Mastering Java: How to Initialize a HashMap
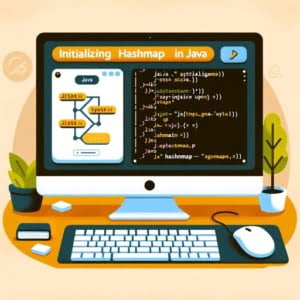
Are you finding it challenging to initialize a HashMap in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling HashMaps in Java, but we’re here to help.
Think of a HashMap in Java as a dictionary – a tool that allows us to store and retrieve values using unique keys. It’s a fundamental part of Java’s collection framework, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of initializing a HashMap in Java, from the basics to more advanced techniques. We’ll cover everything from creating a simple HashMap, manipulating its elements, to more advanced usage scenarios, as well as alternative approaches.
Let’s dive in and start mastering HashMap in Java!
TL;DR: How Do I Initialize a HashMap in Java?
To initialize a HashMap in Java, you can use the
new
keyword before instantiating the HashMap,map = new HashMap<>();
. Here’s a simple example:
HashMap<Boolean, Integer> map = new HashMap<>();
In this example, we’ve created an empty HashMap named map
where the keys are Strings and the values are Integers. This is the most basic way to initialize a HashMap in Java.
But Java’s HashMap capabilities go far beyond this. Continue reading for more detailed examples, advanced usage scenarios, and alternative approaches.
Table of Contents
- Initializing and Using a HashMap: The Basics
- Exploring Advanced HashMap Initialization
- Exploring Alternatives: TreeMap and LinkedHashMap
- Troubleshooting HashMap Initialization and Usage
- Understanding the Fundamentals of HashMaps
- Applying HashMaps in Real-World Java Projects
- Further Resources for Mastering HashMaps in Java
- Wrapping Up: HashMap Initialization in Java
Initializing and Using a HashMap: The Basics
Let’s start with the basics of initializing a HashMap in Java. It’s a simple process that involves the new
keyword. Here’s a simple example:
HashMap<String, Integer> map = new HashMap<>();
In this example, we’ve created an empty HashMap named map
, where the keys are Strings and the values are Integers. The “ is a diamond operator introduced in Java 7 to simplify the creation of instance of generic type.
Adding Elements to the HashMap
Now that we have our HashMap, we can start adding elements to it. Here’s how you can add elements:
map.put("Alice", 25);
map.put("Bob", 30);
Here, we’ve added two elements to our HashMap. The put()
method is used to add elements to the HashMap. The first parameter is the key, and the second is the value.
Retrieving Elements from the HashMap
To retrieve an element from the HashMap, we use the get()
method and pass the key of the element we want to retrieve. Here’s how you can do it:
int aliceAge = map.get("Alice");
System.out.println("Alice's age: " + aliceAge);
# Output:
# Alice's age: 25
In this example, we’re retrieving the age of Alice using the key “Alice” and printing it.
The Importance of Unique Keys
In a HashMap, keys are unique. This means that if you try to insert a value with a key that already exists in the HashMap, the old value will be replaced with the new one. This is crucial to understand when working with HashMaps to avoid unexpected data loss.
map.put("Alice", 26);
int newAliceAge = map.get("Alice");
System.out.println("Alice's new age: " + newAliceAge);
# Output:
# Alice's new age: 26
In this example, we updated Alice’s age by using the same key. When we retrieve Alice’s age again, we see that it’s been updated to the new value.
Exploring Advanced HashMap Initialization
Different Types of Keys and Values
HashMaps in Java are not limited to just Strings and Integers. You can use any object as a key or value. Let’s see an example where we use an object as a key:
class Key {
String key;
public Key(String key) {
this.key = key;
}
}
HashMap<Key, Integer> map = new HashMap<>();
Key key1 = new Key("Key1");
map.put(key1, 100);
System.out.println("Value of key1: " + map.get(key1));
# Output:
# Value of key1: 100
In this example, we’ve created a new class Key
and used it as the key in our HashMap. The HashMap now takes a Key
object and an Integer as a key-value pair.
