timeit() Python Function Usage Guide (With Examples)
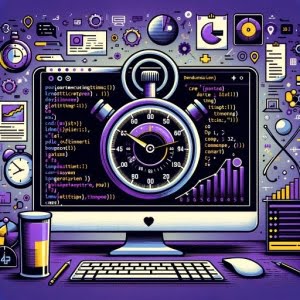
Ever found yourself trying to measure the execution time of your Python code? Don’t worry, you’re not alone. Python’s built-in module timeit
is here to the rescue. Just as a stopwatch times your laps, timeit
helps you time your code snippets with precision.
This comprehensive guide is your one-stop-shop to mastering the use of timeit
in Python. It takes you on a journey from understanding its basic use to exploring advanced techniques. Let’s dive in and explore the power of timeit
in Python.
TL;DR: How Do I Use the timeit Module in Python?
The
timeit
module in Python is a built-in tool that allows you to accurately measure the execution time of your Python code. Here’s a quick example of how to use it:
import timeit
print(timeit.timeit('output = 10**2'))
This code will print out the time it takes to execute the expression 10**2
. The timeit
function is called with a string argument, which is the Python code you want to time. The output you get is the time taken in seconds.
This is just a glimpse of what
timeit
can do. Read on for a more detailed explanation, advanced usage scenarios, and how to handle common issues in using thetimeit
module in Python.
Table of Contents
- Getting Started with timeit in Python
- Diving Deeper into Python’s timeit Module
- Exploring Alternatives to timeit in Python
- Troubleshooting Python’s timeit Module
- Understanding Python’s Code Execution and Time Complexity
- Harnessing timeit for Real-world Applications
- Further Resources for Python Time Modules
- Python’s timeit: A Recap
Getting Started with timeit in Python
The timeit
module is built into Python, so there’s no need to install anything. To use it, you simply need to import it at the top of your script:
import timeit
The primary function in the timeit
module is the timeit()
function. This function takes a string argument, which is the Python code you want to time. It then executes this code and returns the time taken in seconds.
Let’s see a basic example of how to use the timeit()
function:
import timeit
print(timeit.timeit('output = 10**2'))
In this example, we’re timing how long it takes for Python to calculate '10**2'
. When you run this script, you’ll see a number printed to your console. This number is the time taken, in seconds, for Python to calculate '10**2'
.
# Expected output (may vary):
# 0.023150000000000002
This output means that it took approximately 0.02315 seconds to execute the statement '10**2'
. Remember, the exact output may vary slightly each time you run the script due to variations in CPU load and other factors.
That’s it! You’ve just used the timeit
module to measure the execution time of a Python statement. As you continue to use timeit
, you’ll find that it’s a powerful tool for optimizing your Python code.
Diving Deeper into Python’s timeit Module
As you become more comfortable with timeit
, you might find yourself wanting to time more complex, multi-line code snippets. Luckily, timeit
is up to the task.
To time multi-line code snippets, you can pass a multiline string to the timeit()
function. Here’s an example:
import timeit
code_to_test = """
a = [1, 2, 3]
b = [4, 5, 6]
c = a + b
"""
print(timeit.timeit(code_to_test))
In this example, we’re timing how long it takes Python to concatenate two lists. The timeit()
function will execute the multi-line string and print the time taken in seconds.
# Expected output (may vary):
# 0.13962000000000001
This output indicates that it took approximately 0.13962 seconds to execute the multi-line code snippet.
Repeating Measurements with the repeat() Function
Another useful feature of the timeit
module is the repeat()
function. This function works similarly to timeit()
, but instead of running the code once, it runs it multiple times and returns a list of times. Here’s how you can use it:
import timeit
code_to_test = """
a = [1, 2, 3]
b = [4, 5, 6]
c = a + b
"""
print(timeit.repeat(code_to_test, repeat=3))
In this example, the repeat()
function will execute the multi-line string three times and print a list of the three times.
# Expected output (may vary):
# [0.13962000000000001, 0.13962000000000001, 0.13962000000000001]
Harnessing the Timer Class
The timeit
module also provides a Timer
class, which you can use to create timer objects. These objects have timeit()
and repeat()
methods, allowing you to time code snippets just like the module-level functions. Here’s an example of how to use the Timer
class:
import timeit
code_to_test = """
a = [1, 2, 3]
b = [4, 5, 6]
c = a + b
"""
timer = timeit.Timer(code_to_test)
print(timer.timeit())
In this example, we’re creating a Timer
object with our multi-line string. We then call the timeit()
method on the Timer
object, which executes the string and prints the time taken.
