Java ArrayList Length: How to Get the Size of an ArrayList
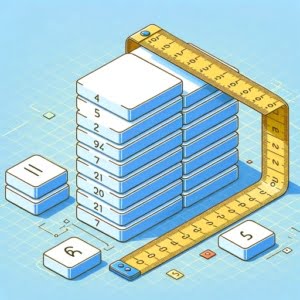
Are you finding it difficult to determine the length of an ArrayList in Java? You’re not alone. Many developers, especially those new to Java, often struggle with this seemingly simple task. But fear not, Java provides robust tools, akin to a measuring tape, that can quickly and accurately determine the size of your ArrayList.
In this guide, we will walk you through the process of finding the length of an ArrayList in Java, from the basic use to more advanced techniques. We’ll cover everything from using the size()
method, handling more complex scenarios like multi-dimensional ArrayLists, to exploring alternative approaches.
So, let’s dive in and start mastering the length of ArrayLists in Java!
TL;DR: How Do I Find the Length of an ArrayList in Java?
The length of an ArrayList in Java can be found using the
size()
method of the ArrayList class, with the syntaxint size = arrayList.size();
. This method returns the number of elements present in the ArrayList.
Here’s a quick example:
ArrayList<String> list = new ArrayList<String>();
list.add('Hello');
int size = list.size();
System.out.println(size);
# Output:
# 1
In this example, we create an ArrayList list
and add a single element ‘Hello’ to it. Then, we use the size()
method to find the length of the ArrayList, which is 1 in this case. The size of the ArrayList is printed to the console.
This is a basic way to find the length of an ArrayList in Java, but there’s much more to learn about handling ArrayLists and dealing with more complex scenarios. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
Understanding the size()
Method
The size()
method is a built-in function in Java’s ArrayList class. It’s the most straightforward way to find the length of an ArrayList. This method returns the number of elements present in the ArrayList, effectively giving you its length.
Let’s take a look at a simple example:
ArrayList<String> myList = new ArrayList<String>();
myList.add('Hello');
myList.add('World');
int size = myList.size();
System.out.println(size);
# Output:
# 2
In this example, we first create an ArrayList myList
and add two elements to it: ‘Hello’ and ‘World’. When we use the size()
method on myList
, it returns the number of elements in the ArrayList, which is 2 in this case.
Advantages of the size()
Method
The size()
method is simple and efficient. It directly gives you the number of elements in the ArrayList, making it a quick and reliable way to find its length.
Potential Pitfalls
While the size()
method is handy, it’s important to remember that it returns the number of elements in the ArrayList, not the capacity. The capacity of an ArrayList refers to the amount of memory allocated for storing elements, regardless of whether those positions are currently holding elements or not. If you’re interested in the capacity, you’ll need to use different techniques, which we’ll cover in the advanced section.
Dealing with Multi-Dimensional ArrayLists
In Java, you can have an ArrayList of ArrayLists, also known as a multi-dimensional ArrayList. In such cases, finding the length of an individual ArrayList within this structure might be a bit tricky. Let’s look at an example:
ArrayList<ArrayList<String>> multiList = new ArrayList<>();
ArrayList<String> list1 = new ArrayList<>(Arrays.asList('Hello', 'World'));
ArrayList<String> list2 = new ArrayList<>(Arrays.asList('Java', 'Programming'));
multiList.add(list1);
multiList.add(list2);
for (ArrayList<String> list : multiList) {
System.out.println(list.size());
}
# Output:
# 2
# 2
In this example, we created a multi-dimensional ArrayList multiList
and added two ArrayLists list1
and list2
to it. Each inner ArrayList contains two elements. We then use a for-each loop to traverse through each inner ArrayList and print its size using the size()
method.
Navigating Multi-Dimensional ArrayLists
When dealing with multi-dimensional ArrayLists, it’s important to remember that each inner ArrayList is an independent entity. This means that you need to access each inner ArrayList individually to find its length.
