ObjectMapper in Java: Conversion with the Jackson API
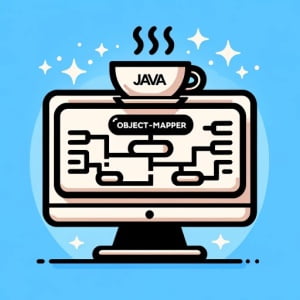
Are you finding it challenging to convert Java objects to JSON and vice versa? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled linguist, ObjectMapper, a key class in the Jackson API, is your bilingual interpreter, translating between the language of Java objects and JSON. These conversions are crucial in today’s data-driven applications, making ObjectMapper an essential tool for any Java developer.
This guide will walk you through the basics to advanced usage of ObjectMapper, helping you become fluent in this essential Java-JSON conversion tool. So, let’s dive in and start mastering ObjectMapper!
TL;DR: How Do I Use ObjectMapper in Java?
ObjectMapper
is a key class in the Jackson API used to convert Java objects to JSON and vice versa. Before use, it must be instantiated with the syntax,ObjectMapper mapper = new ObjectMapper();
. Here’s a quick example:
ObjectMapper mapper = new ObjectMapper();
String jsonString = mapper.writeValueAsString(myObject);
// Output:
// This will convert 'myObject' to a JSON string.
In this example, we create an instance of ObjectMapper and use its writeValueAsString
method to convert a Java object (myObject
) into a JSON string (jsonString
).
This is just a basic usage of ObjectMapper in Java, but there’s much more to learn about handling complex scenarios and advanced techniques. Continue reading for a comprehensive guide on mastering ObjectMapper.
Table of Contents
- ObjectMapper: Basic Java-JSON Conversion
- ObjectMapper: Advanced Java-JSON Conversion Techniques
- Exploring Alternative Libraries for Java-JSON Conversion
- Troubleshooting Common ObjectMapper Issues
- Understanding JSON and Java Objects
- The Relevance of Java-JSON Conversion in Modern Development
- Wrapping Up: ObjectMapper for Java-JSON Conversion
ObjectMapper: Basic Java-JSON Conversion
ObjectMapper’s primary function is to facilitate the conversion between Java objects and JSON. Let’s start with the basics: converting a Java object into a JSON string and vice versa.
Converting Java Object to JSON
Here’s an example of how you can convert a simple Java object into a JSON string using ObjectMapper:
public class Employee {
private String name;
private int age;
// getters and setters
}
Employee employee = new Employee();
employee.setName("John");
employee.setAge(30);
ObjectMapper mapper = new ObjectMapper();
String jsonString = mapper.writeValueAsString(employee);
// Output:
// {"name":"John","age":30}
In this example, we first define a simple Employee class with two properties: name and age. We then create an instance of this class, set some values, and finally use ObjectMapper’s writeValueAsString
method to convert this object into a JSON string.
Converting JSON to Java Object
Now, let’s reverse the process. Here’s how you can convert a JSON string back into a Java object:
String jsonString = "{\"name\":\"John\",\"age\":30}";
ObjectMapper mapper = new ObjectMapper();
Employee employee = mapper.readValue(jsonString, Employee.class);
// Output:
// Employee{name='John', age=30}
In this example, we start with a JSON string representing an employee. We then use ObjectMapper’s readValue
method to convert this JSON string back into an Employee object.
ObjectMapper makes these conversions straightforward and efficient. However, it’s important to handle potential exceptions that could occur during these operations, such as JsonProcessingException
. In the next section, we’ll explore more complex uses of ObjectMapper.
ObjectMapper: Advanced Java-JSON Conversion Techniques
While ObjectMapper’s basic usage is straightforward, it’s also a powerful tool for handling more complex scenarios. Let’s explore some of these advanced techniques.
Custom Serialization and Deserialization
ObjectMapper allows you to customize the serialization and deserialization process by using annotations like @JsonSerialize
and @JsonDeserialize
.
public class Employee {
private String name;
private int age;
@JsonSerialize(using = CustomSerializer.class)
private LocalDate birthDate;
// getters and setters
}
In this example, we use the @JsonSerialize
annotation to specify a custom serializer (CustomSerializer.class
) for the birthDate
field. This allows us to control how this field is converted into JSON.
Handling Dates
Handling dates can be tricky due to different date formats. ObjectMapper provides several ways to handle this, one of which is by using the @JsonFormat
annotation.
public class Employee {
private String name;
private int age;
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd")
private LocalDate birthDate;
// getters and setters
}
In this example, we use the @JsonFormat
annotation to specify the date format for the birthDate
field. This ensures that dates are correctly converted between Java and JSON.
