plt.plot() Matplotlib Python Function Guide
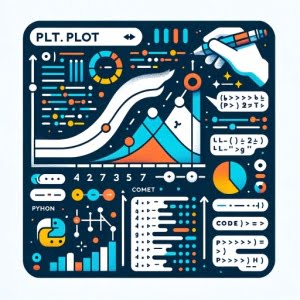
Are you finding it challenging to create plots in Python? You’re not alone. Many developers find themselves puzzled when it comes to creating visualizations. But, think of plt.plot as a Swiss army knife – versatile and handy for various tasks.
Whether you’re generating dynamic graphs, formatting data for output, or even debugging, understanding how to use plt.plot in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using plt.plot in Python, from the basics to more advanced techniques. We’ll cover everything from creating simple line plots to more complex visualizations, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use plt.plot in Python?
To create a basic line plot in Python, you can use the plt.plot function from the matplotlib library. This function allows you to visualize your data in a simple and intuitive way.
Here’s a simple example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.ylabel('some numbers')
plt.show()
# Output:
# A line plot with the y-axis labeled 'some numbers'
In this example, we import the matplotlib.pyplot module and use the plt.plot function to create a line plot. The list [1, 2, 3, 4] represents the y-values, and since we didn’t provide any x-values, Python uses the indices of the list elements as x-values. The plt.ylabel function labels the y-axis, and plt.show displays the plot.
This is a basic way to use plt.plot in Python, but there’s much more to learn about creating and customizing plots. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- plt.plot Basics: Creating Simple Line Plots
- Advanced plt.plot Techniques: Beyond Basic Line Plots
- Exploring Alternatives to plt.plot
- Troubleshooting plt.plot: Common Issues and Solutions
- Best Practices and Optimization
- Understanding matplotlib and plt.plot
- The Power of Data Visualization
- plt.plot Applications: Real-World Scenarios
- Further Resources for Mastering plt.plot
- Wrapping Up: Mastering plt.plot in Python
plt.plot Basics: Creating Simple Line Plots
In Python, plt.plot is one of the most commonly used functions to create simple line plots. It’s straightforward, easy to use, and can be highly customized to meet your needs. Here, we’ll go through a basic example and explain how the function works.
Let’s start with a simple line plot:
import matplotlib.pyplot as plt
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16, 25]
plt.plot(x_values, y_values)
plt.show()
# Output:
# A line plot with x-values [1, 2, 3, 4, 5] and y-values [1, 4, 9, 16, 25]
In the above code, we first import the matplotlib.pyplot module. We then define two lists, x_values and y_values. These lists represent the x and y coordinates of our plot respectively. We pass these lists to the plt.plot function, which plots these points and connects them with a line. Finally, plt.show() is used to display our plot.
The plt.plot function is versatile and easy to use, making it a great choice for beginners. However, it’s important to remember that the x_values and y_values must be the same length. If they aren’t, Python will throw an error.
Understanding the plt.plot Syntax
The plt.plot function has a simple syntax:
plt.plot(x, y)
Where x
and y
are lists or arrays containing the x and y coordinates of the points you want to plot. If you only provide one list or array, plt.plot assumes it’s the y-values and automatically generates the x-values for you.
Understanding the plt.plot function and its syntax is the first step towards mastering data visualization in Python. In the next section, we’ll dive into more advanced uses of plt.plot.
Advanced plt.plot Techniques: Beyond Basic Line Plots
While plt.plot is commonly used for creating line plots, it’s capable of much more. Let’s explore some of the more advanced uses of plt.plot, including creating scatter plots, bar plots, and customizing plot styles.
Creating Scatter Plots with plt.plot
Scatter plots are a great way to visualize the relationship between two variables. Here’s how you can create a scatter plot using plt.plot:
import matplotlib.pyplot as plt
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16, 25]
plt.plot(x_values, y_values, 'o')
plt.show()
# Output:
# A scatter plot with x-values [1, 2, 3, 4, 5] and y-values [1, 4, 9, 16, 25]
In this code, we added a third argument ‘o’ to the plt.plot function. This argument specifies the format of the plot. The ‘o’ indicates that we want a scatter plot.
Creating Bar Plots with plt.plot
Bar plots are useful for comparing quantities across different categories. Here’s how to create a bar plot:
import matplotlib.pyplot as plt
categories = ['Category 1', 'Category 2', 'Category 3']
values = [10, 15, 7]
plt.bar(categories, values)
plt.show()
# Output:
# A bar plot with categories ['Category 1', 'Category 2', 'Category 3'] and values [10, 15, 7]
In this example, we used plt.bar instead of plt.plot. The plt.bar function takes two arguments: the categories and their corresponding values.
