Java’s Math.pow() Function | Guide to Exponents in Java
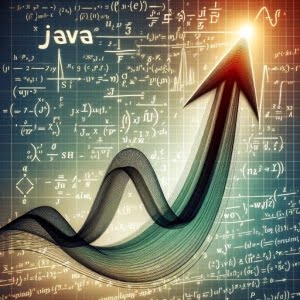
Are you finding it challenging to understand the Math.pow() function in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling mathematical operations in Java, but we’re here to help.
Think of Java’s Math.pow() function as a powerful calculator – allowing us to perform complex mathematical operations with ease, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using Math.pow() in Java, from its basic use to advanced techniques. We’ll cover everything from the basics of the Math.pow() function, its parameters, return type, to its usage in basic and complex mathematical operations.
Let’s get started and master the Math.pow() function in Java!
TL;DR: How Do I Use the Math.pow() Function in Java?
The
Math.pow()
function in Java is used to raise one number to the power of another. It takes two arguments: the base and the exponent, and returns the result as a double, with the syntaxdouble result = Math.pow(base, exponent);
. For instance,Math.pow(2, 3)
raises 2 to the power of 3.
Here’s a simple example:
double result = Math.pow(2, 3);
System.out.println(result);
// Output:
// 8.0
In this example, we use the Math.pow() function to raise 2 to the power of 3, which results in 8.0. The function is part of the Math class in Java, which provides a collection of methods that allows you to perform mathematical operations.
This is just a basic way to use the Math.pow() function in Java, but there’s much more to learn about its usage in different scenarios. Continue reading for a more detailed explanation and advanced usage of Math.pow().
Table of Contents
- Math.pow in Java: Basic Usage
- Expanding Horizons: Advanced Use of Math.pow()
- Exploring Alternatives: Other Ways to Perform Exponentiation in Java
- Troubleshooting Math.pow(): Common Issues and Solutions
- Exponentiation: The Power Behind Math.pow()
- The Role of the Math Class in Java
- Related Functions in the Math Class
- Applying Math.pow(): Real-World Scenarios
- Wrapping Up: Math.pow() in Java
Math.pow in Java: Basic Usage
The Math.pow()
function is a built-in method in the Math class in Java. It is used to perform the power operation, which is raising one number (the base) to the power of another (the exponent). This function takes two arguments and returns the result as a double
.
Understanding the Parameters
The Math.pow()
function accepts two parameters:
base
: This is the first argument, and it is the number that we want to raise to a power.exponent
: This is the second argument, and it is the power to which we want to raise the base.
Here’s a basic example of using the Math.pow()
function:
double base = 2;
double exponent = 3;
double result = Math.pow(base, exponent);
System.out.println(result);
// Output:
// 8.0
In this example, we’re raising 2 (the base) to the power of 3 (the exponent), which gives us the result 8.0.
Potential Pitfalls and Best Practices
While using the Math.pow()
function, it’s essential to keep in mind that both the base and the exponent can be any valid double
values, including negative numbers and zero. However, there are some scenarios where you need to be cautious:
- If the base is zero and the exponent is negative, the function will return
Infinity
, as you’re effectively trying to divide by zero. - If both the base and the exponent are zero, the function will return
1.0
, as any number (except zero) to the power of zero is one.
Always remember to handle these edge cases in your code to prevent unexpected results.
Expanding Horizons: Advanced Use of Math.pow()
As you become more comfortable with Math.pow()
, you’ll find that its applications extend far beyond raising positive integers to the power of another. Let’s explore some of these advanced uses.
Power Operations with Negative Numbers and Fractions
Math.pow()
can handle negative numbers and fractions as both the base and the exponent. Here’s an example of using Math.pow()
with a negative base and a fractional exponent:
double base = -2;
double exponent = 0.5;
double result = Math.pow(base, exponent);
System.out.println(result);
// Output:
// NaN
In this example, we’re trying to find the square root of -2 (since raising to the power of 0.5 is equivalent to taking the square root). However, the square root of a negative number is not a real number, so Math.pow()
returns NaN
, which stands for ‘Not a Number’.
Combining Math.pow() with Other Mathematical Functions
Math.pow()
can be used in conjunction with other mathematical functions to perform more complex calculations. For instance, you can use it with Math.sqrt()
to calculate the cube root of a number:
double base = 27;
double result = Math.pow(base, 1.0/3.0);
System.out.println(result);
// Output:
// 3.0
In this example, we’re calculating the cube root of 27. Since Java doesn’t have a built-in function for cube roots, we can calculate it by raising the number to the power of 1/3.
These examples show the flexibility and power of the Math.pow()
function. With a solid understanding of Math.pow()
, you can tackle a wide range of mathematical problems in Java.
Exploring Alternatives: Other Ways to Perform Exponentiation in Java
While Math.pow()
is a powerful tool for exponentiation, it’s not the only way to perform this operation in Java. Let’s explore some alternative approaches, such as using loops or the BigInteger class.
Exponentiation Using Loops
One straightforward method to calculate the power of a number is to use a loop. Here’s an example:
int base = 2;
int exponent = 3;
int result = 1;
for(int i = 0; i < exponent; i++) {
result *= base;
}
System.out.println(result);
// Output:
// 8
In this example, we’re raising 2 to the power of 3 using a simple for
loop. The loop iterates exponent
times, multiplying the result
by the base
each time. This method can be handy when dealing with integers, but it lacks the flexibility of Math.pow()
, which can handle any double
values.
Exponentiation Using the BigInteger Class
For very large numbers, even Math.pow()
might not be sufficient due to the limitations of the double
type. In these cases, the BigInteger class can come to the rescue. Here’s an example:
BigInteger base = new BigInteger("2");
BigInteger result = base.pow(100);
System.out.println(result);
// Output:
// 1267650600228229401496703205376
In this example, we’re raising 2 to the power of 100 using the pow()
method of the BigInteger class. This method can handle much larger numbers than Math.pow()
, but it only accepts integer exponents and can be slower for small numbers.
