Python next() Function Guide (With Examples)
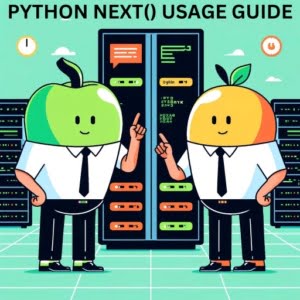
Using the next() function in Python programming is crucial while automating tasks at IOFLOOD, as it allows sequential access to elements in an iterator. In our experience, the next() function streamlines data traversal and facilitates efficient iteration, particularly with complex data structures. Today’s article explores how to effectively use the next() function in Python, providing examples and explanations to empower our customers with valuable scripting techniques for their dedicated cloud service.
This guide will provide a comprehensive understanding of Python’s next() function, from basic use to advanced techniques. We’ll cover everything from the basics of how to use next() to troubleshooting tips, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use the next() Function in Python?
The
next()
function in Python is used to retrieve the next item from an iterator, such as withprint(next(my_list))
, wheremy_list
is an iterable list.
Here’s a simple example:
my_list = iter([1, 2, 3])
print(next(my_list))
# Output:
# 1
In this example, we first convert a list into an iterator using the iter()
function. Then, we use the next()
function to retrieve the first item from the iterator. The output is 1
, which is the first item in our list.
This is just a basic usage of the
next()
function in Python. There’s much more to learn about it, including handling theStopIteration
exception and advanced usage scenarios. Continue reading for a more detailed understanding.
Table of Contents
- Understanding Python’s next() Function
- Handling the StopIteration Exception
- Exploring Alternatives to Python’s next() Function
- Navigating Common Issues with Python’s next()
- Unraveling Python’s Iterables and Iterators
- Python’s next() Function: Beyond Basic Iteration
- Wrapping Up: Mastering Python’s next() Function
Understanding Python’s next() Function
The next()
function is a built-in Python function that retrieves the next item from an iterator. An iterator, in Python, is an object that contains a countable number of values and can be iterated upon. An object which implements the iterator protocol, which consist of the methods __iter__()
and __next__()
.
Let’s dive into a simple example to understand how next()
works:
# Creating an iterator object from a list
my_list = iter(['apple', 'banana', 'cherry'])
# Using next() to retrieve the first item
print(next(my_list))
# Output:
# 'apple'
In this example, we first create an iterator object from a list of fruits using the iter()
function. We then use the next()
function to retrieve the first item from the iterator. The output is 'apple'
, which is the first item in our list.
One of the key advantages of using next()
is that it allows you to control the iteration process manually. This can be useful in scenarios where you need to break out of the loop under certain conditions or when you want to manipulate the iterator object directly.
However, it’s important to note that once an item has been consumed by next()
, it cannot be retrieved again unless you reinitialize the iterator. Also, if there are no more items to return and next()
is called, Python raises a StopIteration
exception. We will cover how to handle this exception in the next section.
Handling the StopIteration Exception
As you delve deeper into the world of Python’s next()
function, you’ll encounter a common roadblock – the StopIteration
exception. This exception is raised when the next()
function exhausts all items in the iterator and there’s nothing left to return.
Let’s illustrate this with a simple example:
# Creating an iterator object from a list
my_list = iter(['apple', 'banana', 'cherry'])
# Using next() to retrieve all items
print(next(my_list))
print(next(my_list))
print(next(my_list))
print(next(my_list))
# Output:
# 'apple'
# 'banana'
# 'cherry'
# StopIteration
In this example, we try to call next()
on our iterator one more time than we have items. The first three calls to next()
return the fruits as expected, but the fourth call raises a StopIteration
exception because there are no more items to return.
Using a Default Value with next()
One way to prevent the StopIteration
exception is by providing a default value to the next()
function. The next()
function accepts two arguments: the iterator and a default value. When all items have been returned and next()
is called again, instead of raising a StopIteration
exception, it will return the default value.
Here’s how you can do it:
# Creating an iterator object from a list
my_list = iter(['apple', 'banana', 'cherry'])
# Using next() with a default value
print(next(my_list, 'no more items'))
print(next(my_list, 'no more items'))
print(next(my_list, 'no more items'))
print(next(my_list, 'no more items'))
# Output:
# 'apple'
# 'banana'
# 'cherry'
# 'no more items'
In this example, we provided ‘no more items’ as the default value to the next()
function. So, when we ran out of items in the iterator, instead of raising a StopIteration
exception, next()
returned ‘no more items’.
