Python f-string | Usage Guide (With Examples)
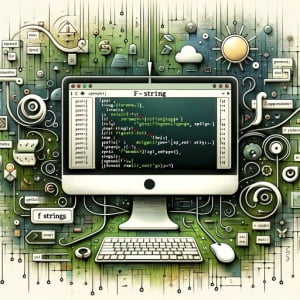
Are you perplexed by f-string formatting in Python? Imagine it as a language interpreter, transforming your raw data into a human-readable string format.
This comprehensive guide is designed to navigate you through the labyrinth of using f-string formatting in Python. We’ll start with the basics and gradually move towards more intricate techniques. By the end, you’ll be able to use Python f-strings like a pro, making your code more efficient and readable.
Let’s delve into the world of Python f-string formatting, unraveling its mysteries one step at a time.
TL;DR: How Do I Use F-String Formatting in Python?
In Python,
f-string
formatting is a technique that allows you to embed expressions inside string literals, making your code cleaner and easier to read. You can utilize f-string expressions by wrapping them in braces {} and the syntax,print(f'sample string, {expression}')
.
Here’s a quick example:
name = 'John'
age = 30
print(f'{name} is {age} years old.')
# Output:
# 'John is 30 years old.'
In the above example, we’ve created two variables – name
and age
. Using Python’s f-string formatting, we’ve embedded these variables directly into a print statement. The result is a neat and readable output: ‘John is 30 years old.’
If you’re intrigued and want to learn more about this powerful feature, continue reading for a deep dive into the world of Python f-string formatting, complete with detailed explanations and advanced usage scenarios.
Table of Contents
The Basics: F-String Formatting
F-string formatting, introduced in Python 3.6, is a way to embed variables and expressions inside string literals. This is incredibly useful for creating dynamic strings that include variable content.
Let’s start with a simple example. Suppose you have two variables, name
and age
, and you want to print a sentence that includes these variables. Here’s how you can do it with f-string formatting:
name = 'John'
age = 30
print(f'{name} is {age} years old.')
# Output:
# 'John is 30 years old.'
In the code above, we’ve created an f-string by prefixing the string with the letter ‘f’. Inside the string, we’ve included our variables inside curly braces {}
. Python automatically replaces these with the values of the variables when the string is printed.
This makes your code more readable and easier to maintain, as you don’t have to concatenate strings and variables using the ‘+’ operator. It also allows for more complex expressions to be embedded within the string, as we’ll see in the next section.
Advanced F-String Formatting
Python’s f-string formatting is not just about embedding simple variables. It’s a powerful tool that can handle different data types, complex expressions, and even formatting specifiers.
F-Strings with Different Data Types
Let’s start with different data types. Python f-strings can handle integers, floats, booleans, and more. Here’s an example:
name = 'John'
age = 30
is_married = False
print(f'{name} is {age} years old. Married: {is_married}')
# Output:
# 'John is 30 years old. Married: False'
In the code above, we’ve embedded a boolean variable is_married
into the f-string, along with the string variable name
and the integer variable age
.
Complex Expressions in F-Strings
You can also include more complex expressions in your f-strings. For example, you can perform arithmetic operations within an f-string. Let’s see how:
x = 5
y = 10
print(f'The sum of {x} and {y} is {x+y}.')
# Output:
# 'The sum of 5 and 10 is 15.'
In the above example, we’re performing the addition operation x+y
inside the f-string. Python evaluates this expression and includes the result in the output string.
Formatting Specifiers with F-Strings
Lastly, Python f-strings support formatting specifiers, which allow you to control how the embedded expressions are converted into strings. For example, you can control the number of decimal places for a float. Here’s how:
pi = 3.14159
print(f'The value of pi to 2 decimal places is {pi:.2f}.')
# Output:
# 'The value of pi to 2 decimal places is 3.14.'
In the above example, we’ve used the format specifier :.2f
inside the curly braces {}
. This tells Python to format the float pi
to 2 decimal places.
