CompletableFuture Java: Handling Asynchronous Tasks
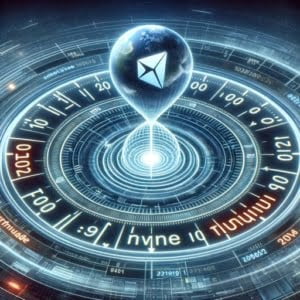
Are you finding it challenging to manage asynchronous tasks in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled orchestra conductor, CompletableFuture in Java is a handy utility that can seamlessly coordinate multiple threads. These threads can run on any system, even those without Java installed.
This guide will walk you through the process of using CompletableFuture in Java, from the basics to more advanced techniques. We’ll cover everything from creating a CompletableFuture, attaching a callback, handling exceptions, to combining multiple CompletableFutures and running them in parallel.
So, let’s dive in and start mastering CompletableFuture in Java!
TL;DR: How Do I Use CompletableFuture in Java?
CompletableFuture is a class in Java that allows you to write asynchronous, non-blocking code, instantiated with the syntax:
CompletableFuture<String> future = CompletableFuture.supplyAsync();
. It’s a powerful tool that can help you manage multiple threads with ease.
Here’s a simple example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello World");
future.thenAccept(System.out::println);
# Output:
# 'Hello World'
In this example, we create a CompletableFuture that runs a simple task asynchronously – it supplies the string ‘Hello World’. The thenAccept
method is then used to consume the result of the computation when it’s ready, without blocking the execution thread. In this case, it simply prints the result.
This is a basic way to use CompletableFuture in Java, but there’s much more to learn about managing asynchronous tasks effectively. Continue reading for a more detailed explanation and advanced usage examples.
Table of Contents
- Creating and Using CompletableFuture in Java
- Combining CompletableFutures: thenCompose and thenCombine
- Running Multiple CompletableFutures in Parallel
- Alternatives to CompletableFuture in Java
- Troubleshooting CompletableFuture in Java
- Understanding Asynchronous Programming in Java
- CompletableFuture in Real-World Applications
- Wrapping Up: CompletableFuture in Java
Creating and Using CompletableFuture in Java
In Java, creating a CompletableFuture is straightforward. Here’s a basic example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello CompletableFuture");
In this example, CompletableFuture.supplyAsync()
is used to create a CompletableFuture that asynchronously executes the provided Supplier function, which in this case, simply returns the string ‘Hello CompletableFuture’.
Attaching Callbacks: thenApply, thenAccept, and thenRun
Once you’ve created a CompletableFuture, you can attach callbacks using the thenApply
, thenAccept
, and thenRun
methods. These methods are used to handle the result of the computation once it’s ready.
Here’s how you can use these methods:
future.thenApply(result -> result.toUpperCase())
.thenAccept(result -> System.out.println("Result: " + result))
.thenRun(() -> System.out.println("Operation Completed."));
# Output:
# 'Result: HELLO COMPLETABLEFUTURE'
# 'Operation Completed.'
In this example, thenApply
is used to transform the result to uppercase. thenAccept
is then used to consume the result, in this case, by printing it. Finally, thenRun
is used to execute a Runnable once the computation is done. It doesn’t consume the result; instead, it simply performs an action.
Handling Exceptions
Just like any other operation in Java, CompletableFuture operations can throw exceptions. Here’s how you can handle them:
future.exceptionally(ex -> "An error occurred: " + ex.getMessage())
.thenAccept(System.out::println);
In this example, exceptionally
is used to handle any exceptions that might occur during the execution of the CompletableFuture. If an exception occurs, it returns a default value and prints an error message.
Combining CompletableFutures: thenCompose and thenCombine
In Java, you can combine multiple CompletableFutures using thenCompose
and thenCombine
methods.
