Python Iterator Mastery: Your Complete Guide
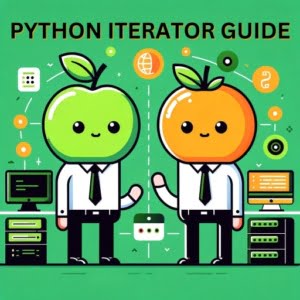
Exploring Python iterators and their usage is essential while scripting on Linux servers at IOFLOOD, enabling efficient data traversal and processing. In our experience, Python iterators offer a modular approach to iterative tasks, enhancing code organization and maintainability. Today’s article delves into what a Python iterator is, how it works, and how to effectively use it, providing examples and insights to empower our dedicated server customers with effective coding practices.
This guide will walk you through the concept of iterators in Python, their usage, and how to create your own. We’ll start from the basics, gradually moving onto more advanced topics. Whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you.
So, let’s dive in and start mastering Python iterators!
TL;DR: What is a Python Iterator and How Do I Use It?
A Python iterator is an object that implements the iterator protocol, which consists of the
__iter__()
and__next__()
methods. To use an iterator, first create an iterator object using theiter()
function, then retrieve items using thenext()
function.
Here’s a simple example of using an iterator:
my_tuple = ('apple', 'banana', 'cherry')
my_iter = iter(my_tuple)
print(next(my_iter))
print(next(my_iter))
print(next(my_iter))
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, we’ve created a tuple and then used the iter()
function to create an iterator. We then use the next()
function to print each item in the tuple one by one.
This is just the tip of the iceberg when it comes to Python iterators. Keep reading for a deeper understanding of Python iterators, including how to create your own.
Table of Contents
- Unraveling Python Iterators: The Basics
- Crafting Your Own Python Iterator
- Exploring Alternatives: Python Generator Functions and Expressions
- Navigating Pitfalls: Troubleshooting Python Iterators
- Python’s Iterator Protocol: A Deep Dive
- Python Iterators in Real-World Applications
- Wrapping Up: Mastering Python Iterators
Unraveling Python Iterators: The Basics
Python’s built-in iterator functions, such as iter()
and next()
, are the stepping stones to understanding and effectively using iterators. Let’s delve into how they work.
The iter()
Function
The iter()
function takes an iterable object (like a list, tuple, or string) and returns an iterator. Here’s an example:
my_list = ['apple', 'banana', 'cherry']
my_iter = iter(my_list)
print(my_iter)
# Output:
# <list_iterator object at 0x7f8f4873c1f0>
In this example, we’ve created a list and then used the iter()
function to create an iterator. The print()
function returns a list_iterator object, which is the iterator we just created.
The next()
Function
The next()
function retrieves the next item from the iterator. If there are no more items to retrieve, it raises a ‘StopIteration’ error. Here’s how you can use it:
print(next(my_iter))
print(next(my_iter))
print(next(my_iter))
# Output:
# 'apple'
# 'banana'
# 'cherry'
Here, we’ve used the next()
function to print each item in the list one by one. Once all items have been processed, the iterator is exhausted, and any further calls to next()
will raise a ‘StopIteration’ error.
These built-in functions are powerful tools in Python, but it’s important to handle them properly to avoid errors. In the upcoming sections, we’ll discuss more advanced uses of iterators and how to handle potential pitfalls.
Crafting Your Own Python Iterator
As you become more comfortable with Python iterators, you might find yourself wanting to create your own. This is where Python’s magic methods __iter__()
and __next__()
come into play. These methods allow you to define your own iterator objects.
The __iter__()
Method
The __iter__()
method returns the iterator object itself. If required, some initialization can be performed.
The __next__()
Method
The __next__()
method must return the next item in the sequence. On reaching the end, and in subsequent calls, it must raise StopIteration.
