Python Open File | Quick Start Guide
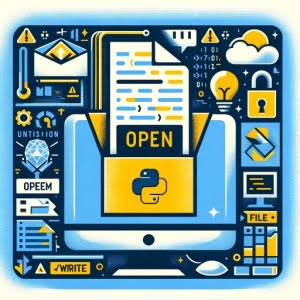
Ever found yourself struggling to open files in Python? If so, you’re not alone. Many beginners, and even some experienced programmers, can stumble over this seemingly simple task. But don’t worry – just like a key opens a door, Python uses specific functions to unlock the contents of a file.
In this comprehensive guide, we’ll walk you through the process of opening files in Python. We’ll start from the basics and gradually move on to more advanced techniques. Whether you’re a newbie just getting started or a seasoned pro looking for a refresher, this guide has got you covered.
So, buckle up and get ready to dive deep into the world of Python file handling!
TL;DR: How Do I Open a File in Python?
You can open a file in Python using the
open()
function. Here’s a basic example:
file = open('myfile.txt', 'r')
print(file.read())
file.close()
# Output:
# [Contents of 'myfile.txt']
This code opens ‘myfile.txt’ in read mode, prints its contents, and then closes the file. It’s a simple and straightforward way to access the contents of a file in Python. But remember, this is just the tip of the iceberg. There’s much more to learn about opening files in Python, including different modes of operation and handling various file types. So, keep reading for a more detailed exploration and advanced usage scenarios.
Table of Contents
- Mastering the Basics: Python’s open() Function
- Going Deeper: Advanced File Handling in Python
- Exploring Alternatives: Other Ways to Open Files in Python
- Overcoming Challenges: Common Issues and Their Solutions
- Understanding Python’s File Handling Capabilities
- Expanding Horizons: The Bigger Picture of File Handling
- Wrapping Up: Python File Opening Demystified
Mastering the Basics: Python’s open()
Function
Python’s open()
function is the key that unlocks the door to file handling. Its basic syntax is quite straightforward:
open(file, mode)
Here, file
is a string that specifies the file name or the file path, and mode
is an optional string that defines which mode you want to open the file in.
There are several modes available in Python, including:
- ‘r’ for read mode (default)
- ‘w’ for write mode
- ‘a’ for append mode
Let’s take a look at a simple example:
file = open('myfile.txt', 'r')
print(file.read())
file.close()
# Output:
# [Contents of 'myfile.txt']
In this example, we’re opening ‘myfile.txt’ in read mode (‘r’), printing its contents with the read()
method, and then closing the file with the close()
method. It’s crucial to close files after you’re done with them to free up system resources.
The open()
function is simple, yet powerful. It provides a straightforward way to handle files in Python. However, it’s important to handle files properly to avoid potential pitfalls, such as memory leaks from not closing files or data loss from opening a file in write mode (‘w’) when you meant to open it in read mode (‘r’).
Going Deeper: Advanced File Handling in Python
Once you’ve mastered the basics of Python’s open()
function, it’s time to explore more advanced usage scenarios. This involves dealing with different file types (text, binary, etc.) and using different file modes.
Different File Types
Python can handle various file types, including text files and binary files. The mode in which you open a file depends on its type. For text files, you use ‘t’ (the default), and for binary files, you use ‘b’.
Here’s how you can read a binary file:
file = open('myfile.bin', 'rb')
print(file.read())
file.close()
# Output:
# b'[Binary content of 'myfile.bin']'
Different File Modes
Python’s open()
function supports several file modes beyond the basic ‘r’, ‘w’, and ‘a’. Some of the more advanced modes include:
- ‘rb’, ‘wb’, ‘ab’ for reading, writing, and appending binary files
- ‘r+’, ‘w+’, ‘a+’ for reading and writing text files
- ‘rb+’, ‘wb+’, ‘ab+’ for reading and writing binary files
Here’s an example of opening a file in ‘r+’ mode, which allows both reading and writing:
file = open('myfile.txt', 'r+')
file.write('Hello, Python!')
file.seek(0) # Move the file pointer to the beginning
print(file.read())
file.close()
# Output:
# Hello, Python!
In this example, we first write ‘Hello, Python!’ to ‘myfile.txt’, then move the file pointer back to the beginning using the seek()
method, and finally read and print the file’s contents.
