Create Matplotlib Histograms in Python: Complete Guide
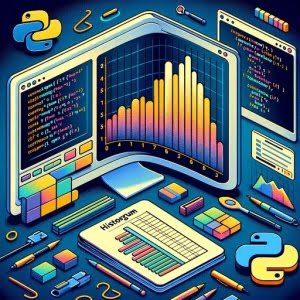
Are you finding it challenging to create histograms in Python using Matplotlib? You’re not alone. Many data analysts and developers grapple with this task, but with the right guidance, you can master it.
Like a skilled artist, you can use Matplotlib, a powerful data visualization library in Python, to paint a vivid picture of your data. These visualizations, particularly histograms, can provide significant insights into your data.
This guide will walk you through the process of creating histograms using Matplotlib in Python. We cover everything from the basics to more advanced techniques.
So, let’s dive in and start mastering Matplotlib histograms!
TL;DR: How Do I Create a Histogram Using Matplotlib in Python?
To create a histogram in Python using Matplotlib, you use the
hist()
function. This function takes in an array-like dataset and plots a histogram.
Here’s a simple example:
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3]
plt.hist(data)
plt.show()
# Output:
# A histogram plot with x-axis representing the data and y-axis representing the frequency.
In this example, we import the Matplotlib module and use the hist()
function to create a histogram. The data array is passed as an argument to the hist()
function. The show()
function is then used to display the histogram.
This is a basic way to create a histogram using Matplotlib in Python, but there’s much more to learn about creating and customizing histograms. Continue reading for a more detailed guide and advanced usage scenarios.
Table of Contents
- Crafting Histograms with Matplotlib: The Basics
- Digging Deeper: Advanced Histogram Customization with Matplotlib
- Exploring Alternatives: Histograms with Seaborn and Pandas
- Navigating Pitfalls: Troubleshooting Common Histogram Issues
- Understanding Histograms and Matplotlib
- The Power of Histograms: Beyond Basic Visualization
- Wrapping Up: Mastering Matplotlib Histograms in Python
Crafting Histograms with Matplotlib: The Basics
Let’s begin by understanding the hist()
function in Matplotlib, which is the cornerstone of creating histograms. The hist()
function takes in an array-like dataset and plots a histogram, which is a graphical representation of the distribution of the data.
Here’s how you can use the hist()
function to create a basic histogram:
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
plt.hist(data)
plt.show()
# Output:
# A histogram plot with x-axis representing the data and y-axis representing the frequency.
In the code block above, we first import the Matplotlib module. We then define an array of data. The hist()
function is called with the data array as an argument, which plots the histogram. The show()
function is used to display the histogram.
The resulting histogram provides a visual representation of the data distribution. Each bar in the histogram represents the frequency of data points in each range.
One of the primary advantages of using the hist()
function to create histograms is its simplicity and versatility. With just a few lines of code, you can create a meaningful visualization of your data. However, it’s important to understand that the hist()
function’s default settings may not always provide the most accurate or useful representation of your data. For instance, the default number of bins (the range of values that are grouped together) might not suit your specific dataset. In the next section, we’ll explore how to customize these parameters for more advanced histogram plotting.
Digging Deeper: Advanced Histogram Customization with Matplotlib
While the basic use of the hist()
function is straightforward, Matplotlib allows you to customize your histograms for more specific data visualization needs. Let’s dive into some of these parameters: ‘bins’, ‘range’, and ‘density’.
Understanding ‘bins’
The ‘bins’ parameter in the hist()
function determines the number of equal-width bins in the range. Let’s see how changing the ‘bins’ parameter affects the histogram.
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
plt.hist(data, bins=20)
plt.show()
# Output:
# A histogram plot with x-axis representing the data and y-axis representing the frequency. The number of bars is increased due to the increased number of bins.
In this example, we’ve set ‘bins’ to 20, which means the data range is divided into 20 equal intervals. Each bar in the histogram now represents the data frequency in each of these intervals.
Working with ‘range’
The ‘range’ parameter specifies the lower and upper range of the bins. Anything outside the range is ignored.