Customizing Plot Styles
plt.plot allows for extensive customization. You can change the line style, color, marker style, and more. Here’s an example:
import matplotlib.pyplot as plt
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16, 25]
plt.plot(x_values, y_values, color='red', linestyle='--', marker='o')
plt.show()
# Output:
# A red dashed line plot with circle markers
In this code, we added color, linestyle, and marker arguments to plt.plot. The color argument changes the color of the line, the linestyle changes the style of the line, and the marker changes the style of the markers.
These advanced techniques can help you create more complex and informative visualizations with plt.plot.
Exploring Alternatives to plt.plot
While plt.plot from matplotlib is a powerful tool for creating visualizations in Python, it’s not the only option available. Other libraries like seaborn, ggplot, and plotly offer additional features and different approaches that might be more suited to your specific needs. Let’s take a look at these alternatives.
Seaborn: Statistical Data Visualization
Seaborn is a Python data visualization library based on matplotlib that provides a high-level interface for creating attractive and informative statistical graphics. It’s particularly well suited for visualizing complex datasets.
Here’s an example of how to create a scatter plot in seaborn:
import seaborn as sns
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16, 25]
sns.scatterplot(x=x_values, y=y_values)
# Output:
# A scatter plot similar to the one created with plt.plot
ggplot: Grammar of Graphics
ggplot is another popular Python data visualization library. It’s based on the Grammar of Graphics, a system for understanding the building blocks of a graph and how they fit together.
Here’s an example of creating a scatter plot in ggplot:
from ggplot import *
ggplot(aes(x='x_values', y='y_values'), data=df) + geom_point()
# Output:
# A scatter plot similar to the ones created with plt.plot and seaborn
Plotly: Interactive Graphing
Plotly is a graphing library that makes interactive, publication-quality graphs. It’s great for making interactive plots that can be embedded in websites.
Here’s an example of creating a scatter plot in Plotly:
import plotly.express as px
fig = px.scatter(x=x_values, y=y_values)
fig.show()
# Output:
# An interactive scatter plot
All three of these libraries offer unique advantages over matplotlib and plt.plot. Seaborn provides a high-level interface for creating attractive statistical graphics, ggplot offers a comprehensive system for understanding the building blocks of a graph, and Plotly excels at creating interactive, publication-quality graphs.
However, they also have their own learning curves and may not be as straightforward to use as plt.plot for beginners. Therefore, the choice between these libraries and plt.plot will depend on your specific needs and preferences.
Troubleshooting plt.plot: Common Issues and Solutions
Like any other tool, plt.plot can sometimes throw up challenges and errors. Here, we’ll look at some common issues you might encounter while using plt.plot and offer solutions to overcome them.
Mismatched Data Length
One common error occurs when the lengths of x and y data do not match. Let’s see this in action:
import matplotlib.pyplot as plt
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16]
plt.plot(x_values, y_values)
plt.show()
# Output:
# ValueError: x and y must have same first dimension
As you can see, Python throws a ValueError because the lengths of x_values and y_values do not match. To solve this, you need to ensure that your x and y data have the same length.
Invalid Format String
Another common error is using an invalid format string. Here’s an example:
import matplotlib.pyplot as plt
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16, 25]
plt.plot(x_values, y_values, 'invalid')
plt.show()
# Output:
# ValueError: Unrecognized character p in format string
In this case, Python throws a ValueError because ‘invalid’ is not a recognized format string. To solve this, you need to use a valid format string. You can find a list of valid format strings in the matplotlib documentation.
Best Practices and Optimization
When using plt.plot, here are a few tips to keep in mind for optimal performance:
- Always label your axes using plt.xlabel and plt.ylabel. This makes your plots easier to understand.
- If you’re creating multiple plots, use plt.figure to create a new figure before each plot. This ensures that each plot is displayed on a separate figure.
- Use plt.grid(True) to display a grid on your plots. This can make your plots easier to read.
By understanding the common issues and best practices associated with plt.plot, you can create high-quality plots more efficiently and effectively.
Understanding matplotlib and plt.plot
Before we delve deeper into plt.plot, it’s crucial to understand its origin – the matplotlib library. matplotlib is a comprehensive library for creating static, animated, and interactive visualizations in Python. It’s widely used in the data science community for its flexibility and control over every aspect of a figure.
plt.plot is a function in matplotlib’s pyplot submodule, often imported under the alias plt. This function generates a two-dimensional plot, which is a key element in visualizing numerical data in Python.
import matplotlib.pyplot as plt
# Sample data
x_values = [1, 2, 3, 4, 5]
y_values = [1, 4, 9, 16, 25]
# Create a line plot
plt.plot(x_values, y_values)
# Display the plot
plt.show()
# Output:
# A line plot with x-values [1, 2, 3, 4, 5] and y-values [1, 4, 9, 16, 25]
In this code block, we import the pyplot module from matplotlib as plt. Next, we define our data as two lists: x_values and y_values. We then use plt.plot to create a line plot of this data. Finally, plt.show displays the plot. The output is a line plot with x-values [1, 2, 3, 4, 5] and y-values [1, 4, 9, 16, 25].
