Install Winston Logging for Node.js | npm User’s Guide
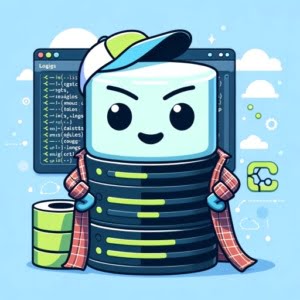
While configuring logging processes for Node.js projects at IOFLOOD, we had to explore various logging libraries. After thorough research and testing, we found Winston to be a reliable choice due to its extensive feature set and customizable configurations. To assist fellow developers facing similar hurdles, we’ve curated this comprehensive guide on using Winston with npm. By sharing practical insights and step-by-step instructions, we aim to simplify your logging implementations.
This guide will walk you through the basics to advanced usage of Winston, making your logging efficient and your debugging process smoother. Whether you’re just starting out with Node.js or you’re a seasoned developer looking for more robust logging solutions, Winston offers the tools you need to keep your application’s logs in check.
Let’s dive in and explore how Winston can transform your logging strategy from a cumbersome chore into a streamlined process.
TL;DR: How Do I Start Logging in Node.js Using Winston?
To start logging with Winston in Node.js, first install it using npm and the command,
npm install winston
, then import Winston into your Node.js application with the code,const winston = require('winston');
. Afterwards, you may adjust the logger configuration to meet your project’s specific requirements.
Here’s a quick configuration example:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'combined.log' })
]
});
logger.info('Hello, Winston!');
# Output:
# 'Hello, Winston!'
In this example, we demonstrate how to quickly set up Winston for logging in a Node.js application. By requiring the winston
module and configuring a logger with both console and file transports, you can start logging messages right away. The logger.info('Hello, Winston!');
line logs a simple message, showcasing the ease with which Winston integrates into your Node.js projects.
For more detailed instructions and advanced features, keep reading to master logging with Winston and enhance your Node.js applications.
Table of Contents
Getting Started with Winston
Logging is an essential part of application development. It helps in debugging and monitoring applications effectively. If you’re new to Node.js or have been working with it but haven’t explored logging deeply, Winston npm is a powerful tool that can simplify your logging needs.
Installing Winston
The first step to using Winston is to install it via npm. Ensure you have Node.js and npm installed on your machine. Then, run the following command in your project directory:
npm install winston
This command installs Winston in your project, adding it to your node_modules
folder and listing it as a dependency in your package.json
file.
Creating Your First Logger
Once Winston is installed, you can easily create your first logger. Here’s how:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'app.log' })
]
});
logger.log('info', 'This is an informational message');
# Output:
# { level: 'info', message: 'This is an informational message' }
In this example, we’ve created a logger that has two transports: one for the console and another for writing to a file named ‘app.log’. The logger.log
method is used to log an informational message. The output shows how the message will appear, indicating the logging level (info
) and the message content.
Understanding Basic Logging Levels
Winston provides several logging levels such as error
, warn
, info
, http
, verbose
, debug
, and silly
. These levels allow you to categorize your logs based on their importance and the detail they provide. For most applications, starting with info
for general messages and error
for errors is a good practice.
By mastering these basics, you’ve taken the first step towards efficient logging in your Node.js applications with Winston. As you become more familiar with Winston, you’ll discover its power in making your debugging process smoother and your application maintenance easier.
Advanced Features of Winston
Once you’ve mastered the basics of Winston npm for logging in your Node.js applications, it’s time to dive into its more advanced capabilities. Winston’s true power lies in its flexibility and the extensive customization it offers. From defining custom logging levels to utilizing various transports and log formatting, Winston ensures that your logging strategy can be as simple or sophisticated as you need.
Custom Logging Levels
Custom logging levels allow you to create a tailored logging hierarchy, making your logs more intuitive and easier to filter. Here’s how you can define custom levels in Winston:
const winston = require('winston');
const customLevels = {
levels: {
fatal: 0,
error: 1,
warn: 2,
info: 3,
debug: 4,
trace: 5
},
colors: {
fatal: 'red',
error: 'orange',
warn: 'yellow',
info: 'green',
debug: 'blue',
trace: 'magenta'
}
};
const logger = winston.createLogger({
levels: customLevels.levels,
transports: [
new winston.transports.Console({
format: winston.format.combine(
winston.format.colorize(),
winston.format.simple()
)
})
]
});
winston.addColors(customLevels.colors);
logger.fatal('This is a fatal error!');
logger.trace('Tracing the issue...');
# Output:
# This is a fatal error! (in red)
# Tracing the issue... (in magenta)
In this example, we defined a set of custom logging levels and associated colors for each level. By using winston.createLogger
with our custom levels and adding a console transport that combines colorization with simple formatting, we can produce logs that are both informative and visually distinct. This customization enhances the readability of logs, especially when monitoring applications in real-time.
