[SOLVED] ‘npm err! missing script: start’ | How to Fix
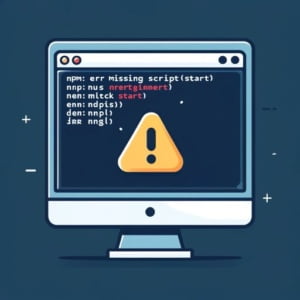
Has the ‘npm err! missing script: start’ message interrupted your development workflow? This error usually indicates a crucial part of your project setup is absent. It’s a hurdle that can halt your progress but, luckily, today’s article holds the solutions.
When developing software for IOFLOOD, quickly overcoming development obstacles is crucial to meet project deadlines and deliver high-quality solutions. To assist other developers utilizing our bare metal cloud servers, we have provided practical steps and insights on resolving the ‘npm err missing script start’ error.
This guide aims to navigate you through resolving this common npm issue, ensuring a smooth start to your projects. By understanding the root cause and following the steps outlined, you’ll be able to fix this error and prevent it from occurring in the future.
Let’s dive in and get your development workflow back on track.
TL;DR: How Do I Resolve ‘npm err missing script start’?
The error usually occurs when the
start
script is missing in yourpackage.json
file. To resolve it, add the line"start": "node [yourMainFile].js"
under “scripts”.
Here’s an example:
"scripts": {
"start": "node yourMainFile.js"
}
Replace ‘yourMainFile.js’ with the entry point of your application. This simple addition tells npm what command to execute when you run npm start
from your terminal.
Here’s a quick example:
npm start
# Output:
# > node yourMainFile.js
In this example, after adding the ‘start’ script to your ‘package.json’, running npm start
in your terminal will execute the ‘node yourMainFile.js’ command, effectively starting your application. This is a fundamental step in setting up your project for development and deployment.
Eager to dive deeper into npm and resolve more complex scenarios? Keep reading for detailed instructions and troubleshooting tips.
Table of Contents
Basic Fixes: ‘missing script start’ err
When you’re just starting out with Node.js and npm (Node Package Manager), encountering errors can be frustrating. One common issue is the ‘npm err missing script start’. This error pops up when npm doesn’t know what command to run to start your application. The solution? You need to tell npm exactly what to do by editing your ‘package.json’ file.
Editing package.json
Your ‘package.json’ file is like the brain of your project. It tells npm how to handle your project’s dependencies and scripts. When you encounter the ‘npm err missing script start’, it’s because the ‘start’ command is missing in this file. Here’s how you can fix it:
{
"name": "your-project-name",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"author": "",
"license": "ISC"
}
In this example, we’ve added a ‘start’ script that tells npm to run ‘node index.js’ when you execute npm start
. It’s crucial that ‘index.js’ is the entry point of your application. If your main file is named differently, replace ‘index.js’ with the correct file name.
Why It’s Important
By specifying a ‘start’ script, you’re not just resolving the ‘npm err missing script start’ error. You’re setting a clear entry point for your application, which is essential for both development and deployment. This small addition makes your project more manageable and easier to understand for anyone who might work on it in the future.
Remember, the ‘start’ script is just the beginning. As you grow more comfortable with npm, you’ll find that the ‘scripts’ section of your ‘package.json’ can be a powerful tool for automating your development workflow.
Advanced npm Scripting Techniques
As you venture deeper into the world of Node.js and npm, you’ll likely encounter more complex project structures or scenarios where the basic ‘start’ script doesn’t quite cut it. This could be due to the nature of globally installed packages, monorepo setups, or projects that require a more intricate startup process. Let’s explore how to handle these situations.
Handling Globally Installed Packages
Sometimes, your project might depend on packages that are installed globally on your machine. While it’s generally a good practice to keep all dependencies local to ensure consistency across environments, there are cases where global packages are necessary. Here’s how you can modify the ‘start’ script to ensure it works in such scenarios:
"scripts": {
"start": "PATH=$(npm bin):$PATH node index.js"
}
This script modification adds the local node_modules/.bin directory to the PATH environment variable, ensuring that any globally installed packages can be accessed. This is particularly useful when your application needs to run tasks or scripts that are installed globally.
Monorepo Setup
In a monorepo setup, where multiple packages are managed within a single repository, starting the application might require initializing several services or components. Here’s an example of a ‘start’ script that handles such complexity:
"scripts": {
"start": "concurrently 'npm run serviceA' 'npm run serviceB'"
}
In this example, we use the ‘concurrently’ package to run multiple npm scripts in parallel. This is ideal for starting different parts of your application simultaneously, such as a backend service and a frontend application. Make sure to install ‘concurrently’ as a development dependency in your project.
