. Despite being more flexible than Date
, Calendar
is also considered outdated and is not recommended for new code.
Making the Right Choice
When deciding which date-time API to use in Java, consider your specific needs. If you need to work with time zones, locales, or perform complex date/time calculations, java.time
is the best choice. However, if you’re working with legacy code or APIs that use Date
or Calendar
, you might need to use these classes.
While working with time in Java, you might encounter some common issues. Understanding these problems and knowing how to resolve them can save you a lot of time and frustration.
Time Zone Issues
Java’s java.time
package provides classes like ZonedDateTime
and OffsetDateTime
for dealing with date and time in specific time zones. However, if you’re not careful, you might still run into time zone issues.
For example, the LocalTime.now()
method returns the current time based on the system’s default time zone. If your system’s time zone is not the one you intended to use, this can lead to incorrect results.
To specify a time zone, you can use the ZonedDateTime.now(ZoneId.of('ZoneId'))
method:
import java.time.ZonedDateTime;
import java.time.ZoneId;
ZonedDateTime now = ZonedDateTime.now(ZoneId.of('America/Los_Angeles'));
System.out.println(now);
# Output:
# Current date and time in the Los Angeles time zone
In this example, we’re specifying ‘America/Los_Angeles’ as the time zone.
Parsing and Formatting Errors
When using DateTimeFormatter
to parse or format date-time objects, you might encounter errors if the string doesn’t match the pattern you’ve specified.
For example, the following code would throw a DateTimeParseException
:
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern('HH:mm');
LocalTime time = LocalTime.parse('10:00:00', formatter);
# Output:
# Exception in thread 'main' java.time.format.DateTimeParseException
In this example, the DateTimeFormatter
expects a string in the ‘HH:mm’ format, but we’re trying to parse a string in the ‘HH:mm:ss’ format. To avoid this error, make sure the string matches the pattern you’ve specified in the DateTimeFormatter
.
Java Time Handling: The Fundamentals
To effectively work with time in Java, it’s vital to understand the underlying concepts and how Java handles date and time.
Local Time, UTC, and Time Zones
LocalTime
in Java represents the time of day, without a date or time zone. It’s a simple concept, ideal for representing wall-clock time.
However, when you’re dealing with real-world applications, you’ll often need to work with Universal Coordinated Time (UTC) and time zones. UTC is the basis for civil time, used by many systems in the world. Time zones are regions of the Earth that have the same standard time.
Java’s java.time
package provides classes like ZonedDateTime
and OffsetDateTime
to work with date and time in specific time zones. For example, to get the current time in a specific time zone, you can use ZonedDateTime.now(ZoneId.of('ZoneId'))
.
Immutable and Thread-Safe Design
The classes in the java.time
package, including LocalTime
, are designed to be immutable and thread-safe. This means that once a date-time object is created, it cannot be changed. Instead, operations on a date-time object return a new instance.
This design has several advantages. It eliminates the need for using synchronization or locks when sharing date-time objects between threads, leading to safer and more reliable code.
Here’s an example of how immutability works in java.time
:
import java.time.LocalTime;
LocalTime time = LocalTime.of(10, 0); // 10:00
LocalTime newTime = time.plusHours(2); // 12:00
System.out.println(time); // 10:00
System.out.println(newTime); // 12:00
# Output:
# 10:00
# 12:00
In this example, the plusHours()
method doesn’t change the original LocalTime
object. Instead, it returns a new LocalTime
object with the modified time.
Time Handling in Larger Java Applications
Java’s date and time APIs are not just for displaying the current time or formatting time strings. They play a crucial role in larger Java applications, where managing time correctly is often critical to the application’s functionality and performance.
Scheduling Tasks
Java provides classes like java.util.Timer
and java.util.concurrent.ScheduledExecutorService
for scheduling tasks to run at specific times or periodically. These classes use java.time
under the hood to manage time.
Here’s an example of scheduling a task to run after a delay using ScheduledExecutorService
:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
Runnable task = () -> System.out.println('Task executed');
executor.schedule(task, 10, TimeUnit.SECONDS);
# Output (after 10 seconds):
# 'Task executed'
In this example, we’re scheduling a Runnable
task to execute after a delay of 10 seconds.
Handling Timestamps in Databases
When working with databases in Java, you’ll often need to handle timestamps. Java’s java.time
package provides the Instant
class for representing a specific moment on the timeline, ideal for storing timestamps in databases.
Further Resources for Mastering Java Time
To deepen your understanding of Java’s date and time APIs and how to use them in real-world applications, consider exploring the following resources:
- Java Date Guide by IOFlood dives into the Date class in Java for advanced date manipulation.
Exploring SimpleDateFormat in Java – Learn how to create custom date formats using SimpleDateFormat patterns.
Understanding LocalDate Class in Java – Explore LocalDate’s functionality for handling dates in Java applications
Java SE 8 Date and Time – Oracle’s official tutorial on the date and time APIs introduced in Java SE.
Java Date and Time API Guide by Baeldung, covers everything from the basics to more advanced topics.
Working with Date and Time in Java – A tutorial by Vogella, with many examples and explanations.
Wrapping Up: Time in Java
In this comprehensive guide, we’ve navigated the complexities of Java’s date and time APIs, providing you with the tools to manage time in your Java applications effectively.
We embarked on our journey with the basics, learning how to get the current time using the LocalTime
class and its now()
method. We then delved deeper, exploring more complex uses of Java’s date and time APIs, such as formatting time with DateTimeFormatter
and performing operations on time with Duration
and Period
.
We tackled common issues you might encounter when working with time in Java, such as time zone issues and parsing/formatting errors, providing you with solutions and workarounds for each problem. We also explored alternative date-time APIs in Java, such as Date
and Calendar
, helping you understand their benefits, drawbacks, and when to use them.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
LocalTime | Precise, easy to use | No support for date or time-zone |
DateTimeFormatter | Flexible formatting and parsing | Errors if string doesn’t match pattern |
Duration and Period | Powerful operations on time | Can be complex |
Date and Calendar | Legacy support | Outdated, less robust |
Whether you’re just starting out with Java or you’re looking to level up your time handling skills, we hope this guide has given you a deeper understanding of how to work with time in Java.
Mastering time in Java is a valuable skill, enabling you to write more robust and reliable applications. Now you’re well equipped to handle any time-related challenges that come your way. Happy coding!