Python Do-While Loop: Ultimate How-To Guide
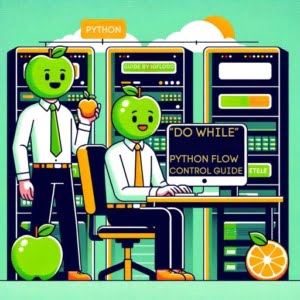
Implementing a do-while loop in Python programming is crucial while programming at IOFLOOD, ensuring at least one execution of a code block before checking the loop condition. In our experience, do-while loops offer a robust solution for handling iterative tasks effectively. In today’s article, we dive into how to create a do-while loop in Python, providing examples and insights to empower our dedicated server hosting customers.
In this guide, we’ll show you how to create a do-while loop in Python, even though Python doesn’t have a built-in do-while construct. We’ll explore the core functionality, delve into its advanced use cases, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the Python do-while loop!
TL;DR: How Do I Create a Do-While Loop in Python?
Python does not have a built-in
do-while
loop. However, you can achieve similar functionality using awhile
loop with an initial condition that always evaluates toTrue
and abreak
statement within the loop.
For example:
while True:
# do something
if not condition:
break
# Output:
# Depends on the condition and the task performed inside the loop.
In this example, we create an infinite loop using while True:
. Inside the loop, we perform a task (represented by # do something
). After performing the task, we check a condition. If the condition is not met (if not condition:
), we break out of the loop using break
.
This is a basic way to create a do-while loop in Python, but there’s much more to learn about loops and control flow in Python. Continue reading for a more detailed explanation and advanced use cases.
Table of Contents
Creating a Do-While Loop in Python: The Basics
To understand the concept of a do-while loop in Python, let’s start with the basics. Python doesn’t have a built-in do-while loop like some other programming languages. However, we can emulate this functionality using a while loop with a condition at the end.
Let’s take a look at a simple example:
count = 0
while True:
count += 1
print('This is loop iteration', count)
if count == 5:
break
# Output:
# 'This is loop iteration 1'
# 'This is loop iteration 2'
# 'This is loop iteration 3'
# 'This is loop iteration 4'
# 'This is loop iteration 5'
In this code, we start by setting a counter variable count
to 0. Then, we initiate an infinite loop using while True:
. Inside this loop, we increase the count by 1 for each iteration (count += 1
) and print the iteration number.
The key part here is the condition check if count == 5:
. This condition checks if the count has reached 5. If it has, we break out of the loop using break
. This is how we emulate a do-while loop: the loop always runs at least once, and then checks a condition at the end to decide whether to continue.
This basic use of a do-while loop in Python is simple yet powerful. It allows us to perform a task repeatedly until a certain condition is met, just like a detective tirelessly gathering clues until the case is solved.
Advanced Use of Python Do-While Loop
As you become more comfortable with Python’s do-while loops, you can start exploring more complex use cases. Two such cases are nested loops and using the else
statement with a while loop.
Nested Do-While Loops
Just like detectives sometimes have to dive deeper into sub-cases within a main case, you can nest do-while loops within each other. Here’s an example:
outer_count = 0
while outer_count < 3:
outer_count += 1
print('Outer loop iteration:', outer_count)
inner_count = 0
while True:
inner_count += 1
print(' Inner loop iteration:', inner_count)
if inner_count == 3:
break
# Output:
# 'Outer loop iteration: 1'
# ' Inner loop iteration: 1'
# ' Inner loop iteration: 2'
# ' Inner loop iteration: 3'
# 'Outer loop iteration: 2'
# ' Inner loop iteration: 1'
# ' Inner loop iteration: 2'
# ' Inner loop iteration: 3'
# 'Outer loop iteration: 3'
# ' Inner loop iteration: 1'
# ' Inner loop iteration: 2'
# ' Inner loop iteration: 3'
In this example, we have an outer loop that runs three times, and for each iteration of the outer loop, an inner loop runs three times. This is a powerful way to perform more complex tasks that require multiple levels of looping.