Best Practices
While multi-dimensional ArrayLists can be useful, they can also lead to confusion if not handled properly. Always ensure that your ArrayLists are well-structured and appropriately sized for your needs. And remember, the size()
method is your friend when you need to determine the length of an ArrayList, even in a multi-dimensional context.
Exploring Java 8 Streams
Java 8 introduced a powerful new concept called Streams, which can also be used to determine the length of an ArrayList. A Stream in Java is a sequence of elements supporting sequential and parallel aggregate operations.
Using Streams to Find ArrayList Length
Let’s look at an example of how you can use Streams to find the length of an ArrayList:
ArrayList<String> myList = new ArrayList<>(Arrays.asList('Hello', 'World'));
long count = myList.stream().count();
System.out.println(count);
# Output:
# 2
In this example, we first create an ArrayList myList
with two elements. We then create a Stream from the ArrayList using the stream()
method, and use the count()
method on the Stream to get the number of elements in the ArrayList.
Advantages of Using Streams
Streams provide a more functional programming approach to handling collections, which can lead to more efficient and cleaner code. They are especially powerful when working with large collections, as they can be processed in parallel.
Disadvantages and Considerations
While Streams can be very powerful, they also come with a steeper learning curve and can lead to more complex code. Therefore, they may not always be the best choice for simple tasks like finding the length of an ArrayList. Furthermore, the count()
method on a Stream is a terminal operation, meaning it consumes the Stream and you won’t be able to perform any further operations on it.
Recommendations
While the size()
method is usually sufficient for finding the length of an ArrayList, it can be beneficial to understand and explore alternative approaches like Streams, especially if you plan on working with larger collections or need to perform more complex operations.
Troubleshooting Common Issues
While working with ArrayLists in Java, you might encounter a few common issues. Let’s discuss these problems and their solutions.
Dealing with ‘IndexOutOfBoundsException’
One of the most common issues you might face is the IndexOutOfBoundsException
. This exception is thrown to indicate that an index of some sort (such as to an ArrayList) is out of range.
ArrayList<String> myList = new ArrayList<String>();
myList.add('Hello');
System.out.println(myList.get(1));
# Output:
# Exception in thread 'main' java.lang.IndexOutOfBoundsException: Index: 1, Size: 1
In the above example, we attempt to access the second element (index 1) of an ArrayList that only has one element. This results in an IndexOutOfBoundsException
.
Solution
To avoid this issue, always ensure that the index you’re trying to access is within the range of the ArrayList’s size. You can do this by checking the size of the ArrayList using the size()
method before attempting to access an element.
ArrayList<String> myList = new ArrayList<String>();
myList.add('Hello');
if (myList.size() > 1) {
System.out.println(myList.get(1));
} else {
System.out.println('Index out of bounds');
}
# Output:
# Index out of bounds
In this modified example, we first check if the ArrayList’s size is greater than 1 before attempting to access the second element. If the size is not greater than 1, we print ‘Index out of bounds’ to the console, avoiding the IndexOutOfBoundsException
.
Tips for Working with ArrayList Length in Java
Here are a few additional tips to keep in mind when working with the length of ArrayLists in Java:
- Always check the size of the ArrayList before attempting to access an element to avoid
IndexOutOfBoundsException
. - Remember that the
size()
method returns the number of elements in the ArrayList, not its capacity. - Be mindful of the difference between the length of an array and the size of an ArrayList. While they may seem similar, they are not interchangeable.
Understanding Java’s ArrayList Class
Before we move further, let’s take a step back and understand what an ArrayList is. In Java, an ArrayList is a part of the Java Collection Framework and is present in java.util package. It provides us with dynamic arrays in Java. Unlike standard arrays, ArrayLists in Java are dynamic and can be resized during runtime.
Why is Knowing the Length Important?
Knowing the length of an ArrayList is crucial in many scenarios. It helps you understand how many elements are currently stored in the list. This information is particularly useful when you’re iterating over the ArrayList, performing operations on each element, or when you’re trying to access elements at specific indices.
