Python Generator Mastery: In-Depth Guide
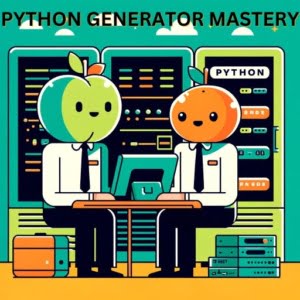
Understanding Python generators is essential while programming on Linux servers at IOFLOOD, as it assists with efficient memory management and iterable generation. In our experience, Python generators offer a memory-efficient approach to data generation. In today’s article, we explore what Python generators are, how they differ from regular functions, and how to use them effectively for iterative operations, providing valuable insights and examples for our bare metal cloud server customers and fellow developers.
In this guide, we’ll walk you through the process of using Python generators, from the basics to more advanced techniques. We’ll cover everything from creating a simple generator, using generator expressions, to handling common issues and their solutions.
Let’s get started mastering Python generators!
TL;DR: What is a Python Generator?
A Python generator is a special type of function that returns an iterable sequence of items, defined by using the
yield
keyword within afunction
. Instead of returning all items at once, it yields one item at a time, which can be more memory-efficient.
Here’s a simple example:
def simple_generator():
yield 1
yield 2
yield 3
for number in simple_generator():
print(number)
# Output:
# 1
# 2
# 3
In this example, we’ve defined a simple Python generator function simple_generator()
. This function yields three numbers one by one. When we loop over the generator, it prints out these numbers one at a time.
This is just the tip of the iceberg when it comes to Python generators. There’s much more to learn about creating and using generators in Python. Continue reading for a more detailed dive into Python generators.
Table of Contents
- Creating and Using Python Generators
- Python Generator Expressions and Built-in Functions
- Alternative Ways to Create Iterable Sequences
- Handling Common Generator Issues
- Understanding Python’s Iterator Protocol
- The Power of the yield Keyword
- The Concept of Lazy Evaluation
- Python Generators in Larger Projects
- Exploring Related Topics
- Wrapping Up: Mastering Python Generators
Creating and Using Python Generators
Python generators are a powerful tool for managing memory when dealing with large data sets. Let’s start by creating a simple Python generator.
def count_up_to(n):
num = 1
while num <= n:
yield num
num += 1
for number in count_up_to(5):
print(number)
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we’ve defined a generator function count_up_to(n)
. This function takes a number n
as an argument and yields numbers from 1 up to n
. When we loop over this generator with n=5
, it prints numbers from 1 to 5, one at a time.
Advantages of Using Generators
Python generators are particularly useful when dealing with large data sets. They allow you to create a data stream that can be consumed one item at a time, instead of loading the entire data set into memory. This can result in significant memory savings, especially when dealing with large data sets.
Potential Pitfalls of Using Generators
While generators are powerful, they come with their own set of challenges. One of the main pitfalls of using generators is that they can only be iterated over once. Once a generator’s data has been consumed, it cannot be reused or reset. This means you need to recreate the generator every time you want to iterate over the data again.
Another potential pitfall is that generators are stateful, meaning they retain the state between executions. This can lead to unexpected behavior if not handled correctly.
Python Generator Expressions and Built-in Functions
As you become more comfortable with Python generators, you can start exploring more complex uses. One such use is generator expressions.
Generator Expressions
Generator expressions are a high-performance, memory–efficient generalization of list comprehensions and generators. Here’s an example:
numbers = (x for x in range(10) if x % 2 == 0)
for number in numbers:
print(number)
# Output:
# 0
# 2
# 4
# 6
# 8
In this example, we create a generator expression that generates even numbers from 0 to 9. We then loop over the generator and print each number. This code does the same thing as our previous count_up_to(n)
function but in a more concise way.