Initializing HashMap with Default Values
Sometimes, you might want to initialize a HashMap with some default values. Java provides several ways to do this. One way is to use the put()
method multiple times. Another way is to use the Map.ofEntries()
static method. Let’s see how to do this:
HashMap<String, Integer> map = new HashMap<>(Map.ofEntries(
entry("Alice", 25),
entry("Bob", 30),
entry("Charlie", 35)
));
System.out.println("Alice's age: " + map.get("Alice"));
System.out.println("Bob's age: " + map.get("Bob"));
System.out.println("Charlie's age: " + map.get("Charlie"));
# Output:
# Alice's age: 25
# Bob's age: 30
# Charlie's age: 35
In this example, we’ve initialized a HashMap with three entries. The Map.ofEntries()
method takes a list of entries and returns a Map containing these entries. We’ve then passed this Map to the HashMap constructor to create our HashMap.
Exploring Alternatives: TreeMap and LinkedHashMap
While HashMaps are incredibly useful, Java provides other data structures that might be more suitable depending on your needs. Two of these are TreeMap and LinkedHashMap.
TreeMap: Sorted Entries
A TreeMap in Java is a Map implementation that keeps its entries sorted according to the natural ordering of its keys or by a comparator provided at the time of TreeMap creation.
TreeMap<String, Integer> treeMap = new TreeMap<>();
treeMap.put("Alice", 25);
treeMap.put("Bob", 30);
treeMap.put("Charlie", 35);
treeMap.forEach((key, value) -> System.out.println(key + ": " + value));
# Output:
# Alice: 25
# Bob: 30
# Charlie: 35
In this example, we’ve created a TreeMap and added some entries. When we print the entries, we see that they are sorted by the key.
LinkedHashMap: Preserving Insertion Order
A LinkedHashMap in Java is another Map implementation that keeps its entries in the order they were inserted. This can be useful when the insertion order matters.
LinkedHashMap<String, Integer> linkedHashMap = new LinkedHashMap<>();
linkedHashMap.put("Alice", 25);
linkedHashMap.put("Bob", 30);
linkedHashMap.put("Charlie", 35);
linkedHashMap.forEach((key, value) -> System.out.println(key + ": " + value));
# Output:
# Alice: 25
# Bob: 30
# Charlie: 35
In this example, we’ve created a LinkedHashMap and added some entries. When we print the entries, we see that they are in the order they were inserted.
When to Use These Alternatives
Choosing between HashMap, TreeMap, and LinkedHashMap depends on your requirements. If you need your entries sorted, use a TreeMap. If you need to preserve the insertion order, use a LinkedHashMap. If you don’t need either of these, a HashMap will be more efficient.
Troubleshooting HashMap Initialization and Usage
While initializing and using HashMaps in Java is generally straightforward, you might encounter some common errors or obstacles. Let’s discuss these issues and their solutions.
Null Keys and Values
One of the first things to note is that HashMaps in Java allow null keys and null values. However, using null keys can lead to unexpected behavior and should be avoided when possible.
HashMap<String, Integer> map = new HashMap<>();
map.put(null, 25);
System.out.println("Null key's value: " + map.get(null));
# Output:
# Null key's value: 25
In this example, we’ve used null as a key. While this is allowed, it can lead to confusion and should be avoided.
Handling Absent Keys
When you try to retrieve a value using a key that doesn’t exist in the HashMap, the get()
method returns null. This can lead to a NullPointerException if not handled correctly.
Integer value = map.get("NonexistentKey");
System.out.println(value.toString());
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’re trying to retrieve a value using a key that doesn’t exist in the HashMap. The get()
method returns null, and when we try to call toString()
on null, a NullPointerException is thrown.
To avoid this, you should always check if a value is null before using it.
Integer value = map.get("NonexistentKey");
if (value != null) {
System.out.println(value.toString());
} else {
System.out.println("Key not found.");
}
# Output:
# Key not found.
HashMap Capacity and Load Factor
When initializing a HashMap, you can specify its initial capacity and load factor. The capacity is the number of buckets in the HashMap, and the load factor is a measure of how full the HashMap is allowed to get before its capacity is automatically increased. Understanding these concepts can help you optimize your HashMap usage.
HashMap<String, Integer> map = new HashMap<>(16, 0.75f);
In this example, we’re creating a HashMap with an initial capacity of 16 and a load factor of 0.75. This means that the HashMap will automatically increase its capacity when it’s 75% full.
Understanding the Fundamentals of HashMaps
Before we delve deeper into the usage of HashMaps, it’s essential to understand what they are and why they are useful in Java.
What is a HashMap?