Using Else with a Do-While Loop
In Python, you can use an else
statement with a while loop. The else
block executes after the while loop finishes, but not if the loop is exited with a break
statement. Here’s an example with a do-while loop:
n = 0
while n < 5:
n += 1
print('Loop iteration:', n)
if n == 3:
print('Breaking out of the loop')
break
else:
print('Loop finished normally')
# Output:
# 'Loop iteration: 1'
# 'Loop iteration: 2'
# 'Loop iteration: 3'
# 'Breaking out of the loop'
In this code, the else
block does not execute because we break out of the loop when n
equals 3. If we didn’t break out of the loop, the else
block would execute after the loop finishes. This can be useful for performing a task only if the loop completed normally, without hitting a break
statement.
Exploring Alternative Loop Constructs in Python
While the do-while loop is a powerful tool in Python, it’s not the only looping construct available. Other options include the for
loop and the while
loop without a break
statement. Understanding these alternatives can help you choose the right tool for your specific task.
Python For Loop
The for
loop in Python is used to iterate over a sequence (like a list, tuple, dictionary, set, or string) or other iterable objects. Here’s a simple example:
for i in range(5):
print('Loop iteration:', i+1)
# Output:
# 'Loop iteration: 1'
# 'Loop iteration: 2'
# 'Loop iteration: 3'
# 'Loop iteration: 4'
# 'Loop iteration: 5'
In this example, range(5)
generates a sequence of numbers from 0 to 4. The for
loop then iterates over this sequence, and for each iteration, it prints the iteration number (i+1
).
Python While Loop Without Break
You can also use a while
loop without a break
statement. Instead of breaking out of the loop when a condition is met, you can set the loop to continue as long as a condition is true. Here’s an example:
n = 0
while n < 5:
n += 1
print('Loop iteration:', n)
# Output:
# 'Loop iteration: 1'
# 'Loop iteration: 2'
# 'Loop iteration: 3'
# 'Loop iteration: 4'
# 'Loop iteration: 5'
In this example, the while
loop continues as long as n
is less than 5. For each iteration, it increases n
by 1 and prints the iteration number.
Both the for
loop and the while
loop without a break
statement have their own benefits and drawbacks. The for
loop is great for tasks that require a specific number of iterations, while the while
loop is ideal for tasks that need to continue until a certain condition is met. Your choice between these constructs should be guided by the specific requirements of your task.
Troubleshooting Python Do-While Loops
As with any programming construct, using a do-while loop in Python can present some challenges. Two common issues are infinite loops and exception handling.
Avoiding Infinite Loops
One common pitfall when using do-while loops is accidentally creating an infinite loop. This happens when the loop’s condition never becomes false, causing the loop to run forever. Here’s an example:
while True:
print('This loop will run forever')
# Output:
# 'This loop will run forever'
# 'This loop will run forever'
# ...
In this code, the condition for the while
loop is always True
, so the loop will never stop running. To avoid this, make sure there’s a way for the loop’s condition to become false.
Handling Exceptions
Another issue to consider when using do-while loops is exception handling. If an exception occurs during a loop iteration, it can cause the program to crash. To handle exceptions, you can use a try/except
block inside the loop. Here’s an example:
n = 0
while n < 5:
try:
# Attempt to perform a task that may raise an exception
print('Loop iteration:', n)
n += 1
if n == 3:
raise ValueError('An exception occurred')
except ValueError as e:
print(e)
break
# Output:
# 'Loop iteration: 0'
# 'Loop iteration: 1'
# 'Loop iteration: 2'
# 'An exception occurred'
In this code, a ValueError
is raised when n
equals 3. The except
block catches this exception and prints its message, preventing the program from crashing.
By understanding these potential issues and how to handle them, you can use do-while loops in Python more effectively and avoid common pitfalls.
Understanding Python Loops and Control Flow
To effectively use do-while loops in Python, it’s essential to understand the broader context of loops and control flow in Python. This includes the while
loop, the for
loop, and how control flow works.