ArrayList<String> myList = new ArrayList<>(Arrays.asList('Hello', 'World', 'Java'));
for (int i = 0; i < myList.size(); i++) {
System.out.println(myList.get(i));
}
# Output:
# Hello
# World
# Java
In the above example, we use the size()
method to set the limit for our loop. This ensures that we only iterate over the indices that contain elements, preventing an IndexOutOfBoundsException
.
The Underlying Data Structure of ArrayList
An ArrayList in Java is internally backed by an array. When elements are added to an ArrayList, it dynamically resizes itself by creating a new, larger array and copying the old elements to the new array. This is why ArrayLists can dynamically grow and shrink at runtime, unlike standard arrays.
Understanding these fundamentals of the ArrayList class in Java will help you better comprehend why and how we determine its length.
The Relevance of ArrayList Length in Larger Projects
Understanding the length of an ArrayList is not just important for simple Java programs; it’s also a crucial aspect when working with larger projects or scripts. Whether you’re dealing with complex data structures, building web applications, or creating multi-threaded programs, knowing the size of your ArrayLists can significantly impact your code’s performance and efficiency.
Exploring Related Concepts
While this guide focuses on the ArrayList, Java offers a variety of other data structures that you might find useful, such as LinkedList and Set. Each of these structures has its own unique characteristics and use cases.
- LinkedList: Unlike ArrayLists, LinkedLists store elements in a doubly-linked list. This means that each element in the list holds a reference to the next element and the previous one, making it easy to add or remove elements from any position in the list.
Set: A Set is a collection that doesn’t allow duplicate elements. It’s useful when you want to store a collection of items, but you’re only interested in whether a particular item exists, not in its order or frequency.
Exploring these related concepts can help broaden your understanding of Java’s collection framework and equip you with the tools to choose the right data structure for your specific needs.
Further Resources for Java Collections
To deepen your understanding of ArrayLists and other Java data structures, here are some external resources you may find useful:
- IOFlood’s Java ArrayList Article – Learn about ArrayList’s underlying data structure and its impact on performance.
How to Sort ArrayList in Java – Master organize ArrayLists data various processing tasks in Java.
Adding Elements to ArrayList in Java – Discover different ways to add elements to ArrayLists in Java.
Oracle’s Official Java Documentation covers all aspects of Java’s collection framework.
Baeldung’s Guide on Java Collections includes info on ArrayLists, LinkedLists, Sets, and more.
Java Code Geeks is a great resource for Java tutorials and articles.
Wrapping Up:
In this comprehensive guide, we’ve delved into the process of determining the length of an ArrayList in Java. We’ve explored the use of the size()
method, dealt with multi-dimensional ArrayLists, and even ventured into alternative approaches like Java 8 Streams.
We began with the basics, learning how to use the size()
method to quickly and effectively determine the length of an ArrayList. We then moved into more complex territory, tackling the challenge of finding the length of an ArrayList within a multi-dimensional ArrayList. We also explored alternative approaches, such as using Java 8 Streams, providing you with a broader toolkit for handling this task.
Along the way, we addressed common issues you might encounter, such as ‘IndexOutOfBoundsException’, and provided solutions and tips to help you avoid these pitfalls. We also discussed the importance of knowing the length of an ArrayList and the underlying data structure of ArrayList.
Here’s a quick comparison of the methods we’ve discussed:
Method | Ease of Use | Complexity | Use Case |
---|---|---|---|
size() Method | High | Low | Simple scenarios |
Multi-Dimensional ArrayList | Moderate | High | Complex structures |
Java 8 Streams | Low | High | Large collections |
Whether you’re just starting out with Java or you’re an experienced developer looking to brush up on your skills, we hope this guide has given you a deeper understanding of how to find the length of an ArrayList in Java.
Understanding the length of an ArrayList is a fundamental part of working with Java collections. With this knowledge, you’re well-equipped to handle ArrayLists more effectively in your future projects. Happy coding!