Bash Script Math Operations: Arithmetic Tutorial
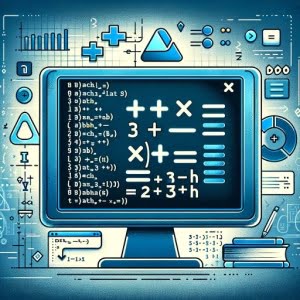
Are you finding it challenging to perform mathematical operations in Bash? You’re not alone. Many developers find themselves in a similar situation, but there’s a solution that can make this process a breeze.
Just like a calculator, Bash can perform a variety of mathematical operations. These operations can be used to automate tasks, perform calculations, and much more. They are a powerful tool in the arsenal of any Bash user.
In this guide, we’ll walk you through the basics to more advanced techniques of performing math in Bash. We’ll cover everything from simple arithmetic operations to complex calculations, as well as alternative approaches and common issues you might encounter.
So, let’s dive in and start mastering Bash Math!
TL;DR: How Do I Perform Math in Bash?
To perform math in bash, you can use the
$(( ))
syntax. This syntax allows you to perform a variety of mathematical operations, such as addition, subtraction, multiplication, and division.
Here’s a simple example:
a=$(( 5 + 3 ))
echo $a
# Output:
# 8
In this example, we’ve used the $(( ))
syntax to perform an addition operation. The numbers 5 and 3 are added together, and the result is stored in the variable a
. When we echo a
, it outputs the result of the operation, which is 8.
This is just a basic way to perform math in bash, but there’s much more to learn about bash math. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Bash Math: The $(( )) Syntax
- Advanced Bash Math: Floating Point Arithmetic with ‘bc’
- Alternative Bash Math Methods: ‘expr’ and ‘let’
- Troubleshooting Common Bash Math Issues
- Understanding Bash and Its Mathematical Capabilities
- The Relevance of Bash Math in Scripting and Automation
- Wrapping Up: Mastering Bash Math
Basic Bash Math: The $(( )) Syntax
The $(( )) syntax is the most straightforward method for performing basic arithmetic operations in Bash. It is similar to what you would find in most programming languages.
Here’s how it works:
b=$(( 10 - 2 ))
echo $b
# Output:
# 8
In this example, we’re using the $(( )) syntax to subtract 2 from 10. The result of the operation (8) is stored in the variable b
. When we echo b
, it outputs the result of the operation.
The $(( )) syntax can handle all four basic arithmetic operations: addition (+), subtraction (-), multiplication (*), and division (/).
# Addition
c=$(( 7 + 3 ))
echo $c
# Output:
# 10
# Multiplication
d=$(( 5 * 2 ))
echo $d
# Output:
# 10
# Division
e=$(( 20 / 4 ))
echo $e
# Output:
# 5
As you can see, the $(( )) syntax is quite versatile. However, it’s important to note that it only performs integer division. This means that it will round down the result to the nearest integer. For example:
f=$(( 10 / 3 ))
echo $f
# Output:
# 3
Even though the exact result of 10 divided by 3 is approximately 3.33, the $(( )) syntax rounds it down to 3. If you need more precise results, you’ll need to use more advanced techniques, which we’ll cover in the next section.
Advanced Bash Math: Floating Point Arithmetic with ‘bc’
For more complex mathematical operations, especially those involving floating point arithmetic, Bash offers the ‘bc’ command. The ‘bc’ command is a language that supports arbitrary precision numbers with interactive execution of statements.
Here’s an example of how to use ‘bc’ for floating point arithmetic:
result=$(echo "scale=2; 10 / 3" | bc)
echo $result
# Output:
# 3.33
In this example, we’re using the ‘bc’ command to perform a division operation with a precision of two decimal places. The scale=2
part sets the number of decimal places, and 10 / 3
is the operation we’re performing. The result is stored in the variable result
, and when we echo result
, it outputs the precise result of the operation.
This is a significant improvement over the $(( )) syntax, which only performs integer division and rounds down the result.
It’s important to note that ‘bc’ can handle more than just basic arithmetic operations. It also supports more advanced operations, like power and square root calculations. Here’s an example:
# Power calculation
power=$(echo "scale=2; 2 ^ 3" | bc)
echo $power
# Output:
# 8.00
# Square root calculation
root=$(echo "scale=2; sqrt(16)" | bc)
echo $root
# Output:
# 4.00
In these examples, we’re using ‘bc’ to perform a power calculation and a square root calculation. The results are precise and accurate, demonstrating the power and flexibility of the ‘bc’ command for advanced mathematical operations in Bash.