The While Loop in Python
The while
loop in Python is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. Here’s a simple example:
n = 0
while n < 5:
print('Loop iteration:', n+1)
n += 1
# Output:
# 'Loop iteration: 1'
# 'Loop iteration: 2'
# 'Loop iteration: 3'
# 'Loop iteration: 4'
# 'Loop iteration: 5'
In this example, the while
loop keeps running as long as n
is less than 5. For each loop iteration, it prints the iteration number and increases n
by 1.
The For Loop in Python
The for
loop in Python is used to iterate over a sequence (like a list, tuple, string) or other iterable objects. Here’s an example:
for i in range(5):
print('Loop iteration:', i+1)
# Output:
# 'Loop iteration: 1'
# 'Loop iteration: 2'
# 'Loop iteration: 3'
# 'Loop iteration: 4'
# 'Loop iteration: 5'
In this example, range(5)
generates a sequence of numbers from 0 to 4. The for
loop then iterates over this sequence, and for each iteration, it prints the iteration number (i+1
).
Control Flow in Python
Control flow is a fundamental concept in programming that determines the order in which code is executed. In Python, control flow is managed through conditional statements (like if
, elif
, and else
), loops (like while
and for
), and control flow statements (like break
and continue
).
Understanding these fundamentals is key to mastering the use of do-while loops in Python. With this knowledge, you can write more efficient code and solve more complex problems.
Python Do-While Loops in Larger Programs
Python’s do-while loop, like many other programming constructs, isn’t limited to simple, standalone tasks. It can also be a key component in larger Python programs, such as those used in game development and data analysis.
Do-While Loops in Game Development
In game development, loops are essential for creating game cycles, such as updating the game state or rendering graphics. A do-while loop can be used to keep the game running until a certain condition is met, such as the player reaching a certain score or losing all their lives.
Do-While Loops in Data Analysis
In data analysis, loops can be used to process large datasets. A do-while loop can be particularly useful when you need to keep processing data until a certain condition is met, such as reaching a specific statistical threshold.
Related Topics to Explore
To further enhance your Python skills, consider exploring related topics like conditional statements (like if
, elif
, and else
) and functions in Python. These topics, combined with a solid understanding of loops, can make you a more versatile and effective Python programmer.
Further Resources for Mastering Python Loops
To continue your journey towards mastering Python loops, you might find these resources helpful:
- Python Loop Structures – A guide to mastering loops in Python and their various types.
Python “switch-case” Alternatives – Discover how to implement switch-case statements in Python.
Python Generators: Streamlining Iteration – Dive into Python generators and their role in memory-efficient data processing.
Control Flow Documentation – The official Python documentation on control flow statements in Python, including loops.
Loops in Python – Real Python offers a detailed tutorial on loops in Python, with examples and exercises.
Python Loops – This tutorial from Python for Beginners provides an easy-to-understand introduction to loops in Python.
By incorporating these resources into your learning and expanding your understanding of Python loops, you can amplify your programming skills in Python.
Wrapping Up: Mastering Python Do-While Loop
This comprehensive guide has walked you through the ins and outs of implementing a do-while loop in Python. We’ve explored the basics, delved into more complex use cases, and even discussed common issues and their solutions.
We began with the fundamentals, creating a do-while loop using a while loop with a condition at the end. We then explored more advanced use cases, such as nested loops and using the else
statement with a while loop. We also discussed common issues you might encounter when using do-while loops in Python, such as infinite loops and exception handling, and provided solutions to help you overcome these challenges.
Additionally, we explored alternative loop constructs in Python, such as the for
loop and the while
loop without a break
statement. Understanding these alternatives can help you choose the right tool for your specific tasks.
Here’s a quick comparison of the loop constructs we’ve discussed:
Loop Construct | Use Case | Complexity |
---|---|---|
Do-While | Repeated tasks until condition is met | Moderate |
For Loop | Iterating over a sequence or iterable | Simple |
While Loop without Break | Tasks that continue as long as a condition is true | Simple |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to implement a do-while loop in Python and the power of this construct. Happy coding!