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
plt.hist(data, range=[2, 3])
plt.show()
# Output:
# A histogram plot with x-axis representing the data and y-axis representing the frequency. The plot only includes data within the specified range.
In this example, we’ve set the ‘range’ to [2, 3]. As a result, the histogram only includes the data points between 2 and 3.
Exploring ‘density’
The ‘density’ parameter, when set to True, normalizes the histogram such that the total area (or integral) under the histogram will sum to 1. This is useful when you want to visualize the probability distribution.
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
plt.hist(data, density=True)
plt.show()
# Output:
# A histogram plot with x-axis representing the data and y-axis representing the probability density. The total area under the histogram sums to 1.
In this example, by setting ‘density’ to True, the y-axis now represents the probability density of each bin in the histogram.
These are just a few examples of the parameters you can adjust when creating histograms with Matplotlib. By understanding and effectively using these parameters, you can create more insightful and tailored visualizations of your data.
Exploring Alternatives: Histograms with Seaborn and Pandas
While Matplotlib provides a robust platform for creating histograms, there are other libraries in Python that offer alternative methods. Let’s explore two popular ones: Seaborn and Pandas.
Seaborn: An Enhanced Visualization Library
Seaborn is a statistical plotting library built on top of Matplotlib. It provides a high-level interface for creating attractive graphics, including histograms.
import seaborn as sns
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
sns.histplot(data)
# Output:
# A histogram plot similar to Matplotlib but with a different style.
In this example, we use the histplot()
function from Seaborn to create a histogram. The output is similar to Matplotlib’s histogram, but it comes with a distinct Seaborn style.
Pandas: Data Manipulation and Analysis
Pandas is a powerful data manipulation library in Python. It also provides a function to create histograms from a DataFrame.
import pandas as pd
data = pd.DataFrame([1, 2, 2, 3, 3, 3, 4, 4, 4, 4], columns=['Values'])
data['Values'].plot(kind='hist')
# Output:
# A histogram plot similar to Matplotlib but created from a DataFrame.
In this example, we create a DataFrame from our data and use the plot()
function with ‘hist’ as the kind of plot we want to create.
Library | Advantages | Disadvantages |
---|---|---|
Matplotlib | Versatile, Customizable | More complex for advanced plots |
Seaborn | Attractive, Easy to use | Less customizable |
Pandas | Works well with DataFrame, Easy to use | Limited functionality |
While Matplotlib offers more customization options, Seaborn and Pandas can be easier to use and integrate better with their respective libraries. Your choice should depend on your specific needs and the complexity of your data.
While creating histograms with Matplotlib, Seaborn, or Pandas, you might encounter some common issues. Let’s discuss a few of these problems and how to solve them.
‘ValueError’
One common issue is the ‘ValueError’, which can occur when the input to the hist()
function is not valid.
import matplotlib.pyplot as plt
data = 'invalid input'
try:
plt.hist(data)
except ValueError as e:
print(f'Error: {e}')
# Output:
# Error: x must be 1D or 2D
In this example, we tried to pass a string as data to the hist()
function, which raised a ValueError. The error message indicates that the input data must be 1D or 2D. The solution here would be to ensure that the input data is a valid array-like object.
Problems with Binning
Another common issue relates to binning. If the ‘bins’ parameter is not set appropriately, the histogram may not accurately represent the data distribution.
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
plt.hist(data, bins=100)
plt.show()
# Output:
# A histogram plot with more bars than data points, making it difficult to interpret.
In this example, we set ‘bins’ to 100 for a small dataset. This resulted in a histogram with more bars than data points, making it difficult to interpret. A solution would be to choose an appropriate number of bins based on the size and distribution of the data.
These are just a few examples of the issues you might encounter while creating histograms. The key to effective troubleshooting is understanding the functions and parameters you’re working with and carefully inspecting error messages when they occur.
Understanding Histograms and Matplotlib
Before we delve deeper into creating histograms using Matplotlib, it’s important to understand the fundamental concepts underlying histograms and the Matplotlib library.
What is a Histogram?