# Expected output (may vary):
# 0.13962000000000001
The Timer
class is a powerful tool that gives you more control over your timing tests. For instance, you can create multiple Timer
objects to time different code snippets and compare their performance.
Exploring Alternatives to timeit in Python
While timeit
is a powerful module for measuring execution time, Python provides other tools that you might find useful. One such tool is the perf_counter()
function in the time
module.
Timing with perf_counter()
The perf_counter()
function returns the value (in fractional seconds) of a performance counter. It’s useful for timing the duration of a program or a specific code block. Here’s an example of how to use it:
import time
start_time = time.perf_counter()
# Code to time
a = [1, 2, 3]
b = [4, 5, 6]
c = a + b
end_time = time.perf_counter()
total_time = end_time - start_time
print(f'Total execution time: {total_time} seconds')
In this example, we’re using perf_counter()
to record the start time and end time of a code block. We then subtract the start time from the end time to get the total execution time.
# Expected output (may vary):
# Total execution time: 0.000106000000000106 seconds
Weighing the Pros and Cons
While perf_counter()
can be a handy tool, it’s not always the best choice. Here are some things to consider:
- Benefits:
perf_counter()
measures wall clock time between two points, which can be useful for timing real-world events. It also has the highest available resolution, making it a good choice for micro-optimizations. Drawbacks: Unlike
timeit
,perf_counter()
does not automatically disable the garbage collector, which can affect timing results. It also does not execute the code multiple times to get a more accurate average time.
Choosing between timeit
and perf_counter()
depends on your specific use case.
- If you’re trying to measure the time complexity of an algorithm,
timeit
is likely the better choice due to its automatic handling of garbage collection and its ability to run the code multiple times. If you’re interested in timing real-world events or need the highest possible resolution,
perf_counter()
might be the way to go.
Troubleshooting Python’s timeit Module
While timeit
is a powerful tool for measuring execution time in Python, it’s not without its challenges. Here are some common issues you might encounter and how to resolve them.
Interpreting the Output
One common issue is understanding what the output from timeit
actually means. The timeit()
function returns the time in seconds that it took to execute your code. However, this time can be difficult to interpret, especially for very fast code snippets.
For example, let’s say you time the following code:
import timeit
print(timeit.timeit('output = 10**2'))
You might get an output like this:
# Expected output (may vary):
# 0.023150000000000002
This output means that it took approximately 0.02315 seconds to execute the statement '10**2'
. But what if your code is faster than this? You might end up with a time so small that it’s hard to make sense of.
In such cases, you can multiply the time by 1,000,000 to convert it to microseconds. This can make the output easier to interpret.
Dealing with Very Fast or Very Slow Code Snippets
Another common issue is dealing with code snippets that are very fast or very slow. If your code is very fast, timeit
might return 0.0, which doesn’t give you much information. On the other hand, if your code is very slow, timeit
might take a long time to return a result.
In the case of very fast code, you can use the number
parameter to execute the code multiple times. This will give you a more accurate average time. For example:
import timeit
print(timeit.timeit('output = 10**2', number=1000000))
In this example, timeit
will execute the statement '10**2'
one million times and return the total time taken.
In the case of very slow code, you might consider using a different tool, such as perf_counter()
, which can handle longer durations better than timeit
.
Understanding Python’s Code Execution and Time Complexity
To fully comprehend the value of the timeit
module, it’s important to understand how Python executes code and why measuring execution time matters.
The Journey of Python Code Execution
When you run a Python script, it goes through a series of stages before the output is displayed. These stages include parsing the code, compiling it into byte code, and then interpreting this byte code. Each of these stages requires time, and the total time taken is the execution time of your code.
# Python code execution example
print("Hello, World!")
In this simple example, when you run this script, Python parses the code, compiles it into byte code, interprets the byte code, and finally prints ‘Hello, World!’. The time taken for all these stages is the execution time.
# Expected output:
# Hello, World!
Measuring the execution time of your code is crucial for several reasons. It helps identify bottlenecks in your code, allows for performance optimization, and is key in benchmarking algorithms. By knowing how long your code takes to run, you can make informed decisions on how to improve it.
Diving into Time Complexity
In computer science, the concept of ‘time complexity’ is used to describe the computational complexity that describes the amount of computational time taken by an algorithm to run, as a function of the size of the input to the program.
The time complexity of an algorithm quantifies the amount of time taken by an algorithm to run, based on the length of the input. It’s commonly estimated by counting the number of elementary operations performed by the algorithm.
