Learn Python: Read a File Line by Line (With Examples)
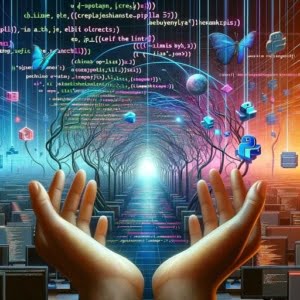
Python’s versatile file handling capabilities are one of its many strengths, and today, we’re going to delve into one specific aspect of it – reading files line by line.
Whether you’re a beginner starting your Python journey or a seasoned developer brushing up on your skills, this guide is designed to equip you with the knowledge to read files line by line using Python effectively. So, let’s get started and uncover the magic of Python’s file handling!
TL;DR: How do I read files line by line in Python?
Python offers several methods to read files line by line, including the ‘readline’ method, the ‘readlines’ method, and the ‘for’ loop method. Each method has its unique advantages and use cases. For more advanced methods, background, tips, and tricks, continue reading the article.
Example with 'For'
loop:
file = open('myfile.txt', 'r')
for line in file:
print(line)
file.close()
Table of Contents
Python Methods for Reading Files Line by Line
Python offers an array of methods to read files line by line, each catering to different needs and use cases. We’ll delve into three of the most commonly employed methods.
Method | Description | Ideal Use-Case |
---|---|---|
‘readline’ | Simple, reads one line at a time, requires a while loop and manual line reading | When simplicity is preferred |
‘readlines’ | Convenient, returns a list of lines, reads entire file into memory | When all lines need to be stored in a list for further processing |
‘for’ loop | Efficient, suitable for large files, reads one line at a time | When dealing with large files |
Each of these methods has its strengths and weaknesses. The ‘readline’ method is simple and reads one line at a time, but it requires a while loop and manual line reading. The ‘readlines’ method is convenient and returns a list of lines, but it might not be the best choice for large files. The ‘for’ loop method is efficient and suitable for large files, but it might not be as intuitive for beginners.
The ‘readline’ Method
The ‘readline’ method is a simple, yet effective way to read a file line by line in Python. When invoked, it reads the subsequent line from the file and returns it as a string. Here’s a basic illustration:
file = open('myfile.txt', 'r')
line = file.readline()
while line:
print(line)
line = file.readline()
file.close()
In this code snippet, we’re opening a file, reading a line, printing it, and then sequentially reading the next line in a loop until there are no more lines to read.
The ‘readlines’ Method
The ‘readlines’ method, alternatively, reads all lines from the file simultaneously and returns them as a list of strings. Each string in the list represents a line in the file. Here’s how you can utilize it:
file = open('myfile.txt', 'r')
lines = file.readlines()
for line in lines:
print(line)
file.close()
The ‘readlines’ method can be more convenient if you need to store all lines from the file in a list for subsequent processing. However, it might not be ideal for large files as it reads the entire file into memory.
The ‘for’ Loop Method
Finally, you can employ a ‘for’ loop to iterate over the file object, which will return one line at a time. This method is efficient and doesn’t load the entire file into memory, making it suitable for large files. Here’s an example:
file = open('myfile.txt', 'r')
for line in file:
print(line)
file.close()
Choosing the right method depends on your specific use case. If you’re dealing with a large file, the ‘for’ loop method might be your best bet. If you want to store all lines in a list for further processing, ‘readlines’ could be the way to go. And if you prefer simplicity, ‘readline’ might be your method of choice.
Understanding File Handling in Python
Before we delve deeper into the art of reading files line by line in Python, let’s take a moment to understand the basics of file handling and its importance in Python.
What is File Handling?
In the simplest terms, file handling refers to the process of performing operations on files, such as creating, reading, writing, and closing them. Python provides a built-in file object that you can use to perform these operations.
Operation | Description |
---|---|
Creating | Making a new file |
Reading | Accessing data from a file |
Writing | Adding data to a file |
Closing | Ending the access to a file | Here’s a basic example of how you can open a file, write some text to it, and then close it: |
file = open('myfile.txt', 'w')
file.write('Hello, world!')
file.close()
In this code snippet, we’re creating a new file (or opening an existing one), writing a line of text to it, and then closing it. It’s as simple as that!
Delving into Advanced File Handling in Python
Having covered the basics of file handling in Python, it’s time to delve further into the realm of advanced file handling. We’ll explore the ‘with’ statement, handling large files, and error handling in file handling.
Advanced Topic | Description |
---|---|
‘with’ Statement | Simplifies the management of resources like file streams |
Handling Large Files | Techniques to read large files line by line or in chunks |
Error Handling | Catching and handling exceptions during file operations |
The ‘with’ Statement and Its Advantages
The ‘with’ statement in Python is used in exception handling to make the code cleaner and much more readable. It simplifies the management of resources like file streams. Here’s how you can use the ‘with’ statement to read a file:
with open('myfile.txt', 'r') as file:
for line in file:
print(line)
In this code snippet, the ‘with’ statement automatically takes care of closing the file once it’s no longer needed. This not only makes the code simpler and more readable but also helps prevent bugs that could occur if the file was accidentally left open.
