Python Keywords: A Comprehensive Usage Guide
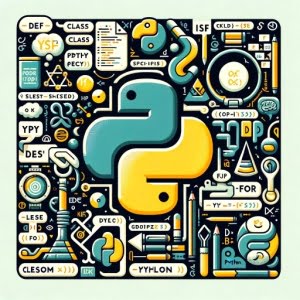
Ever felt overwhelmed by Python keywords? You’re not alone. Many programmers find Python keywords a bit intimidating. But think of Python keywords as the keys on a piano – each one plays a crucial role in composing a Python program.
Python keywords are the building blocks of your code, the fundamental elements that define the syntax and structure of the Python language. They’re like the grammar rules in a language, guiding how sentences (or in this case, code) should be formed.
In this guide, we’ll help you master Python keywords. We’ll explain what Python keywords are, how they are used, and what each keyword does. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Python keywords!
TL;DR: What are Python Keywords?
Python keywords are reserved words that are used to define the syntax and structure of the Python language. They are the fundamental elements that form the ‘grammar’ of Python code. Here’s a simple way to view all Python keywords:
import keyword
print(keyword.kwlist)
# Output:
# ['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
In this example, we import the keyword
module and use the kwlist
function to print a list of all Python keywords. These keywords, such as ‘if’, ‘else’, ‘for’, ‘while’, and so on, are the building blocks of Python programming.
But there’s much more to Python keywords than just knowing what they are. Continue reading for a detailed explanation and examples of each keyword, from basic to advanced usage scenarios.
Table of Contents
Python Keywords: The Basics
Python keywords are reserved words that cannot be used as identifiers such as variable names, function names, or any other identifiers. They are the very foundation of Python programming, defining the language’s syntax and structure.
Let’s take a look at a few basic Python keywords and how they are used:
The ‘if’ Keyword
The ‘if’ keyword is used to create conditional statements in Python. Here’s a simple example:
x = 10
if x > 5:
print('x is greater than 5')
# Output:
# 'x is greater than 5'
In this example, we’ve used the ‘if’ keyword to check if the variable ‘x’ is greater than 5. Since ‘x’ is indeed greater than 5, the print statement is executed.
The ‘for’ Keyword
The ‘for’ keyword is used to create loops in Python. Here’s how it works:
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, we’ve used the ‘for’ keyword to create a loop that prints the numbers 0 through 4. The ‘range’ function generates a sequence of numbers, and the ‘for’ loop iterates over each number in the sequence.
Python keywords are powerful tools that allow you to control the flow of your program. However, it’s important to remember that each keyword has a specific usage and must be used correctly to avoid syntax errors. For example, the ‘if’ keyword must always be followed by a condition, and the ‘for’ keyword must always be followed by an iterable.
Complete List of Keywords
Before we go into more depth, you might find it useful to see a full list of keywords and their descriptions. The table below can be a handy reference:
Keyword | Description | Syntax Example |
---|---|---|
and | A logical operator that returns True if both statements are true | x = 5; y = 12; if x > 1 and y > 1: print(“x and y are greater than 1”) |
as | Used to create an alias | import pandas as pd |
assert | Used for debugging purposes to test a condition | assert x > 1, “x should be greater than 1” |
break | Used to stop the loop before it has looped through all the items | for x in “Python”: if x == "o" : break |
class | Used to create a class | class MyClass: x = 5 |
continue | Skips the current iteration of the loop and continues with the next | for x in “Python”: if x == "o" : continue |
def | Used to define a function | def myFunction: print(“Hello”) |
del | Used to delete a variable | del x |
elif | Used in conditional structures, if the previous conditions were not met, it checks its condition | if x < 5: print(“x is less than 5”) elif x < 10: print(“x is less than 10”) |
else | If the conditions are not met, executes code in the else block | if x > 10: print(“x is larger than 10”) else: print(“x is less than or equal to 10”) |
except | Used in the try..except block, to catch and handle exceptions | try: print(x) except: print(“An exception occurred”) |
False | Represents the boolean value False | print(False) |
finally | At the end of the try…except block. Code inside the finally block will run regardless if there was an exception or not | try: print(x) finally: print(“Finished”) |
for | Used to create a for loop | for x in “Python”: print(x) |
from | Used to import specific parts of a module | from pandas import DataFrame |
global | Used to declare a global variable | global x; x = 10 |
if | Used to create a conditional statement | if x > 5: print(“x is greater than 5”) |
import | Used to import a module | import pandas |