A histogram is a graphical representation of the distribution of a dataset. It is an estimate of the probability distribution of a continuous variable. To construct a histogram, the first step is ‘binning’ the range of values—that is, dividing the entire range of values into a series of intervals—and then counting how many values fall into each interval. The bins are usually specified as consecutive, non-overlapping intervals of a variable.
What is Matplotlib?
Matplotlib is a plotting library for the Python programming language and its numerical mathematics extension, NumPy. It provides an object-oriented API for embedding plots into applications using general-purpose GUI toolkits like Tkinter, wxPython, Qt, or GTK.
Matplotlib is also a popular library for creating static, animated, and interactive visualizations in Python. Matplotlib can be used in Python scripts, the Python and IPython shell, web application servers, and various graphical user interface toolkits.
Key Concepts in Histograms
When dealing with histograms, there are a few key concepts to understand:
- Bins: These are the intervals that you divide your data into. The number of bins can greatly affect the resulting visualization and its interpretability.
Range: This is the extent of values that the histogram covers. The range of a histogram can be specified, and values outside this range are usually ignored.
Density: In a histogram, if ‘density’ is set to True, the sum of the histogram values will not be equal to 1. If ‘density’ is set to False, the total area under the histogram (calculated via integration) will be equal to 1.
Understanding these concepts will help you better understand the process and customization of creating histograms with Matplotlib.
The Power of Histograms: Beyond Basic Visualization
Histograms, while a simple concept, play a vital role in various fields. They are especially relevant in data analysis and machine learning applications, where understanding data distribution is crucial.
Histograms in Data Analysis
In data analysis, histograms are used to visualize and understand the underlying distribution of data. They give a clear picture of the central tendency, dispersion, and skewness of the data. Understanding the distribution of data is essential in making decisions based on the data.
Histograms in Machine Learning
In machine learning, histograms are often used in exploratory data analysis to understand the distribution of inputs and outputs. They can be particularly useful in identifying outliers and understanding whether data transformation is necessary for algorithms that require normally distributed data.
Exploring Related Concepts
While histograms are a powerful tool, they are just one part of a larger ecosystem of data visualization and statistical analysis techniques. Other related concepts worth exploring include scatter plots, box plots, and bar charts for data visualization, and mean, median, mode, standard deviation for statistical analysis.
Further Resources for Matplotlib Histogram Mastery
To deepen your understanding of histograms and Matplotlib, here are a few resources to get you started:
- Matplotlib Tutorial: Getting Started – Explore bar charts, histograms, and pie charts in Python.
Scatter Plots in Matplotlib with plt.scatter: A Tutorial on how to create Python scatter plots with Matplotlib’s “plt.scatter”.
Python Histograms: Analyzing Data Distribution – Explore Python’s histogram plotting capabilities for data analysis.
Matplotlib: Visualization with Python – The official Matplotlib documentation that covers all aspects of the library.
Python Data Science Handbook by Jake VanderPlas is an excellent resource for anyone working with data in Python.
Intro to Data Visualization with Matplotlib – This course from DataCamp provides a hands-on introduction to Matplotlib.
Wrapping Up: Mastering Matplotlib Histograms in Python
In this comprehensive guide, we’ve journeyed through the process of creating histograms using Matplotlib in Python.
We began with the basics, learning how to create a simple histogram using the hist()
function in Matplotlib. We then ventured into more advanced territory, exploring the different parameters of the hist()
function such as ‘bins’, ‘range’, and ‘density’, and how they can be used to customize histograms for specific data visualization needs.
Along the way, we tackled common challenges you might face when creating histograms, such as ‘ValueError’ and problems with binning, and provided solutions to help you overcome these hurdles.
We also looked at alternative approaches to creating histograms, comparing Matplotlib with other libraries like Seaborn and Pandas. Here’s a quick comparison of these methods:
Library | Versatility | Ease of Use |
---|---|---|
Matplotlib | High | Moderate |
Seaborn | Moderate | High |
Pandas | Moderate | High |
Whether you’re a beginner just starting out with Matplotlib or an experienced Python developer looking to level up your data visualization skills, we hope this guide has given you a deeper understanding of how to create histograms with Matplotlib and its alternatives.
Keep exploring, keep coding!