Python String format() Function Guide (With Examples)
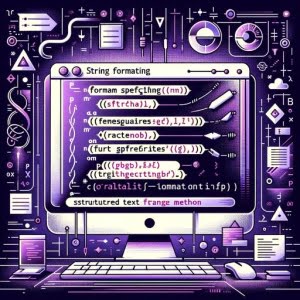
Ever found yourself wrestling with Python string formatting? Fear not, you’re not alone. Python, like a skilled typesetter, offers a multitude of ways to format strings. These tools help make your code cleaner, more readable, and efficient.
In this comprehensive guide, we’ll journey through the various methods of string formatting in Python. We’ll start from the basics, perfect for those just dipping their toes in, and gradually progress to more advanced techniques.
By the end of this guide, you’ll be able to wield Python’s string formatting functions like a pro, making your coding life easier and more enjoyable. So, let’s dive in and start mastering Python string formatting!
TL;DR: How Do I Format Strings in Python?
One common way to format strings in Python is using the
format()
function. Here’s a simple example:
name = 'John'
greeting = 'Hello, {}'.format(name)
print(greeting)
# Output:
# 'Hello, John'
In the above example, we’ve used the format()
function to insert a variable (name
) into a string (greeting
). This is a basic method of string formatting in Python, but it’s just the tip of the iceberg. Stick with us for a more detailed understanding of Python string formatting and its advanced usage.
Table of Contents
- Python String Formatting: The Basics
- Delving Deeper: Positional and Keyword Arguments in Python String Formatting
- Exploring Alternative Python String Formatting Techniques
- Troubleshooting Common Python String Formatting Issues
- Python String Formatting: The Fundamentals
- Beyond Basics: Python String Formatting in Real-world Applications
- Python String Formatting: A Recap
Python String Formatting: The Basics
The format()
function is a built-in Python method for string objects, and it’s one of the simplest ways to format strings. It allows you to insert specific values into a string in a clearly defined manner.
Let’s look at a basic example:
name = 'Alice'
age = 25
message = 'Hello, {}. You are {} years old.'.format(name, age)
print(message)
# Output:
# 'Hello, Alice. You are 25 years old.'
In this example, the format()
function is used to insert the variables name
and age
into the message
string. The {}
placeholders in the string are replaced by the arguments provided in the format()
function in the order they are given.
The format()
function is quite handy as it provides a clear and concise way to include variable data within strings. However, it’s important to note that the number of {}
placeholders in the string must match the number of arguments given to the format()
function. Failure to do so will result in an error, which is one of the potential pitfalls when using this method.
Delving Deeper: Positional and Keyword Arguments in Python String Formatting
As you become more comfortable with Python string formatting, you can start to leverage more complex techniques such as positional and keyword arguments with the format()
function.
Positional Arguments
With positional arguments, you can specify the position of your variables in the format()
function, and they will replace the placeholders accordingly. This gives you more control over the output.
Let’s see this in action:
first_name = 'Ada'
last_name = 'Lovelace'
message = 'Hello, {1}, {0}'.format(first_name, last_name)
print(message)
# Output:
# 'Hello, Lovelace, Ada'
In the above example, we’ve used positional arguments in the format()
function. The numbers inside the {}
placeholders refer to the positions of the arguments in the format()
function. {1}
refers to the second argument (last_name
), and {0}
refers to the first argument (first_name
). This allows us to switch the order of the variables in the output string.
Keyword Arguments
Keyword arguments take this a step further by allowing you to assign values to specific keywords, which can then be used as placeholders in your string.
Here’s an example:
message = 'Hello, {first_name}, {last_name}'.format(first_name='Alan', last_name='Turing')
print(message)
# Output:
# 'Hello, Alan, Turing'
In this case, we’ve assigned the values ‘Alan’ and ‘Turing’ to the keywords first_name
and last_name
respectively. These keywords are then used as placeholders in the string. This provides a high level of readability and clarity to your code, making it easier to understand and maintain.
