Math.max() Java Function | Usage, Syntax, and Examples
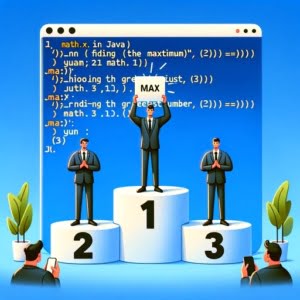
Are you finding it challenging to determine the maximum of two numbers in Java? You’re not alone. Many developers grapple with this task, but there’s a function that can make this process a breeze.
Like a competitive athlete, the Math.max() function in Java can help you find the winner. This function is a handy utility that can seamlessly find the maximum of two numbers, making it a valuable tool for various tasks.
This guide will walk you through the usage of Math.max() function in Java, from the basics to more advanced techniques. So, let’s dive in and start mastering Math.max in Java!
TL;DR: How Do I Find the Maximum of Two Numbers in Java?
To find the maximum of two numbers in Java, you can use the Math.max() function, with the syntax
int max = Math.max(firstInt, secondInt)
. This function takes two arguments and returns the larger of the two.
Here’s a simple example:
int max = Math.max(5, 10);
System.out.println(max);
# Output:
# 10
In this example, we use the Math.max() function to find the maximum of the numbers 5 and 10. The function returns 10, which is the larger of the two numbers, and this value is then printed to the console.
This is a basic way to use Math.max in Java, but there’s much more to learn about this function and its applications. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
Understanding Math.max: Basic Use
The Math.max() function in Java is a static method that belongs to the Math class. This function takes two numbers as arguments and returns the one with the higher value. It’s a straightforward and efficient way to find the maximum of two numbers.
Here’s a basic example of how to use the Math.max() function:
int num1 = 5;
int num2 = 10;
int max = Math.max(num1, num2);
System.out.println(max);
# Output:
# 10
In this example, we have two integers, 5 and 10. We use Math.max() to find the maximum of these two values. The function returns 10, which is stored in the variable ‘max’ and then printed to the console.
Advantages of Math.max()
The Math.max() function is a simple and efficient way to find the maximum of two numbers. It’s a built-in function in Java, which means you don’t need to write any additional code to use it. This can make your code cleaner and easier to read.
Potential Pitfalls
While Math.max() is a useful function, there are a few things to keep in mind. It only works with two arguments. If you want to find the maximum of more than two numbers, you’ll need to call the function multiple times or use a different approach (which we’ll discuss in the ‘Advanced Use’ section).
Also, the Math.max() function can only compare numbers. If you try to use it with non-numerical values, your program will throw an error. Always make sure your inputs are numbers when using Math.max().
Advanced Use of Math.max: Handling Arrays and Collections
While the basic use of the Math.max() function is limited to two numbers, its application can be extended to handle more complex scenarios, such as finding the maximum in an array or a collection. This can be achieved by using the function in a loop or in conjunction with other Java features.
Finding Maximum in an Array with Math.max()
Consider you have an array of numbers and you want to find the maximum value. You can do this by initializing a variable to the first element of the array and then iterating through the array, using Math.max() to compare each element with the current maximum.
Here’s an example:
int[] numbers = {1, 2, 3, 4, 5};
int max = numbers[0];
for (int number : numbers) {
max = Math.max(max, number);
}
System.out.println(max);
# Output:
# 5
In this example, we start by initializing ‘max’ to the first element of the array. We then use a for-each loop to iterate through the array. For each element, we use Math.max() to compare the element with the current maximum, updating ‘max’ if the element is larger. After the loop, ‘max’ holds the maximum value in the array, which is then printed to the console.
Best Practices
When using Math.max() with arrays or collections, it’s important to handle edge cases. For instance, if the array is empty, the above code will throw an error, as there’s no ‘first element’ to initialize ‘max’ to. Always check if the array is empty before trying to find its maximum.