Understanding these different file types and modes is crucial for advanced file handling in Python. It allows you to handle a wider range of files and perform more complex tasks. Remember to always close your files after you’re done with them, and be careful when using modes that can modify the file, like ‘w’, ‘w+’, ‘a’, and ‘a+’.
Exploring Alternatives: Other Ways to Open Files in Python
As you become more proficient in Python, you’ll discover that there are alternative approaches to opening files. These methods can offer more convenience or functionality, depending on your specific needs.
Automatic File Closing: The with
Statement
One of the most common pitfalls in file handling is forgetting to close a file after you’re done with it. Python offers a solution to this problem: the with
statement, which automatically closes the file once you’re done with it.
Here’s how you can use it:
with open('myfile.txt', 'r') as file:
print(file.read())
# Output:
# [Contents of 'myfile.txt']
In this example, the with
statement automatically closes ‘myfile.txt’ after it’s read, saving you from potential memory leaks.
Handling CSV Files: The pandas Library
If you’re dealing with CSV files, Python’s built-in csv
module can get the job done. But for more advanced tasks, the pandas library is a powerful tool. It can handle large datasets with ease and offers a wide range of data manipulation functions.
Here’s an example of how you can use pandas to read a CSV file:
import pandas as pd
df = pd.read_csv('myfile.csv')
print(df)
# Output:
# [DataFrame representation of 'myfile.csv']
In this example, pandas reads ‘myfile.csv’ into a DataFrame, a two-dimensional data structure that’s easy to manipulate.
These alternative approaches to opening files in Python can make your code more efficient and readable. However, they may not be necessary for all tasks. For simple file handling, Python’s open()
function is often more than enough. Always consider your specific needs and the resources at your disposal when choosing a method to open files.
Overcoming Challenges: Common Issues and Their Solutions
As you work with Python to open files, you may encounter some common issues. These can include ‘FileNotFoundError’, problems with file paths, and more. But don’t worry – every problem has a solution.
Handling ‘FileNotFoundError’
One of the most common issues you’ll encounter when opening files in Python is ‘FileNotFoundError’. This error occurs when Python can’t locate the file you’re trying to open. Here’s an example:
try:
file = open('nonexistent_file.txt', 'r')
except FileNotFoundError:
print('File not found.')
# Output:
# File not found.
In this example, we’re trying to open ‘nonexistent_file.txt’, which doesn’t exist. Python raises a ‘FileNotFoundError’, which we catch and handle by printing ‘File not found.’
Dealing with File Paths
Another common issue is problems with file paths. If you’re not in the correct directory or if you don’t provide the correct path to your file, Python won’t be able to open it. Here’s how you can specify an absolute file path in Python:
file = open('/path/to/myfile.txt', 'r')
print(file.read())
file.close()
# Output:
# [Contents of '/path/to/myfile.txt']
In this example, we’re opening ‘myfile.txt’ located at ‘/path/to/myfile.txt’. By providing the absolute path to the file, we can open it regardless of our current directory.
These are just a few of the common issues you may encounter when opening files in Python. Always remember to handle exceptions, provide correct file paths, and close your files when you’re done with them. With these considerations in mind, you’ll be able to handle files in Python with confidence and ease.
Understanding Python’s File Handling Capabilities
Before we delve deeper into the practical aspects of opening files in Python, it’s important to understand the fundamentals of Python’s file handling capabilities. This includes the concept of file objects, file modes, and the importance of closing files.
