Handling Java User Input: Tips and Tricks
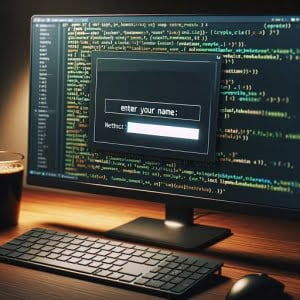
Are you finding it challenging to handle user input in Java? You’re not alone. Many developers find themselves in a bind when it comes to managing user input in Java, but we’re here to help.
Think of Java’s user input handling as a skilled receptionist – capable of efficiently managing user input with the right tools. These tools provide a versatile and handy solution for various tasks.
In this guide, we’ll walk you through the process of handling user input in Java, from basic usage to advanced techniques. We’ll cover everything from the basics of the Scanner
class to more advanced methods, as well as alternative approaches.
So, let’s dive in and start mastering user input in Java!
TL;DR: How Do I Handle User Input in Java?
In Java, you can handle user input using the
Scanner
class. To use the class you must first import it with,import java.util.Scanner;
and then instantiate an instance with.Scanner scanner = new Scanner(System.in);
. This class provides methods to read user input from the console.
Here’s a simple example:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter something:");
String input = scanner.nextLine();
System.out.println("You entered: " + input);
}
}
# Output:
# Enter something:
# [Your input here]
# You entered: [Your input here]
In this example, we create a Scanner
object and use its nextLine()
method to read a line of text from the user. We then print out the text that the user entered.
This is a basic way to handle user input in Java, but there’s much more to learn about the
Scanner
class and other methods of handling user input. Continue reading for a more detailed guide on handling user input in Java.
Table of Contents
Getting Started with the Scanner
Class
The Scanner
class in Java is a simple text scanner which can parse primitive types and strings. It’s a part of java.util
package, so you’ll need to import this package in your Java program.
The Scanner
class is used to get user input, and it is found in the java.util
package. To use the Scanner
class, create an object of the class and use any of the available methods found in the Scanner
class documentation.
Here is a basic example of how to use the Scanner
class to read user input:
import java.util.Scanner; // Import the Scanner class
public class MyClass {
public static void main(String[] args) {
Scanner myObj = new Scanner(System.in); // Create a Scanner object
System.out.println("Enter username");
String userName = myObj.nextLine(); // Read user input
System.out.println("Username is: " + userName); // Output user input
}
}
# Output:
# Enter username
# [Your username here]
# Username is: [Your username here]
In this example, we first import the Scanner
class. Then, we create a Scanner
object named myObj
and use it to read a string as input from the user. After the user inputs a string, the program prints the string.
The Scanner
class is quite versatile and can handle different types of input, not just strings. It’s a good choice for simple applications where you need to read and process user input.
However, it’s important to note that the Scanner
class can be slower compared to other methods, especially for large inputs, and it does not provide the same level of control over your input as some of the more advanced methods.
Handling Different Types of User Input
As you become more comfortable with the Scanner
class, you’ll find its ability to handle different types of user input invaluable. It’s not just about reading strings – the Scanner
class can also read other types of input, such as integers, doubles, and booleans.
Let’s take a look at how to handle these different types of input.
Reading Integers
To read an integer input from the user, you can use the nextInt()
method. Here’s an example:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter an integer:");
int number = scanner.nextInt();
System.out.println("You entered: " + number);
}
}
# Output:
# Enter an integer:
# [Your integer here]
# You entered: [Your integer here]
In this code, we use the nextInt()
method to read an integer input from the user.
Reading Doubles
Similarly, to read a double input from the user, you can use the nextDouble()
method:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a double:");
double number = scanner.nextDouble();
System.out.println("You entered: " + number);
}
}
# Output:
# Enter a double:
# [Your double here]
# You entered: [Your double here]
Here, we use the nextDouble()
method to read a double input from the user.
Reading Booleans
Finally, to read a boolean input from the user, you can use the nextBoolean()
method:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a boolean:");
boolean bool = scanner.nextBoolean();
System.out.println("You entered: " + bool);
}
}
# Output:
# Enter a boolean:
# [Your boolean here]
# You entered: [Your boolean here]
In this code, we use the nextBoolean()
method to read a boolean input from the user.
These are just a few examples of the many methods available in the Scanner
class for reading different types of user input. By understanding these methods, you can make your Java programs more interactive and versatile.
Exploring Alternative Methods for User Input
While the Scanner
class is a versatile tool for handling user input, Java offers other classes that can be used for this purpose. Two of these are the BufferedReader
class and the Console
class. Let’s take a closer look at these alternatives.
The BufferedReader
Class
The BufferedReader
class can be used to read text from a character-input stream, buffering characters to provide efficient reading of characters, arrays, and lines. Here’s a simple example:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter a line of text:");
String input = reader.readLine();
System.out.println("You entered: " + input);
}
}
# Output:
# Enter a line of text:
# [Your text here]
# You entered: [Your text here]
In this example, we create a BufferedReader
object and use it to read a line of text from the user. The BufferedReader
class can be more efficient than the Scanner
class for reading large amounts of input, but it’s also more complex and requires more boilerplate code.
The Console
Class
The Console
class is another alternative for handling user input. It has a simpler syntax than BufferedReader
, but it’s not as versatile as Scanner
. Here’s how you can use the Console
class to read user input:
public class Main {
public static void main(String[] args) {
String input = System.console().readLine("Enter a line of text: ");
System.out.println("You entered: " + input);
}
}
# Output:
# Enter a line of text:
# [Your text here]
# You entered: [Your text here]
In this code, we use the readLine()
method of the Console
class to read a line of text from the user. The Console
class is easy to use, but it’s not as flexible as the Scanner
class because it doesn’t provide methods for reading different types of input.
