Python Histograms Guide: Syntax, Usage, Examples
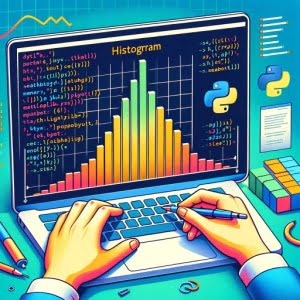
Are you finding it challenging to create histograms in Python? You’re not alone. Many developers find themselves puzzled when it comes to creating histograms in Python. Think of Python as your personal data artist, capable of painting detailed histograms with just a few lines of code.
Whether you’re dealing with data analysis, data visualization, or simply trying to understand your data better, understanding how to create histograms in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of creating histograms in Python, from the basics to more advanced techniques. We’ll cover everything from the hist()
function, using different bins and ranges, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Create a Histogram in Python?
You can create a histogram in Python using the
hist()
function from the matplotlib library. This function allows you to visualize data distribution in a convenient and straightforward way.
Here’s a simple example:
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3]
plt.hist(data)
plt.show()
# Output:
# A histogram with the data values on the x-axis and their frequencies on the y-axis.
In this example, we first import the matplotlib library, which provides the hist()
function. We then define a list of data values and pass it to the hist()
function. The show()
function is used to display the histogram. The resulting histogram shows the frequency of each data value in the list.
This is a basic way to create a histogram in Python, but there’s much more to learn about creating and manipulating histograms. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the hist() Function: The Basics
- Mastering Histograms: Different Bins and Ranges
- Exploring Alternative Histogram Libraries: Seaborn
- Troubleshooting Histograms: Common Issues and Solutions
- Understanding Histograms: The Fundamentals
- Expanding Your Python Visualization Toolkit
- Wrapping Up: Mastering Histogram Creation in Python
Understanding the hist()
Function: The Basics
Python’s hist()
function, part of the matplotlib library, is a powerful tool for creating histograms. Histograms are graphical representations of data distribution over a set of intervals or bins. They provide a visual interpretation of numerical data by indicating the number of data points that lie within a range of values, known as ‘bins’.
Let’s start by understanding how to use the hist()
function to create a basic histogram.
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3]
plt.hist(data)
plt.show()
# Output:
# A histogram with the data values on the x-axis and their frequencies on the y-axis.
In the above code, we first import the matplotlib.pyplot library and alias it as plt. We then create a list of data values which we pass to the hist()
function. The show()
function is used to display the histogram.
The hist()
function automatically calculates the size of the bins based on the range of data, and the number of bins is by default set to 10. The function then counts how many data points fall into each bin and plots the bins along with their frequencies.
While the hist()
function is a powerful tool, it’s important to remember that the visualization may vary depending on the bin size. If the bins are too large, important details can be missed. On the other hand, if the bins are too small, the histogram can become cluttered and difficult to interpret.
In the next section, we’ll delve into more advanced usage scenarios and learn how to manipulate bins and ranges for more complex histograms.
Mastering Histograms: Different Bins and Ranges
As you become more comfortable with creating basic histograms, you might find yourself needing more control over the visualization. This is where manipulating bins and ranges come into play.
The hist()
function allows you to specify the number of bins and the range of data to be included in the histogram. By adjusting these parameters, you can create more detailed and informative histograms.
Let’s take a look at an example:
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5]
plt.hist(data, bins=5, range=[1,5])
plt.show()
# Output:
# A histogram with the data values on the x-axis and their frequencies on the y-axis. The x-axis is divided into five equal bins from 1 to 5.
In this example, we’ve specified bins=5
and range=[1,5]
. This means that the hist()
function will divide the data into five equal intervals from 1 to 5 and calculate the frequency of data points in each bin. The resulting histogram provides a more detailed view of the data distribution.
Manipulating bins and ranges allows you to customize your histograms and make them more informative. However, it’s important to choose these parameters carefully. Too many bins can make the histogram cluttered and difficult to interpret, while too few can oversimplify the data and miss important details.
In the next section, we’ll explore alternative approaches to creating histograms in Python.
Exploring Alternative Histogram Libraries: Seaborn
While matplotlib’s hist()
function is a powerful tool for creating histograms, Python offers other libraries that can provide more advanced features and styles. One such library is Seaborn, a statistical plotting library built on top of matplotlib.
Seaborn provides a high-level interface for drawing attractive and informative statistical graphics. Its distplot()
function can create histograms and KDE plots together for more detailed data distribution analysis.
Let’s take a look at an example:
import seaborn as sns
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5]
sns.distplot(data, bins=5, kde=True)
# Output:
# A histogram with a KDE plot overlay.
In this example, we use Seaborn’s distplot()
function to create a histogram with a KDE (Kernel Density Estimation) plot overlay. The kde=True
parameter enables the KDE plot, providing a smooth curve that estimates the probability density function of the data.
Seaborn’s histograms offer a more advanced and visually appealing alternative to matplotlib’s hist()
function. However, they can be more complex to configure and may not be necessary for simple data analysis tasks.