in | Used to check if a value is in a sequence | if “P” in “Python”: print(“P is in Python”) |
is | Used to check if two variables are the same object | if x is y: print(“x and y are the same object”) |
lambda | Used to define a small anonymous function | x = lambda a: a+10 |
None | Represents a null value or a void | x = None |
nonlocal | Used to declare a non-local variable or to indicate that a variable inside a nested function (function inside a function) is not local to it, meaning it belongs to the outer function | def outerFunc(): x = 5; def innerFunc(): nonlocal x; x = 10 |
not | A logical operator that inverts the truth value | if not False: print(“not False, is True!”) |
or | A logical operator that returns True if at least one statement is true | if x > 5 or y > 5: print(“x or y is greater than 5”) |
pass | A placeholder statement, nothing happens when it is executed | def myFunction: pass |
raise | Used to raise an exception | raise TypeError |
return | Used to exit a function and return a value | def x(): return 5 |
True | Represents the boolean value True | print(True) |
try | Used to create a try…except block | try: print(x) |
while | Used to create a while loop | while x < 5: print(x); x += 1 |
with | Used to simplify exception handling by cleaning up and providing some support for the context management protocol | with open(‘file.txt’) as f: read_data = f.read() |
yield | Used in a function like a return statement but it will return a generator | def my_gen(): yield 1; yield 2; yield 3 |
The table above is a complete summary of the Python keywords with their descriptions and an example use case. Refer to it to know specific use cases and syntax expectations.
Advanced Python Keywords Usage
As you progress in your Python journey, the power of Python keywords becomes more evident. Let’s delve deeper into some specific Python keywords and their usage.
The ‘def’ Keyword
The ‘def’ keyword is used to define a function in Python. Functions are reusable chunks of code that perform a specific task.
def greet(name):
print(f'Hello, {name}!')
greet('Alice')
# Output:
# 'Hello, Alice!'
In this example, we’ve defined a function called ‘greet’ that takes one parameter, ‘name’. When we call the function with the argument ‘Alice’, it prints ‘Hello, Alice!’.
The ‘class’ Keyword
The ‘class’ keyword is used to define a class in Python. Classes are the foundation of object-oriented programming in Python.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f'{self.name} says hello!')
animal = Animal('Dog')
animal.speak()
# Output:
# 'Dog says hello!'
In this example, we’ve defined a class called ‘Animal’. The ‘Animal’ class has an __init__
method that sets the name of the animal, and a ‘speak’ method that prints a greeting from the animal. When we create an instance of the ‘Animal’ class and call the ‘speak’ method, it prints ‘Dog says hello!’.
These are just a few examples of Python keywords and their usage. By understanding and correctly using Python keywords, you can write efficient, readable, and powerful Python code.
Alternative Approaches to Python Keywords
While Python keywords are essential in writing standard Python code, there are alternative ways to accomplish similar tasks without using certain keywords. Let’s explore these alternatives.
Using Ternary Expressions
Instead of using the ‘if’ keyword for simple conditions, you can use a ternary expression. A ternary expression allows you to write a shorter version of a simple if-else statement.
x = 10
message = 'x is greater than 5' if x > 5 else 'x is not greater than 5'
print(message)
# Output:
# 'x is greater than 5'
In this example, we’ve used a ternary expression to check if ‘x’ is greater than 5. The expression after the ‘if’ keyword is executed if the condition is true, otherwise the expression after the ‘else’ keyword is executed. Ternary expressions provide a more concise way to write simple if-else statements, but they can be harder to read for complex conditions.
Using List Comprehensions
Instead of using the ‘for’ keyword to create a loop, you can use a list comprehension. A list comprehension allows you to generate a new list from an existing list.
numbers = [1, 2, 3, 4, 5]
squares = [number**2 for number in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, we’ve used a list comprehension to generate a list of squares from the ‘numbers’ list. The expression before the ‘for’ keyword is executed for each item in the ‘numbers’ list. List comprehensions provide a more concise way to create new lists, but they can be harder to read for complex transformations.
These alternative approaches can make your Python code more concise and Pythonic. However, they may also make your code harder to read and understand for beginners. As always, the best approach depends on your specific situation.
Troubleshooting Python Keywords
As you work with Python keywords, you might encounter common issues such as syntax errors. Let’s discuss some of these problems and how to resolve them.