Alternative Bash Math Methods: ‘expr’ and ‘let’
While the $(( ))
syntax and the ‘bc’ command are excellent tools for performing mathematical operations in Bash, they are not the only options available. There are alternative methods, such as using ‘expr’ or ‘let’, which can offer different advantages depending on your specific needs.
The ‘expr’ Command
The ‘expr’ command is an older method for performing arithmetic in Bash. It’s a bit more verbose than the $(( ))
syntax, but it’s still quite capable.
Here’s an example of how to use ‘expr’:
result=`expr 10 + 20`
echo $result
# Output:
# 30
In this example, we’re using ‘expr’ to perform an addition operation. The result is stored in the variable result
, and when we echo result
, it outputs the result of the operation.
The ‘let’ Command
The ‘let’ command is another method for performing arithmetic operations in Bash. It’s similar to the $(( ))
syntax, but it doesn’t require the dollar sign or the double parentheses.
Here’s an example of how to use ‘let’:
let result=10*20
echo $result
# Output:
# 200
In this example, we’re using ‘let’ to perform a multiplication operation. The result is stored in the variable result
, and when we echo result
, it outputs the result of the operation.
Comparing the Methods
Method | Advantages | Disadvantages |
---|---|---|
$(( )) | Simple and straightforward | Only performs integer division |
‘bc’ | Supports floating point arithmetic and advanced operations | Requires piping and echo commands |
‘expr’ | Doesn’t require special syntax | More verbose than other methods |
‘let’ | Doesn’t require dollar sign or double parentheses | Only performs integer division |
Each method has its own advantages and disadvantages, so the best one to use depends on your specific needs. If you need to perform simple arithmetic, the $(( ))
syntax or the ‘let’ command will likely be sufficient. If you need more advanced capabilities, such as floating point arithmetic, the ‘bc’ command is probably your best bet. And if you prefer a more traditional syntax, you might find ‘expr’ to be the most comfortable method.
Troubleshooting Common Bash Math Issues
While Bash provides several methods for performing mathematical operations, it’s not uncommon to encounter issues. Let’s discuss some of these common problems and how to solve them.
Syntax Errors
One of the most common issues when performing bash math is syntax errors. This is usually due to missing spaces or incorrect use of special characters.
For example, consider the following code:
result=$((10+20))
echo $result
# Output:
# bash: syntax error near unexpected token `)'
This code will fail because there are no spaces around the ‘+’ operator. To fix this, we need to add spaces around the operator:
result=$((10 + 20))
echo $result
# Output:
# 30
Issues with Floating Point Arithmetic
Another common issue is related to floating point arithmetic. As we discussed earlier, the $(( ))
syntax and the ‘let’ command only perform integer division. This can lead to unexpected results.
For example, consider the following code:
result=$((10 / 3))
echo $result
# Output:
# 3
Even though the exact result of 10 divided by 3 is approximately 3.33, the $(( ))
syntax rounds it down to 3. To get a more precise result, we need to use the ‘bc’ command:
result=$(echo "scale=2; 10 / 3" | bc)
echo $result
# Output:
# 3.33
By being aware of these common issues and knowing how to solve them, you can avoid potential pitfalls and make the most of bash math.
Understanding Bash and Its Mathematical Capabilities
Before we delve deeper into Bash math, it’s essential to understand the background and fundamentals of Bash and shell scripting. This knowledge will help you grasp the concepts underlying bash math more effectively.
Bash: A Brief Overview
Bash, or the Bourne Again SHell, is a command processor that typically runs in a text window. It allows users to type commands that cause actions. Bash can also read and execute commands from a file, called a shell script.
Arithmetic Expansion in Bash
In Bash, arithmetic expansion provides a powerful tool for performing integer arithmetic, regardless of shell settings. The format for arithmetic expansion is $(( expression ))
. The expression is treated as if it were within double quotes, but a double quote inside the parentheses is not treated specially.
Here’s an example of arithmetic expansion in Bash:
result=$((10 * 2))
echo $result
# Output:
# 20
In this example, the expression 10 * 2
is evaluated, and the result is assigned to the variable result
. When we echo result
, it outputs the result of the operation.
