Python OS Module: A Comprehensive Guide
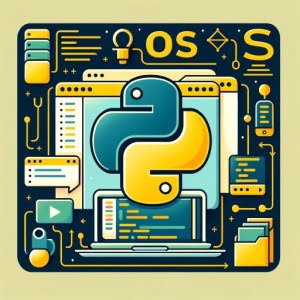
Are you finding it difficult to interact with your operating system using Python? You’re not alone. Many developers face this challenge, but there’s a tool in Python that can make this process a breeze.
Just like a universal remote, Python’s OS module can control many aspects of the operating system. It provides a way of using operating system dependent functionality, making it a powerful tool in a developer’s arsenal.
This guide will walk you through the ins and outs of using the OS module in Python, from basic use to advanced techniques. We’ll explore the OS module’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the Python OS module!
TL;DR: What is the OS Module in Python?
The OS module in Python is a built-in module that provides a way of using operating system dependent functionality. It allows Python programs to interact with the operating system in a variety of ways, such as reading from and writing to the file system, starting and stopping processes, and more.
Here’s a simple example:
import os
print(os.name)
# Output:
# 'posix'
In this example, we import the OS module and print the name of the operating system. The output ‘posix’ indicates that the underlying operating system is a Unix-like system such as Linux or MacOS.
This is just a basic introduction to the OS module in Python. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Getting Started with Python OS Module
The OS module in Python is a built-in module, which means you don’t need to install anything to start using it. You can simply import it into your script using the import
statement.
import os
Once you’ve imported the OS module, you can start using its functions. Let’s start with a simple example. To start, you can use os
module to get the list of files and directories in a given directory.
import os
# Get the list of all files and directories in the root directory
path = "/"
dir_list = os.listdir(path)
print("Files and directories in '", path, "' :")
print(dir_list)
The os.listdir()
function returns a list containing names of the entries in the directory given by the path
. If no path is specified, it returns from the current directory. Please replace the path
variable by your desired directory path.
The output will look something like this:
Files and directories in ' / ' :
['home', 'usr', 'var', 'lib', 'etc', 'bin', 'sbin', 'boot', 'tmp', 'srv', 'root', 'opt', 'mnt', 'media', 'proc', 'sys', 'dev', '.dockerenv']
The OS module in Python provides a host of other functions that allow you to interact with the operating system. For example, you can use os.getcwd()
to get the current working directory, os.listdir()
to list all files and directories in the current directory, and os.mkdir()
to create a new directory.
import os
# Get the current working directory
print(os.getcwd())
# List all files and directories in the current directory
print(os.listdir())
# Create a new directory
os.mkdir('test_directory')
# Verify if the new directory has been created
print('test_directory' in os.listdir())
# Output:
# /home/user
# ['file1', 'file2', 'dir1']
# True
In this code block, we first print the current working directory. Then we list all files and directories in the current directory. After that, we create a new directory named ‘test_directory’. Finally, we verify if the new directory has been created by checking if ‘test_directory’ is in the list of files and directories.
The OS module is a powerful tool that can help you automate and script many tasks that would otherwise require manual intervention. However, it’s also a tool that requires careful use. For example, functions like os.remove()
and os.rmdir()
can delete files and directories, so you should always double-check your code to avoid accidentally deleting important files.
Advanced Python OS Module Techniques
As we delve deeper into the Python OS module, we’ll discover that it offers much more than just basic file and directory operations. It also provides tools for manipulating process parameters and interacting with environment variables, among other things.
Manipulating Process Parameters
The Python OS module provides several functions for interacting with process parameters. For example, the os.getpid()
function returns the current process ID, while the os.getppid()
function returns the parent process ID.
import os
# Get the current process ID
print(os.getpid())
# Get the parent process ID
print(os.getppid())
# Output:
# 12345
# 12344
In this example, ‘12345’ is the ID of the current process, and ‘12344’ is the ID of the parent process.
Interacting with Environment Variables
Environment variables are a type of variable that is available system-wide and can be accessed by any process. The Python OS module provides several functions for interacting with environment variables. For example, the os.environ
object is a dictionary-like object that allows you to access and modify the current environment variables.
import os
# Get the value of the 'HOME' environment variable
print(os.environ['HOME'])
# Set a new environment variable
os.environ['TEST'] = 'Hello, World!'
# Verify that the new environment variable has been set
print(os.environ['TEST'])
# Output:
# /home/user
# Hello, World!
In this example, we first get the value of the ‘HOME’ environment variable, which typically contains the path to the current user’s home directory. Then we set a new environment variable named ‘TEST’ and verify that it has been set correctly.
These are just a few examples of the advanced functionality provided by the Python OS module. With a little creativity, you can use these tools to automate and script complex tasks, making your Python programs more powerful and flexible.
Exploring Alternatives to Python OS Module
While the Python OS module is a powerful tool for interacting with the operating system, it’s not the only tool available. There are other modules, such as the sys and subprocess modules, that also provide ways to interact with the operating system.
The Sys Module
The sys module provides access to some variables used or maintained by the Python interpreter and to functions that interact strongly with the interpreter. It is always available.
Here’s a simple example where we use the sys module to print out the Python version:
import sys
# Print Python version
print(sys.version)
# Output:
# 3.9.1 (default, Dec 11 2020, 06:28:49)
# [GCC 10.2.0]
In this example, we import the sys module and print the Python version. The output indicates the Python version along with additional information about the build.
The Subprocess Module
The subprocess module allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. It’s a more powerful tool for running shell commands from Python scripts.