The thenCompose
method is used when a CompletableFuture’s result is dependent on another CompletableFuture. Here’s an example:
CompletableFuture<String> firstTask = CompletableFuture.supplyAsync(() -> "Hello");
CompletableFuture<String> secondTask = firstTask.thenCompose(result -> CompletableFuture.supplyAsync(() -> result + " CompletableFuture"));
secondTask.thenAccept(System.out::println);
# Output:
# 'Hello CompletableFuture'
In this example, secondTask
is dependent on firstTask
. The thenCompose
method is used to chain these tasks together, so secondTask
won’t start until firstTask
is completed.
On the other hand, thenCombine
is used when you want to combine two independent CompletableFutures. Here’s an example:
CompletableFuture<String> firstTask = CompletableFuture.supplyAsync(() -> "Hello");
CompletableFuture<String> secondTask = CompletableFuture.supplyAsync(() -> " CompletableFuture");
CompletableFuture<String> combinedTask = firstTask.thenCombine(secondTask, (firstResult, secondResult) -> firstResult + secondResult);
combinedTask.thenAccept(System.out::println);
# Output:
# 'Hello CompletableFuture'
In this example, firstTask
and secondTask
are independent of each other. The thenCombine
method is used to combine their results once both are completed.
Running Multiple CompletableFutures in Parallel
In a real-world scenario, you might need to run multiple CompletableFutures in parallel. Here’s how you can do it:
CompletableFuture<String> firstTask = CompletableFuture.supplyAsync(() -> "Task 1");
CompletableFuture<String> secondTask = CompletableFuture.supplyAsync(() -> "Task 2");
CompletableFuture<Void> combinedTask = CompletableFuture.allOf(firstTask, secondTask);
firstTask.thenAccept(result -> System.out.println(result + " completed."));
secondTask.thenAccept(result -> System.out.println(result + " completed."));
combinedTask.thenRun(() -> System.out.println("All tasks completed."));
# Output:
# 'Task 1 completed.'
# 'Task 2 completed.'
# 'All tasks completed.'
In this example, CompletableFuture.allOf()
is used to create a CompletableFuture that is completed only when both firstTask
and secondTask
are completed. This allows you to run multiple CompletableFutures in parallel.
Alternatives to CompletableFuture in Java
While CompletableFuture is a powerful tool for handling asynchronous tasks, it’s not the only way to manage them in Java. Two other key approaches include using the Future interface and the ExecutorService class.
Exploring the Future Interface
The Future interface has been around since Java 5 and provides a way to handle asynchronous tasks but lacks some of the powerful features of CompletableFuture. Here’s a simple example of how it’s used:
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> "Hello Future");
executorService.shutdown();
try {
System.out.println(future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
# Output:
# 'Hello Future'
In this example, we create an ExecutorService and submit a Callable to it. The submit
method returns a Future, which we can use to retrieve the result of the computation once it’s ready. However, the get
method blocks until the computation is done, which is one of the limitations of the Future interface.
Leveraging the ExecutorService Class
The ExecutorService class is another way to handle asynchronous tasks in Java. It provides methods to manage and control thread execution in concurrent Java applications.
Here’s an example of how to use it:
ExecutorService executorService = Executors.newFixedThreadPool(2);
Future<String> future1 = executorService.submit(() -> "Task 1");
Future<String> future2 = executorService.submit(() -> "Task 2");
executorService.shutdown();
try {
System.out.println(future1.get() + " completed.");
System.out.println(future2.get() + " completed.");
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
# Output:
# 'Task 1 completed.'
# 'Task 2 completed.'
In this example, we create an ExecutorService with a fixed thread pool. We then submit two tasks to it, each returning a Future. We can use these Futures to retrieve the results of the computations once they’re done.
While ExecutorService provides more control over thread execution compared to the Future interface, it still lacks the ability to chain and combine Futures, which is one of the strengths of CompletableFuture.
Making the Right Choice
Choosing between CompletableFuture, Future, and ExecutorService depends on your specific needs. If you need to chain and combine Futures or want to leverage functional-style operations, CompletableFuture is the way to go. If you need more control over thread execution, consider using ExecutorService. If your needs are simple and you don’t mind blocking for results, the Future interface might be sufficient.
Troubleshooting CompletableFuture in Java
Like any tool, CompletableFuture comes with its own set of potential issues and considerations. Let’s explore some common challenges and their solutions.