The Importance of Flexibility
These advanced use cases highlight the importance of flexibility in your ‘start’ script. By understanding and adapting to the specific needs of your project, you can create a development environment that is both robust and efficient. Whether you’re dealing with global dependencies or managing a complex monorepo, the right npm scripts can make all the difference in streamlining your workflow.
Alternate Tools for npm Scripts
When you’ve mastered the basics and intermediate levels of npm scripting, it’s time to dive into more sophisticated development workflows. These can significantly enhance your development experience and efficiency. Let’s explore some alternative tools and npm scripts that cater to complex setups or specific workflow requirements.
Live Reloading with Nodemon
One of the most sought-after features during development is live reloading. It allows you to automatically restart your application whenever file changes in the directory are detected. This is where nodemon
shines. Unlike the basic node
command used in the ‘start’ script, nodemon
monitors for any changes in your source and automatically restarts your server, saving you time and hassle.
"scripts": {
"dev": "nodemon yourMainFile.js"
}
After adding this script to your ‘package.json’, run it using npm run dev
:
npm run dev
# Output:
# [nodemon] starting `node yourMainFile.js`
# [nodemon] watching path(s): *.*
# [nodemon] watching extensions: js,mjs,json
This output indicates that nodemon
is actively monitoring your project files. Any changes will trigger a restart, providing an instant feedback loop for your development process.
Scripting for Different Environments
Crafting npm scripts that are environment-specific can greatly improve your project’s adaptability and deployment process. For instance, you might want different behaviors for development, testing, and production environments. Here’s a simple way to achieve this differentiation:
"scripts": {
"start": "NODE_ENV=production node yourMainFile.js",
"start:dev": "NODE_ENV=development nodemon yourMainFile.js"
}
By prefixing the node
or nodemon
command with NODE_ENV=production
or NODE_ENV=development
, you can set environment variables directly in your npm scripts. This approach allows you to tailor the behavior of your application based on the current environment, enhancing both development flexibility and deployment reliability.
Making the Right Choice
Exploring alternative tools and scripting techniques can significantly elevate your development workflow. Whether it’s achieving live reloading with nodemon
or setting up environment-specific scripts, these advanced approaches offer a path to more efficient and enjoyable development experiences.
Avoiding Common npm Script Pitfalls
While resolving the ‘npm err missing script start’ error, developers often encounter a few common pitfalls. Understanding these can save you time and frustration. Let’s discuss these issues and how to avoid them.
Syntax Errors in package.json
A frequent source of headaches is syntax errors within the ‘package.json’ file. Even a missing comma can cause npm to throw errors, preventing your scripts from running correctly.
{
"scripts": {
"start": "node app.js",
// Incorrect comment style leads to syntax error
}
}
In JSON files, comments are not allowed, and trailing commas can lead to syntax errors. Ensuring your ‘package.json’ is valid JSON is crucial. Use JSON validators or linters to catch these errors early.
Handling Missing Dependencies
Another common issue is missing dependencies. If your ‘start’ script relies on a package that isn’t installed, you’ll run into problems.
node app.js
# Output:
# Error: Cannot find module 'express'
In this example, attempting to start an application that uses Express without having it installed results in an error. The solution is straightforward: ensure all dependencies are correctly listed in your ‘package.json’ and installed using npm install
.
Environmental Issues
Environmental issues, such as incorrect Node.js versions or PATH settings, can also lead to the ‘npm err missing script start’ error. For instance, if your application requires a specific Node.js version that isn’t installed or properly configured, npm won’t be able to execute the start script.
npm start
# Output:
# Error: version not found: node
This error might occur if the Node.js version specified in your ‘package.json’ or a .nvmrc file isn’t installed on your system. Using a version manager like nvm (Node Version Manager) can help manage and switch between Node.js versions seamlessly.
Best Practices for Troubleshooting
Troubleshooting ‘npm err missing script start’ often requires a keen eye for detail and an understanding of common pitfalls. By paying attention to syntax in your ‘package.json’, ensuring all dependencies are installed, and managing environmental issues, you can overcome the ‘npm err missing script start’ error efficiently.
Understanding npm and package.json
To effectively tackle the ‘npm err missing script start’ issue, a solid understanding of npm (Node Package Manager) and the pivotal role of the ‘package.json’ file in a Node.js project is essential. npm not only manages package installations but also defines project scripts that automate tasks, including starting your application.
What is npm?
npm is the world’s largest software registry, offering a plethora of packages to streamline development processes. It comes bundled with Node.js, allowing developers to install, share, and manage dependencies in their projects.