The Concept of File Objects
In Python, when you open a file using the open()
function, you create a file object. This object acts as a bridge between your Python program and the file you’re working with. Here’s an example:
file = open('myfile.txt', 'r')
print(type(file))
file.close()
# Output:
# <class '_io.TextIOWrapper'>
In this example, we open ‘myfile.txt’ and print the type of the file
object, which is ‘_io.TextIOWrapper’. This shows that file
is indeed a file object.
File Modes in Python
File modes determine how you interact with a file. As we’ve seen, Python supports several file modes, including ‘r’ for reading, ‘w’ for writing, and ‘a’ for appending. Choosing the right mode is crucial for successful file handling.
The Importance of Closing Files
Closing files is an essential aspect of file handling in Python. If you don’t close a file after you’re done with it, you can end up wasting system resources. You can close a file using the close()
method:
file = open('myfile.txt', 'r')
file.close()
print(file.closed)
# Output:
# True
In this example, we open ‘myfile.txt’, close it, and then check if it’s closed using the closed
attribute, which returns True
.
Understanding these fundamentals is key to mastering file handling in Python. With this knowledge in hand, you’ll be better equipped to open files in Python and handle them effectively.
Expanding Horizons: The Bigger Picture of File Handling
So far, we’ve focused on the specifics of opening files in Python. But it’s important to understand that these skills are not just theoretical – they have practical applications in larger projects and real-world scenarios.
File Handling in Data Analysis and Web Scraping
Data analysis and web scraping are two fields where file handling is crucial. For instance, you might need to open a CSV file to analyze data or save scraped data to a text file. Here’s an example of how you can use the pandas
library to read a CSV file for data analysis:
import pandas as pd
df = pd.read_csv('data.csv')
print(df.describe())
# Output:
# [Statistical summary of 'data.csv']
In this example, we read ‘data.csv’ into a pandas DataFrame and then print a statistical summary of the data using the describe()
method.
Exploring Related Concepts
Once you’re comfortable with opening files in Python, there are many related concepts to explore. These can include file I/O operations, working with different file formats, and more. For example, you might want to learn how to read and write JSON or XML files, or how to handle binary data.
Further Resources
To deepen your understanding of file handling in Python, consider exploring the following documentations:
- Python OS Module Explained – Discover the “os.path” submodule for path-related operations and validations.
Duplicating Files in Python – A tutorial on copying files between directories in Python.
How-To List Files in a Directory – Explore Python’s “os” module to list files in a directory.
JavaTpoint’s reading and writing files in Python guide teaches you about file operations in Python.
Programiz’s Python classes tutorial – This resource offers an in-depth understanding of classes in Python.
Python tutorial video on file handling – This Youtube video guide gives a visual explanation of handling files in Python.
Opening files in Python is a fundamental skill, but it’s just the beginning. By applying these related concepts in larger projects you can become a more versatile Python programmer.
Wrapping Up: Python File Opening Demystified
Throughout this comprehensive guide, we’ve explored the process of opening files in Python, starting from the basics and moving on to more advanced techniques. We’ve learned how to use Python’s open()
function, tackled common issues like ‘FileNotFoundError’, and even delved into alternative methods of file opening.
To summarize, here are the key takeaways:
- Python’s
open()
function is the key to file handling. It’s simple yet powerful, and can handle a wide range of file types and modes. - Common issues when opening files include ‘FileNotFoundError’ and problems with file paths. These can be avoided by handling exceptions and providing correct file paths.
- Alternative approaches to opening files include using the
with
statement for automatic file closing and the pandas library for handling CSV files.
Here’s a comparison of the methods we’ve discussed:
Method | Use Case | Advantages | Disadvantages |
---|---|---|---|
open() function | Basic file opening | Simple, versatile | Must remember to close files |
with statement | Automatic file closing | Convenient, avoids memory leaks | None |
pandas library | Handling CSV files | Powerful, easy data manipulation | Overkill for simple tasks |
Remember, the best method to open files depends on your specific needs and the resources at your disposal. Whether you’re a beginner just getting started or a seasoned pro looking for a refresher, we hope this guide has given you a deeper understanding of how to open files in Python.