Using Python to Run Shell Commands
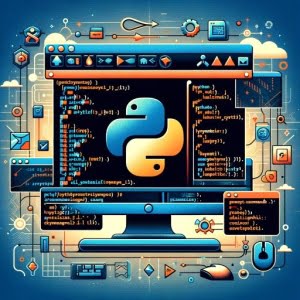
Struggling to run shell commands using Python? You’re not alone. Many developers find themselves scratching their heads when they first try to interact with the shell using Python. But fear not, this comprehensive guide is here to help.
In this guide, we will walk you through the process of running shell commands with Python. We’ll start from the basics and gradually move towards more advanced techniques. By the end of this guide, you’ll be able to use Python to run shell commands with confidence and ease.
Let’s dive in and start exploring the power of Python and shell commands!
TL;DR: How Do I Run Shell Commands in Python?
You can use the
subprocess
module in Python to run shell commands. Here’s a simple example:
import subprocess
subprocess.run(['ls', '-l'])
# Output:
# [Expected output from the ls -l command]
In this example, we’re using the subprocess.run()
function to execute the ‘ls -l’ shell command. This command lists the files in the current directory in long format, showing details like file permissions, number of links, owner, group, size, and time of last modification.
This is just the tip of the iceberg. Keep reading for a more in-depth explanation and advanced usage scenarios.
Table of Contents
Python’s Subprocess.Run: A Beginner’s Guide
The subprocess.run()
function is the recommended approach to invoking shell commands in Python. It was added in Python 3.5 and is a part of the subprocess
module. This function is versatile and can handle a wide variety of tasks.
Here’s a simple example of how you can use subprocess.run()
to execute a shell command:
import subprocess
result = subprocess.run(['ls', '-l'])
# Output:
# [Expected output from the ls -l command]
In this code block, we’re importing the subprocess
module and then using the run()
function to execute the ‘ls -l’ shell command. The command is passed as a list of strings to the function, where the first item is the command itself and the subsequent items are the arguments to the command.
The function runs the command, waits for it to complete, and then returns a subprocess.CompletedProcess
instance. This instance contains information about the completed command, such as the return code, output, and any errors.
Advantages of Subprocess.Run
The subprocess.run()
function has several advantages. It’s simple to use, yet powerful and flexible. It can execute both simple and complex shell commands, and it provides a high level of control over how the command is run and how its output is handled.
Potential Pitfalls
While subprocess.run()
is powerful, it’s not without its potential pitfalls. For example, if the command you’re running produces a lot of output, it could cause your program to hang. This is because the run()
function captures the command’s output in memory, and if the output is too large, it could consume all available memory.
Also, if you’re running a command that takes a long time to complete, the run()
function will block until the command has finished. This could cause your program to become unresponsive. However, these issues can be mitigated using various techniques, which we’ll discuss in the ‘Advanced Use’ section.
Complex Tasks with Subprocess Module
As we delve deeper into the subprocess
module, we discover its capability to handle more complex tasks. For instance, it can capture output, handle errors, and even run shell commands using the shell=True
argument.
Capturing Output
To capture the output of a shell command, we can use the stdout
parameter in the subprocess.run()
function. Here’s how you can do it:
import subprocess
result = subprocess.run(['ls', '-l'], stdout=subprocess.PIPE)
print(result.stdout.decode())
# Output:
# [Expected output from the ls -l command]
In this example, we pass stdout=subprocess.PIPE
to the run()
function. This tells the function to capture the standard output of the command. We then print the output using result.stdout.decode()
. The decode()
function converts the output from bytes to a string.
Error Handling
Error handling is a crucial aspect of running shell commands. The subprocess
module can handle errors using the check
parameter. If check
is set to True and the process exits with a non-zero exit status, a subprocess.CalledProcessError
exception will be raised. Here’s an example:
import subprocess
try:
subprocess.run(['ls', '-l'], check=True)
except subprocess.CalledProcessError as e:
print(f'Command {e.cmd} failed with error {e.returncode}')
# Output:
# Command ['ls', '-l'] failed with error 1
In this code, if the ‘ls -l’ command fails for any reason, a subprocess.CalledProcessError
exception is raised, and the error message is printed.
Using Shell=True
In certain cases, you might want to run a shell command as a string, rather than as a list of strings. This can be done using the shell=True
argument. However, this method is less secure and can make your program vulnerable to shell injection attacks, so use it with caution. Here’s an example:
import subprocess
result = subprocess.run('ls -l | grep .py', shell=True)
# Output:
# [Expected output from the ls -l | grep .py command]
In this example, we’re using a pipe (|
) to combine two shell commands. This is not possible when passing the command as a list of strings, so we use shell=True
to pass the command as a string instead.
As we can see, the
subprocess
module is a powerful tool for running shell commands in Python. It provides a high level of control over how commands are run and how their output is handled, making it a great choice for both simple and complex tasks.
Alternative Methods to Run Shell Commands
While the subprocess
module is a powerful tool for running shell commands in Python, it’s not the only option. There are other methods available that might be more suitable depending on the specific needs of your project. Let’s explore some of these alternatives.
Using os.system()
The os.system()
function is a simple way to run a shell command. Here’s an example:
import os
os.system('ls -l')
# Output:
# [Expected output from the ls -l command]
In this code block, we’re using os.system()
to execute the ‘ls -l’ shell command. The command is passed as a string to the function, which then runs the command and returns the exit status.
However, os.system()
has some limitations. It doesn’t allow capturing the output of the command or handling errors in a granular way. It’s also considered less secure than subprocess.run()
.
