Python String Contains | Methods and Usage Examples
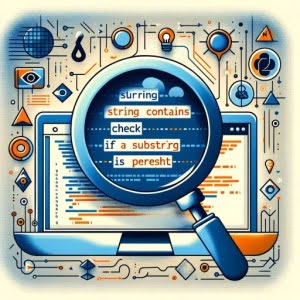
Ever wondered how to check if a string contains a certain substring in Python? It’s like being a word detective, sifting through lines of text to find that one clue (substring) hidden in the larger narrative (string).
Python, with its powerful and diverse set of tools, makes this task a breeze. Whether you’re a beginner just starting out or an experienced programmer looking for advanced techniques, this comprehensive guide is your roadmap to mastering the art of finding substrings in Python.
TL;DR: How Do I Check If a String Contains a Substring in Python?
You can use the
in
keyword in Python to check if a string contains a certain substring. Here’s a simple example:
text = 'Hello, World!'
print('World' in text)
# Output:
# True
In this example, we have a string ‘Hello, World!’ and we are checking if it contains the substring ‘World’. The in
keyword in Python returns True
if the substring exists in the string, and False
otherwise. In our case, since ‘World’ is indeed a part of ‘Hello, World!’, the output is True
.
Stick around for more detailed information and advanced usage scenarios. We’re just getting started!
Table of Contents
Python’s in
Keyword: The Substring Detective
Python’s in
keyword is a simple yet powerful tool when it comes to checking if a string contains a certain substring. It’s straightforward, easy to use, and gets the job done with minimal fuss.
Let’s dive into a basic example:
sentence = 'Python is fun!'
word = 'fun'
print(word in sentence)
# Output:
# True
In this example, we’re checking if the word ‘fun’ is in the sentence ‘Python is fun!’. The in
keyword checks for the presence of ‘fun’ in ‘Python is fun!’ and returns True
since ‘fun’ is indeed a part of our sentence.
The in
keyword essentially scans the string from start to end, looking for the substring. If it finds the substring, it stops scanning and returns True
. If it doesn’t find the substring by the end of the string, it returns False
.
One of the main advantages of the in
keyword is its simplicity and readability. It’s also pretty fast, especially for shorter strings and substrings.
However, it’s worth noting that the in
keyword is case-sensitive. This means that ‘Fun’ and ‘fun’ are considered different substrings. If case-insensitive checks are what you need, you’ll have to convert both the string and substring to the same case (either lower or upper) before using the in
keyword.
Stay tuned for more advanced techniques and alternative approaches to checking if a Python string contains a substring.
Mastering Case-Insensitive Checks and Regex
As you progress in your Python journey, you’ll encounter scenarios where a simple in
keyword check might not suffice. For instance, what if you want to check for a substring in a case-insensitive manner? Or what if you need to match a pattern rather than a specific substring? Python has got you covered.
Case-Insensitive Checks
Python’s lower()
or upper()
methods can be used to convert both the string and the substring to the same case before performing the check. Here’s how you do it:
sentence = 'Python is Fun!'
word = 'fun'
print(word.lower() in sentence.lower())
# Output:
# True
In this example, we’re checking if the word ‘fun’ is in the sentence ‘Python is Fun!’ in a case-insensitive manner. By converting both the string and the substring to lowercase, we ensure that the check is not affected by the letter case.
Pattern Matching with Regular Expressions
Sometimes, you might need to check if a string matches a certain pattern. This is where regular expressions come into play. Python’s re
module provides the search()
function for this purpose.
import re
sentence = 'Python is fun!'
pattern = 'fun'
print(bool(re.search(pattern, sentence)))
# Output:
# True
In this example, we’re using the re.search()
function to check if the pattern ‘fun’ exists in the sentence ‘Python is fun!’. The function returns a match object if the pattern is found, and None
otherwise. By casting the result to bool
, we get a True
or False
value similar to the in
keyword.
Regular expressions are incredibly powerful and flexible, but they can be a bit tricky to get the hang of. They’re a great tool to have in your arsenal when dealing with more complex string matching scenarios.
In the next section, we’ll explore additional methods to check if a Python string contains a substring. Stay tuned!