Advanced Transports and Log Formatting
Winston supports a variety of transports – mechanisms through which logs are outputted or stored. Beyond the console and file transports covered in the basics, Winston allows for logging to databases, remote services, and more. Coupled with advanced formatting options, you can ensure that your logs are not only stored appropriately but are also easy to read and interpret.
Here’s an example showcasing a custom transport and formatting:
const winston = require('winston');
const customFormat = winston.format.printf(({ level, message, label, timestamp }) => {
return `${timestamp} [${label}] ${level}: ${message}`;
});
const logger = winston.createLogger({
format: winston.format.combine(
winston.format.label({ label: 'MyApp' }),
winston.format.timestamp(),
customFormat
),
transports: [
new winston.transports.Http({
level: 'warn',
format: winston.format.json()
})
]
});
logger.warn('Warning: Configuration mismatch detected.');
# Output:
# 2023-03-15T12:34:56.789Z [MyApp] warn: Warning: Configuration mismatch detected.
This code snippet demonstrates the use of a custom format along with an HTTP transport. Logs are now prefixed with a timestamp, label, and level, making them more descriptive. The HTTP transport could be used to send warning-level logs to a remote logging server or service, showcasing Winston’s capability to adapt to various logging requirements.
By leveraging Winston’s advanced features, you can create a comprehensive logging strategy that not only aids in debugging but also in monitoring your Node.js applications’ health and performance.
Exploring Alternatives to Winston NPM
While Winston is a powerful logging library for Node.js, it’s part of a broader ecosystem of logging solutions. Understanding how Winston compares to other libraries and how it can be integrated with other Node.js frameworks is crucial for creating the most effective logging strategy for your applications.
Winston vs. Other Logging Libraries
There are several other logging libraries available for Node.js, such as Bunyan and Morgan. Each has its strengths and use cases.
- Bunyan is another popular Node.js logging library that focuses on JSON logging, making logs easily parseable by machines. It’s particularly useful in microservices architectures where logs from several services need to be aggregated and analyzed.
Morgan is designed for logging HTTP requests in Node.js applications. It’s incredibly useful in web applications for monitoring the nature and volume of incoming requests.
Here’s how you might configure Bunyan for basic logging, to compare with Winston:
const bunyan = require('bunyan');
const log = bunyan.createLogger({name: 'myapp'});
log.info('Hello from Bunyan');
# Output:
# {"name":"myapp","hostname":"yourHost","pid":123,"level":30,"msg":"Hello from Bunyan","time":"2023-03-15T12:34:56.789Z","v":0}
This code snippet demonstrates Bunyan’s default behavior of logging in a JSON format. This structured logging is incredibly useful for automated log analysis tools.
Integrating Winston with Node.js Frameworks
Winston can be seamlessly integrated into various Node.js frameworks such as Express.js, Koa, and Hapi. This integration allows for more granular control over logging, especially in web applications where you might want to log specific routes, request types, or response statuses.
For instance, integrating Winston with Express.js to log all incoming requests might look like this:
const express = require('express');
const winston = require('winston');
const expressWinston = require('express-winston');
const app = express();
app.use(expressWinston.logger({
transports: [
new winston.transports.Console()
],
format: winston.format.combine(
winston.format.colorize(),
winston.format.json()
)
}));
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => console.log('App listening on port 3000'));
# Output when visiting '/':
# {"req":{"method":"GET","url":"/","headers":{...},"remoteAddress":"::1","remotePort":12345},"res":{"statusCode":200,"responseTime":5},"message":"HTTP GET /"}
In this example, express-winston
middleware is used to automatically log all incoming requests to the Express.js application. The logs include detailed information about each request, such as the method, URL, response status, and response time, making it easier to monitor the application’s health and performance.