In addition, remember that Math.max() can only compare two numbers at a time. If you’re dealing with a large collection, using Math.max() in a loop could be less efficient than other methods. In such cases, consider using other Java features, such as streams, which we’ll discuss in the ‘Beyond’ section.
Exploring Alternatives to Math.max in Java
While Math.max() is a straightforward and efficient way to find the maximum of two numbers, Java offers other methods that can be used in specific scenarios. Let’s explore some of these alternatives, including the ternary operator and sorting an array.
Using the Ternary Operator
The ternary operator is a shorthand way of writing an if-else statement. It can be used to find the maximum of two numbers as follows:
int num1 = 5;
int num2 = 10;
int max = (num1 > num2) ? num1 : num2;
System.out.println(max);
# Output:
# 10
In this example, the expression (num1 > num2) ? num1 : num2;
checks if ‘num1’ is greater than ‘num2’. If it is, ‘num1’ is returned; otherwise, ‘num2’ is returned. This is a quick and concise way to find the maximum of two numbers, though it may be less readable than Math.max() for those unfamiliar with the ternary operator.
Sorting an Array to Find the Maximum
If you have an array of numbers and want to find the maximum, one approach is to sort the array in ascending order and pick the last element. Here’s how you can do this in Java:
import java.util.Arrays;
int[] numbers = {1, 2, 3, 4, 5};
Arrays.sort(numbers);
int max = numbers[numbers.length - 1];
System.out.println(max);
# Output:
# 5
In this example, we use the Arrays.sort() method to sort the array in ascending order, and then we pick the last element, which is the maximum. This method can be useful if you need to sort the array for other purposes. However, it’s less efficient than Math.max() or a loop for finding the maximum, as sorting takes O(n log n) time while the other methods take O(n) time.
Choosing the Right Method
Each of these methods has its advantages and disadvantages. Math.max() is simple and efficient, but it only works with two numbers. The ternary operator is concise and can work with any two comparable values, but it may be less readable. Sorting an array can find the maximum and sort the array in one go, but it’s less efficient. Choose the method that best fits your needs and the specific scenario you’re dealing with.
Troubleshooting Math.max: Common Issues and Solutions
While using Math.max() in Java is generally straightforward, there are a few common issues that you might encounter. In this section, we’ll discuss how to handle these situations and provide solutions and workarounds.
Handling NaN Values
One potential issue when using Math.max() is dealing with NaN (Not a Number) values. If either of the arguments passed to Math.max() is NaN, the function will return NaN. Here’s an example:
double max = Math.max(5, Double.NaN);
System.out.println(max);
# Output:
# NaN
In this example, we try to find the maximum of 5 and NaN. The Math.max() function returns NaN, which is then printed to the console.
To avoid this, you can check if either of the arguments is NaN before calling Math.max(). If an argument is NaN, you can handle it in a way that makes sense for your program, such as treating it as a specific value or ignoring it.
Dealing with Negative Numbers
Another consideration when using Math.max() is handling negative numbers. The function works with negative numbers just like it does with positive numbers, returning the number that is closer to positive infinity. However, if you’re dealing with negative numbers and you want to find the one that is ‘least negative’ (i.e., closest to zero), you might need to adjust your approach.
Here’s an example:
int max = Math.max(-5, -10);
System.out.println(max);
# Output:
# -5
In this example, we use Math.max() to find the maximum of -5 and -10. The function returns -5, which is closer to positive infinity, even though -5 is actually the ‘least negative’ of the two numbers.
To find the ‘least negative’ number, you could use Math.max() with the absolute values of the numbers, and then take the negative of the result. However, this might not be the most efficient or clear solution, especially if you’re dealing with large amounts of data or complex calculations. Always consider the specific requirements of your program and choose the approach that best meets your needs.
Understanding Java’s Math Class
To better comprehend the Math.max() function, it’s beneficial to delve into the Math class in Java and its methods. The Math class, part of java.lang package, is a utility class that houses numerous static methods for performing common mathematical functions.