Syntax Errors with Python Keywords
One common issue is syntax errors. These occur when Python keywords are not used correctly in the code. For instance, forgetting the colon at the end of an ‘if’ statement can lead to a syntax error.
x = 10
if x > 5
print('x is greater than 5')
# Output:
# SyntaxError: expected an indented block
In this example, we forgot to include the colon at the end of the ‘if’ statement, leading to a SyntaxError. The solution is simple: always remember to include the colon at the end of your ‘if’, ‘for’, ‘while’, ‘def’, and ‘class’ statements.
Incorrect Keyword Usage
Another common issue is using a Python keyword as an identifier. Remember, Python keywords are reserved words and cannot be used as identifiers such as variable names, function names, or class names.
import = 10
# Output:
# SyntaxError: invalid syntax
In this example, we tried to use ‘import’, which is a Python keyword, as a variable name, leading to a SyntaxError. The solution is to avoid using Python keywords as identifiers.
Understanding Python keywords and their correct usage is key to avoiding these common issues. Always remember the syntax rules for each keyword and avoid using keywords as identifiers.
Understanding Python’s Syntax and Structure
Before diving deeper into Python keywords, it’s important to understand the basic syntax and structure of the Python language. Python’s syntax is designed to be readable and straightforward, which makes it a great language for beginners.
Python Syntax Basics
Python uses indentation to define blocks of code. For example, the code within a function, loop, or conditional statement is indented under that statement. Here’s an example using the ‘if’ keyword:
x = 10
if x > 5:
print('x is greater than 5')
# Output:
# 'x is greater than 5'
In this example, the ‘print’ statement is indented under the ‘if’ statement, indicating that it’s part of the ‘if’ block. If ‘x’ is greater than 5, the ‘print’ statement will be executed.
Python Keywords and Syntax
Python keywords play a crucial role in Python’s syntax. Each keyword has a specific meaning and usage rules. For instance, the ‘def’ keyword is used to define functions, the ‘if’ keyword is used to create conditional statements, and the ‘class’ keyword is used to define classes.
Understanding Python’s syntax and structure will help you better understand the role and importance of Python keywords. In the next sections, we’ll explore each Python keyword in detail, including its usage and examples.
Python Keywords: The Big Picture
Python keywords are not just for small scripts or individual functions. They play a significant role in larger projects, helping to structure your code and control its flow. Mastering Python keywords is a stepping stone towards becoming a proficient Python developer.
Python Keywords in Larger Projects
In larger projects, Python keywords help to structure your code and make it more readable. For instance, the ‘def’ keyword is used to define functions, allowing you to break down your code into manageable chunks. The ‘class’ keyword is used to define classes, enabling you to use object-oriented programming principles to structure your code.
Exploring Related Concepts
Once you’ve mastered Python keywords, there are many related concepts to explore. For instance, you might want to learn about Python operators, which are symbols that perform operations on one or more operands. You might also want to delve deeper into Python data types, which define the type of value that a variable can hold.
Further Resources for Mastering Python Keywords
There are plenty of resources available to help you continue your Python journey. Here are a few recommended ones:
- In Python: Exploring Usage and Syntax – Explore the usage of “in” keyword in Python for efficient data searching.
Python.org’s Official Documentation provides a comprehensive overview of Python keywords.
GeeksforGeeks’ Python Keywords offers a detailed guide on Python keywords with examples.
Real Python offers a wealth of tutorials and articles on various Python topics, including Python keywords.
Remember, mastering Python keywords is a journey. Don’t rush it. Take your time to understand each keyword, practice using it in different contexts, and before you know it, you’ll be writing Python code like a pro!
Wrapping Up: Mastering Python Keywords
In this comprehensive guide, we’ve delved into the world of Python keywords, exploring their importance in crafting efficient and readable Python code.
We began with the basics, shedding light on what Python keywords are and their significance in Python programming. We then progressed to more advanced usage, discussing specific Python keywords, their roles in Python syntax, and how they control the flow of a Python program.
We also explored alternative approaches to tasks without using certain keywords, introducing concepts like ternary expressions and list comprehensions for more concise and Pythonic code.
Along the way, we tackled common challenges you might encounter when using Python keywords and provided solutions to help you overcome these hurdles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Using Python Keywords | Standard, widely understood | Can be verbose for simple tasks |
Using Ternary Expressions | More concise for simple conditions | Harder to read for complex conditions |
Using List Comprehensions | More concise for creating new lists | Harder to read for complex transformations |
Whether you’re a beginner just starting out with Python or an experienced developer looking to refine your skills, we hope this guide has deepened your understanding of Python keywords and their usage.
Now, you’re well equipped to write more efficient, readable, and powerful Python code. Happy coding!