A HashMap is part of Java’s collection framework. It’s a Map-based collection class that is used for storing Key & Value pairs, it’s denoted as HashMap
or HashMap
. This class makes no guarantees as to the order of the map.
How Does a HashMap Work?
HashMap works on the principle of hashing. Hashing is a process of converting an object into an integer, which can be used as an index to find the original object. When you put a key-value pair into the HashMap, the key is hashed, and the resulting hash code is used as an index where the value is stored within the table.
HashMap<String, Integer> map = new HashMap<>();
map.put("Alice", 25);
System.out.println("Hash code for 'Alice': " + "Alice".hashCode());
# Output:
# Hash code for 'Alice': 63593011
In this example, we’re creating a HashMap and adding a key-value pair. We’re then printing the hash code for the key ‘Alice’. The hashCode()
method is a built-in method of the Object class, and it returns the hash code of the object for which this method is invoked.
Why are HashMaps Useful?
HashMaps are extremely useful for retrieving data. Since data is stored with a key, you can quickly get a value by just knowing its key. This makes HashMaps perfect for situations where you need fast lookups and are working with large amounts of data.
Applying HashMaps in Real-World Java Projects
HashMaps are not just a theoretical concept – they’re a practical tool that you’ll often use in real-world Java projects. Let’s discuss a couple of scenarios where HashMaps come in handy.
Database Management with HashMaps
HashMaps can be used to cache data from a database. When you’re working with a database, you often need to retrieve data based on a unique identifier. By storing this data in a HashMap, you can significantly speed up these lookups.
HashMap<Integer, String> databaseCache = new HashMap<>();
// Assume we've loaded the cache from the database
String userName = databaseCache.get(userId);
System.out.println("User name: " + userName);
# Output:
# User name: Alice
In this example, we’re simulating a database cache using a HashMap. The keys are user IDs, and the values are user names. We can quickly retrieve a user’s name using their ID.
Web Applications and Session Management
In web applications, HashMaps can be used for session management. You can store user-specific data in a HashMap on the server, using the session ID as the key.
HashMap<String, User> sessions = new HashMap<>();
// Assume we've added some sessions
User user = sessions.get(sessionId);
System.out.println("Logged in as: " + user.getName());
# Output:
# Logged in as: Alice
In this example, we’re simulating session management in a web application. The keys are session IDs, and the values are User objects. We can retrieve the User object for a session using the session ID.
Further Resources for Mastering HashMaps in Java
If you’re interested in learning more about HashMaps and other data structures in Java, here are some resources you might find helpful:
- Mastering HashMaps in Java – Explore the Java HashMap class for key-value pair storage and retrieval.
Java HashSet Explained – Explore HashSet in Java for implementing sets based on hash tables.
Understanding Hash Tables in Java – Learn about hashing functions, collision resolution strategies, and key-value storage.
Java Collections Framework Tutorial by Oracle provides an in-depth understanding of the Java Collections Framework.
Java HashMap Tutorial – Baeldung’s tutorial for effectively using Java’s HashMap data structure.
Data Structures and Algorithms in Java Course – A Coursera specialization for learning data structures and algorithms in Java.
Wrapping Up: HashMap Initialization in Java
In this comprehensive guide, we’ve explored the intricacies of initializing and using HashMaps in Java, a fundamental part of Java’s collection framework.
We started off with the basics, learning how to initialize a HashMap, add elements to it, and retrieve them. We also touched on the importance of unique keys in a HashMap and how they relate to values. From there, we ventured into more advanced territory, discussing different types of keys and values, and initializing a HashMap with default values.
We also explored alternative data structures, such as TreeMap and LinkedHashMap, and discussed when to use these alternatives. Moreover, we delved into common challenges you might encounter when initializing and using HashMaps, and provided solutions for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
HashMap | Fast, allows null keys/values, unordered | No order preservation, single null key |
TreeMap | Sorted entries, no null keys/values | Slower due to sorting |
LinkedHashMap | Preserves insertion order, allows null keys/values | Slightly slower due to maintaining order |
Whether you’re just starting out with HashMaps or you’re looking to level up your Java skills, we hope this guide has given you a deeper understanding of HashMaps and their capabilities.
With its balance of speed, flexibility, and ease of use, HashMap is a powerful tool for handling key-value pairs in Java. Now, you’re well equipped to enjoy those benefits. Happy coding!