Using a default value with next()
can be a best practice in situations where you want to avoid exceptions and ensure the smooth running of your code.
Exploring Alternatives to Python’s next() Function
While next()
is a powerful function for iterating over objects in Python, it’s not the only option available. There are other methods that you can use to achieve the same result, including for
loops and list comprehensions.
Using a For Loop
A for
loop is a simple and straightforward way to iterate over an object. Here’s how you can use a for
loop to iterate over a list:
# Creating a list
my_list = ['apple', 'banana', 'cherry']
# Using a for loop to iterate over the list
for item in my_list:
print(item)
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, we use a for
loop to iterate over the list and print each item. This is a more traditional approach to iteration and is widely used due to its simplicity and readability.
Using List Comprehension
List comprehension is a more advanced method of iteration in Python. It provides a concise way to create lists based on existing lists. Here’s an example:
# Creating a list
my_list = ['apple', 'banana', 'cherry']
# Using list comprehension to create a new list
new_list = [item.upper() for item in my_list]
print(new_list)
# Output:
# ['APPLE', 'BANANA', 'CHERRY']
In this example, we use list comprehension to create a new list where each item is the uppercase version of an item from the original list. List comprehension is a powerful tool that can simplify your code and improve its efficiency.
Method | Advantages | Disadvantages |
---|---|---|
next() | Control over iteration process, ability to provide a default value | Raises StopIteration if no default value is provided |
for loop | Simple, easy to read | Less control over iteration process |
list comprehension | Concise, efficient | Can be harder to read for complex operations |
As you can see, each method has its own advantages and disadvantages. The best method to use depends on your specific needs and the complexity of your task. While next()
provides more control over the iteration process, for
loops and list comprehensions are simpler and can be more efficient for certain tasks.
While Python’s next()
function is a powerful tool for iterating over objects, it’s not without its challenges. Let’s discuss some common issues you might encounter and offer solutions to navigate these hurdles.
Dealing with StopIteration
As we’ve discussed, the StopIteration
exception is raised when there are no more items to return in the iterator. One way to handle this is by providing a default value to the next()
function. This prevents the StopIteration
exception and allows your code to continue running smoothly. Here’s an example:
# Creating an iterator object from a list
my_list = iter(['apple', 'banana', 'cherry'])
# Using next() with a default value
print(next(my_list, 'no more items'))
print(next(my_list, 'no more items'))
print(next(my_list, 'no more items'))
print(next(my_list, 'no more items'))
# Output:
# 'apple'
# 'banana'
# 'cherry'
# 'no more items'
In this example, we provided ‘no more items’ as the default value to the next()
function. So, when we ran out of items in the iterator, instead of raising a StopIteration
exception, next()
returned ‘no more items’.
Handling Non-Iterable Objects
Another common issue is trying to use next()
on a non-iterable object. In Python, an iterable is an object capable of returning its members one at a time. If you try to use next()
on a non-iterable object, Python will raise a TypeError
.
To handle this, you can use the isinstance()
function to check if an object is an iterator before using next()
. Here’s an example:
# Creating a non-iterator object
my_object = 12345
# Checking if the object is an iterator
if isinstance(my_object, str):
print(next(my_object))
else:
print('Object is not an iterator')
# Output:
# 'Object is not an iterator'
In this example, we check if the object is an iterator using the isinstance()
function. If it is, we use next()
to retrieve the next item. If it’s not, we print a message indicating that the object is not an iterator.
By being aware of these common issues and knowing how to handle them, you can make the most of Python’s next()
function and write more robust code.
Unraveling Python’s Iterables and Iterators
To truly master Python’s next()
function, it’s essential to understand the underlying concepts of iterable objects and iterators.
What are Iterable Objects?
In Python, an iterable is any object capable of returning its elements one at a time. Common examples of iterables include lists, tuples, strings, and dictionaries. Iterables are handy because you can read their items sequentially without loading them all into memory at once.
