Hello World: Your First Java Programming Guide
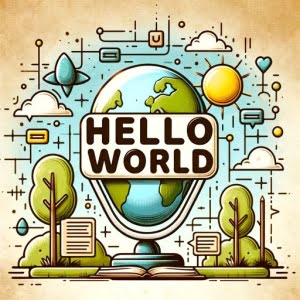
Are you finding it challenging to write your first Java program? You’re not alone. Many new developers find this first step intimidating, but there’s a simple program that can make this process a breeze.
Like a key to a vast kingdom, writing a ‘Hello, World!’ program in Java is your initiation into one of the most widely-used programming languages in the world. It’s a simple yet powerful program that forms the basis of many more complex applications.
This guide will walk you through the process of writing and running your first Java program, from writing your first line of code to understanding its structure and finally, executing it. We’ll cover everything from the basics of Java syntax to more advanced concepts, as well as troubleshooting common issues.
So, let’s dive in and start our journey into the world of Java programming!
TL;DR: How Do I Write and Run a ‘Hello, World!’ Program in Java?
To create a ‘Hello, World!’ program in Java, you first need to write the code in a text file named ‘HelloWorld.java’, ensure it containts the source code
public class HelloWorld {
, compile it using the command ‘javac HelloWorld.java’, and then run it using the command ‘java HelloWorld’.
public static void main (String args[]) {
System.out.println("Hello World");
}
}
Here’s a simple ‘Hello, World!’ program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# Output:
# 'Hello, World!'
To run this program, follow these steps:
- Save the code in a file named ‘HelloWorld.java’.
- Open your command prompt or terminal and navigate to the directory where you saved the file.
- Compile the program using the command
javac HelloWorld.java
. - Run the program using the command
java HelloWorld
.
In this example, we’ve created a simple Java program that prints ‘Hello, World!’ to the console. The public static void main(String[] args)
function is the entry point for any Java program, and System.out.println('Hello, World!');
is the line that prints the text to the console.
This is a basic way to write and run a ‘Hello, World!’ program in Java, but there’s much more to learn about Java programming. Continue reading for a more detailed guide and advanced usage scenarios.
Table of Contents
Writing and Running Your First Java Program
Let’s break down the process of writing and running your first ‘Hello, World!’ program in Java. We will cover the structure of a Java program, how to save, compile, and run it.
Understanding the Structure of a Java Program
First, let’s understand the basic structure of a Java program. A simple Java program has the following structure:
public class ClassName {
public static void main(String[] args) {
// Your code goes here
}
}
In this structure, public class ClassName
is the declaration of a new class. A class is a blueprint for creating objects (a particular data structure), providing initial values for state (member variables or attributes), and implementations of behavior (member functions or methods). The name of the class should match the filename.
public static void main(String[] args)
is the main method that’s executed when a Java program runs. Your program’s commands are placed inside the main method.
Writing Your First ‘Hello, World!’ Program
Now, let’s write our ‘Hello, World!’ program. Open a text editor, copy the following code, and save it as ‘HelloWorld.java’.
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
In this program, System.out.println('Hello, World!');
is the command that prints ‘Hello, World!’ to the console.
Compiling and Running Your First Java Program
Once you’ve written your program, it’s time to compile and run it. Here’s how:
- Open your command prompt or terminal.
- Navigate to the directory where you saved ‘HelloWorld.java’.
- Compile the program using the command
javac HelloWorld.java
. This command compiles your Java code into bytecode that the Java Virtual Machine (JVM) can understand. - Run the program using the command
java HelloWorld
. This command starts the JVM and runs the compiled bytecode.
Here’s the expected output:
# Output:
# 'Hello, World!'
Congratulations, you’ve just written and run your first Java program!
Diving Deeper into Java Programming
Now that you’ve written and run your first Java program, it’s time to explore more complex aspects of Java programming. This includes understanding the role of Integrated Development Environments (IDEs), Java’s object-oriented programming (OOP) structure, and the Java Development Kit (JDK).
Integrated Development Environments (IDEs)
While we’ve used a basic text editor to write our ‘Hello, World!’ program, most Java developers use Integrated Development Environments (IDEs) for larger projects. IDEs offer features like code suggestions, debugging tools, and built-in terminal windows, which can significantly speed up your coding process.
Two popular Java IDEs are Eclipse and IntelliJ IDEA. To run our ‘Hello, World!’ program in these IDEs, you would create a new project, write the program in the project’s main class, and then run the program using the IDE’s run button.
Understanding Java’s Object-Oriented Programming (OOP) Structure
Java is an object-oriented programming language, which means it represents concepts as “objects” that have data fields (attributes) and associated procedures known as methods. Objects are instances of classes, which can be thought of as blueprints for creating objects.
