The Best Python Boolean Guide: True or False?
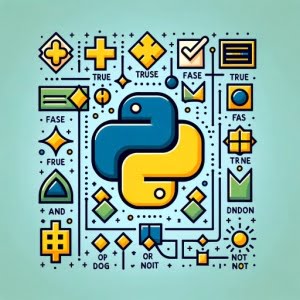
Are you finding it challenging to understand booleans in Python? You’re not alone. Many developers, especially those new to Python, often find themselves puzzled when it comes to understanding and using booleans effectively.
Think of Python’s boolean as a light switch – it can only have two states – True or False.
In this guide, we’ll walk you through the process of using booleans in Python, from the basics to more advanced techniques. We’ll cover everything from declaring a boolean, using booleans in conditional statements, to more complex uses such as logical operations and list comprehensions.
Let’s get started!
TL;DR: What is a Boolean in Python?
In Python, a boolean is a built-in data type that can take up two values: True and False. It’s a fundamental concept in Python and is used extensively in conditional and control flow statements.
Here’s a simple example:
is_active = True
is_inactive = False
print(is_active, is_inactive)
# Output:
# True False
In this example, we’ve declared two boolean variables: is_active
and is_inactive
. We’ve assigned the value True
to is_active
and False
to is_inactive
. When we print these variables, we get their respective boolean values as output.
This is just the tip of the iceberg when it comes to using booleans in Python. There’s much more to learn, including how to use booleans in conditional statements, logical operations, and more. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Understanding Python Booleans: The Basics
The concept of booleans is fundamental in Python. As we mentioned earlier, a boolean in Python can have two values: True
or False
. Let’s dive deeper and see how we can use booleans in Python, particularly in conditional statements.
Declaring a Boolean
First things first, let’s see how to declare a boolean variable in Python. Here’s a simple example:
is_active = True
is_inactive = False
print(is_active, is_inactive)
# Output:
# True False
In this code, we’ve declared two boolean variables: is_active
and is_inactive
. We’ve assigned the value True
to is_active
and False
to is_inactive
. When we print these variables, we get their respective boolean values as output. Simple, right?
Using Booleans in Conditional Statements
One of the most common uses of booleans in Python is in conditional statements. Let’s see how:
is_active = True
if is_active:
print('The user is active.')
else:
print('The user is not active.')
# Output:
# The user is active.
In this example, we first declare a boolean variable is_active
and assign it the value True
. We then use this boolean in an if
statement. If is_active
is True
, the message ‘The user is active.’ is printed. If is_active
is False
, the message ‘The user is not active.’ is printed.
Booleans are incredibly useful in such scenarios where you need to check a condition and execute code based on whether the condition is true or false. Understanding how to use booleans in such a way is a critical skill for any Python developer.
Python Booleans in Logical Operations
As you get more comfortable with Python booleans, you can start using them in more complex scenarios. One such use case is in logical operations. Python supports three logical operations: and
, or
, and not
.
Booleans in ‘and’ Operations
In an and
operation, if both operands are true, the result is True
. Otherwise, it’s False
. Here’s an example:
is_active = True
is_verified = True
if is_active and is_verified:
print('The user is active and verified.')
else:
print('The user is not active or not verified.')
# Output:
# The user is active and verified.
In this example, both is_active
and is_verified
are True
. Hence, the and
operation returns True
, and ‘The user is active and verified.’ is printed.
Booleans in ‘or’ Operations
In an or
operation, if at least one of the operands is true, the result is True
. If both are false, the result is False
.
is_active = True
is_verified = False
if is_active or is_verified:
print('The user is active or verified.')
else:
print('The user is neither active nor verified.')
# Output:
# The user is active or verified.
In this example, is_active
is True
, and is_verified
is False
. Hence, the or
operation returns True
, and ‘The user is active or verified.’ is printed.
Booleans in ‘not’ Operations
The not
operation in Python negates the boolean value. If the operand is True
, not
returns False
and vice versa.
is_active = True
if not is_active:
print('The user is not active.')
else:
print('The user is active.')
# Output:
# The user is active.
In this example, is_active
is True
. The not
operation negates this, returning False
. Hence, ‘The user is active.’ is printed.
Understanding these logical operations and how to use them with booleans can greatly enhance your Python programming skills.
Alternative Methods: Using Booleans in Python
As you become more proficient in Python, you’ll discover there are several ways to use booleans that can help you write more efficient, readable code. Let’s explore some of these methods.
The bool() Function
In Python, you can use the bool()
function to convert a value to a boolean. This function returns False
if the value is omitted or false, and True
otherwise.
print(bool(0))
print(bool(1))
print(bool([]))
print(bool([1, 2, 3]))
# Output:
# False
# True
# False
# True
In this example, we use the bool()
function to convert different values to booleans. The function returns False
for 0
and an empty list []
(as these are considered false in Python), and True
for 1
and [1, 2, 3]
.
Booleans in List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. You can use booleans in list comprehensions to filter out values.
numbers = [1, 2, 3, 4, 5]
even_numbers = [number for number in numbers if number % 2 == 0]
print(even_numbers)
# Output:
# [2, 4]
In this example, we have a list of numbers. We use a list comprehension with a conditional statement to create a new list even_numbers
that only includes the even numbers from the original list.
These alternative methods provide more flexibility when dealing with booleans in Python. They can help you write more concise and efficient code, especially as your programs become more complex.
Troubleshooting Python Boolean Issues
Working with booleans in Python is generally straightforward, but you may occasionally run into some common issues. Let’s discuss these and provide some solutions and workarounds.