Exploring ‘bc’, ‘expr’, and Other Tools
As we’ve discussed earlier, Bash offers several tools for performing mathematical operations, including ‘bc’ and ‘expr’. These tools can handle more complex calculations and offer more flexibility than the basic arithmetic expansion.
For instance, ‘bc’ is a language that supports arbitrary precision numbers with interactive execution of statements. It can handle floating point arithmetic, power calculations, square root calculations, and more.
On the other hand, ‘expr’ is an older method for performing arithmetic in Bash. It doesn’t require special syntax, but it’s more verbose than other methods.
By understanding these fundamentals, you’ll be better equipped to handle bash math and use the right tools for your specific needs.
The Relevance of Bash Math in Scripting and Automation
Understanding and mastering bash math can open up a world of possibilities for scripting and automation tasks. Bash math is not just about performing calculations; it’s a fundamental aspect of bash scripting that can help automate complex tasks and make your scripts more efficient.
For instance, bash math can be used to calculate the time it takes to execute a script, to determine the size of a file, or to automate the process of resizing images in a directory based on specific criteria.
Exploring Related Concepts: Conditional Statements and Loops in Bash
To further enhance your bash scripting skills, it’s worth exploring related concepts like conditional statements and loops in bash.
Conditional statements in bash allow you to make decisions in your scripts. For example, you can use an if statement to execute a specific part of your script based on the outcome of a bash math operation.
Similarly, loops in bash can be used to repeat a section of your script a certain number of times, which can be determined using bash math.
Here’s an example of a bash script that uses bash math, conditional statements, and loops:
for i in {1..10}
do
if (( $i % 2 == 0 ))
then
echo "$i is even"
else
echo "$i is odd"
fi
done
# Output:
# 1 is odd
# 2 is even
# 3 is odd
# 4 is even
# 5 is odd
# 6 is even
# 7 is odd
# 8 is even
# 9 is odd
# 10 is even
In this script, we’re using a for loop to iterate over the numbers 1 through 10. We then use an if statement and bash math to determine whether each number is even or odd.
By exploring these related concepts and integrating them with bash math, you can create powerful and efficient bash scripts.
Further Resources for Bash Math Mastery
To deepen your understanding of bash math and related concepts, here are a few external resources that you might find helpful:
- GNU Bash Manual: This is the official manual for Bash. It provides a comprehensive overview of the language, including a detailed section on arithmetic expressions.
BashGuide on Greg’s Wiki: This guide provides a practical introduction to bash scripting. It includes numerous examples and exercises that can help you get hands-on experience.
Bash Scripting Tutorial on Ryan’s Tutorials: This tutorial covers a wide range of topics, including variables, loops, and conditional statements. It also includes a section on arithmetic operations in bash.
Wrapping Up: Mastering Bash Math
In this comprehensive guide, we’ve delved into the world of Bash Math, a powerful tool in the arsenal of any Bash user. From simple arithmetic operations to advanced calculations, we’ve covered a wide range of mathematical operations you can perform in Bash.
We started with the basics, learning how to use the $(( ))
syntax for simple arithmetic operations. We then explored more advanced techniques, such as using the ‘bc’ command for floating point arithmetic and other complex calculations. Along the way, we tackled common issues you might encounter when performing Bash Math, such as syntax errors and issues with floating point arithmetic, providing you with solutions and workarounds for each issue.
We also looked at alternative methods for performing math in Bash, such as using ‘expr’ or ‘let’. Each method has its own advantages and disadvantages, so the best one to use depends on your specific needs. Here’s a quick comparison of these methods:
Method | Advantages | Disadvantages |
---|---|---|
$(( )) | Simple and straightforward | Only performs integer division |
‘bc’ | Supports floating point arithmetic and advanced operations | Requires piping and echo commands |
‘expr’ | Doesn’t require special syntax | More verbose than other methods |
‘let’ | Doesn’t require dollar sign or double parentheses | Only performs integer division |
Whether you’re just starting out with Bash Math or you’re looking to level up your Bash scripting skills, we hope this guide has given you a deeper understanding of Bash Math and its capabilities.
With its balance of simplicity, power, and flexibility, Bash Math is a key skill for any Bash user. Keep practicing, keep exploring, and happy scripting!