Let’s look at an example of how to create your own iterator class:
class CountDown:
def __init__(self, start):
self.current = start
def __iter__(self):
return self
def __next__(self):
if self.current <= 0:
raise StopIteration
else:
self.current -= 1
return self.current + 1
# Using the iterator
for number in CountDown(5):
print(number)
# Output:
# 5
# 4
# 3
# 2
# 1
In this example, we’ve defined a class CountDown
that starts counting down from a number specified by the user. The __iter__()
method returns the iterator object (in this case, self
). The __next__()
method decreases the current number and returns the previous number. If the current number is less than or equal to zero, it raises a StopIteration, signaling that all values have been returned.
By defining your own iterator class, you gain more control over the iteration process and can customize it to suit your specific needs.
Exploring Alternatives: Python Generator Functions and Expressions
While Python’s built-in iterator methods and creating your own iterator class are powerful tools, there are alternative approaches that can offer additional benefits. Specifically, Python’s generator functions and expressions provide an alternative way to create iterators.
Generator Functions
A generator function is a special kind of function that returns an iterator. It looks like a normal function except that it contains yield
expressions for producing a series of values usable in a for-loop or that can be retrieved one at a time with the next()
function.
def countdown(num):
print('Starting countdown')
while num > 0:
yield num
num -= 1
# Using the generator function
for number in countdown(5):
print(number)
# Output:
# Starting countdown
# 5
# 4
# 3
# 2
# 1
In this example, countdown
is a generator function that produces a series of values from a given start number down to 1. The yield
keyword is used to produce a value and suspend the function’s execution. The function resumes where it left off when subsequent next()
calls are made.
Generator Expressions
Generator expressions are a high-performance, memory–efficient generalization of list comprehensions and generators. Here’s an example:
numbers = (number for number in range(5, 0, -1))
for number in numbers:
print(number)
# Output:
# 5
# 4
# 3
# 2
# 1
In this example, we’ve created a generator expression that produces the numbers from 5 down to 1. Generator expressions are a compact way to create iterators, but they can only be iterated over once.
Both generator functions and expressions are powerful tools, but they come with their own trade-offs. Generator functions offer more flexibility but are more verbose. Generator expressions are more compact but less flexible. Your choice between the two will depend on your specific needs.
As with any coding concept, working with iterators in Python can sometimes lead to issues. One common error you may encounter is the ‘StopIteration’ error. But don’t worry – with a bit of understanding and the right approach, these issues can be easily resolved.
Understanding the ‘StopIteration’ Error
The ‘StopIteration’ error is raised when there are no more elements to retrieve using the next()
function. It signals that all values have been returned and the iteration is complete. Here’s an example:
my_list = ['apple', 'banana', 'cherry']
my_iter = iter(my_list)
print(next(my_iter))
print(next(my_iter))
print(next(my_iter))
print(next(my_iter))
# Output:
# 'apple'
# 'banana'
# 'cherry'
# StopIteration
In this example, we’ve exhausted the iterator by retrieving all items from the list. When we try to call next()
again, it raises a ‘StopIteration’ error.
Handling the ‘StopIteration’ Error
A good way to handle the ‘StopIteration’ error is by using a for
loop or the itertools
module’s islice
function. The for
loop automatically catches this exception and stops calling next()
. Here’s how you can do it:
my_list = ['apple', 'banana', 'cherry']
my_iter = iter(my_list)
for item in my_iter:
print(item)
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, the for
loop automatically calls next(my_iter)
at each iteration. When there are no more items to retrieve, it catches the ‘StopIteration’ error and breaks the loop.
Understanding and handling potential issues when working with Python iterators can save you a lot of debugging time and make your coding process more efficient.
Python’s Iterator Protocol: A Deep Dive
To truly master Python iterators, it’s essential to understand the underlying protocol that drives them. This is known as Python’s iterator protocol, a simple yet powerful concept that forms the basis of all iteration in Python.
Iterable vs Iterator: What’s the Difference?
In Python, an iterable is an object capable of returning its members one at a time. Lists, tuples, strings, dictionaries, and sets are examples of iterable objects. An iterator, on the other hand, is an object that iterates over an iterable using the __iter__()
and __next__()
methods.
