How to Sort ArrayList in Java: A Step-by-Step Guide
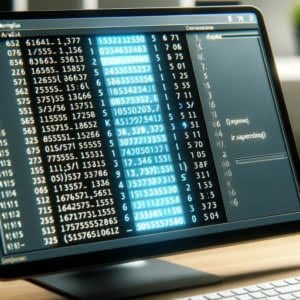
Ever found yourself puzzled when trying to sort ArrayLists in Java? You’re not alone. Many developers find this task a bit challenging, but Java provides us with the tools to make it easier. Think of Java’s sorting tools as a librarian arranging books – they help us organize our ArrayLists in a logical and efficient manner.
This guide will walk you through the process of sorting ArrayLists in Java, from the basics to more advanced techniques. We’ll cover everything from using the Collections.sort()
method, handling ArrayLists with custom objects using a Comparator, to exploring alternative approaches with Java 8’s Stream API.
So, let’s dive in and start mastering ArrayList sorting in Java!
TL;DR: How Do I Sort an ArrayList in Java?
Java provides the
Collections.sort()
method to sort an ArrayList. This method sorts the ArrayList in ascending order, making it easier to manage and navigate through your data.
Here’s a simple example:
ArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(5, 3, 8, 1));
Collections.sort(numbers);
System.out.println(numbers);
# Output:
# [1, 3, 5, 8]
In this example, we create an ArrayList named ‘numbers’ and populate it with the integers 5, 3, 8, and 1. We then use the Collections.sort()
method to sort the ArrayList. The output is the sorted ArrayList: [1, 3, 5, 8].
This is just a basic way to sort an ArrayList in Java, but there’s much more to learn about sorting ArrayLists, especially when dealing with custom objects or when you want to sort in descending order. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Sorting ArrayLists with Collections.sort()
- Sorting Custom Objects in ArrayLists
- Alternative Methods for Sorting ArrayLists
- Handling Common Issues in ArrayList Sorting
- Understanding Java’s ArrayList and Sorting Algorithms
- Exploring the Relevance of ArrayList Sorting
- Wrapping Up: Mastering ArrayList Sorting in Java
Sorting ArrayLists with Collections.sort()
Java offers a built-in method to sort ArrayLists: Collections.sort()
. This method sorts the elements of the ArrayList in ascending order. It’s a simple and efficient way to organize your data.
Here’s an example of how to use Collections.sort()
:
ArrayList<String> fruits = new ArrayList<>(Arrays.asList('Orange', 'Apple', 'Banana'));
Collections.sort(fruits);
System.out.println(fruits);
# Output:
# [Apple, Banana, Orange]
In this example, we have an ArrayList named ‘fruits’ containing three elements. We use the Collections.sort()
method to sort the fruits in alphabetical order.
Understanding Collections.sort()
The Collections.sort()
method works on the principle of ‘comparison’. It compares two elements at a time and swaps them if they are in the wrong order. The process is repeated until the entire list is sorted.
Advantages and Pitfalls of Collections.sort()
The main advantage of Collections.sort()
is its simplicity. It’s easy to understand and implement. However, it may not be the best choice for all scenarios. For instance, Collections.sort()
sorts in ascending order by default. If you need to sort in descending order, you’ll need to modify the method or use an alternative approach.
Additionally, Collections.sort()
can only sort ArrayLists of objects that implement the Comparable
interface. If your ArrayList contains custom objects that don’t implement Comparable
, you’ll need to provide a Comparator
.
Sorting Custom Objects in ArrayLists
When working with ArrayLists of custom objects, sorting becomes a bit more complex. The Collections.sort()
method alone won’t be enough, as Java needs to know how to compare these custom objects. This is where a Comparator
comes into play.
A Comparator
is a functional interface that defines the compare()
method. We can use it to tell Java how to compare and sort our custom objects.
Using a Comparator to Sort ArrayLists
Let’s say we have an ArrayList
of Person
objects, and we want to sort them by name. Here’s how we can do it:
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return name;
}
}
ArrayList<Person> people = new ArrayList<>();
people.add(new Person('Alice', 30));
people.add(new Person('Bob', 25));
people.add(new Person('Charlie', 35));
Collections.sort(people, Comparator.comparing(Person::getName));
System.out.println(people);
# Output:
# [Alice, Bob, Charlie]
In this example, we define a Person
class with a name
and age
attribute. We then create an ArrayList of Person
objects and add three persons to it. We sort the ArrayList using a Comparator
that compares Person
objects based on their name
attribute.