Dive into Java’s Math Class
double pi = Math.PI;
System.out.println(pi);
# Output:
# 3.141592653589793
In this example, we’re accessing the constant PI from the Math class, which represents the ratio of the circumference of a circle to its diameter, approximately 3.14.
Exploring Math Class Methods
The Math class provides a plethora of methods to perform mathematical operations. These include trigonometric operations, logarithmic calculations, exponentiation, rounding, and more. Of course, it also includes the Math.max() function we’re focusing on in this guide.
Here’s an example of another method from the Math class:
int absValue = Math.abs(-10);
System.out.println(absValue);
# Output:
# 10
In this example, we’re using the Math.abs() function, which returns the absolute value of a number. We pass in -10, and the function returns 10, the absolute value.
Understanding the Math class and its methods provides a solid foundation for understanding the Math.max() function. It’s part of a larger toolkit that Java provides for performing mathematical operations, making it a vital part of any Java developer’s skillset.
Extending Your Knowledge Beyond Math.max
The Math.max() function is just one piece of the puzzle when it comes to data manipulation and analysis in Java. Understanding its use and application can open the door to a deeper understanding of sorting algorithms, data analysis applications, and other related concepts.
Relevance of Maximum in Sorting Algorithms
Finding the maximum of a collection of numbers is a common operation in many sorting algorithms. For example, in selection sort, one of the simplest sorting algorithms, the maximum (or minimum, depending on the order of sorting) of the unsorted part of the array is repeatedly identified and moved to the sorted part of the array. Understanding how to efficiently find the maximum of a collection is therefore key to understanding and implementing these algorithms.
Data Analysis Applications
In data analysis, finding the maximum is often a crucial step in understanding your data. For example, the maximum can give you a sense of the range of your data, help identify outliers, or allow you to normalize your data. The Math.max() function, therefore, is not just a tool for programming, but also a tool for understanding and interpreting data.
Exploring Related Concepts
If you’re interested in diving deeper into Java and its capabilities for data manipulation and analysis, there are many related concepts and functions to explore. For instance, other functions in the Math class, such as Math.min(), Math.abs(), and Math.sqrt(), provide more tools for working with numerical data. Additionally, Java’s powerful features for working with arrays and collections, such as streams and the Collections framework, offer more advanced tools for data manipulation.
Further Resources for Mastering Java’s Math Class
To continue expanding your knowledge and skills, consider exploring the following resources:
- IOFlood’s Java Math Class Guide – Discover Java’s Math class for handling exponential and power functions.
Math.pow() in Java – Discover how to calculate the power of a number using Math.pow() in Java.
Finding Absolute Value in Java – Explore the concept of absolute value and its significance in mathematical calculations.
Oracle’s Official Java Documentation is the definitive source for information on the Math class and its methods.
GeeksforGeeks’ Java Math Class Section covers many methods of the Math class, including examples and explanations.
Baeldung’s Guide to Java Math provides a deeper dive into the Math class and some of its methods.
Wrapping Up: Mastering Math.max() in Java
In this comprehensive guide, we’ve delved into the world of Java’s Math.max() function, a simple yet powerful tool for finding the maximum of two numbers.
We began with the basics, exploring how to use Math.max() to find the maximum of two numbers. We then delved into more advanced usage, demonstrating how to use this function to find the maximum in an array or collection. Along the way, we tackled common issues that you might encounter when using Math.max(), such as handling NaN values and negative numbers, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to finding the maximum of two numbers, such as using the ternary operator and sorting an array. These alternatives offer different advantages and trade-offs, and can be more suitable in certain scenarios.
Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Efficiency | Flexibility |
---|---|---|---|
Math.max() | High | High | Low |
Ternary Operator | Moderate | High | Moderate |
Sorting an Array | Low | Moderate | High |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its built-in functions, we hope this guide has given you a thorough understanding of the Math.max() function and its applications.
With the knowledge and techniques shared in this guide, you’re now equipped to find the maximum of two numbers in Java efficiently and effectively. Happy coding!