Numpy.vstack() Function | Array Stacking Guide
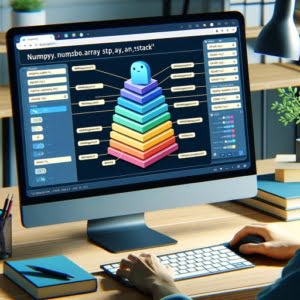
Struggling with stacking arrays in Python? Like a skilled builder, Numpy’s vstack function can help you construct a larger array from smaller ones.
This guide will walk you through the ins and outs of using numpy.vstack. We’ll cover everything from the basics of how numpy.vstack works, to advanced techniques and alternative approaches.
So let’s dive in and start stacking those arrays!
TL;DR: How Do I Use numpy vstack in Python?
To stack arrays vertically in Python, you use the
numpy.vstack()
function. Here’s a simple example:
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
c = np.vstack((a,b))
print(c)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we first import the numpy module. We then create two 1-dimensional arrays, a
and b
. The numpy.vstack()
function is used to stack these arrays vertically, resulting in a 2-dimensional array c
. The print statement outputs this new array.
This is just the tip of the iceberg when it comes to numpy.vstack. Read on for more detailed explanations and advanced usage scenarios.
Table of Contents
- Understanding the Basics: numpy.vstack()
- Handling Different Shapes and Dimensions with numpy.vstack
- Exploring Alternative Approaches to Vertical Stacking
- Troubleshooting Common Issues with numpy.vstack
- Unraveling the Numpy Library and Array Data Type
- numpy.vstack: More Than Just Stacking Arrays
- Further Resources for Numpy Mastery
- Wrapping Up: Mastering numpy.vstack in Python
Understanding the Basics: numpy.vstack()
The numpy.vstack()
function is a tool in Python’s Numpy library that allows you to stack arrays vertically. In simpler terms, it takes a sequence of arrays and stacks them vertically to make a single array.
Let’s explore this concept with a basic example:
import numpy as np
# Create two 1-dimensional arrays
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# Use numpy.vstack() to stack them vertically
c = np.vstack((a,b))
print(c)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we’ve created two 1-dimensional arrays a
and b
. When we pass these arrays to the numpy.vstack()
function, it stacks them vertically, hence creating a new 2-dimensional array c
. The print statement then outputs this new array.
One of the main advantages of numpy.vstack()
is its ability to handle arrays of different dimensions. It can automatically promote lower-dimensional arrays to match the highest dimension of the input arrays. However, it’s important to note that all arrays must have the same shape along all but the first axis.
This is just a basic use case of numpy.vstack()
. As we move forward, we’ll explore more complex scenarios and how to handle potential pitfalls.
Handling Different Shapes and Dimensions with numpy.vstack
As we move forward with the numpy.vstack()
function, it’s crucial to understand how it deals with arrays of different shapes and dimensions. Remember, the function can handle arrays of different dimensions by promoting lower-dimensional arrays to match the highest dimension of the input arrays. However, all arrays must have the same shape along all but the first axis.
Let’s illustrate this with a code example involving multi-dimensional arrays:
import numpy as np
# Create two 2-dimensional arrays
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.array([[7, 8, 9], [10, 11, 12]])
# Use numpy.vstack() to stack them vertically
c = np.vstack((a,b))
print(c)
# Output:
# [[ 1 2 3]
# [ 4 5 6]
# [ 7 8 9]
# [10 11 12]]
In this example, we’ve created two 2-dimensional arrays a
and b
. When we pass these arrays to the numpy.vstack()
function, it stacks them vertically, hence creating a new 2-dimensional array c
that has four rows.
The key takeaway here is that numpy.vstack()
can seamlessly handle multi-dimensional arrays, provided they have the same shape along all but the first axis. This makes it a versatile tool for data manipulation in Python, particularly in scenarios where you need to combine data from different sources or formats.
Exploring Alternative Approaches to Vertical Stacking
While numpy.vstack()
is a powerful tool for vertical stacking, it’s not the only method available in Python’s Numpy library. One viable alternative is the numpy.concatenate()
function. This function also stacks arrays, but it provides the flexibility to specify the axis along which the concatenation should occur.
Let’s illustrate this with an example:
import numpy as np
# Create two 1-dimensional arrays
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
# Use numpy.concatenate() to stack them vertically
c = np.concatenate((a,b), axis=0)
print(c)
# Output:
# [1 2 3 4 5 6]
In this example, we’ve used numpy.concatenate()
with the axis
parameter set to 0 to stack two 1-dimensional arrays vertically. The result is a new 1-dimensional array c
.
The advantage of numpy.concatenate()
is its flexibility – it can concatenate along any given axis. However, it requires that all input arrays have the same shape except along the specified axis. On the other hand, numpy.vstack()
is more straightforward for vertical stacking, but it’s less flexible than numpy.concatenate()
.
In conclusion, the choice between numpy.vstack()
and numpy.concatenate()
depends on your specific needs. If you need straightforward vertical stacking, numpy.vstack()
is the way to go. But if you need more flexibility in terms of the axis of concatenation, numpy.concatenate()
might be a better choice.
Method | Flexibility | Use Case |
---|---|---|
numpy.vstack() | Less flexible; stacks vertically | Straightforward vertical stacking |
numpy.concatenate() | More flexible; can concatenate along any axis | Requires specific axis concatenation |
Troubleshooting Common Issues with numpy.vstack
While numpy.vstack()
is a reliable function for vertical stacking of arrays in Python, you might encounter some issues during its use. One of the most common issues is the ValueError: all the input array dimensions except for the concatenation axis must match exactly
. This error occurs when you try to stack arrays that don’t have the same shape along all but the first axis.