By considering alternative logging libraries and integrating Winston with other Node.js frameworks, you can tailor your logging strategy to meet the specific needs of your application, whether it requires simple logging, structured JSON logs for machine analysis, or detailed request logging in web applications.
Issues and Solutions for Winston
Even the most robust tools like Winston npm for Node.js logging can present challenges. Understanding common issues and how to address them can save you time and headaches. This section covers troubleshooting tips for logging levels, file management, and asynchronous logging – ensuring your logging strategy remains effective and efficient.
Logging Levels: Ensuring Accuracy
A common issue developers face with Winston is incorrect logging levels leading to missing or unexpected log entries. This often occurs due to misconfiguration or misunderstanding of the logging levels hierarchy.
const winston = require('winston');
const logger = winston.createLogger({
level: 'warn',
transports: [
new winston.transports.Console()
]
});
logger.info('This will not show.');
logger.warn('This will show.');
# Output:
# 'This will show.'
In this example, the logger is configured with a ‘warn’ level, meaning only logs of ‘warn’ level or higher (e.g., ‘error’) will be outputted. The ‘info’ level message is not shown because it’s lower than the configured ‘warn’ level. Understanding and correctly setting the logging level is crucial for capturing the intended log messages.
File Management: Handling Log Rotation
Managing log files effectively is vital to prevent them from consuming too much disk space. Winston doesn’t handle log rotation natively, but it can be integrated with modules like ‘winston-daily-rotate-file’ for this purpose.
const winston = require('winston');
require('winston-daily-rotate-file');
const transport = new winston.transports.DailyRotateFile({
filename: 'application-%DATE%.log',
datePattern: 'YYYY-MM-DD',
maxSize: '20m',
maxFiles: '14d'
});
const logger = winston.createLogger({
transports: [transport]
});
logger.info('Log rotation example.');
# Output:
# Log file created with date and size constraints.
This setup configures Winston to rotate log files daily, with each file named by the date. It limits log files to 20MB and retains them for 14 days. Implementing log rotation helps manage disk space and keeps logs organized and accessible.
Asynchronous Logging: Managing Performance
Asynchronous logging can improve your application’s performance by offloading the logging process. However, it requires careful handling to ensure logs are not lost during application shutdown or crashes.
const winston = require('winston');
const { promisify } = require('util');
const fs = require('fs');
const writeFile = promisify(fs.writeFile);
const logger = winston.createLogger({
transports: [
new winston.transports.File({ filename: 'async-log.log' })
]
});
async function logMessage(message) {
await writeFile('async-log.log', message);
logger.info('Message logged asynchronously.');
}
logMessage('Testing asynchronous logging.');
# Output:
# 'Message logged asynchronously.'
This example demonstrates a simple asynchronous logging setup using Node.js’s native fs
module and promisify
to write log messages to a file asynchronously. While Winston handles the logging internally, this approach shows how you can extend logging functionality to suit your application’s needs, ensuring performance isn’t compromised.
By addressing these common issues with informed solutions, you can leverage Winston npm for Node.js logging more effectively, making your application’s logging strategy robust and reliable.
The Importance of Logging
Logging is a cornerstone of software development, serving as the eyes and ears of developers, especially when it comes to debugging and monitoring applications. It’s the process of recording information about the operation of a program, providing a traceable history of events, errors, and system states. Effective logging can mean the difference between quickly resolving an issue and spending hours, if not days, troubleshooting.
Winston in the Node.js Ecosystem
Within the Node.js ecosystem, Winston npm has emerged as a leading logging solution, favored for its versatility and ease of use. Winston allows developers to log messages with varying levels of severity, from critical errors (error
) to verbose debugging information (debug
). This flexibility makes Winston an invaluable tool in a developer’s arsenal.
Here’s a brief example demonstrating how Winston can log different severity levels:
const winston = require('winston');
const logger = winston.createLogger({
level: 'debug',
format: winston.format.simple(),
transports: [
new winston.transports.Console()
]
});
logger.error('Error level log');
logger.warn('Warn level log');
logger.info('Info level log');
logger.debug('Debug level log');
# Output:
# Error level log
# Warn level log
# Info level log
# Debug level log
In this code snippet, we configure Winston to log messages of all levels to the console. The output demonstrates Winston’s ability to categorize logs based on severity, which is crucial for filtering and analyzing log data effectively.