# A list is an iterable
my_list = ['apple', 'banana', 'cherry']
# An iterator is an object that iterates over the iterable
my_iter = iter(my_list)
print(next(my_iter))
print(next(my_iter))
print(next(my_iter))
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, my_list
is an iterable, and my_iter
is an iterator that iterates over my_list
.
Python’s For Loop and Iterators
Under the hood, Python’s for
loop uses iterators to loop over the iterable. It first calls iter()
to get an iterator, then calls next()
to get each item from the iterator.
# A for loop in Python
for item in my_list:
print(item)
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, the for
loop is internally using an iterator to loop over my_list
.
Understanding these fundamental concepts is key to mastering Python iterators. With this knowledge, you can manipulate data in Python more efficiently and effectively.
Python Iterators in Real-World Applications
Python iterators, while seemingly simple, are powerful tools that can be applied in a variety of real-world coding scenarios. They are used in list comprehensions, generator expressions, and built-in functions like map()
and filter()
, to name a few.
Iterators in List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. Under the hood, they use iterators to iterate over the original list.
numbers = [1, 2, 3, 4, 5]
squares = [number**2 for number in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, we’ve used a list comprehension to create a new list (squares
) based on an existing list (numbers
). The iterator (number in numbers
) is used to iterate over the original list.
Iterators in Generator Expressions
Like list comprehensions, generator expressions also use iterators. However, they return a generator object that can be iterated over lazily, providing potential performance benefits.
numbers = (number**2 for number in range(6))
for number in numbers:
print(number)
# Output:
# 0
# 1
# 4
# 9
# 16
# 25
In this example, we’ve created a generator expression that produces the squares of the numbers from 0 to 5. The iterator (number in range(6)
) is used to iterate over the range.
Iterators in Built-in Functions
Python’s built-in functions like map()
and filter()
also use iterators. The map()
function applies a given function to each item of an iterable and returns a list of the results. The filter()
function constructs a list from elements of an iterable for which a function returns true.
numbers = [1, 2, 3, 4, 5]
doubles = list(map(lambda x: x * 2, numbers))
print(doubles)
# Output:
# [2, 4, 6, 8, 10]
In this example, we’ve used the map()
function to double each item in the list. The iterator (x in numbers
) is used to iterate over the original list.
Further Resources for Python Iterator Mastery
For those interested in delving deeper into Python iterators, the following resources offer more in-depth information:
- IOFlood’s tutorial on Python Loops teaches you how loops can help with iteration and data comprehension.
Using the “next()” Function – Learn about iterator exhaustion and handling StopIteration with “next.”
Python Loop Control with “continue” – Learn how to use “continue” for efficient loop processing.
Python’s Official Documentation on Iterators provides an overview of iterator classes in Python.
Guide to Python Iterators on Real Python delves into Python’s iter tools module.
Python Iterators – A beginner-friendly tutorial on DataCamp that introduces the concept of iterators in Python.
Wrapping Up: Mastering Python Iterators
In this comprehensive guide, we’ve delved into the concept of Python iterators, explored their usage, and learned how to create our own.
We began with the basics, understanding the built-in iterator functions like iter()
and next()
. We then progressed to creating our own iterator objects by defining __iter__()
and __next__()
methods in our class. Along the way, we encountered and learned how to handle common issues such as the ‘StopIteration’ error.
We also explored alternative approaches to creating iterators, including Python’s generator functions and expressions. We compared these methods, discussing their benefits, drawbacks, and decision-making considerations. Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Built-in Functions | Low | Low | Simple Iterations |
Custom Iterator Class | High | High | Customized Iterations |
Generator Functions | Moderate | Moderate | Large Data Sets |
Generator Expressions | Low | Low | One-time Iterations |
Whether you’re a beginner just starting out with Python iterators or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python iterators and their capabilities. With this knowledge, you can manipulate data in Python more efficiently and effectively. Happy coding!