The Power of Data Visualization
Data visualization is a fundamental aspect of data analysis. It enables us to understand complex data sets by representing them in a visual, easy-to-understand format. With plt.plot and matplotlib, you can create a wide range of plots and charts, enabling you to visualize data in a way that makes sense for your specific needs.
Whether you’re looking to identify trends and patterns, communicate results effectively, or make data-driven decisions, understanding how to use plt.plot in Python is a valuable skill in your data analysis toolkit.
plt.plot Applications: Real-World Scenarios
plt.plot is not just a tool for creating plots; it’s a gateway to understanding your data and making it understandable to others. It’s widely used in various real-world scenarios, particularly in data analysis and machine learning.
Data Analysis with plt.plot
In data analysis, plt.plot can help you understand trends, patterns, and outliers in your data. For example, you might use plt.plot to visualize sales data over time, helping you identify periods of high and low sales.
import matplotlib.pyplot as plt
import numpy as np
# Simulate sales data
np.random.seed(0)
days = list(range(1, 31))
sales = np.random.randint(1, 100, size=(30,))
# Plot sales data
plt.plot(days, sales)
plt.xlabel('Day')
plt.ylabel('Sales')
plt.title('Sales over Time')
plt.show()
# Output:
# A line plot showing sales data over 30 days
In this example, we simulated sales data over a 30-day period using numpy’s random number generator. We then used plt.plot to visualize this data, helping us see how sales fluctuated over time.
Machine Learning with plt.plot
In machine learning, plt.plot can help you visualize your model’s performance, such as plotting a model’s learning curve or visualizing the decision boundaries of a classification model.
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import plot_confusion_matrix
import matplotlib.pyplot as plt
# Load iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=0)
# Fit KNN classifier
knn = KNeighborsClassifier()
knn.fit(X_train, y_train)
# Plot confusion matrix
plot_confusion_matrix(knn, X_test, y_test, cmap=plt.cm.Blues)
plt.show()
# Output:
# A confusion matrix showing the performance of the KNN classifier
In this example, we trained a K-Nearest Neighbors (KNN) classifier on the iris dataset and used plot_confusion_matrix, which utilizes plt.plot under the hood, to visualize the classifier’s performance.
Further Resources for Mastering plt.plot
To continue your journey in mastering plt.plot and data visualization in Python, here are some additional resources:
- IOFlood’s Matplotlib Guide dives deep into Matplotlib’s integration with Jupyter notebooks.
Visualizing Data Distributions with Python – Learn how to visualize data distributions effectively using Python.
Python Data Visualization: Exploring Python Visualizers – Explore Python’s visualization tools and libraries for data exploration.
Python Graph Gallery – This website offers a wide range of examples and tutorials on creating plots in Python.
matplotlib Official Documentation is a comprehensive resource that covers all aspects of the library.
DataCamp’s Tutorial on Matplotlib provides clear explanations and multiple examples of different plot types with matplotlib.
Wrapping Up: Mastering plt.plot in Python
In this comprehensive guide, we’ve journeyed through the versatile world of plt.plot in Python. This function from the matplotlib library is a powerful tool for creating a wide range of plots and visualizations, which are essential for data analysis and machine learning.
We started with the basics, learning how to create simple line plots using plt.plot. We then explored more advanced uses, such as creating scatter plots, bar plots, and customizing plot styles. We also tackled common issues that you might encounter when using plt.plot, such as mismatched data length and invalid format strings, providing you with solutions to overcome these challenges.
We broadened our horizons by looking at alternative plotting libraries in Python, such as seaborn, ggplot, and plotly. Each of these libraries offers unique advantages and different approaches to data visualization.
Here’s a quick comparison of these libraries:
Library | Versatility | Ease of Use | Interactivity |
---|---|---|---|
matplotlib (plt.plot) | High | High | Low |
seaborn | High | Moderate | Low |
ggplot | Moderate | Moderate | Low |
plotly | High | High | High |
Whether you’re a novice just starting out with plt.plot or an experienced data scientist looking to level up your data visualization skills, we hope this guide has given you a deeper understanding of plt.plot and its capabilities.
With its balance of versatility, ease of use, and wide range of applications, plt.plot is a powerful tool for data visualization in Python. Happy coding!