Understanding the Comparator
The Comparator
interface provides multiple utility methods for comparator construction, including comparing()
. In our example, we use Comparator.comparing(Person::getName)
to create a comparator that compares Person
objects by their name
attribute.
Best Practices for Using Comparators
When you’re using comparators, it’s important to ensure that your compare()
method is consistent. This means that it must always return the same result for the same inputs. Also, if compare(x, y)
returns zero, then compare(y, x)
should also return zero. Following these rules will help ensure that your sorting works correctly and predictably.
Alternative Methods for Sorting ArrayLists
While Collections.sort()
and Comparator
are common tools for sorting ArrayLists, Java offers other powerful options. One such alternative is Java 8’s Stream API, which provides a more functional programming approach to handling collections.
Sorting ArrayLists using Stream API
The Stream API provides a sorted()
method, which returns a stream consisting of the elements sorted according to their natural order, or by a custom Comparator
.
Here’s an example of sorting an ArrayList using Stream API:
ArrayList<String> fruits = new ArrayList<>(Arrays.asList('Orange', 'Apple', 'Banana'));
List<String> sortedFruits = fruits.stream().sorted().collect(Collectors.toList());
System.out.println(sortedFruits);
# Output:
# [Apple, Banana, Orange]
In this example, we create a stream from the fruits
ArrayList, sort it using the sorted()
method, and collect the results into a new list. The output is a new list with the fruits sorted in alphabetical order.
Advantages and Disadvantages of Using Stream API
One advantage of using the Stream API is its flexibility. It provides a wide range of operations, such as filtering, mapping, or reducing, which can be combined in a chain to form complex data processing pipelines.
However, the Stream API can be less intuitive to beginners compared to Collections.sort()
. It also has performance considerations: for small lists, the difference is negligible, but for larger lists, Collections.sort()
can be faster.
Choosing the Right Tool for Sorting
When choosing a sorting method, consider the size of your list, the type of your elements, and the specific requirements of your use case. Collections.sort()
is a good default choice for its simplicity and efficiency. A Comparator
is necessary when you’re dealing with custom objects. The Stream API is a powerful tool for more complex data processing tasks.
Handling Common Issues in ArrayList Sorting
While sorting ArrayLists in Java, you might encounter several common issues. Let’s look at these problems and discuss how to resolve them.
Dealing with ‘ClassCastException’
A ClassCastException
is thrown when an attempt is made to cast an object to a type with which it is not compatible. This might occur when trying to sort an ArrayList of custom objects without providing a proper Comparator
.
ArrayList<Object> list = new ArrayList<>();
list.add('Apple');
list.add(1);
Collections.sort(list);
# Output:
# Throws ClassCastException
In this example, we’re trying to sort an ArrayList that contains incompatible types (String
and Integer
). This will result in a ClassCastException
.
To resolve this, ensure that your ArrayList contains elements of the same or compatible types. If you’re working with custom objects, provide a Comparator
that defines how to sort the objects.
Handling Null Values
Another common issue is dealing with null values. The Collections.sort()
method doesn’t handle null values well and will throw a NullPointerException
if it encounters one.
ArrayList<String> list = new ArrayList<>();
list.add('Apple');
list.add(null);
Collections.sort(list);
# Output:
# Throws NullPointerException
In this case, you can avoid the exception by filtering out null values before sorting. The Stream API provides a convenient way to do this:
ArrayList<String> list = new ArrayList<>(Arrays.asList('Apple', null));
List<String> sortedList = list.stream().filter(Objects::nonNull).sorted().collect(Collectors.toList());
System.out.println(sortedList);
# Output:
# [Apple]
This code filters out null values from the list before sorting it, preventing the NullPointerException
.
Remember, understanding the nature of your data and the capabilities of your sorting tools will help you avoid these common issues and effectively sort your ArrayLists.
Understanding Java’s ArrayList and Sorting Algorithms
Before we delve deeper into sorting ArrayLists in Java, it’s crucial to understand the fundamental concepts underlying these operations: the ArrayList data structure and sorting algorithms.
What is an ArrayList in Java?
An ArrayList is a resizable array, also known as a dynamic array. It provides us with dynamic-sized, ordered collections of elements. Unlike traditional arrays, ArrayLists in Java can grow and shrink in size dynamically. This makes them a versatile tool for storing data in your applications.