Exploring Alternative Python String Formatting Techniques
Python offers several other methods for string formatting, including f-strings and the percent (%) operator. These techniques can provide more efficient or readable ways to format strings, depending on the situation.
F-Strings
F-strings, introduced in Python 3.6, provide a concise and convenient way to embed expressions inside string literals for formatting. Let’s see an example:
first_name = 'Grace'
last_name = 'Hopper'
message = f'Hello, {first_name} {last_name}'
print(message)
# Output:
# 'Hello, Grace Hopper'
In this example, we’ve used an f-string (denoted by the f
before the string) to embed the first_name
and last_name
variables directly into the string. This can make your code cleaner and easier to read, especially for complex strings.
The Percent (%) Operator
The percent (%) operator is another method for formatting strings in Python. It’s similar to the syntax used in C’s printf
function. Here’s how it works:
name = 'Guido'
age = 64
message = 'Hello, %s. You are %d years old.' % (name, age)
print(message)
# Output:
# 'Hello, Guido. You are 64 years old.'
In this case, we’ve used %s
and %d
as placeholders for a string and an integer, respectively. The variables are then provided in a tuple after the %
operator. While this method is less commonly used in modern Python coding, it can still be useful in certain situations, particularly when dealing with older codebases.
Each of these methods has its advantages and disadvantages, and the best one to use depends on your specific situation. F-strings can provide a high level of readability and efficiency, especially for complex strings. The format()
function and the percent (%) operator offer more control over the formatting, which can be useful for more complex formatting requirements. It’s recommended to be familiar with all these methods, as they each have their place in a Python programmer’s toolkit.
Troubleshooting Common Python String Formatting Issues
As with any coding technique, Python string formatting can sometimes lead to unexpected errors. Two of the most common are TypeError
and ValueError
. Let’s explore these issues and discuss ways to resolve them.
Handling TypeError
A TypeError
often occurs when you try to combine incompatible types. For instance, attempting to concatenate a string and an integer without proper formatting will result in a TypeError
.
age = 25
message = 'Your age is ' + age
# Output:
# TypeError: can only concatenate str (not "int") to str
In this case, Python is expecting a string to concatenate with ‘Your age is ‘, but it received an integer (age
). The format()
function or f-string formatting can help prevent this issue:
age = 25
message = 'Your age is {}'.format(age)
print(message)
# Output:
# 'Your age is 25'
Resolving ValueError
A ValueError
is typically raised when a function receives an argument of the correct type, but an inappropriate value. For example, this could occur if you provide more or fewer arguments to the format()
function than there are placeholders in the string.
name = 'Alice'
message = 'Hello, {}'.format(name, 'Bob')
# Output:
# ValueError: too many arguments for format string
In the above example, we’ve provided two arguments to the format()
function, but only one placeholder in the string. To resolve this issue, ensure that the number of arguments matches the number of placeholders in the string.
Understanding these common issues and how to resolve them can help you avoid pitfalls and become more proficient with Python string formatting. Remember, practice makes perfect!
Python String Formatting: The Fundamentals
To fully grasp Python string formatting, it’s essential to understand the basics of Python’s string data type and how string formatting works.
Python’s String Data Type
In Python, a string is a sequence of characters. It can be declared using single, double, or triple quotes.
# String declaration in Python
str1 = 'Hello, World!'
str2 = "Hello, World!"
str3 = '''Hello,
World!'''
print(str1)
print(str2)
print(str3)
# Output:
# Hello, World!
# Hello, World!
# Hello,
# World!
In the above code, str1
and str2
are examples of single-line strings, while str3
is a multi-line string.
The Importance of String Formatting
String formatting is crucial in Python for several reasons:
- Readability: Properly formatted strings are easier to read and understand, making your code more maintainable.
Efficiency: String formatting allows you to dynamically insert values into a string, saving time and reducing the potential for errors.
Flexibility: Python’s string formatting techniques provide a high level of flexibility, allowing you to control how and where values are inserted into a string.