Using next()
with Generators
Python’s built-in next()
function allows you to manually retrieve the next item from a generator. Here’s how you can use it:
generator = count_up_to(5)
print(next(generator))
print(next(generator))
# Output:
# 1
# 2
In this code, we create a generator using our previously defined count_up_to(5)
function. We then use the next()
function to print the first two numbers in the sequence. This allows us to control the execution of the generator and retrieve values one at a time.
These advanced techniques can help you write more efficient and readable code when working with Python generators.
Alternative Ways to Create Iterable Sequences
Python provides several other ways to create iterable sequences, such as lists, tuples, and the itertools module. Each of these methods has its own strengths and weaknesses compared to Python generators.
Lists and Tuples
Lists and tuples are the most basic ways to create iterable sequences in Python. They are simple to use and understand, but they can be memory-intensive when dealing with large data sets.
Here’s a simple example of creating an iterable sequence with a list:
numbers = [x for x in range(10) if x % 2 == 0]
for number in numbers:
print(number)
# Output:
# 0
# 2
# 4
# 6
# 8
In this example, we create a list comprehension that generates even numbers from 0 to 9. We then loop over the list and print each number. This does the same thing as our previous generator expression but loads all the numbers into memory at once.
The itertools Module
The itertools module is a collection of tools for handling iterators. It provides various functions that return iterators, which can be used to create complex iterable sequences.
Here’s an example of using the itertools.count()
function to create an infinite iterator:
import itertools
numbers = itertools.count(1)
for i, number in enumerate(numbers):
if i >= 5:
break
print(number)
# Output:
# 1
# 2
# 3
# 4
# 5
In this code, we use itertools.count(1)
to create an infinite iterator that starts counting from 1. We then use a for loop with enumerate()
to print the first five numbers in the sequence. This demonstrates the power of the itertools module but also shows that it can be more complex to use than simple generators.
While these methods can be useful in certain situations, they lack the memory efficiency of Python generators when dealing with large data sets.
Handling Common Generator Issues
While Python generators can be incredibly useful, they can also raise certain issues if not used correctly. Let’s explore some of these problems and their solutions.
The StopIteration
Error
One common issue when working with generators is the StopIteration
error. This error occurs when you try to retrieve a value from a generator that has no more values to yield.
Here’s an example:
generator = count_up_to(3)
for _ in range(5):
print(next(generator))
# Output:
# 1
# 2
# 3
# StopIteration
In this example, we try to retrieve five numbers from a generator that only yields three numbers. After the third number, the next()
function raises a StopIteration
error.
To handle this error, we can use a try/except block:
generator = count_up_to(3)
for _ in range(5):
try:
print(next(generator))
except StopIteration:
break
# Output:
# 1
# 2
# 3
In this code, we catch the StopIteration
error with an except block and break out of the loop. This prevents the error from stopping our program and allows us to handle the end of the generator gracefully.
Generator Exhaustion
As mentioned before, generators can only be iterated over once. Attempting to iterate over a generator that has already been exhausted will not raise an error, but it will not produce any output either.
generator = count_up_to(3)
for number in generator:
print(number)
for number in generator:
print(number)
# Output:
# 1
# 2
# 3
In this example, we try to iterate over the same generator twice. The first loop works as expected, but the second loop does not produce any output because the generator has already been exhausted.
To work around this issue, you can create a new generator each time you want to iterate over the data again.
Understanding Python’s Iterator Protocol
To truly grasp Python generators, it’s crucial to understand the iterator protocol in Python. In essence, the iterator protocol is a specific way to make an object iterable. In other words, you can loop over it.
An object is considered an iterator in Python if it has implemented two special methods, __iter__()
and __next__()
. The __iter__()
method returns the iterator object itself, while the __next__()
method returns the next value from the iterator.
Generators are a simple and powerful tool for creating iterators. They automatically implement the iterator protocol and allow you to iterate over sequences of data without the need to build a custom class.