Exploring Alternative Methods
While the in
keyword and regular expressions are great tools for checking if a Python string contains a substring, they are not the only options. Python’s rich library offers other methods like find()
and index()
. These methods not only check for the existence of a substring but also provide additional information, such as the position of the substring in the string.
Using the find()
Method
Python’s find()
method returns the index of the start of the substring if it’s found in the string. If it doesn’t find the substring, it returns -1
. Here’s an example:
sentence = 'Python is fun!'
word = 'fun'
print(sentence.find(word))
# Output:
# 10
In this example, the find()
method found the word ‘fun’ at index 10 in the sentence ‘Python is fun!’. If ‘fun’ was not found in the sentence, the method would have returned -1
.
Using the index()
Method
The index()
method works similarly to the find()
method. The key difference is that index()
raises an exception if it doesn’t find the substring. Let’s see it in action:
sentence = 'Python is fun!'
word = 'fun'
try:
print(sentence.index(word))
except ValueError:
print('Substring not found')
# Output:
# 10
In this example, the index()
method found ‘fun’ at index 10 in ‘Python is fun!’. If ‘fun’ was not found, it would have raised a ValueError
exception, which we handle by printing ‘Substring not found’.
Comparison Table
Method | Returns | Case-Sensitive | Supports Pattern Matching |
---|---|---|---|
in | True or False | Yes | No |
re.search() | True or False | Yes | Yes |
find() | Index or -1 | Yes | No |
index() | Index or ValueError | Yes | No |
While each method has its own advantages, the best one to use depends on your specific needs. If you just need a simple check, the in
keyword is your best bet. For pattern matching, go with re.search()
. If you need the position of the substring, consider find()
or index()
.
In the following section, we’ll discuss common issues you might encounter when checking if a Python string contains a substring and how to troubleshoot them.
While checking if a Python string contains a substring is generally straightforward, you might encounter a few bumps along the way. This section aims to guide you through some common issues and how to troubleshoot them.
Dealing with ‘TypeError’
One common error you might encounter is the TypeError
. This usually happens when you try to use non-string types with the in
keyword, find()
, or index()
methods. Let’s see an example:
sentence = 'Python is fun!'
number = 123
try:
print(number in sentence)
except TypeError:
print('TypeError encountered')
# Output:
# TypeError encountered
In this example, we tried to check if the integer 123 is in the string ‘Python is fun!’. Python throws a TypeError
because it expects a string, not an integer. The solution is to ensure that both the string and the substring are indeed strings. If necessary, you can convert other types to strings using the str()
function.
Case-Sensitive Checks
Another thing to remember is that Python’s string methods are case-sensitive. This means that ‘Fun’ and ‘fun’ are considered different substrings. If you need to perform a case-insensitive check, make sure to convert both the string and the substring to the same case (either lower or upper) before performing the check.
Regular Expressions and Special Characters
When using regular expressions with the re.search()
function, keep in mind that some characters have special meanings in regular expressions. These include characters like .
, *
, +
, ?
, ^
, $
, (
, )
, [
, ]
, {
, }
, \
, |
, and /
. If you want to match these characters literally, you need to escape them with a backslash (\
).
In the next section, we’ll take a step back and discuss the fundamentals of Python’s string data type and the concept of substrings to give you a better understanding of the process.
Python Strings and Substrings: The Basics
Before we delve further into checking if a Python string contains a substring, let’s take a step back and understand the basics of Python’s string data type and the concept of substrings.
Understanding Python Strings
In Python, a string is a sequence of characters enclosed in quotes. You can use either single quotes ('
) or double quotes ("
). Python treats both types of quotes as equal. Here’s an example of a Python string:
sentence = 'Python is fun!'
print(sentence)
# Output:
# Python is fun!
In this example, ‘Python is fun!’ is a string. We’ve assigned this string to the variable sentence
and then printed it.
What is a Substring?
A substring is a part of a string. It could be the entire string, just one character, or any number of consecutive characters from the string. Here are some examples of substrings from the string ‘Python is fun!’:
- ‘Python’
- ‘is’
- ‘fun’
- ‘Python is fun’
- ‘!’