When choosing between these methods, consider the range and type of the numbers you’re working with, as well as the performance implications. Understanding these different approaches will enable you to choose the best tool for your specific needs.
Troubleshooting Math.pow(): Common Issues and Solutions
While Math.pow()
is a versatile tool, it’s not without its quirks. Let’s explore some common issues you might encounter when using this function and how to solve them.
Dealing with Very Large Numbers
Math.pow()
returns a double
, which can represent a wide range of values. However, it’s not infinite. If the result of Math.pow()
is too large to fit in a double
, it will return Infinity
:
double base = 2;
double exponent = 1024;
double result = Math.pow(base, exponent);
System.out.println(result);
// Output:
// Infinity
In this example, we’re trying to calculate 2 to the power of 1024, which is larger than the maximum value a double
can represent. As a result, Math.pow()
returns Infinity
.
Precision Issues
Another potential pitfall with Math.pow()
is precision. Due to the way floating-point numbers are represented, Math.pow()
might not always return the exact result you expect. For example:
double base = 2;
double exponent = 0.5;
double result = Math.pow(base, exponent);
System.out.println(result);
// Output:
// 1.4142135623730951
In this example, we’re calculating the square root of 2. The exact result is an irrational number that can’t be represented exactly as a double
, so Math.pow()
returns an approximation.
To handle these issues, consider using alternative methods for exponentiation when dealing with very large numbers or when precision is critical. Understanding these potential pitfalls can help you write more robust and accurate code.
Exponentiation: The Power Behind Math.pow()
Exponentiation is a fundamental mathematical operation. It involves taking one number (the base) and raising it to the power of another number (the exponent). In simple terms, it means multiplying the base by itself, the number of times indicated by the exponent.
double base = 2;
double exponent = 3;
double result = Math.pow(base, exponent);
System.out.println(result);
// Output:
// 8.0
In this example, we’re raising 2 (the base) to the power of 3 (the exponent), which gives us the result 8.0. This operation is equivalent to multiplying 2 by itself three times (2 * 2 * 2).
The Role of the Math Class in Java
In Java, the Math
class provides a set of static methods that can be used to perform mathematical operations. These include basic operations like addition, subtraction, multiplication, and division, as well as more complex operations like exponentiation, square root, and trigonometric functions.
The Math.pow()
function is one of these methods. It provides a quick and easy way to perform exponentiation in Java.
Related Functions in the Math Class
Besides Math.pow()
, the Math class in Java provides a host of other useful methods for mathematical operations. Some of these include:
Math.sqrt()
: This function returns the square root of a number.Math.abs()
: This function returns the absolute value of a number.Math.ceil()
: This function rounds a number up to the nearest integer.Math.floor()
: This function rounds a number down to the nearest integer.
Understanding these related functions can help you perform a wide range of mathematical operations in Java.
Applying Math.pow(): Real-World Scenarios
Understanding the Math.pow()
function is one thing, but applying it in real-world scenarios is where the true power of this function shines.
Algorithms and Scientific Computing
Math.pow()
can be instrumental in algorithms and scientific computing. For instance, it can be used in algorithms that require exponential calculations, such as calculating compound interest, or in scientific computing for calculations involving physics or engineering formulas.
Exploring Related Topics
Beyond Math.pow()
, Java offers a host of other features that can help you handle complex mathematical operations. These include support for big numbers using the BigInteger and BigDecimal classes, and precise control over floating point arithmetic.
These features can be incredibly useful in scenarios where you need to handle very large numbers, or when you need a high degree of precision in your calculations.
Further Resources for Mastering Java’s Math Class
To deepen your understanding of Math.pow()
and other features of Java’s Math class, you might find the following resources helpful:
- Understanding Java’s Math Class Functions – Dive into Java’s Math class to handle complex mathematical tasks efficiently.
Using Math.max() Method in Java – Explore the Math.max() method in Java for efficient comparison of numerical values.
Math.random() in Java – Learn about generating random numbers in Java using the Math.random() method.
Oracle’s Official Documentation on the Math Class – This is a comprehensive guide to all the methods in the Math class.
Java’s Math Class Explained by Baeldung – This article provides an in-depth look at Java’s math classes.
Math.pow() Method in Java by GeeksforGeeks explains how to calculate the power of a number via the Math.pow() method in Java.
Wrapping Up: Math.pow() in Java
In this comprehensive guide, we’ve delved deep into the world of Java’s Math.pow()
function. We’ve explored its usage, from basic to advanced, and discussed how it can be used to perform complex mathematical operations.
We began with the basics, understanding how Math.pow()
works and how to use it for simple power operations. We then ventured into more advanced territory, learning how to use Math.pow()
with negative numbers, fractions, and in conjunction with other mathematical functions.
We also tackled common issues you might encounter when using Math.pow()
, such as dealing with very large numbers and precision issues, and provided solutions to help you overcome these challenges. Additionally, we explored alternative approaches to exponentiation in Java, such as using loops or the BigInteger class.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Math.pow() | Versatile, supports any double values | May return Infinity for very large numbers, precision issues |
Using loops | Simple, good for integer power operations | Less flexible, can’t handle fractional exponents |
BigInteger.pow() | Can handle very large numbers | Only accepts integer exponents, slower for small numbers |
Whether you’re just starting out with Math.pow()
or you’re looking to level up your Java skills, we hope this guide has given you a deeper understanding of Math.pow()
and its capabilities.
With its versatility and power, Math.pow()
is a valuable tool for any Java developer. Keep exploring, keep learning, and happy coding!