Here’s an example where we use the subprocess module to list files in the current directory:
import subprocess
# Run a shell command and get its output
output = subprocess.check_output('ls', universal_newlines=True)
# Print the output
print(output)
# Output:
# file1
# file2
# dir1
In this example, we use the subprocess.check_output()
function to run the ‘ls’ command, which lists files in the current directory. The universal_newlines=True
argument causes the output to be returned as a string instead of bytes.
While the sys and subprocess modules offer powerful alternatives to the OS module, they also come with their own complexities and potential pitfalls. For example, the subprocess module requires careful handling of shell commands to avoid security vulnerabilities. As always, it’s essential to understand the tools you’re using and the implications of your code.
Troubleshooting Common Python OS Module Issues
As you work with the Python OS module, you may encounter certain issues. This section aims to discuss common problems and provide solutions and workarounds.
OS Compatibility Issues
The Python OS module is designed to work with various operating systems, but not all functions are available on all platforms. For instance, certain functions are only available on Unix-like systems and not on Windows.
To avoid compatibility issues, it’s important to check the documentation and ensure that the functions you’re using are supported on your target operating system.
Permission Errors
When working with file and directory operations, you might encounter permission errors. These errors occur when you try to access a file or directory that your user does not have permission to access.
For example, trying to delete a file that you don’t have permission to delete will result in a permission error:
import os
try:
os.remove('/path/to/file')
except PermissionError:
print('Permission error: Unable to delete file')
# Output:
# Permission error: Unable to delete file
In this example, we try to delete a file. If we don’t have permission to delete the file, a PermissionError
is raised, and we print a message indicating the problem.
To avoid permission errors, ensure that your user has the necessary permissions for the files and directories you’re working with. If you’re writing a script for others to use, consider adding error handling to gracefully handle permission errors and provide useful feedback to the user.
Remember, the Python OS module is a powerful tool, but with that power comes responsibility. Always double-check your code to avoid unintentionally modifying or deleting important files.
Python OS Module: Under the Hood
Before we delve further into the advanced use cases of the Python OS module, it’s essential to understand its fundamentals.
The OS Module in Python
The OS module in Python is a built-in module, which means it comes with the standard Python library and you don’t need to install it separately. It provides a way of using operating system dependent functionality, such as reading or writing to the file system, starting or stopping processes, and much more.
Under the hood, the OS module provides a portable way of using operating system specific features. It does this by exposing functions that the underlying operating system provides in its system calls. This is why some functions in the OS module are not available on all operating systems.
Python and the Operating System
When you run a Python program, it’s not just interacting with the code you’ve written. It’s also interacting with the operating system. The operating system is the software that manages your computer’s hardware and software resources. It provides services such as file management, process scheduling, and memory management.
Python, like other high-level programming languages, provides an abstraction layer between your code and the operating system. This abstraction layer makes it easier to write code that works on multiple operating systems. The Python OS module is part of this abstraction layer.
Let’s take a look at a simple example of how Python interacts with the operating system using the OS module:
import os
# Get the current working directory
current_directory = os.getcwd()
print(current_directory)
# Output:
# '/home/user'
In this example, we use the os.getcwd()
function to get the current working directory. This function interacts with the operating system to get this information and then returns it to your Python program.
Understanding these fundamentals will help you better understand the examples and techniques discussed in the rest of this guide.
Python OS Module in Larger Projects
The Python OS module is not just for small scripts or one-off tasks. It can also play a significant role in larger projects, such as web applications and data analysis.
Python OS Module in Web Applications
In web applications, the Python OS module can be used for a variety of tasks. For instance, it can be used to read and write files, which is useful for tasks like logging and data storage. It can also be used to interact with the underlying operating system in ways that are specific to the needs of the application.
Python OS Module in Data Analysis
In data analysis, the Python OS module can be particularly useful for managing data files. For instance, you can use it to list all files in a directory, read data from files, and write data to files. This can be especially helpful when you’re working with large datasets that are stored across multiple files.
Expanding Your Knowledge
While this guide provides a comprehensive overview of the Python OS module, there’s always more to learn. You might want to explore related concepts like file handling, process management, and more. Here are a few resources to help you dive deeper:
- Python GetCurrentDirectory() A guide to retrieving the current directory in Python effortlessly.
How to Open Python Files – Discover the methods of file reading and writing using different modes.
Python’s official OS module documentation gives insights about Python’s OS module and operating system interactions.
Python’s official tutorial on input and output teaches how to handle various input and output operations in Python.
Real Python’s detailed guide on managing files in Python offers practical approaches to work with files in Python.
Wrapping Up: Mastering the Python OS Module
In this comprehensive guide, we’ve ventured into the world of Python’s OS module, a powerful tool for interacting with the operating system.
We began with the basics, understanding how to import and use the OS module for simple tasks like getting the current working directory, listing files, and creating new directories. We then delved into more advanced topics, exploring how the OS module can be used to manipulate process parameters and interact with environment variables.
Along the way, we encountered common issues that might arise when using the OS module, such as OS compatibility issues and permission errors, and provided solutions and workarounds for each.
We also discussed alternative approaches to interacting with the operating system, introducing the sys and subprocess modules as other tools in Python’s arsenal.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Complexity |
---|---|---|
Python OS Module | File and Directory Operations, Process Parameters, Environment Variables | Moderate |
Sys Module | Access Python Interpreter Variables and Functions | Low |
Subprocess Module | Run Shell Commands, Manipulate Processes | High |
Whether you’re a beginner just starting out with the Python OS module or an intermediate user looking to level up, we hope this guide has provided you with a deeper understanding and practical knowledge of the OS module and its capabilities.
The OS module is a powerful tool in Python’s standard library, providing a host of functionalities to interact with the underlying operating system. Now, you’re well equipped to harness this power in your Python programs. Happy coding!