Our ‘Hello, World!’ program is a simple example of a class. When we run the program, we create an instance of the HelloWorld class and call its main method.
The Role of the Java Development Kit (JDK)
The Java Development Kit (JDK) is a software development environment used for developing Java applications. It includes the Java Runtime Environment (JRE), an interpreter/loader (java), a compiler (javac), an archiver (jar), a documentation generator (javadoc), and other tools needed in Java development.
In our ‘Hello, World!’ program, we used the JDK to compile and run our program. Specifically, we used the javac command to compile our program into bytecode, and the java command to run the compiled bytecode.
Understanding these advanced aspects of Java programming can help you write more complex programs and better understand how Java works under the hood.
Exploring Alternative Approaches to Java Hello World
While the previous sections have covered the basics and some advanced aspects of writing a ‘Hello, World!’ program in Java, there are other ways to write this program, especially for more experienced programmers. In this section, we’ll explore alternative approaches, such as using different development environments or tools, and writing a program that interacts with the user.
Leveraging Different Development Environments
Java is versatile and can be written and compiled in various development environments. For instance, online compilers like JDoodle and IDEs like JDeveloper, NetBeans, or BlueJ can be used to write and run Java programs. Here’s how you can run your ‘Hello, World!’ program using JDoodle:
- Go to JDoodle.
- Copy and paste your code into the editor.
- Click ‘Execute’ to run your program.
Interactive Java Hello World
While ‘Hello, World!’ is a static message, you can make your program interactive by asking the user for input. The following program prompts the user to enter their name and then greets them personally.
import java.util.Scanner;
public class HelloWorld {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println('Enter your name: ');
String name = scanner.nextLine();
System.out.println('Hello, ' + name + '!');
}
}
# Output:
# Enter your name:
# [user enters name]
# Hello, [name]!
In this program, we’ve used the Scanner class to read the user’s input. The System.out.println('Enter your name: ');
line prompts the user to enter their name, and the String name = scanner.nextLine();
line reads the user’s input and stores it in a variable. Finally, the System.out.println('Hello, ' + name + '!');
line prints a personalized greeting.
These alternative approaches can provide a more dynamic and interactive programming experience.
Troubleshooting Common Java Errors
Even with a simple ‘Hello, World!’ program, you might encounter some common errors. Let’s discuss these potential pitfalls and how to resolve them. Additionally, we’ll share some best practices and optimization tips for your Java programming journey.
Common Errors and Their Solutions
- ClassDefNotFoundError or NoClassDefFoundError: This error occurs when the Java Virtual Machine (JVM) or a ClassLoader instance tries to load the definition of a class and the definition could not be found. The common reason is that the class is present at compile time but not at runtime. Make sure that your class file is in the correct directory and you’re in the correct directory in your terminal or command prompt when you run the java command.
ClassNotFoundException: This exception indicates that the class was not found on the classpath. This typically means that you’ve attempted to reference a class that doesn’t exist or the class does exist but is in the wrong directory. Check your typing and your file’s location.
Syntax errors: These are mistakes in your code’s syntax, like a missing semicolon or mismatched parentheses. Most IDEs will highlight syntax errors before you compile your code. If you’re using a text editor, the javac command will display an error message if it encounters a syntax error.
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!') // missing semicolon
}
}
# Output:
# error: ';' expected
# System.out.println('Hello, World!')
# ^
In the example above, we’ve omitted the semicolon at the end of the print statement, which causes a compile-time error.
Best Practices and Optimization Tips
- Use meaningful class and variable names: While our ‘Hello, World!’ program only has one class and no variables, more complex programs will have multiple classes and variables. Using meaningful names can make your code easier to read and understand.
Follow Java’s coding conventions: Java has established coding conventions that cover filenames, class names, variable names, and other coding elements. Following these conventions can make your code more readable and maintainable.
Comment your code: While our ‘Hello, World!’ program is simple enough that it doesn’t need comments, more complex programs can benefit from comments that explain what different parts of the code do.
Remember, the key to successful programming is practice and patience. Don’t be discouraged by errors; they’re opportunities to learn and improve your coding skills.
Understanding Java and Its Syntax
Before we delve deeper into Java programming, let’s take a step back and understand the language’s background and fundamentals, including its syntax, the role of classes, methods, and statements, and its ‘write once, run anywhere’ philosophy.
A Brief Overview of Java
Java, developed by Sun Microsystems in 1995, is an object-oriented programming language known for its simplicity, robustness, and platform independence. It’s widely used in web development, mobile application development (especially Android apps), and building enterprise-scale applications.