Unexpected Boolean Values
One common issue is getting unexpected boolean values. This usually happens when you use the bool()
function on different data types.
print(bool(''))
print(bool('Hello'))
print(bool([]))
print(bool([1, 2, 3]))
# Output:
# False
# True
# False
# True
In this example, the bool()
function returns False
for an empty string ''
and an empty list []
, and True
for the non-empty string 'Hello'
and list [1, 2, 3]
. This is because in Python, empty sequences and collections (like lists and strings) are considered False
, while non-empty ones are considered True
.
Boolean Operations Returning Unexpected Results
Another common issue is boolean operations returning unexpected results. This can occur when you’re not familiar with Python’s rules for boolean operations.
print(True and 'Hello')
print(False and 'Hello')
print(True or 'Hello')
print(False or 'Hello')
# Output:
# Hello
# False
# True
# Hello
In this example, the and
operation returns the second operand if the first is True
, and the first operand if it’s False
. The or
operation returns the first operand if it’s True
, and the second operand if it’s False
.
Understanding these quirks can help you avoid common pitfalls when working with booleans in Python. Always remember to test your code thoroughly to ensure it behaves as expected.
Unpacking Python’s Boolean Data Type
To fully grasp how booleans work in Python, it’s essential to understand their origin and role in Python programming.
Origin of Python’s Boolean
The boolean data type in Python, named after George Boole, is a direct manifestation of Boolean Algebra. It’s a fundamental data type in Python and many other programming languages, and it represents the truth value of an expression.
print(type(True))
print(type(False))
# Output:
# <class 'bool'>
# <class 'bool'>
In this example, we use the type()
function to check the data type of True
and False
. As you can see, both are of the type bool
, short for boolean.
Role in Python Programming
Booleans play a crucial role in Python programming. They are primarily used in conditional statements and loops, which are the building blocks of any Python program.
is_active = True
if is_active:
print('The user is active.')
# Output:
# The user is active.
In this example, we use a boolean is_active
in an if
statement to check whether a user is active.
Truthy and Falsy Values in Python
In Python, values are considered True
or False
in a boolean context. This concept is known as truthy and falsy values.
print(bool(1))
print(bool(0))
print(bool('Hello'))
print(bool(''))
# Output:
# True
# False
# True
# False
In this example, 1
and 'Hello'
are truthy values, so they return True
. On the other hand, 0
and ''
(empty string) are falsy values, so they return False
.
Understanding these fundamentals can help you use booleans effectively in your Python programs.
Broadening Your Python Boolean Knowledge
Python booleans are not limited to conditional statements and logical operations. They have wider applications that can significantly impact your Python programming.
Booleans in Control Flow
Control flow is a fundamental concept in Python that allows your program to execute different code blocks based on specific conditions. Booleans play a crucial role in this.
is_active = True
if is_active:
print('Start processing.')
else:
print('Wait for the user to become active.')
# Output:
# Start processing.
In this example, the boolean is_active
controls which code block gets executed. If is_active
is True
, ‘Start processing.’ is printed. If is_active
is False
, ‘Wait for the user to become active.’ is printed.
Booleans in Data Filtering
Data filtering is another area where booleans are incredibly useful. You can use booleans to filter out specific data from a dataset.
numbers = [1, 2, 3, 4, 5]
even_numbers = [number for number in numbers if number % 2 == 0]
print(even_numbers)
# Output:
# [2, 4]
In this example, we use a boolean in a list comprehension to filter out the even numbers from a list.
Exploring Related Concepts
Understanding booleans can also help you grasp related concepts like conditional statements and logical operators. These are fundamental concepts in Python that you’ll use frequently in your programs.
Further Resources for Python Boolean Mastery
To learn more about Python booleans and conditional statements, check out these resources:
- Python If Statements for Beginners – A Hands-On Tutorial on if statements and their role in event handling and GUI programming.
Advanced Assignment with the Walrus Operator – Explore Python’s walrus operator (:=) and its role in assignment expressions.
Logical AND in Python: Combining Conditions – Learn how the “and” operator works for combining Python boolean conditions.
The Official Python Documentation: Boolean Operations provides an overview of booleans and operators in Python.
Python Boolean Type – A detailed guide by Real Python that thoroughly covers the boolean type in Python.
Python Booleans: The Definitive Guide – An exhaustive guide by W3Schools that focuses on understanding and working with booleans in Python.
These resources provide in-depth explanations and examples that can help you master Python booleans.
Wrapping Up: Python Booleans Unraveled
In this comprehensive guide, we’ve explored the intriguing world of Python booleans. We’ve uncovered their basic usage, delved into advanced techniques, and even looked at some alternative approaches to handling booleans in Python. From the simple declaration of booleans to their use in logical operations, list comprehensions and beyond, we’ve covered it all.
We began with the basics, understanding how to declare a boolean and use it in simple conditional statements. We then ventured into more advanced territory, exploring how to use booleans in logical operations like and
, or
, and not
. We also learned how to use the bool()
function and implement booleans in list comprehensions.
Along the way, we tackled common issues you might encounter when working with booleans in Python, such as unexpected boolean values and boolean operations returning unexpected results. We provided solutions and workarounds for each issue to help you navigate these challenges with ease.
We also compared the standard approach of using booleans with alternative methods, giving you a broader perspective on handling booleans in Python. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Standard Boolean Usage | Simple and straightforward | Limited functionality |
Using bool() Function | Can convert different data types to booleans | Can return unexpected values for certain data types |
Using Booleans in List Comprehensions | Allows for efficient data filtering | Requires understanding of list comprehensions |
Whether you’re a beginner just starting out with Python booleans or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python booleans and their capabilities. Happy coding!