In Python, you can extract substrings by slicing the string. Here’s an example:
sentence = 'Python is fun!'
word = sentence[0:6]
print(word)
# Output:
# Python
In this example, we’re extracting the substring ‘Python’ from the string ‘Python is fun!’ by slicing. The numbers in the square brackets represent the start and end indices of the substring. Python’s indices start at 0, so the start index is 0 for the first character. The end index is one more than the index of the last character, so it’s 6 for the sixth character.
Understanding Python’s string data type and the concept of substrings is fundamental to the process of checking if a Python string contains a substring. With this knowledge, you can better understand how the in
keyword, the find()
and index()
methods, and regular expressions work in Python.
The Bigger Picture: Substrings in Real-World Applications
Checking if a Python string contains a substring might seem like a simple task, but it has far-reaching applications in real-world scenarios. From text processing to data analysis, the ability to locate substrings is a fundamental skill in many areas of programming and data science.
Text Processing and Data Analysis
In text processing, you might need to find specific words or phrases in large bodies of text. This could be for sentiment analysis, where you’re looking for positive or negative words in social media posts or customer reviews. Similarly, in data analysis, you might need to find specific patterns or anomalies in large datasets.
review = 'This product is excellent!'
positive_words = ['excellent', 'great', 'awesome', 'fantastic', 'superb']
print(any(word in review.lower() for word in positive_words))
# Output:
# True
In this example, we’re checking if a product review contains any positive words. The any()
function returns True
as soon as it finds a word from the positive_words
list in the review, indicating a positive sentiment.
Exploring Related Concepts
The ability to check if a Python string contains a substring is just the tip of the iceberg. Python offers a wealth of tools for string manipulation and pattern matching, such as string formatting, splitting and joining strings, and using regular expressions for complex pattern matching.
For those looking to dig deeper into Python’s string manipulation capabilities and regular expressions, Python’s official documentation and various online tutorials offer a wealth of resources.
Remember, mastering Python is a journey. Each new concept you learn, like checking if a string contains a substring, is another step forward on this journey. Keep exploring, keep learning, and most importantly, keep coding!
Further Resources for Python Strings
If you’re interested in learning more ways to handle strings in Python, here are a few resources that you might find helpful:
- Python String Manipulation: Techniques and Best Practices: Discover techniques and best practices for Python string manipulation, enhancing code quality and efficiency.
Python rstrip(): Removing Trailing Characters from a String: Learn how to use the rstrip() method in Python to remove trailing characters from a string, with detailed explanations and examples.
Python String Format: Formatting Strings in Python: This tutorial provides an overview of different ways to format strings in Python using the string format() method, with examples and explanations of formatting options.
Python String Contains Substring: A Comprehensive Guide: Real Python provides a comprehensive guide on various methods to check if a string contains a substring in Python, with examples and explanations.
Check if String contains Substring in Python: GeeksforGeeks offers methods and examples to check if a string contains a substring in Python.
Python String Contains: How to Check If a String Contains a Substring: DigitalOcean presents different approaches to check if a string contains a substring in Python, along with code examples.
Unraveling Python Substring Checks: A Recap
Throughout this comprehensive guide, we’ve explored the art of checking if a Python string contains a certain substring. From the simplicity of the in
keyword to the power of regular expressions, Python offers a rich set of tools to handle this task.
In our journey, we’ve seen that the in
keyword is a quick and easy way to check for substrings. However, it’s case-sensitive, and doesn’t support pattern matching or provide the substring’s position. For case-insensitive checks, we’ve learned to use the lower()
or upper()
methods to convert both the string and substring to the same case.
For more advanced scenarios, we’ve explored regular expressions with Python’s re
module. While they can be a bit tricky, they’re incredibly powerful and flexible, supporting complex pattern matching.
We’ve also looked at alternative methods like find()
and index()
, which not only check for substrings but also provide their starting index. While find()
returns -1
if it doesn’t find the substring, index()
raises a ValueError
exception.
Here’s a quick comparison of the methods we’ve discussed:
Method | Returns | Case-Sensitive | Supports Pattern Matching |
---|---|---|---|
in | True or False | Yes | No |
re.search() | True or False | Yes | Yes |
find() | Index or -1 | Yes | No |
index() | Index or ValueError | Yes | No |
Remember, the best method to use depends on your specific needs. Whether you’re a beginner just starting out or an experienced programmer, Python’s string manipulation capabilities are a powerful addition to your toolkit.