The Heart of Your Project: package.json
The ‘package.json’ file acts as the blueprint for your project. It specifies metadata, including the project’s name, version, and dependencies. Crucially, it defines scripts that control various tasks such as testing, building, and starting your application.
{
"name": "example-project",
"version": "1.0.0",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"dependencies": {
"express": "^4.17.1"
}
}
In this ‘package.json’ example, you see a basic structure with a ‘test’ script defined. While this doesn’t include a ‘start’ script, adding one is straightforward, as demonstrated in previous sections.
The Role of Scripts
Scripts in ‘package.json’ serve to automate tasks. For instance, a ‘start’ script specifies the command to run your application, making it executable with a simple npm start
. This streamlines the development process, ensuring consistency across environments.
npm run test
# Output:
# Error: no test specified
This code block demonstrates running the ‘test’ script defined in our ‘package.json’. The output indicates no test is specified, showcasing how npm scripts execute commands defined under the ‘scripts’ section. Understanding and utilizing these scripts can significantly enhance your development workflow and mitigate common errors like ‘npm err missing script start’.
Expanding npm Script Capabilities
As you become more comfortable resolving common issues like ‘npm err missing script start’, it’s crucial to look beyond immediate fixes and understand the broader context in which npm scripts operate. These scripts are not just about starting your application; they’re a gateway to automating your entire development, build, and deployment processes.
Automating Development Workflows
npm scripts can be used to automate repetitive tasks, making your development process more efficient. For example, you can create a script that watches for file changes and automatically runs linting and tests:
"scripts": {
"watch": "nodemon --exec 'npm test'"
}
npm run watch
# Output:
# [nodemon] starting `npm test`
# [nodemon] watching path(s): *.*
In this example, nodemon
is used to watch for any file changes and execute npm test
each time a change is detected. This setup ensures that your tests are run automatically, helping you catch errors early.
Streamlining Build Processes
npm scripts can also streamline your build process. For instance, you can set up scripts to minify your JavaScript files or compile your Sass files into CSS. This automation simplifies the build process, ensuring consistency and saving time.
Deployment Made Easy
Deployment can be automated using npm scripts as well. Scripts can be set up to run tests, build your application, and deploy it to your hosting platform. This level of automation ensures that your application is always deployed in a consistent state, reducing the chances of deployment-related errors.
Further Resources for npm Script Mastery
To deepen your understanding of npm and its capabilities, here are three invaluable resources:
- npm Documentation – The official npm documentation offers comprehensive guides on npm commands, including scripting and package management.
Node.js Guides – The Node.js official site provides tutorials and guides that cover a wide range of topics, from getting started with Node.js to advanced concepts.
The npm Blog – For the latest updates, features, and best practices, the npm blog is an excellent resource. It offers insights into the evolving npm ecosystem and how to make the most of it.
By leveraging npm scripts, you can automate a significant portion of your development workflow, streamline build processes, and simplify deployment. The resources provided will help you explore further and harness the full potential of npm and Node.js in your projects.
Solving ‘npm err missing script start’
In this comprehensive guide, we’ve tackled the ‘npm err missing script start’ error, a common stumbling block for developers working with Node.js and npm. This error, while initially daunting, can be resolved with a deeper understanding of npm and the pivotal role of the ‘package.json’ file.
We began with the basics, illustrating how to rectify the error by adding a ‘start’ script to your ‘package.json’. This fundamental step ensures that npm knows how to initiate your application, setting the stage for a smooth development process.
Moving forward, we explored more complex scenarios, such as dealing with globally installed packages and managing projects within a monorepo setup. These intermediate solutions demonstrated the flexibility and power of npm scripts in accommodating diverse project structures.
We also delved into expert-level strategies, including using alternative tools like ‘nodemon’ for live reloading and crafting scripts for different development environments. These advanced techniques underscore the potential of npm scripts to streamline and enhance your development workflow.
Approach | Use Case | Complexity |
---|---|---|
Basic ‘start’ Script | Single-entry projects | Beginner |
Globally Installed Packages | Projects with global dependencies | Intermediate |
‘nodemon’ and Environment Scripts | Complex setups requiring live reloads or specific environment configurations | Expert |
As we wrap up, it’s clear that mastering npm and its scripting capabilities can significantly impact your productivity and project management. Whether you’re troubleshooting the ‘npm err missing script start’ error or looking to optimize your development workflow, the insights and strategies shared in this guide offer a solid foundation.
With a blend of basic fixes and advanced troubleshooting techniques, you’re now better equipped to tackle npm-related challenges head-on. Remember, the journey doesn’t end here. The world of npm is vast, and there’s always more to learn and explore. Happy coding!