ArrayList<String> fruits = new ArrayList<>();
fruits.add('Apple');
fruits.add('Banana');
fruits.add('Cherry');
System.out.println(fruits);
# Output:
# [Apple, Banana, Cherry]
In this example, we create an ArrayList of Strings and add three fruits to it. The ArrayList grows in size to accommodate these elements.
Sorting Algorithms: The Basics
Sorting is the process of arranging elements in a specific order, typically ascending or descending. Various algorithms can accomplish this task, each with its own advantages and drawbacks. Java uses a modified version of the MergeSort algorithm for sorting objects, which offers a good balance between performance and stability.
ArrayList<Integer> numbers = new ArrayList<>(Arrays.asList(5, 3, 8, 1));
Collections.sort(numbers);
System.out.println(numbers);
# Output:
# [1, 3, 5, 8]
In this example, we create an ArrayList of integers and sort it using the Collections.sort()
method. This method uses the MergeSort algorithm under the hood to sort the ArrayList in ascending order.
Understanding these fundamentals will provide you with the necessary background to comprehend and effectively use the sorting techniques discussed in this guide.
Exploring the Relevance of ArrayList Sorting
Sorting ArrayLists in Java is not just a programming exercise. It’s a fundamental operation that has wide-ranging applications in data processing, algorithms, and more.
Sorting in Data Processing
In data processing, sorting is often the first step in organizing and structuring data. Whether you’re working with a list of customers, a database of products, or a collection of sensor readings, sorting your data can help you find patterns, identify anomalies, and make sense of your data.
Sorting in Algorithms
Many algorithms also rely on sorting. For example, binary search, a fast algorithm for finding elements in a list, requires the list to be sorted. Similarly, many graph algorithms, such as Dijkstra’s shortest path algorithm, often start by sorting a list of edges.
Exploring Related Concepts
If you’re interested in sorting ArrayLists, you might also want to explore related data structures in Java, such as LinkedLists, Sets, and Maps. Each of these structures has its own characteristics and use cases, and understanding them can make you a more versatile and effective programmer.
Further Resources for Mastering Java Collections
If you’re interested in going beyond the basics of ArrayLists and sorting, here are a few resources that can help you deepen your understanding:
- Tips and Techniques for Using ArrayList in Java – Discover techniques for manipulating ArrayLists in Java.
Java ArrayList Initialization – Explore different initialization methods for populating ArrayLists.
Java ArrayList Length – Explore methods like size() and isEmpty() for checking the size of ArrayLists.
Java Collections Framework Tutorial provides an overview of Java Collections, like ArrayLists, LinkedLists, Sets, and Maps.
Java Algorithms and Clients provides Java implementations of fundamental algorithms and data types.
Java Programming Masterclass covers the core Java skills and a deep dive into the Collections Framework.
Remember, mastering a programming language is not just about learning syntax. It’s about understanding the concepts and tools at your disposal, and knowing how to use them effectively to solve problems.
Wrapping Up: Mastering ArrayList Sorting in Java
In this comprehensive guide, we’ve delved into the process of sorting ArrayLists in Java, demystifying this common task from the basics to more advanced techniques.
We began with the basics, learning how to use the Collections.sort()
method to sort an ArrayList. We then moved on to more advanced scenarios, exploring how to sort ArrayLists of custom objects using a Comparator
. We also introduced alternative approaches to sorting, such as using the Stream API, providing you with a variety of tools to tackle any sorting task.
Along the way, we discussed common challenges you might encounter when sorting ArrayLists, such as dealing with ‘ClassCastException’ and handling null values, and provided solutions to help you navigate these issues. We also delved into the fundamentals of ArrayLists and sorting algorithms, giving you a deeper understanding of the principles underlying these operations.
Here’s a quick comparison of the sorting methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
Collections.sort() | Simple, efficient | Cannot handle null values, sorts in ascending order by default |
Comparator | Can sort custom objects, flexible | More complex to implement |
Stream API | Functional programming approach, flexible | Less intuitive, slower for large lists |
Whether you’re just starting out with sorting ArrayLists in Java or you’re looking to deepen your understanding, we hope this guide has been a valuable resource.
Sorting ArrayLists is a fundamental skill in Java programming, and mastering it can greatly enhance your data manipulation capabilities. Now, you’re well equipped to handle any ArrayList sorting task that comes your way. Happy coding!