Dealing with Optional Fields
Optional fields can be ignored during serialization or deserialization using the @JsonInclude
annotation.
@JsonInclude(JsonInclude.Include.NON_EMPTY)
public class Employee {
private String name;
private int age;
private Optional<String> nickname;
// getters and setters
}
In this example, the @JsonInclude
annotation is used to ignore the nickname
field if it’s empty. This helps prevent unnecessary fields from being included in the JSON output.
These are just a few examples of the advanced techniques you can use with ObjectMapper. By understanding and leveraging these techniques, you can handle a wide range of Java-JSON conversion scenarios.
Exploring Alternative Libraries for Java-JSON Conversion
While ObjectMapper is a powerful tool for Java-JSON conversion, it’s not the only option available. There are other libraries, such as Gson and JSON-B, which can also be used for this purpose. Let’s explore these alternatives.
Converting Java-JSON with Gson
Gson, a library provided by Google, is another popular choice for Java-JSON conversion. Here’s an example of how you can use Gson for this purpose:
import com.google.gson.Gson;
public class Employee {
private String name;
private int age;
// getters and setters
}
Employee employee = new Employee();
employee.setName("John");
employee.setAge(30);
Gson gson = new Gson();
String jsonString = gson.toJson(employee);
// Output:
// {"name":"John","age":30}
In this example, we use Gson’s toJson
method to convert a Java object into a JSON string. Similar to ObjectMapper, Gson also provides a fromJson
method for converting a JSON string back into a Java object.
Java-JSON Conversion with JSON-B
JSON-B is a Java API for JSON binding. It’s part of the Java EE, making it a good choice for developers already working with this platform. Here’s an example of how you can use JSON-B for Java-JSON conversion:
import javax.json.bind.Jsonb;
import javax.json.bind.JsonbBuilder;
public class Employee {
private String name;
private int age;
// getters and setters
}
Employee employee = new Employee();
employee.setName("John");
employee.setAge(30);
Jsonb jsonb = JsonbBuilder.create();
String jsonString = jsonb.toJson(employee);
// Output:
// {"name":"John","age":30}
In this example, we use JSON-B’s toJson
method to convert a Java object into a JSON string. Like ObjectMapper and Gson, JSON-B also provides a method for converting a JSON string back into a Java object.
Choosing the Right Library
Choosing the right library for Java-JSON conversion depends on your specific needs and the context of your project. ObjectMapper, Gson, and JSON-B all provide robust and flexible options for this task. However, ObjectMapper tends to be the most popular due to its extensive features and flexibility. If you’re already working with the Jackson library or need advanced features like custom serialization/deserialization, ObjectMapper would be a good choice. On the other hand, if you’re working with Java EE or prefer a simpler API, you might want to consider JSON-B. Lastly, if you’re looking for a lightweight and easy-to-use library, Gson could be a good fit.
Troubleshooting Common ObjectMapper Issues
While ObjectMapper is a powerful tool, you may encounter some common issues when using it. Let’s discuss these challenges and how to address them.
Handling Null Values
One common issue is dealing with null values during serialization. By default, ObjectMapper includes null fields in the JSON output. However, you can configure ObjectMapper to exclude null fields using the setSerializationInclusion
method.
ObjectMapper mapper = new ObjectMapper();
mapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
In this code block, we configure ObjectMapper to exclude null fields from the JSON output. This can be useful when you want to keep your JSON output clean and concise.
Dealing with Unknown Properties
Another common issue is dealing with unknown properties during deserialization. By default, ObjectMapper throws an exception when it encounters a JSON property that doesn’t match a property in the Java object. You can configure ObjectMapper to ignore unknown properties using the configure
method.
ObjectMapper mapper = new ObjectMapper();
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
In this code block, we configure ObjectMapper to ignore unknown properties during deserialization. This can be useful when your JSON data may contain additional properties that you don’t need to process.
Other Considerations
There are many other considerations when using ObjectMapper, such as handling date formats, customizing serialization/deserialization, and dealing with optional fields. It’s important to understand these considerations and how to address them to effectively use ObjectMapper for Java-JSON conversion.
Remember, every challenge presents an opportunity to learn and grow. By understanding these common issues and their solutions, you can become more proficient in using ObjectMapper and handling Java-JSON conversion.