Here’s a simple example of an iterable:
# Creating a list (an iterable object)
my_list = ['apple', 'banana', 'cherry']
# Iterating over the list using a for loop
for item in my_list:
print(item)
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, we created a list of fruits, which is an iterable object. We then used a for
loop to iterate over the list and print each item.
Understanding Iterators
An iterator, on the other hand, is an object that implements the iterator protocol. The iterator protocol consists of two methods: __iter__()
and __next__()
.
The __iter__()
method returns the iterator object itself, while the __next__()
method returns the next value from the iterator. When there are no more items to return, it raises the StopIteration
exception.
Here’s a simple example of an iterator:
# Creating an iterator from a list
my_iterator = iter(['apple', 'banana', 'cherry'])
# Using the next() function to retrieve items from the iterator
print(next(my_iterator))
print(next(my_iterator))
print(next(my_iterator))
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, we first created an iterator from a list using the iter()
function. We then used the next()
function to retrieve each item from the iterator.
By understanding the concepts of iterable objects and iterators, you’ll have a solid foundation for mastering Python’s next()
function.
Python’s next() Function: Beyond Basic Iteration
Python’s next()
function is not just for iterating over lists or tuples. It’s a versatile tool that plays a crucial role in various aspects of Python programming, including data processing and file handling.
next() in Data Processing
In the realm of data processing, next()
can be used to read data from large files that don’t fit into memory. It allows you to process the data one line at a time, reducing memory usage.
Here’s a simple example:
# Opening a file
with open('large_file.txt', 'r') as file:
while True:
line = next(file, None)
if line is None:
break
print(line)
# Output:
# Prints each line of 'large_file.txt'
In this example, we use next()
to read a large file line by line. We provide None
as the default value to next()
, so when we reach the end of the file, next()
returns None
and we break the loop.
next() in File Handling
When working with files, next()
can be used to skip the header line in a CSV file before processing the rest of the lines.
Here’s how you can do it:
import csv
# Opening a CSV file
with open('data.csv', 'r') as file:
reader = csv.reader(file)
header = next(reader)
for row in reader:
print(row)
# Output:
# Prints each row of 'data.csv', excluding the header
In this example, we use next()
to skip the header line of a CSV file. We then use a for
loop to process the rest of the lines.
Exploring Related Concepts: Generators and Coroutines
If you’re interested in going beyond the next()
function, you might want to explore related concepts like generators and coroutines. Generators are a type of iterable that generate values on the fly, while coroutines are generalizations of subroutines used for non-preemptive multitasking.
Further Resources for Mastering Python’s next()
- IOFlood’s Python Loop Guide explores the use of enumeration in loops for tracking iteration count.
Python Iteration with Generators – Explore the generator concept for creating iterable sequences in Python.
Python Iterators Guide – Learn the fundamentals of navigating data in Python with iterators.
Python’s Official Documentation on Built-in Functions provides an overview of
next()
and other built-in functions.Python’s Official Tutorial on Iterators offers a deep dive into the world of iterators and iterable objects.
Real Python’s Guide on Iterators and Generators provides practical examples and use-cases of
next()
, generators, and more.
Wrapping Up: Mastering Python’s next() Function
In this comprehensive guide, we’ve delved deep into the world of Python’s next()
function, a versatile tool for iterating over iterable objects.
We began with the basics, learning how to use next()
to retrieve items from an iterator one by one. We then tackled the StopIteration
exception, a common issue that arises when all items have been consumed from the iterator. We explored how to handle this exception by providing a default value to the next()
function, thus preventing our code from breaking and ensuring a smooth iteration process.
As we ventured into more advanced territory, we discussed alternative approaches to iteration, such as for
loops and list comprehensions. We also handled common issues one might encounter during iteration, such as dealing with non-iterable objects, and provided solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
next() | Control over iteration process, ability to provide a default value | Raises StopIteration if no default value is provided |
for loop | Simple, easy to read | Less control over iteration process |
list comprehension | Concise, efficient | Can be harder to read for complex operations |
Whether you’re just starting out with Python’s next()
function or looking to level up your skills, we hope this guide has given you a deeper understanding of its capabilities and its role in Python programming.
With its balance of control and versatility, Python’s next()
function is a powerful tool for handling iteration in Python. Happy coding!