Handling Large Files in Python
When dealing with large files, reading the entire file into memory can be inefficient or even impossible if the file is larger than the available memory. In such cases, you can read the file line by line or in chunks. We’ve already seen how to read a file line by line using a ‘for’ loop. Here’s how you can read a large file in chunks:
with open('largefile.txt', 'r') as file:
while True:
chunk = file.read(1024)
if not chunk:
break
process(chunk)
In this code snippet, we’re reading the file in chunks of 1024 bytes and processing each chunk separately. This method allows you to handle large files efficiently without loading the entire file into memory.
Error Handling and Exceptions in File Handling
Error handling is an important aspect of file handling. When working with files, many things can go wrong. The file might not exist, you might not have permission to access it, or there could be an error while reading or writing the file. Python provides exceptions that you can catch and handle these errors gracefully:
try:
with open('myfile.txt', 'r') as file:
for line in file:
print(line)
except FileNotFoundError:
print('File not found')
except IOError:
print('An error occurred while reading the file')
In this code snippet, we’re using a try/except block to catch and handle FileNotFoundError
and IOError
exceptions. This allows us to provide a helpful error message instead of letting the program crash.
Python Libraries for Enhanced File Handling
Python’s standard library provides a strong foundation for file handling, but the language’s prowess doesn’t stop there. Several third-party libraries, such as Pandas, can further streamline file handling in Python and offer additional features that aren’t available in the standard library.
This section will explore these libraries and their benefits.
Boosting Efficiency with Pandas
Pandas is a highly-regarded Python library for data analysis and manipulation. It introduces two powerful data structures: Series and DataFrame, which greatly simplify data manipulation in Python. But Pandas also significantly simplifies file handling in Python.
Pandas offers several functions to read different types of files, including read_csv
for CSV files, read_excel
for Excel files, and read_json
for JSON files. These functions read the file and return a DataFrame, which you can then manipulate using Pandas’ data manipulation functions.
Here’s an example of using Pandas to read a CSV file:
import pandas as pd
data = pd.read_csv('myfile.csv')
print(data)
In this code snippet, we’re using Pandas to read a CSV file and print its contents. It’s as straightforward as that!
Libraries like Pandas can significantly streamline file handling in Python. They provide high-level functions that abstract away the low-level details of file handling, enabling you to read and write data with just a few lines of code. Additionally, they offer extra features, such as reading and writing data in various formats, handling missing data, and executing data manipulation tasks.
Reading Files Line by Line with Python Libraries
While Python libraries like Pandas are often used to read entire files at once, they can also be used to read files line by line. For instance, you can use the chunksize
parameter in Pandas’ read_csv
function to read a large CSV file in chunks:
Example of using Pandas to read a file line by line:
import pandas as pd
with open('myfile.csv', 'r') as file:
for line in pd.read_csv(file, chunksize=1):
print(line)
And an example where we read 1000 lines at a time:
import pandas as pd
for chunk in pd.read_csv('largefile.csv', chunksize=1000):
process(chunk)
In this code snippet, we’re reading a large CSV file in chunks of 1000 lines and processing each chunk separately. This method allows us to handle large files efficiently without loading the entire file into memory.
Further Resources for Python File I/O Operations
To expand your Python file handling skills here are some articles that you might find useful:
- Python OS Module Efficiency: Key Points – Master the art of searching for files and directories using “os” functions.
Python File Reading Techniques – Dive into the world of file reading modes and understand text and binary files.
Efficient File Writing in Python – Quick tips on writing data to files for data persistence and storage.
Tutorial on File Handling in Python offers a thorough understanding of how to handle and manipulate files in Python.
File handling functions in Python – This article explains Python’s built-in functions for manipulating files.
Python Advanced File Handling: Youtube video offers a detailed guide on advanced file handling techniques in Python.
Take the time to explore these resources and integrate them into your learning roadmap. Your journey in Python development is just getting started, and there’s much more to explore and learn!
Final Words
Throughout this comprehensive guide, we’ve delved into the various methods Python offers for reading files line by line.
We started off with the basics, discussing the ‘readline’, ‘readlines’, and ‘for’ loop methods, each with their unique advantages and use cases.
We then took a deeper dive into the world of file handling, exploring the ‘with’ statement, handling large files, error handling, and file management libraries like Pandas.
Whether you’re a beginner starting your Python journey or an experienced developer looking to brush up your skills, understanding Python’s file handling capabilities can help you write more efficient, cleaner, and more readable code. So keep exploring, keep learning, and harness the power of Python in your data processing tasks.