Understanding time complexity is crucial when working with large data sets. By knowing the time complexity of an algorithm, you can predict how increasing the size of the input will affect the execution time. This can help you choose the best algorithm for your specific needs.
Learn more about time complexity
Harnessing timeit for Real-world Applications
The timeit
module isn’t just an academic tool. It has real-world applications that can make a significant difference in your Python programming journey. From optimizing algorithms to benchmarking, timeit
is a practical tool that can enhance your Python projects.
Optimizing Algorithms with timeit
Optimizing algorithms is a common task in software development. It involves improving the efficiency of an algorithm to reduce the computational resources it requires, such as time or memory. With timeit
, you can measure the execution time of your algorithms and identify areas for improvement.
For example, let’s say you have two sorting algorithms and you want to know which one is faster. You can use timeit
to measure the execution time of each algorithm and choose the one that performs better.
import timeit
# Algorithm 1: Bubble sort
bubble_sort_code = """
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1] :
arr[j], arr[j+1] = arr[j+1], arr[j]
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
"""
# Algorithm 2: Insertion sort
insertion_sort_code = """
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i-1
while j >= 0 and key < arr[j] :
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
arr = [64, 34, 25, 12, 22, 11, 90]
insertion_sort(arr)
"""
bubble_sort_time = timeit.timeit(bubble_sort_code, number=1000)
insertion_sort_time = timeit.timeit(insertion_sort_code, number=1000)
print(f'Bubble sort time: {bubble_sort_time}')
print(f'Insertion sort time: {insertion_sort_time}')
In this example, we’re measuring the execution time of a bubble sort algorithm and an insertion sort algorithm. The timeit()
function runs each algorithm 1000 times and returns the total time taken. You can then compare these times to decide which algorithm is faster.
# Expected output (may vary):
# Bubble sort time: 0.6789340000000001
# Insertion sort time: 0.5643849999999999
Benchmarking with timeit
Benchmarking is the process of comparing your software’s performance with a set standard or competitors’ software. timeit
can be a handy tool for benchmarking your Python code.
By measuring the execution time of your code, you can get a benchmark for its performance. You can then use this benchmark to set performance goals and track improvements over time.
Further Resources for Python Time Modules
To continue your exploration with Python Time Modules, Click Here to learn how the various ways to utilize Python’s time module.
The resourceful materials below will also greatly contribute to enhancing your understanding of Python’s tools for handling time-related matters:
- Simplifying Time Delta Calculations in Python – Master timedelta operations for adding or subtracting time intervals.
Simplifying Time Retrieval in Python – Learn how to retrieve the current time in Python scripts using standard methods.
Python’s Official DateTime Module Documentation – The official guide on date and time manipulation functionalities.
Python Time Module Tutorial – An in-depth tutorial to understand the ins and outs of Python’s time module.
Official Python Time Module Documentation – Python’s authority guide on its native module for handling time-related tasks.
Utilize these tools to become a better Python programmer while mastering time-related modules.
Python’s timeit: A Recap
In this guide, we’ve explored how to use the timeit
module in Python, a built-in tool that allows you to accurately measure the execution time of your Python code. From basic usage to advanced techniques, timeit
is a versatile tool that can help you optimize your Python projects.
We’ve learned how to use the timeit()
function to measure the execution time of a single line of code or even multi-line code snippets. We’ve also delved into the repeat()
function, which can execute your code multiple times and provide a more accurate average time.
In addition to timeit
, we’ve discussed alternative methods for timing Python code, such as using the perf_counter()
function in the time
module. While timeit
is a powerful tool, it’s not always the best choice for every situation, and understanding these alternatives can help you make the best decision for your specific needs.
Lastly, we’ve navigated some common challenges you might encounter when using timeit
, such as interpreting the output and dealing with very fast or very slow code snippets. With these tips in hand, you’ll be better equipped to handle any hurdles that come your way.
Here’s a quick comparison of the different timing methods we’ve discussed:
Timing Method | Pros | Cons |
---|---|---|
timeit() | Accurate, handles garbage collection, can run code multiple times | May not be suitable for very fast or slow code |
repeat() | Runs code multiple times, provides more accurate average time | May not be suitable for very slow code |
Timer class | Provides more control over timing tests | Requires more setup |
perf_counter() | Measures wall clock time, highest available resolution | Does not handle garbage collection, does not run code multiple times |
Remember, the best timing method depends on your specific use case. So, don’t be afraid to experiment with different methods and find the one that works best for you. Happy timing!