How-To Run Java Programs From Command Line
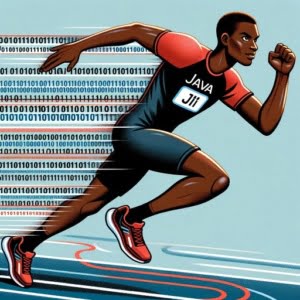
Are you finding it difficult to run your Java programs? You’re not alone. Many developers find themselves in a similar situation, but we’re here to help.
Think of running a Java program like a marathon runner starting a race. The runner needs the right environment and commands to start. Similarly, a Java program requires a specific setup and commands to run successfully.
In this guide, we’ll walk you through the process of running Java programs, from the basics to more advanced techniques. We’ll cover everything from compiling and executing Java programs in the command line, handling Java programs with arguments, to dealing with packages and using alternative approaches like Integrated Development Environments (IDEs) or build tools like Maven or Gradle.
Let’s lace up and start running with Java!
TL;DR: How Do I Run a Java Program?
You can run a Java program from the command line using the
java
command followed by the name of the class that contains the main method.
Here’s a simple example:
java simpleAddition 1 2
# Output:
# '1 + 2 = 3'
In this example, we’ve used the java
command followed by the name of the class simpleAddition
. This class contains the main method, which is the entry point for any Java program. When you run this command, it will execute the simpleAddition
program and print the sum of all passed integers to the console.
This is just a basic way to run a Java program, but there’s much more to learn about running Java programs, handling arguments, dealing with packages, and using alternative approaches. Continue reading for more detailed instructions and advanced usage scenarios.
Table of Contents
Running Java Programs: The Basics
Running a Java program from the command line involves two main steps: compiling your Java code into bytecode and then running that bytecode using the Java Virtual Machine (JVM).
Compiling Java Code with javac
Firstly, you need to compile your Java code. Java source code is written in a .java file and then compiled into bytecode by the Java compiler. The javac
command is used for this purpose.
Here’s an example of how to compile a Java program:
javac HelloWorld.java
In this example, HelloWorld.java
is the name of the Java file that you want to compile. The javac
command compiles this file and creates a new file called HelloWorld.class
that contains the Java bytecode.
Running Java Bytecode with java
After you’ve compiled your Java code, you can run it with the java
command. The java
command starts a JVM and runs the bytecode that was created by the javac
command.
Here’s an example of how to run a Java program:
java HelloWorld
# Output:
# 'Hello, World!'
In this example, HelloWorld
is the name of the class that you want to run. The java
command runs this class and prints ‘Hello, World!’ to the console.
Benefits and Potential Pitfalls
The main advantage of running Java programs from the command line is that it gives you complete control over the Java environment. You can specify the classpath, pass arguments to the JVM, and do other advanced tasks.
However, it can be easy to make mistakes when running Java programs from the command line. For example, you might forget to compile your code before running it, or you might run the wrong class. It’s also easy to get confused by error messages if you’re not familiar with them. That’s why it’s important to understand what each command does and how to use it correctly.
Running Java Programs: The Intermediate Level
As you gain more experience with Java, you’ll find that running a program isn’t always as straightforward as compiling a single file and running a single class. You might need to pass arguments to your program, use a classpath, or deal with packages. Let’s explore these scenarios in more detail.
Passing Arguments to Java Programs
Java programs can accept command-line arguments. These arguments are passed to the main method as an array of String objects. Here’s an example of how to run a Java program with arguments:
java Greeting Alice Bob
# Output:
# 'Hello, Alice!'
# 'Hello, Bob!'
In this example, Greeting
is the name of the class that you want to run, and Alice
and Bob
are the arguments that you’re passing to the program. The program prints a greeting for each argument.