Handling Exceptions
One common issue with CompletableFuture is dealing with exceptions. Unchecked exceptions thrown during the computation are wrapped in an ExecutionException
and thrown when you call get()
. Here’s an example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Exception during computation");
return "Hello CompletableFuture";
});
try {
System.out.println(future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
# Output:
# java.util.concurrent.ExecutionException: java.lang.RuntimeException: Exception during computation
In this example, an unchecked exception is thrown during the computation. When we call get()
, this exception is wrapped in an ExecutionException
and rethrown.
To handle this, you can use the exceptionally
method, as we discussed in the ‘Basic Use’ section.
Dealing with Blocking Code
Another common issue is dealing with blocking code. If you’re not careful, you can end up blocking the execution thread while waiting for a CompletableFuture to complete. Here’s an example:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello CompletableFuture");
try {
System.out.println(future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
# Output:
# 'Hello CompletableFuture'
In this example, the get()
method blocks the execution thread until the CompletableFuture is completed. To avoid this, you can use the thenApply
, thenAccept
, or thenRun
methods to attach a callback to the CompletableFuture, as we discussed in the ‘Basic Use’ section.
Managing Thread Pools
Finally, managing thread pools can be a challenge when using CompletableFuture. By default, CompletableFuture uses the ForkJoinPool.commonPool()
, but you can specify a custom Executor if you need more control over the thread pool. Here’s an example:
ExecutorService executorService = Executors.newFixedThreadPool(2);
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello CompletableFuture", executorService);
executorService.shutdown();
try {
System.out.println(future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
# Output:
# 'Hello CompletableFuture'
In this example, we create a custom ExecutorService with a fixed thread pool and pass it to supplyAsync()
. This allows us to control the thread pool used by the CompletableFuture.
Understanding Asynchronous Programming in Java
Asynchronous programming is a design pattern that allows multiple tasks to be executed concurrently, improving the performance of your application. In Java, this is achieved through the use of threads. A thread is a separate path of execution, allowing multiple tasks to be performed at the same time.
The Role of the Future Interface
In Java, the Future interface plays a crucial role in asynchronous programming. It represents the result of an asynchronous computation and provides methods to check if the computation is complete, to wait for its completion, and to retrieve the result of the computation.
Here’s a simple example:
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> future = executorService.submit(() -> "Hello Future");
executorService.shutdown();
try {
System.out.println(future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
# Output:
# 'Hello Future'
In this example, we submit a Callable to an ExecutorService, which returns a Future. We can then use the get()
method to retrieve the result of the computation, blocking if necessary until it is ready.
The CompletionStage Interface and CompletableFuture
The CompletionStage interface, introduced in Java 8, is a step up from Future. It represents a stage of a possibly asynchronous computation, that performs an action when the computation is complete.
CompletableFuture is a class that implements both the Future interface and the CompletionStage interface. It provides a large number of methods for creating, chaining, and combining multiple stages, making it a powerful tool for handling asynchronous tasks in Java.
Here’s a simple example of how CompletableFuture can be used:
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello CompletableFuture");
future.thenAccept(System.out::println);
# Output:
# 'Hello CompletableFuture'
In this example, CompletableFuture.supplyAsync()
is used to create a CompletableFuture that runs a task asynchronously. The thenAccept()
method is then used to consume the result of the computation when it’s ready, without blocking the execution thread.
CompletableFuture in Real-World Applications
CompletableFuture is not just a theoretical concept, it’s a practical tool that can be used in various real-world applications. Let’s explore some of them.
CompletableFuture in Web Programming
In web programming, CompletableFuture can be used to handle asynchronous tasks, such as making multiple HTTP requests simultaneously. This can significantly improve the performance of your web application by reducing the waiting time for IO operations.