Let’s illustrate this with a code example:
import numpy as np
# Create two arrays with different shapes
a = np.array([1, 2, 3])
b = np.array([[4, 5, 6], [7, 8, 9]])
try:
c = np.vstack((a,b))
except ValueError as e:
print(e)
# Output:
# all the input array dimensions for the concatenation axis must match exactly
In this example, we’ve tried to stack a 1-dimensional array a
and a 2-dimensional array b
using numpy.vstack()
. However, since these arrays don’t have the same shape along all but the first axis, the function raises a ValueError
.
The solution to this issue is to ensure that all input arrays have the same shape along all but the first axis. This might involve reshaping your arrays before using numpy.vstack()
. It’s also a good practice to always check the shapes of your arrays before stacking them.
Understanding these considerations and potential pitfalls will help you use numpy.vstack()
more effectively in your Python projects.
Unraveling the Numpy Library and Array Data Type
Before we dive deeper into numpy.vstack()
, it’s essential to understand the basics of Python’s Numpy library and the array data type. Numpy, which stands for ‘Numerical Python’, is a powerful library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
In Numpy, the term ‘array’ refers to a grid of elements, which can be indexed by integers. These arrays can be one-dimensional (like a simple list of numbers), two-dimensional (like a table of numbers with rows and columns), or even multi-dimensional.
Here’s a simple example of a Numpy array:
import numpy as np
# Create a 1-dimensional array
a = np.array([1, 2, 3])
print(a)
# Output:
# [1 2 3]
In this example, we’ve created a 1-dimensional array a
using the numpy.array()
function. The print statement outputs this array.
An important concept in Numpy is the shape of an array, which is a tuple indicating the size of the array in each dimension. For a 2-dimensional array, the shape would be (n,m) where n is the number of rows and m is the number of columns. For a 1-dimensional array, the shape would be (n,) where n is the number of elements in the array.
Understanding these fundamental concepts is crucial for working with numpy.vstack()
, as the function operates on Numpy arrays and relies on their shapes and dimensions.
numpy.vstack: More Than Just Stacking Arrays
The numpy.vstack()
function is more than just a tool for stacking arrays – it’s a fundamental part of data manipulation in Python, with wide-ranging applications in fields like data analysis and machine learning.
In data analysis, you often need to combine data from different sources or formats. This is where numpy.vstack()
comes in handy, allowing you to stack arrays vertically and create a single, unified dataset.
In machine learning, numpy.vstack()
is useful for preparing your data. You might need to stack different features of your dataset vertically before feeding it into your machine learning model. Here’s an illustrative example:
import numpy as np
# Create two arrays representing different features
feature1 = np.array([1, 2, 3])
feature2 = np.array([4, 5, 6])
# Stack them vertically to create a dataset
dataset = np.vstack((feature1, feature2))
print(dataset)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we’ve created a dataset by stacking two arrays feature1
and feature2
representing different features of the dataset.
While numpy.vstack()
is a powerful tool, it’s just one piece of the puzzle. There are many related concepts worth exploring, such as horizontal stacking of arrays using numpy.hstack()
, reshaping arrays using numpy.reshape()
, and many more. Each of these functions has its own use cases and advantages, and understanding them will give you a more comprehensive grasp of data manipulation in Python.
Further Resources for Numpy Mastery
To delve deeper into Numpy topics, Click Here for a Complete Guide on Numpy in Python. This guide will explore the benefits of using NumPy for numerical computing in various scientific disciplines.
You can also consider checking out the following resources for additional Numpy related concepts
- Transposing Arrays with numpy.transpose() – Discover how to transpose NumPy arrays in Python using the “numpy transpose” operation.
np.linspace() Guide with Examples – Learn about generating evenly spaced values in NumPy for numerical simulations.
Official Numpy Documentation – The official documentation of Numpy offering a detailed overview of the module’s functionalities.
Numpy Questions on StackOverflow – A collection of StackOverflow questions and answers focused on Numpy troubleshooting and learning.
Numpy Essentials for Data Science – A Towards Data Science article covering the fundamental concepts of Numpy for data science.
Wrapping Up: Mastering numpy.vstack in Python
In this guide, we’ve taken a deep dive into the numpy.vstack()
function in Python. We’ve explored its basic usage, handling arrays of different shapes and dimensions, and even delved into some common issues and their solutions.
At its core, numpy.vstack()
is a versatile tool for stacking arrays vertically, making it invaluable for data manipulation in Python. Remember, the function can handle arrays of different dimensions, but all arrays must have the same shape along all but the first axis.
We also looked at alternative approaches like the numpy.concatenate()
function. While numpy.vstack()
is more straightforward for vertical stacking, numpy.concatenate()
offers more flexibility in terms of the axis of concatenation.
Method | Flexibility | Use Case |
---|---|---|
numpy.vstack() | Less flexible; stacks vertically | Straightforward vertical stacking |
numpy.concatenate() | More flexible; can concatenate along any axis | Requires specific axis concatenation |
Remember, the choice between numpy.vstack()
and numpy.concatenate()
depends on your specific needs. Always consider the shape and dimensions of your arrays before choosing a method.
In conclusion, mastering numpy.vstack()
opens up a world of possibilities in data manipulation, analysis, and machine learning in Python. Happy coding!