Using the Classpath
The classpath is a parameter that tells the JVM where to find user-defined classes and packages. It’s specified with the -cp
or -classpath
option when running the java
command. Here’s an example:
java -cp /path/to/classes HelloWorld
# Output:
# 'Hello, World!'
In this example, /path/to/classes
is the directory that contains the HelloWorld.class
file. The -cp
option tells the JVM to look for classes in this directory.
Dealing with Packages
Packages are used in Java to prevent naming conflicts, control access, and organize code. If a class is part of a package, you need to include the package name when running the class. Here’s an example:
java com.example.HelloWorld
# Output:
# 'Hello, World!'
In this example, com.example.HelloWorld
is the fully qualified name of the class. The com.example
part is the package name, and HelloWorld
is the class name.
Running Java programs with arguments, using the classpath, and dealing with packages can be more complicated than running a simple Java program, but these techniques give you more flexibility and control over your Java environment.
Exploring Alternative Methods: IDEs and Build Tools
The command line isn’t the only way to run Java programs. There are other methods that offer additional features and conveniences, such as Integrated Development Environments (IDEs) and build tools like Maven and Gradle. Let’s dive into these alternatives.
Running Java Programs in an IDE
An Integrated Development Environment (IDE) is a software application that provides comprehensive facilities to programmers for software development. An IDE normally consists of a source code editor, build automation tools, and a debugger. Most modern IDEs have intelligent code completion.
Here’s an example of how to run a Java program in an IDE like IntelliJ IDEA:
// In the Project tool window, right-click the class you want to run and select 'Run'
# Output:
# 'Hello, World!'
In this example, you simply right-click the class you want to run and select ‘Run’. The IDE compiles and runs the program for you and displays the output in a console window.
Running Java Programs with Maven or Gradle
Maven and Gradle are popular build automation tools that can be used to compile, test, and run Java programs. They handle dependencies, build a project from source, and more. Here’s an example of how to run a Java program with Maven:
mvn exec:java -Dexec.mainClass="com.example.HelloWorld"
# Output:
# 'Hello, World!'
In this example, mvn exec:java
is the Maven command to execute a Java program, and -Dexec.mainClass="com.example.HelloWorld"
specifies the main class to run. Maven compiles and runs the program and displays the output.
Running Java programs in an IDE or with a build tool like Maven or Gradle can be more convenient than running them from the command line, especially for large projects. However, these methods also have their downsides. They can be more complicated to set up, and they might not give you as much control over the Java environment.
While running Java programs, you might encounter some common issues. These can range from ‘Class not found’ to ‘Could not find or load main class’ errors. Let’s discuss these problems and provide some solutions and workarounds.
Handling ‘Class not found’ Errors
A ‘Class not found’ error typically occurs when the JVM can’t locate the class you’re trying to run. This might be due to a wrong class name or a missing classpath.
java HelloWorld
# Output:
# Error: Could not find or load main class HelloWorld
In this case, ensure that you’ve compiled your Java file into a .class file and that you’re using the correct class name. Also, check that the class is in the classpath that you’ve specified when running the java
command.
Dealing with ‘Could not find or load main class’ Errors
The ‘Could not find or load main class’ error often happens when the main class is not correctly defined in your program. The main class is the entry point for your program and should contain a public static void main(String[] args)
method.
java MainClass
# Output:
# Error: Could not find or load main class MainClass
In this scenario, check that your main class has the correct method signature. Also, ensure that you’re using the fully qualified name of the class (including the package name, if any) when running the java
command.
While these errors can be frustrating, they’re also an opportunity to better understand how Java works. By learning how to troubleshoot these issues, you’ll become a more proficient Java developer.
Understanding Java Fundamentals
Before diving into running Java programs, it’s crucial to understand the fundamental components that make Java work. These include the Java Runtime Environment (JRE), the Java Development Kit (JDK), and the process of compiling and running Java programs.
Java Runtime Environment (JRE)
The Java Runtime Environment (JRE) is what your computer uses to run Java programs. It includes the Java Virtual Machine (JVM), which loads and executes Java bytecode, and the Java Class Library, which provides a standard set of classes for performing common tasks.