The Power of the yield
Keyword
At the heart of every generator in Python is the yield
keyword. Unlike the return
keyword, which terminates a function entirely, yield
produces a value and suspends the function’s execution. The function can then be resumed right where it left off, allowing it to produce a series of values over time, rather than computing them all at once and sending them back like a list.
Here’s an example of a generator that uses the yield
keyword:
def countdown(num):
print('Starting')
while num > 0:
yield num
num -= 1
cd = countdown(3)
for number in cd:
print(number)
# Output:
# Starting
# 3
# 2
# 1
In this example, the countdown
generator function uses the yield
keyword to produce a sequence of numbers from a given number down to 1. Each time the generator is iterated over, it yields the next number in the sequence.
The Concept of Lazy Evaluation
One of the key benefits of Python generators is that they support lazy evaluation. This means that a value is produced only when it is needed. This is particularly useful when working with large data sets, as it allows you to create a data stream that can be consumed one item at a time, without having to load the entire data set into memory.
In the context of our countdown
generator, lazy evaluation means that the next number in the countdown isn’t calculated until it’s requested. This makes generators incredibly memory-efficient when dealing with large sequences of data.
Python Generators in Larger Projects
Python generators are not just for small scripts or exercises. They are incredibly useful in larger projects, particularly in the realms of data analysis and web scraping.
Generators in Data Analysis
In data analysis, you often deal with large data sets that can be cumbersome to load into memory all at once. Python generators can help you handle these data sets more efficiently. They allow you to process your data in chunks, loading only one piece of data into memory at a time.
Generators in Web Scraping
Similarly, in web scraping, you might need to crawl through thousands or even millions of web pages. Python generators can help you manage this task more efficiently. They allow you to fetch and process pages one at a time, rather than downloading all pages at once.
Exploring Related Topics
Once you’ve mastered Python generators, there are other related topics you might want to explore. These include coroutines and asynchronous programming in Python.
Coroutines
Coroutines are a generalization of generators. They not only can produce values (like generators) but also can consume values passed to them. This makes them ideal for tasks that involve a lot of back-and-forth communication, such as cooperative multitasking, event-driven programming, or building data pipelines.
Asynchronous Programming
Asynchronous programming is a style of programming that is designed to handle tasks that can be executed concurrently. This is particularly useful in network programming, where tasks often involve waiting for data to be sent or received over a network. Python’s asyncio
module provides support for asynchronous I/O through coroutines and futures.
Further Resources for Mastering Python Generators
Here are some additional resources to help you deepen your understanding of Python generators and related topics:
- IOFlood’s Python Loop Article can teach you how to simplify tasks like list comprehension and data traversal.
Python “do-while” Loop – Learn how to implement a do-while loop in Python for conditional iterating.
“next()” Iterator in Python – A quick guide on how “next” advances the iterator and retrieves the next item.
Python’s Official Documentation on Generators provides an understanding of generator classes in Python.
Real Python’s Guide to Python Generators explores the concept of generators in Python in detail.
Python’s Official Documentation on Coroutines and Asyncio helps you understand coroutines and the asyncio library for asynchronous I/O.
Wrapping Up: Mastering Python Generators
In this comprehensive guide, we’ve delved into the world of Python generators, a powerful feature of Python that allows for the creation of iterable sequences in a memory-efficient way.
We began with the basics, learning how to create and use a simple Python generator. We then explored more advanced topics, such as generator expressions and using built-in Python functions with generators. Along the way, we tackled common issues you might encounter when using Python generators, such as StopIteration
errors and generator exhaustion, providing solutions and workarounds for each issue.
We also looked at alternative ways to create iterable sequences in Python, such as using lists, tuples, and the itertools module. Here’s a quick comparison of these methods:
Method | Memory Efficiency | Ease of Use | Reusability |
---|---|---|---|
Python Generators | High | High | No |
Lists/Tuples | Low | High | Yes |
itertools Module | High | Moderate | Yes |
Whether you’re a beginner just starting out with Python generators or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python generators and their capabilities. Happy coding!