As you can see, Python’s f-string formatting is a versatile tool that can handle a wide range of scenarios, making your code more efficient and easier to read.
Alternative Formatting Methods
While Python’s f-string formatting is a powerful and efficient tool, it’s not the only way to format strings in Python. Two other popular methods are the str.format()
method and the %
operator. Let’s take a look at each of these.
The str.format() Method
Before f-strings were introduced in Python 3.6, the str.format()
method was a common way to format strings. Here’s an example of how it works:
name = 'John'
age = 30
print('{} is {} years old.'.format(name, age))
# Output:
# 'John is 30 years old.'
In the code above, we’ve used {}
placeholders in the string, and then filled these placeholders with variables using the str.format()
method. This method is still widely used and is compatible with older versions of Python.
The % Operator
The %
operator is another method for string formatting, which has its roots in C programming. Here’s an example:
name = 'John'
age = 30
print('%s is %d years old.' % (name, age))
# Output:
# 'John is 30 years old.'
In the above example, we’ve used %s
and %d
as placeholders for a string and an integer, respectively. The variables are then provided after the %
operator. This method is less intuitive and more error-prone than the other two, but it’s still used in certain scenarios, especially in older codebases.
Comparing the Three Methods
Each of these methods has its advantages and disadvantages. F-string formatting is the most modern and efficient method, offering a clean syntax and the ability to embed complex expressions. The str.format()
method is also clean and flexible, but it’s a bit more verbose than f-string formatting. The %
operator, while less intuitive, is still useful in certain scenarios and offers compatibility with older Python code.
In conclusion, while f-string formatting is a powerful tool in Python, it’s important to be familiar with these alternative methods, as you may encounter them in different coding scenarios or when working with older Python code.
Common Issues with Python F-String
As with any coding technique, you may encounter some challenges when working with Python f-string formatting. Let’s discuss some common issues and their solutions, along with tips for best practices and optimization.
Syntax Errors
One common issue is syntax errors. These can occur if you forget to prefix the string with ‘f’ or if you forget to enclose the variable or expression in curly braces {}
. Here’s an example:
name = 'John'
print(f'{name is 30 years old.')
# Output:
# SyntaxError: f-string: expecting '}'
In the code above, we’ve forgotten to close the curly brace after name
. Python raises a SyntaxError
, indicating that it’s expecting a closing brace. To fix this, simply ensure that every opening brace {
has a corresponding closing brace }
.
Type Errors
Another common issue is type errors. These can occur if you try to embed an object that can’t be converted to a string. Here’s an example:
class MyClass:
pass
obj = MyClass()
print(f'{obj}')
# Output:
# <__main__.MyClass object at 0x7f8c0a2e3c10>
In the code above, we’ve tried to embed an instance of a custom class MyClass
into the f-string. Python doesn’t know how to convert this object into a string, so it simply prints the object’s default string representation, which is not very helpful. To fix this, you can define a __str__
method in your class to provide a custom string representation.
Best Practices and Optimization
When using f-string formatting, it’s best to keep your embedded expressions simple and readable. While it’s possible to embed complex expressions, this can make your code harder to understand and maintain. If you find yourself embedding a complex expression, consider breaking it down into simpler parts or calculating the result beforehand.
In conclusion, while Python’s f-string formatting is a powerful and efficient tool, it’s important to be aware of these potential issues and how to fix them. By following these tips and best practices, you can write clean, efficient, and bug-free code.
Understanding Python Formatting
String formatting is a fundamental concept in Python, and understanding it is crucial for writing clean, efficient, and readable code. In essence, string formatting is the process of injecting data into a string in a specified format.
Python provides several ways to format strings, and these methods have evolved over time to become more powerful and flexible.
The Evolution of String Formatting in Python
In the early days of Python, string formatting was done using the %
operator, similar to the printf-style formatting in C. Here’s an example:
name = 'John'
age = 30
print('%s is %d years old.' % (name, age))
# Output:
# 'John is 30 years old.'
In the code above, we’ve used %s
and %d
as placeholders for a string and an integer, respectively. The variables are then provided after the %
operator.