Java Development Kit (JDK)
The Java Development Kit (JDK) is a superset of the JRE. It includes everything in the JRE, plus tools for developing, debugging, and monitoring Java applications. This includes the javac
compiler, which you use to compile your Java programs.
The Process of Compiling and Running Java Programs
Java programs go through two main stages: compilation and execution. During the compilation stage, the javac
compiler takes your Java source code and translates it into Java bytecode. This bytecode is a platform-independent code that can be run on any machine that has a JVM.
javac HelloWorld.java
In this example, the javac
command compiles the HelloWorld.java
source file into a HelloWorld.class
file containing Java bytecode.
During the execution stage, the JVM takes the bytecode and translates it into machine code that your computer can understand and execute.
java HelloWorld
# Output:
# 'Hello, World!'
In this example, the java
command runs the HelloWorld
class. The JVM loads the HelloWorld.class
file, translates the bytecode into machine code, and executes it.
Understanding these key components and the process of compiling and running Java programs is fundamental to becoming proficient in Java and mastering the Java Runner.
The Relevance of Java Runner in Software Development
Running Java programs is not just a one-off task you perform while learning Java. It’s a critical aspect of software development, testing, and deployment. Understanding how to run Java programs effectively can significantly impact your efficiency and productivity as a developer.
Debugging and Java Runner
Debugging is an essential part of software development. When your Java program doesn’t work as expected, you’ll need to run it in a specific way to identify and fix the problem. Understanding how to run Java programs is crucial for effective debugging.
Unit Testing and Java Runner
Unit testing involves testing individual components of your software to ensure they work correctly. In Java, these tests are often written as separate programs that need to be run. Understanding how to run these tests is key to ensuring the reliability of your software.
Continuous Integration and Java Runner
Continuous Integration (CI) is a development practice where developers integrate code into a shared repository frequently. Each integration is then verified by an automated build and automated tests. Running Java programs is a critical part of this process, and understanding how to do this effectively is crucial for successful CI implementation.
Further Resources for Java Programming
To deepen your understanding of running Java programs and related concepts, consider exploring the following resources:
- IOFlood’s Java Tutorial Article explains how to handle exceptions and errors in Java applications.
Your First Java Program – Learn how to write and run the traditional introductory program in Java.
Java Code Illustrations – Explore Java programming practices through real-world code demonstrations.
Oracle’s Java Tutorials cover a wide range of Java topics, including running programs.
Baeldung’s Guide to Java provides information on various Java topics, including running programs.
GeeksforGeeks’ Java Programming Language Resource offers a variety of tutorials on running programs, debugging, and testing in Java.
Wrapping Up: Running Java Programs
In this comprehensive guide, we’ve journeyed through the intricacies of running Java programs using different methods and techniques. From the basics to more advanced strategies, we’ve covered a wide range of topics to help you master the Java Runner.
We began with the basics, learning how to compile and run a Java program from the command line using the javac
and java
commands. We then delved into more advanced usage, exploring how to run Java programs with arguments, using the classpath, and dealing with packages. We also provided solutions and workarounds for common issues you might encounter when running Java programs, such as ‘Class not found’ and ‘Could not find or load main class’ errors.
Beyond the command line, we explored alternative approaches to running Java programs, such as using an Integrated Development Environment (IDE) or a build tool like Maven or Gradle. Each method has its own advantages and potential pitfalls, offering different levels of control and convenience.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Command Line | Complete control, flexible | Easy to make mistakes, confusing error messages |
IDE | Convenient, integrated tools | More complex setup, less control |
Maven/Gradle | Handles dependencies, automates build | More complex setup, less control |
Whether you’re just starting out with Java or looking to deepen your understanding of running Java programs, we hope this guide has provided you with valuable insights and practical knowledge.
With a solid understanding of Java Runner and its various aspects, you’re now better equipped to tackle any challenges you might face when running Java programs. Happy coding!