Understanding JSON and Java Objects
Before we delve further into the advanced usage of ObjectMapper, it’s crucial to understand the fundamentals of JSON and Java objects, as well as the concepts of serialization and deserialization.
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. It’s a text format that’s completely language-independent but uses conventions familiar to programmers of the C family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
A basic JSON structure looks like this:
{
"name": "John",
"age": 30
}
In this example, name
and age
are properties of a JSON object. The values of these properties are John
and 30
, respectively.
What is a Java Object?
In Java, an object is an instance of a class. A class is a blueprint or template that describes the behavior/state that the object of its type supports. Here’s a simple Java object:
public class Employee {
private String name;
private int age;
// getters and setters
}
In this example, Employee
is a class with two properties: name
and age
. An instance of this class is a Java object.
Serialization and Deserialization
Serialization is the process of converting a Java object into a format (like JSON) that can be transmitted or stored. Deserialization is the reverse process: converting a JSON string back into a Java object.
ObjectMapper plays a crucial role in both these processes. It provides methods like writeValueAsString
for serialization and readValue
for deserialization, making Java-JSON conversion a breeze.
Understanding these fundamentals is key to mastering ObjectMapper and Java-JSON conversion.
The Relevance of Java-JSON Conversion in Modern Development
Java-JSON conversion, facilitated by tools like ObjectMapper, plays a crucial role in modern development practices, especially in the realm of REST APIs and microservices.
Java-JSON Conversion in REST APIs
REST APIs often use JSON as the format for exchanging data. When developing a REST API in Java, you’ll frequently need to convert Java objects to JSON for sending responses, and convert JSON to Java objects for processing requests. ObjectMapper simplifies these conversions, making it an invaluable tool for REST API development.
Java-JSON Conversion in Microservices
Microservices often communicate with each other using JSON over HTTP. ObjectMapper can help serialize and deserialize the data being exchanged, ensuring smooth communication between different microservices.
Handling JSON in Spring Boot
If you’re using Spring Boot for developing REST APIs or microservices, you’ll be glad to know that it integrates well with ObjectMapper. Spring Boot’s @RequestBody
and @ResponseBody
annotations use ObjectMapper under the hood for converting between Java objects and JSON.
Further Resources for Mastering Java-JSON Conversion
If you’re looking to dive deeper into Java-JSON conversion and ObjectMapper, here are some resources that you might find helpful:
- IOFlood’s blog post on Java Classes provides info – Java classes tutorial: practical tips and examples.
Mastering JSONObject Class in Java – Explore Java’s JsonObject class for handling of JSON objects in applications.
Exploring CompletableFuture Functionality – Learn concurrent and parallel processing using CompletableFuture in Java.
Tutorialspoint’s Jackson ObjectMapper Tutorial covers ObjectMapper concepts related to JSON parsing and serialization.
Baeldung’s The Guide to Jackson covers various features of the Jackson library, including ObjectMapper.
Java JSON Processing API Guide – An in-depth guide from Oracle on working with JSON in Java.
Mastering Java-JSON conversion with ObjectMapper will not only help you handle data more efficiently but also make you a more versatile Java developer.
Wrapping Up: ObjectMapper for Java-JSON Conversion
In this comprehensive guide, we’ve explored the ins and outs of ObjectMapper, a key class in the Jackson API for Java-JSON conversion.
We began with the basics, learning how to use ObjectMapper for simple Java-JSON conversions. We then delved into more advanced usage scenarios, such as custom serialization and deserialization, handling dates, and dealing with optional fields. Along the way, we tackled common challenges you might encounter when using ObjectMapper, such as handling null values and dealing with unknown properties, providing you with solutions and workarounds for each issue.
We also ventured into alternative approaches to Java-JSON conversion, comparing ObjectMapper with other libraries like Gson and JSON-B. Here’s a quick comparison of these libraries:
Library | Flexibility | Speed | Ease of Use |
---|---|---|---|
ObjectMapper | High | High | Moderate |
Gson | Moderate | High | High |
JSON-B | Moderate | Moderate | High |
Whether you’re just starting out with ObjectMapper or you’re looking to level up your Java-JSON conversion skills, we hope this guide has given you a deeper understanding of ObjectMapper and its capabilities.
With its balance of flexibility, speed, and power, ObjectMapper is a powerful tool for Java-JSON conversion. Now, you’re well-equipped to make the most of ObjectMapper in your Java projects. Happy coding!