Fundamentals of Logging Process
The logging process involves more than just printing messages to a console or file. It encompasses the creation, formatting, filtering, and storage of log data. A well-designed logging system provides clear, concise, and useful information that can be acted upon, without overwhelming the developer with noise.
Winston’s architecture supports this process by offering customizable transports for outputting logs, various formats for log messages, and the ability to set logging levels. This ensures that logs are not only informative but are also manageable and relevant to the context in which they’re needed.
By understanding the importance of logging and how tools like Winston fit into the Node.js ecosystem, developers can build more resilient and maintainable applications. Logging with Winston npm provides a foundation for effective debugging and monitoring, essential components of successful software development.
Additional Usage Cases with Winston
As you become more proficient in managing logs within your Node.js applications using Winston, you might wonder what’s next. Logging is not just about capturing information; it’s a critical component of application monitoring and performance optimization. By leveraging the right tools and libraries alongside Winston, you can elevate your logging strategy to not only detect and diagnose issues but also to proactively improve your application’s health and efficiency.
Integrating Monitoring Tools
One of the powerful aspects of logging is its role in application monitoring. Tools such as Datadog, Splunk, and Elasticsearch provide platforms for log aggregation, analysis, and visualization. These tools can ingest logs generated by Winston, allowing you to monitor your application’s performance in real-time, set up alerts based on specific log patterns, and analyze historical data for trends.
Consider integrating Winston with Elasticsearch for powerful log analysis and visualization capabilities:
const winston = require('winston');
require('winston-elasticsearch');
const esTransportOpts = {
level: 'info',
clientOpts: {
node: 'http://localhost:9200'
}
};
const logger = winston.createLogger({
transports: [
new winston.transports.Elasticsearch(esTransportOpts)
]
});
logger.info('Log message for Elasticsearch');
# Output:
# Document indexed in Elasticsearch with message 'Log message for Elasticsearch'
In this example, we configure Winston to send logs to Elasticsearch. This setup enables you to leverage Elasticsearch’s powerful search and analysis features, making it easier to derive insights from your logs.
Optimizing Performance with Logs
Logs can also play a critical role in performance optimization. By analyzing log data, you can identify bottlenecks, inefficient operations, and potential areas for optimization. This proactive approach to using logs can significantly enhance the user experience and reduce operational costs.
Further Resources for Mastering Winston Logging
To continue your journey in mastering logging with Winston and exploring its integration with monitoring and analysis tools, here are three recommended resources:
- Winston GitHub Repository: Explore Features and Configurations – Dive deep into the features and configurations of Winston through its official GitHub repository.
Elasticsearch for Logging: Comprehensive Log Management – Learn to leverage the power of Elasticsearch for managing your application logs from the official website.
Datadog for Node.js Monitoring: Complement Your Logging Strategy – Discover how Datadog can enhance your logging strategy for Node.js applications on their official website.
By exploring these resources and integrating advanced logging, monitoring, and analysis tools with Winston, you can unlock new levels of insight and control over your Node.js applications.
Recap: Winston Logging in Node.js
In this comprehensive guide, we’ve explored the ins and outs of using Winston npm for logging in Node.js applications. From the initial setup to diving into the library’s advanced features, we’ve covered everything you need to know to implement effective logging strategies in your projects.
We began with the basics, showing you how to install Winston and create your first logger. We then moved on to more advanced topics, such as custom logging levels, transports, and log formatting, providing you with the tools to tailor Winston to your application’s specific needs.
Next, we discussed alternative logging libraries and how Winston compares, offering insights into integrating Winston with other Node.js frameworks for more complex logging solutions. We also tackled common troubleshooting issues, ensuring you’re well-equipped to handle any logging challenges that come your way.
Feature | Winston | Alternative Libraries |
---|---|---|
Customizability | High | Varies |
Ease of Use | High | Moderate to High |
Integration with Node.js | Seamless | Requires Additional Configuration |
Whether you’re just starting out with logging in Node.js or looking to enhance your application’s logging capabilities, we hope this guide has provided you with valuable insights into Winston’s powerful features and how to use them effectively.
Effective logging is crucial for the development and maintenance of any application. With Winston, you can ensure that your logging strategy is not only efficient but also adaptable to the evolving needs of your project. Happy logging!