However, this method has its drawbacks. It’s less intuitive, more error-prone, and doesn’t handle complex expressions well.
The Advent of F-String Formatting
To address these limitations, Python introduced the str.format()
method in Python 2.6, and then f-string formatting in Python 3.6. These newer methods offer a cleaner syntax, the ability to embed complex expressions, and better performance.
As we’ve seen in the previous sections, f-string formatting is a powerful tool that makes your code more efficient and easier to read. Understanding its basics and advanced usage, as well as its potential issues and how to troubleshoot them, can greatly enhance your Python coding skills.
Practica Uses of F-String Formatting
The power of Python’s f-string formatting extends beyond simple scripts and plays a crucial role in larger projects as well. It aids in generating dynamic content, logging, debugging, and more. For example, in a web application, f-strings can be used to generate dynamic HTML content based on user input or database queries.
user_name = 'John'
user_balance = 500
message = f'Hello, {user_name}! Your current balance is {user_balance} dollars.'
print(message)
# Output:
# 'Hello, John! Your current balance is 500 dollars.'
In the above example, user_name
and user_balance
could be variables that are retrieved from a database in a real-world web application. Using f-string formatting, we can easily construct a personalized message for the user.
Delving Deeper into Python
While mastering Python f-string formatting is a significant step, it’s just the tip of the iceberg. Python offers a plethora of other features and concepts that can help you write more efficient and powerful code.
For instance, you might want to explore string manipulation techniques, such as splitting and joining strings, replacing substrings, changing case, and more. Regular expressions in Python are another powerful tool for pattern matching and text processing.
As you continue your Python journey, remember to leverage the power of f-string formatting and beyond. There are plenty of resources available online, including Python’s official documentation, online courses, and tutorials, to help you deepen your understanding and hone your skills.
Further Resources for Python Strings
If you’re interested in learning more ways to handle strings in Python, here are a few resources that you might find helpful:
- Optimizing String Usage in Python for Better Code: Optimize your Python code by mastering the use of strings, focusing on performance and readability.
Python Docstring: Writing Documentation for Your Code: This article explains what a docstring is in Python and how to write effective documentation for your code.
How to Reverse a String in Python: Learn different techniques to reverse a string in Python, including built-in functions and slicing.
Python f-strings: An In-Depth Guide: An in-depth guide on Real Python that explains the usage and benefits of f-strings, which are a convenient way to format strings in Python.
Formatted String Literals (f-strings) in Python: An article on GeeksforGeeks that provides an overview of f-strings in Python and demonstrates their usage with examples.
Input and Output: Python Documentation: The official Python documentation on input and output, covering various techniques for reading input and writing output in Python programs.
Recap: Python F-String Formatting
We’ve journeyed through the world of Python f-string formatting, starting from the basics and venturing into more advanced techniques. We’ve seen how f-strings can embed variables and expressions, handle different data types, and even support formatting specifiers.
We’ve also explored common issues with f-string formatting and how to troubleshoot them, including syntax errors and type errors. We’ve discussed best practices for using f-strings, emphasizing the importance of keeping your embedded expressions simple and readable.
In addition, we’ve looked at alternative methods for string formatting in Python, namely the str.format()
method and the %
operator. Each of these has its own strengths and weaknesses, and the choice between them depends on your specific needs and the context of your code.
Here’s a quick comparison of the three methods:
Method | Advantages | Disadvantages |
---|---|---|
F-String Formatting | Clean syntax, powerful features, efficient | Requires Python 3.6 or higher |
str.format() Method | Flexible, compatible with older Python versions | More verbose than f-strings |
% Operator | Compatible with older Python code | Less intuitive, more error-prone |
In conclusion, Python’s f-string formatting is a powerful tool that can make your code more efficient and easier to read. Whether you’re a beginner or a seasoned Pythonista, mastering f-string formatting is a valuable skill that will serve you well in your Python journey.