CompletableFuture<HttpResponse<String>> future1 = HttpClient.newHttpClient().sendAsync(HttpRequest.newBuilder(URI.create("http://example.com")).build(), HttpResponse.BodyHandlers.ofString());
CompletableFuture<HttpResponse<String>> future2 = HttpClient.newHttpClient().sendAsync(HttpRequest.newBuilder(URI.create("http://example.org")).build(), HttpResponse.BodyHandlers.ofString());
CompletableFuture.allOf(future1, future2).join();
# Output:
# [The responses from the HTTP requests]
In this example, we use CompletableFuture.allOf()
to make two HTTP requests simultaneously. Once both requests are completed, the responses are available for further processing.
CompletableFuture for Database Access
Similarly, CompletableFuture can be used to handle asynchronous database operations. This can be particularly useful when you need to perform multiple database operations that don’t depend on each other.
CompletableFuture<ResultSet> future1 = CompletableFuture.supplyAsync(() -> database.query("SELECT * FROM table1"));
CompletableFuture<ResultSet> future2 = CompletableFuture.supplyAsync(() -> database.query("SELECT * FROM table2"));
CompletableFuture.allOf(future1, future2).join();
# Output:
# [The results of the database queries]
In this example, we use CompletableFuture.allOf()
to perform two database queries simultaneously. Once both queries are completed, the results are available for further processing.
CompletableFuture in Multi-Threaded Applications
In multi-threaded applications, CompletableFuture can be used to manage and coordinate multiple threads. This can help you create more efficient and responsive applications.
CompletableFuture<Void> future1 = CompletableFuture.runAsync(() -> System.out.println("Hello from Thread 1"));
CompletableFuture<Void> future2 = CompletableFuture.runAsync(() -> System.out.println("Hello from Thread 2"));
CompletableFuture.allOf(future1, future2).join();
# Output:
# 'Hello from Thread 1'
# 'Hello from Thread 2'
In this example, we use CompletableFuture.runAsync()
to run two tasks on separate threads. With CompletableFuture.allOf()
, we wait for both tasks to complete.
Further Resources for CompletableFuture
If you want to learn more about CompletableFuture and related topics, here are some resources you might find helpful:
- Defining and Using Java Classes: Tutorial – Discover the versatility of Java classes.
JFrame in Java: A Quick Guide – Dive into Java’s JFrame class for creating interactive graphical user interfaces.
A Java ObjectMapper Comprehensive Guide – Master the ObjectMapper JSON serialization and deserialization in Java.
Java CompletableFuture Tutorial with Examples – Practical guide that illustrates the use of Java CompletableFuture.
Asynchronous Programming in Java – A tutorial on understanding and implementing asynchronous programming in Java.
Java 8: CompletableFuture in Action – Explore the capabilities and applications of CompletableFuture in Java 8.
These resources cover a wide range of topics, from the basics of CompletableFuture to more advanced techniques. They also provide real-world examples that you can use as a reference in your own projects.
Wrapping Up: CompletableFuture in Java
In this comprehensive guide, we’ve delved into the world of CompletableFuture, a powerful tool in Java for handling asynchronous tasks.
We embarked with the basics, learning how to create a CompletableFuture and attach callbacks using methods like thenApply
, thenAccept
, and thenRun
. We also covered how to handle exceptions, a fundamental aspect of working with CompletableFuture.
Venturing into more advanced territory, we explored how to combine multiple CompletableFutures using thenCompose
and thenCombine
methods, and how to run multiple CompletableFutures in parallel. We also peered into alternative approaches for handling asynchronous tasks in Java, such as using the Future interface and the ExecutorService class, providing you with a broader landscape of tools for asynchronous programming.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
CompletableFuture | Powerful, supports chaining and combining Futures | May require troubleshooting for complex tasks |
Future | Simple and easy to use | Lacks advanced features of CompletableFuture |
ExecutorService | More control over thread execution | Lacks the ability to chain and combine Futures |
We also tackled common challenges you might encounter when using CompletableFuture, such as dealing with blocking code and managing thread pools. With each challenge, we provided solutions and workarounds to help you overcome them.
Whether you’re just starting out with CompletableFuture or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of CompletableFuture and its capabilities.
With its balance of power and flexibility, CompletableFuture is a powerful tool for handling asynchronous tasks in Java. Happy coding!