The Role of Classes, Methods, and Statements
In Java, a class is a blueprint for creating objects. A class encapsulates data for the object. Data is represented by attributes, and actions are represented by methods. For example, in our ‘Hello, World!’ program, HelloWorld
is a class.
A method is a collection of statements that perform an operation. In our ‘Hello, World!’ program, public static void main(String[] args)
is a method. It’s the entry point for any standalone Java application.
A statement is an instruction in Java. It could be an assignment statement (like int x = 10;
), a method call (like System.out.println();
), a flow control statement (like if
condition), and so on.
Here’s a simple example to illustrate classes, methods, and statements:
public class HelloWorld {
public static void main(String[] args) {
String greeting = 'Hello, World!';
System.out.println(greeting);
}
}
# Output:
# 'Hello, World!'
In the example above, HelloWorld
is a class, main
is a method, and String greeting = 'Hello, World!';
and System.out.println(greeting);
are statements.
Java’s ‘Write Once, Run Anywhere’ Philosophy
Java was designed with the philosophy of ‘write once, run anywhere’ (WORA). This means that Java code (which is platform-independent) can run on any device that has a Java Virtual Machine (JVM). The JVM interprets the compiled Java code (bytecode) and runs it on the host machine.
This philosophy is one of the reasons for Java’s popularity. It allows developers to write code that can run on any platform without recompilation. This is a significant advantage when developing software for multiple platforms.
Understanding these fundamental aspects of Java will provide a solid foundation for your journey into Java programming.
Applying ‘Hello, World!’ to Larger Java Projects
The ‘Hello, World!’ program, while simple, forms the foundation for more complex Java applications. This section discusses how the knowledge you’ve gained can be applied to larger projects, such as creating a graphical user interface (GUI) or building a web application.
Creating a Graphical User Interface (GUI)
In Java, you can use the Swing library to create a GUI. Let’s modify our ‘Hello, World!’ program to display the greeting in a GUI.
import javax.swing.JOptionPane;
public class HelloWorld {
public static void main(String[] args) {
JOptionPane.showMessageDialog(null, 'Hello, World!');
}
}
In the example above, we’ve imported the JOptionPane class from the Swing library. The JOptionPane.showMessageDialog(null, 'Hello, World!');
line creates a dialog box that displays ‘Hello, World!’.
Building a Web Application
Java is widely used in web development. Frameworks like Spring Boot make it easy to create enterprise-grade applications. For example, you could create a web application that displays ‘Hello, World!’ in a web browser.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class HelloWorld {
@RestController
public static class HelloController {
@RequestMapping('/')
public String hello() {
return 'Hello, World!';
}
}
public static void main(String[] args) {
SpringApplication.run(HelloWorld.class, args);
}
}
In the example above, we’ve created a simple Spring Boot application that displays ‘Hello, World!’ when you access the root URL (/
).
Further Resources for Mastering Java
If you’re interested in learning more about Java, here are some resources that might help:
- Java Tutorial: A Quick Overview – Learn about Java GUI programming using Swing and JavaFX libraries.
Running Java from Command Line – Explore options and commands available for running Java applications efficiently.
Exploring Java Project Ideas – Explore different types of Java projects, from beginner to advanced levels.
Oracle’s Java Tutorials cover everything from the basics to advanced topics.
Codecademy’s Learn Java Course includes quizzes and projects to help you practice your new skills.
Coursera’s Java Programming and Software Engineering Fundamentals covers the basics of programming and software development using Java.
Remember, the best way to learn programming is by doing. Don’t be afraid to experiment with different projects and challenges – they’re great ways to practice and solidify your understanding of Java.
Wrapping Up: Your First Java Program
In this comprehensive guide, we’ve navigated through the process of writing and running your first ‘Hello, World!’ program in Java.
We started with the basics, learning how to write a simple ‘Hello, World!’ program in Java, understanding its structure, and executing it. We then delved into more advanced aspects of Java programming, exploring the use of Integrated Development Environments (IDEs), Java’s object-oriented programming (OOP) structure, and the Java Development Kit (JDK).
We also discussed alternative approaches to writing a ‘Hello, World!’ program in Java, such as leveraging different development environments and making the program interactive. Along the way, we tackled common errors you might encounter when writing and running a Java program, and provided solutions to these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Command Line | Full control, good for learning | Manual process, slower for large projects |
IDEs | Faster development, debugging tools | Can be overwhelming for beginners |
Online Compilers | No setup required | Limited features, internet-dependent |
Whether you’re just starting out on your Java journey or you’re looking to strengthen your fundamentals, we hope this guide has given you a solid foundation and a deeper understanding of Java programming.
The ability to write and run a simple Java program is a stepping stone to more complex projects. With the knowledge you’ve gained here, you’re well on your way to becoming a proficient Java programmer. Happy coding!