Method | Advantages | Disadvantages |
---|---|---|
Scanner | Versatile, handles different types of input | Slower for large inputs |
BufferedReader | Efficient for large inputs | More complex, more boilerplate code |
Console | Simple syntax | Less versatile |
These are just a few of the many ways you can handle user input in Java. Depending on your specific needs, you might find one method more suitable than the others. It’s worth taking the time to understand these different methods and how they can benefit your Java programming.
Troubleshooting Common Issues with User Input
When working with user input in Java, you may encounter a few common issues. Let’s discuss some of these problems and how to solve them.
Handling InputMismatchException
If you’re using the Scanner
class to read an integer or a double, but the user enters a string, you’ll run into an InputMismatchException
. Here’s how it might look:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter an integer:");
int number = scanner.nextInt(); // This line throws an exception if the input is not an integer
System.out.println("You entered: " + number);
}
}
# Output:
# Enter an integer:
# [Your non-integer input here]
# Exception in thread "main" java.util.InputMismatchException
To handle this exception, you can use a try-catch
block:
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter an integer:");
try {
int number = scanner.nextInt();
System.out.println("You entered: " + number);
} catch (InputMismatchException e) {
System.out.println("That's not an integer!");
}
}
}
# Output:
# Enter an integer:
# [Your non-integer input here]
# That's not an integer!
In this example, if the user enters a non-integer input, the program catches the InputMismatchException
and prints a custom error message.
Dealing with Different Types of Input
When reading user input, it’s important to consider the type of input you’re expecting. For instance, if you’re using the nextLine()
method of the Scanner
class to read a string, but the user enters an integer, the program will read the integer as a string.
To ensure that your program can handle different types of input, you can use the hasNextInt()
, hasNextDouble()
, and hasNextBoolean()
methods of the Scanner
class to check the type of the next token in the input.
Understanding these common issues and their solutions will help you write robust code that can handle user input effectively.
Understanding Java’s Input/Output Operations
Before diving deeper into handling user input in Java, it’s crucial to understand the fundamental concepts of Java’s input/output (I/O) operations and streams.
Java I/O Operations
Java’s I/O operations are designed to handle any form of input and output, from reading and writing to local disks, interacting with users, or communicating over networks. These operations are a fundamental part of any interactive application, including those that handle user input.
The Concept of Streams
In the context of Java’s I/O operations, a stream can be thought of as a sequence of data. There are two main types of streams in Java:
- Input Stream: This is used to read data from a source (like user input).
- Output Stream: This is used to write data to a destination.
When handling user input, we’re primarily dealing with input streams.
Understanding the Scanner
, BufferedReader
, and Console
Classes
These three classes are some of the main tools in Java for handling user input, each with its own strengths and use cases.
Scanner
: As we’ve seen, theScanner
class is a versatile tool for reading different types of input from a stream. It can parse primitive types and strings using regular expressions, making it a flexible option for many applications.BufferedReader
: This class reads text from a character-input stream, buffering characters to provide efficient reading. It’s a good choice when you need to read large amounts of data.Console
: TheConsole
class is a convenient way to read strings and passwords from the console. However, it’s less flexible than the other two classes and might not be suitable for all applications.
Understanding these fundamental concepts and classes will provide a solid foundation for handling user input in Java.
The Power of User Input in Interactive Applications
Handling user input is a fundamental aspect of creating interactive applications. Whether you’re building a command-line tool, a web application, or a complex enterprise software, understanding how to handle user input effectively can make your application more interactive and user-friendly.
For instance, consider a command-line tool that performs different operations based on user input. Without effective handling of user input, the tool would be less interactive and harder to use.
Exploring Related Concepts
Beyond handling user input, there are many related concepts in Java that you might find interesting. For example, file I/O operations allow your application to read from and write to files, which can be used to store user input for later use.
Another related concept is networking. By understanding how to handle user input, you can build applications that communicate over the network, accept input from users, and respond accordingly.
Further Resources for Mastering Java User Input
To help you further explore these concepts, here are some additional resources:
- Understanding Java Methods – Learn how to define and call methods in Java.
Using Scanner Class in Java – Learn how to use Scanner for reading input from the console or files
Oracle’s Java Tutorials covers a wide range of topics, including I/O operations and networking.
Java I/O Fundamentals – This guide from Baeldung dives deep into Java’s I/O operations.
Java Networking Tutorial – This tutorial from JavaTpoint provides a detailed introduction to networking in Java.
By understanding how to handle user input in Java and exploring related concepts, you can greatly enhance your Java programming skills.
Wrapping Up: User Input in Java
In this comprehensive guide, we’ve delved into the world of handling user input in Java, exploring the various methods and techniques that can be used to read and process user input.
We started with the basics, learning how to use the Scanner
class to read different types of input from the user. We then explored more advanced uses of the Scanner
class, including reading integers, doubles, and booleans.
We also looked at alternative methods for handling user input, such as the BufferedReader
class and the Console
class. Along the way, we discussed common issues that you might encounter when handling user input in Java and provided solutions to these problems.
Here’s a quick comparison of the methods we’ve discussed:
Method | Versatility | Efficiency | Complexity |
---|---|---|---|
Scanner | High | Moderate | Low |
BufferedReader | Moderate | High | Moderate |
Console | Low | High | Low |
Whether you’re a beginner just starting out with Java or an experienced developer looking for a refresher, we hope this guide has helped you understand how to handle user input in Java more effectively.
Understanding user input is a fundamental aspect of creating interactive applications in Java. With the knowledge you’ve gained from this guide, you’re now well-equipped to handle user input in your Java programs. Happy coding!