Understanding the fundamentals of Python’s string data type and the importance of string formatting is the first step towards mastering Python string formatting. With this foundation, you can better appreciate the power and flexibility that Python’s string formatting techniques bring to your coding toolbox.
Beyond Basics: Python String Formatting in Real-world Applications
Python string formatting is not just a theoretical concept, but a practical tool that you’ll use in various Python applications.
Data Processing
In data processing, you often need to format strings to present data in a readable and understandable format. This could involve inserting values into a string, formatting numbers to a certain number of decimal places, or even generating dynamic SQL queries.
# Formatting numbers for data presentation
pi = 3.14159
message = 'The value of pi to 2 decimal places is {:.2f}'.format(pi)
print(message)
# Output:
# The value of pi to 2 decimal places is 3.14
In the above example, we’ve used the format()
function to format the value of pi to 2 decimal places.
File Handling
Python string formatting can also be useful in file handling operations. For instance, you might need to create file names dynamically based on certain parameters.
# Creating a file name dynamically
file_number = 5
file_name = 'output_file_{:03d}.txt'.format(file_number)
print(file_name)
# Output:
# 'output_file_005.txt'
In this case, we’ve used string formatting to generate a file name with a three-digit file number, padded with zeros if necessary.
Further Resources for Python Strings
As you continue your Python journey, you might want to explore related concepts such as string methods and regular expressions. These can provide even more flexibility and control over your string manipulation tasks.
Python’s string methods include functions like split()
, join()
, replace()
, and many more. Regular expressions, on the other hand, provide a powerful way to match and manipulate strings based on complex patterns.
For deeper understanding and further study, here are a few resources that you might find helpful:
- Python Strings: Building Foundation for Text-Intensive Applications: Build a solid foundation for developing text-intensive applications by mastering Python strings.
Python String Contains: Checking if a String Contains a Substring: This article explains different methods to check if a string contains a substring in Python, including the use of in operator and the find() method.
Python String Interpolation: Formatting Strings in Python: Learn how to interpolate variables and expressions into strings using different string interpolation techniques in Python, such as f-strings, format(), and %-formatting.
Python String format() Method: A Comprehensive Guide: A comprehensive guide on w3schools.com that explains the format() method in Python, used for formatting strings with placeholders and variables.
String Formatting in Python: GeeksforGeeks provides tutorials and examples on string formatting in Python, including different formatting techniques and placeholders.
Python String Formatting: A Practical Guide: Real Python offers a practical guide on string formatting in Python, covering various formatting options and best practices.
Python String Formatting: A Recap
Throughout this guide, we’ve explored the ins and outs of Python string formatting. From the basic format()
function, handling positional and keyword arguments, to the more advanced techniques like f-strings and the percent (%) operator, we’ve covered a wide range of methods to handle string formatting in Python.
Let’s summarize the key points:
- The
format()
function is a versatile tool for inserting variables into strings. It’s simple to use and provides clear and concise code. Positional and keyword arguments with the
format()
function provide more control over the formatting, allowing you to specify the order of variables in the string or assign values to specific keywords.F-strings offer a concise and convenient way to embed expressions into strings, which can enhance code readability, especially for complex strings.
The percent (%) operator, while less commonly used in modern Python coding, can still be beneficial in certain situations, particularly when dealing with older codebases.
Common issues like
TypeError
andValueError
can arise from misusing these string formatting techniques. Understanding these issues and how to resolve them can help you avoid pitfalls in your coding journey.String formatting plays a crucial role in real-world Python applications, including data processing and file handling.
Here’s a quick comparison of the different methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
format() function | Clear and concise, provides control over formatting | Can be verbose for complex strings |
F-strings | Concise and readable, especially for complex strings | Not available in Python versions before 3.6 |
Percent (%) operator | Provides control over formatting, useful for older codebases | Less readable compared to other methods |
Remember, the best method to use depends on your specific situation and requirements. By understanding these different techniques, you’re now well-equipped to handle any Python string formatting task that comes your way. Keep practicing, keep exploring, and happy coding!