Using os.popen()
The os.popen()
function allows you to open a pipe to or from a command. This can be useful for running a command and processing its output. Here’s an example:
import os
result = os.popen('ls -l').read()
print(result)
# Output:
# [Expected output from the ls -l command]
In this example, we’re using os.popen()
to execute the ‘ls -l’ command and capture its output. The output can then be read and processed.
While os.popen()
provides more control over the command’s output than os.system()
, it’s still less flexible and less secure than subprocess.run()
.
Using shlex.split()
The shlex.split()
function can be used to split a command string into a list of strings. This can be useful when you want to pass a command as a list of strings to subprocess.run()
. Here’s an example:
import subprocess
import shlex
command = 'ls -l'
subprocess.run(shlex.split(command))
# Output:
# [Expected output from the ls -l command]
In this example, we’re using shlex.split()
to split the command string into a list of strings, which is then passed to subprocess.run()
.
The shlex.split()
function is a handy tool when dealing with complex commands, but it doesn’t provide the same level of control over the command execution as subprocess.run()
.
While there are several alternatives to
subprocess.run()
, each with their own advantages and disadvantages,subprocess.run()
remains the recommended method for running shell commands in Python due to its flexibility, control, and security.
Running shell commands from Python isn’t always smooth sailing. There are several common issues that developers often encounter. Let’s discuss some of these problems and their solutions.
Command Not Found Errors
One common issue is getting a ‘command not found’ error. This usually happens when the command you’re trying to run isn’t available in the system’s PATH. Here’s an example:
import subprocess
try:
subprocess.run(['nonexistentcommand'], check=True)
except subprocess.CalledProcessError as e:
print(f'Command {e.cmd} failed with error {e.returncode}')
# Output:
# Command ['nonexistentcommand'] failed with error 1
In this case, the solution is to either use the full path to the command or add the directory containing the command to the system’s PATH.
Permission Issues
Another common issue is permission errors. This happens when the command you’re trying to run requires certain permissions that your Python script doesn’t have. Here’s an example:
import subprocess
try:
subprocess.run(['command_needing_permissions'], check=True)
except subprocess.CalledProcessError as e:
print(f'Command {e.cmd} failed with error {e.returncode}')
# Output:
# Command ['command_needing_permissions'] failed with error 1
The solution here is to either modify the permissions of the command or run your Python script with the necessary permissions.
Running shell commands from Python can be a powerful tool, but it also comes with its own set of challenges. Understanding these common issues and how to solve them is crucial for effective and efficient command execution.
Shell Commands and Automation
Running shell commands with Python has a wide range of applications. It’s particularly relevant in automation, scripting, and system administration. By integrating shell commands into your Python scripts, you can automate repetitive tasks, manage system resources, and much more.
Python Scripting and Task Automation
Python is a popular choice for scripting and task automation. Here’s a simple Python script that automates the process of creating a directory and listing its contents:
import subprocess
# Create a new directory
subprocess.run(['mkdir', 'new_directory'])
# List the contents of the new directory
result = subprocess.run(['ls', '-l', 'new_directory'], stdout=subprocess.PIPE)
print(result.stdout.decode())
# Output:
# total 0
In this script, we’re using the subprocess.run()
function to create a new directory and list its contents. This is a simple example, but the same principles can be applied to automate more complex tasks.
System Administration with Python
System administrators often use Python to manage system resources. For instance, they might use it to monitor system performance, manage files, or automate software installation. Python’s ability to run shell commands makes it a powerful tool for these tasks.
import subprocess
# Get system information using the 'uname -a' command
result = subprocess.run(['uname', '-a'], stdout=subprocess.PIPE)
print(result.stdout.decode())
# Output:
# [Expected output from the uname -a command]
In this example, we’re using the uname -a
command to get information about the system. This can be useful for system administrators who need to monitor system performance or diagnose issues.
Expanding Your Knowledge
Running shell commands with Python is a vast topic with many related concepts to explore. If you’re interested in learning more, you might want to look into Python scripting, task automation, system administration, or the subprocess
module in more depth.
There are many resources available online, including Python’s official documentation, online tutorials, and community forums.
Python and Shell Commands: A Recap
Running shell commands using Python is a powerful feature that can significantly enhance your scripts. We’ve explored how to use the subprocess.run()
function to execute commands, capture their output, and handle errors. We’ve also discussed some potential pitfalls, such as memory overflow and blocking issues, and how to mitigate them.
Alongside subprocess.run()
, we’ve looked at alternative methods like os.system()
, os.popen()
, and shlex.split()
. While these methods can be useful in certain scenarios, subprocess.run()
remains the recommended choice due to its flexibility, control, and security.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
subprocess.run() | Powerful, flexible, secure | Can cause memory overflow if output is too large |
os.system() | Simple to use | Cannot capture output, less secure |
os.popen() | Can capture output | Less flexible and secure than subprocess.run() |
shlex.split() | Useful for splitting command strings | Does not execute commands |
Common issues when running shell commands from Python include ‘command not found’ errors and permission issues. We’ve discussed how to solve these problems and the importance of error handling in your scripts.
Lastly, we’ve touched on the broader applications of running shell commands with Python, including automation, scripting, and system administration. Python’s ability to execute shell commands opens up a wide range of possibilities, making it a valuable skill for any Python developer.
We hope this guide has been helpful in your journey to mastering shell commands in Python. Remember, practice is key when learning a new skill. So don’t hesitate to get your hands dirty and start coding!