Here’s a comparison of the two methods:
Method | Advantages | Disadvantages |
---|---|---|
Matplotlib hist() | Simple to use, Flexible bin sizes and ranges | Basic visual style, Manual configuration required for advanced features |
Seaborn distplot() | Advanced features like KDE, Attractive visual style | More complex to configure, May be overkill for simple tasks |
Choosing the right method for creating histograms in Python depends on your specific needs and level of comfort with the libraries. Both matplotlib and Seaborn offer powerful tools for data visualization and can help you create detailed and informative histograms.
In the next section, we’ll discuss common issues you might encounter when creating histograms and how to troubleshoot them.
Troubleshooting Histograms: Common Issues and Solutions
Creating histograms in Python is generally a straightforward process, but like any coding task, it can come with its own set of challenges. Here, we’ll discuss some common issues you might encounter when creating histograms, and provide solutions and workarounds.
Dealing with Missing Data
One common issue when creating histograms is dealing with missing data. If your data set contains null or NaN values, the hist()
function might throw an error.
To handle this, you can use the dropna()
function from pandas to remove these values before passing the data to hist()
.
Here’s an example:
import matplotlib.pyplot as plt
import pandas as pd
data = pd.Series([1, 2, 2, 3, 3, 3, None, 4, 4, 4, 4, 5, 5, 5, 5, 5])
data = data.dropna()
plt.hist(data)
plt.show()
# Output:
# A histogram of the data, excluding the null value.
In this example, we first create a pandas Series with some data, including a null value. We then use dropna()
to remove the null value before passing the data to hist()
. The resulting histogram excludes the null value.
Handling Outliers
Another common issue when creating histograms is dealing with outliers. Outliers can distort a histogram and make it difficult to interpret the data.
To handle outliers, you can use techniques such as the IQR method to identify and remove them before creating the histogram. Alternatively, you can use the range
parameter in the hist()
function to limit the data included in the histogram.
Creating histograms in Python is a powerful way to visualize and understand your data. By being aware of these common issues and knowing how to troubleshoot them, you can create more accurate and informative histograms.
Understanding Histograms: The Fundamentals
Before we dive deeper into creating histograms in Python, it’s important to understand what a histogram is and why it is a powerful tool for data analysis.
A histogram is a graphical representation of data distribution over a set of intervals, or ‘bins’. It provides a visual interpretation of numerical data by indicating the number of data points that fall within a range of values.
Histograms are widely used in statistics and data analysis to understand the underlying distribution of data. They can help identify patterns, trends, and outliers, providing valuable insights for decision-making.
Here’s a simple example of how a histogram works:
import matplotlib.pyplot as plt
data = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5]
plt.hist(data)
plt.show()
# Output:
# A histogram with the data values on the x-axis and their frequencies on the y-axis.
In this example, the hist()
function divides the data into bins and counts the number of data points in each bin. The resulting histogram shows the frequency of each data value, providing a visual representation of the data distribution.
Understanding the fundamentals of histograms is crucial for effective data analysis. With this knowledge, you can use Python’s powerful libraries to create detailed and informative histograms, gaining deeper insights into your data.
Expanding Your Python Visualization Toolkit
Histograms are an essential part of data analysis and visualization. They provide a quick and easy way to understand the distribution of data. But Python’s data visualization capabilities extend far beyond histograms.
Python offers a rich ecosystem of libraries for creating a wide variety of plots, such as scatter plots, box plots, and heatmaps. Libraries like matplotlib, seaborn, and plotly provide powerful tools for creating these plots and many more.
By exploring these libraries and the different types of plots they offer, you can gain a deeper understanding of your data and extract more meaningful insights.
Diving Deeper into Data Visualization and Analysis
If you’re interested in diving deeper into data visualization and analysis in Python, there are plenty of resources available. Here are a few recommended ones:
- Matplotlib Efficiency Tips – Discover real-world examples and use cases of Matplotlib.
Python Data Analysis: Matplotlib Histograms – Discover techniques for customizing and enhancing Matplotlib histograms.
Plotting Data with plt.plot() in Python – Learn how to visualize data trends and patterns using “plt.plot.”
Python Graph Gallery – A comprehensive collection of Python visualization methods and examples.
Data Visualization: Python and Seaborn – A multi-part series on creating detailed, informative plots using Python and Seaborn.
Python for Data Analysis – A book by Wes McKinney, the creator of pandas, covering all aspects of data analysis in Python.
Wrapping Up: Mastering Histogram Creation in Python
In this comprehensive guide, we’ve navigated through the process of creating histograms in Python. We’ve covered everything from the basic usage of the hist()
function in matplotlib to more advanced techniques, such as manipulating bins and ranges.
We began with the basics, explaining how the hist()
function works with data to create histograms. We then progressed to more advanced usage, discussing how to deal with different types of data and create more complex histograms. We also introduced alternative approaches, such as using the seaborn library for more advanced features and styles.
Along the way, we addressed common issues that you might encounter when creating histograms, such as dealing with missing data or outliers, and provided solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
Matplotlib hist() | Simple to use, Flexible bin sizes and ranges | Basic visual style, Manual configuration required for advanced features |
Seaborn distplot() | Advanced features like KDE, Attractive visual style | More complex to configure, May be overkill for simple tasks |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your data visualization skills, we hope this guide has given you a deeper understanding of creating histograms in Python.
With this knowledge, you can more effectively analyze and understand your data. Happy coding!