Python Nested Dictionary Guide (With Examples)
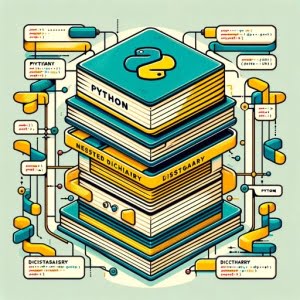
Are you finding it challenging to work with nested dictionaries in Python? You’re not alone. Many developers find themselves tangled in the branches of these complex data structures, but there’s a way to navigate through.
Think of a nested dictionary in Python as a family tree, where each branch can have its own smaller branches. It’s a powerful tool for handling complex data structures, providing a versatile and handy tool for various tasks.
This guide will walk you through the creation, manipulation, and access of nested dictionaries in Python. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Python nested dictionaries!
TL;DR: How Do I Create and Use a Nested Dictionary in Python?
A nested dictionary in Python is essentially a dictionary within a dictionary. It’s a powerful way to store and organize complex data structures in Python. Here’s a simple example:
my_dict = {'John': {'Age': 30, 'Job': 'Engineer'}, 'Jane': {'Age': 28, 'Job': 'Doctor'}}
print(my_dict['John']['Age'])
# Output:
# 30
In this example, we’ve created a nested dictionary my_dict
with two keys: ‘John’ and ‘Jane’. Each key has its own dictionary as a value, containing further key-value pairs. We then access the ‘Age’ of ‘John’ by chaining the keys, resulting in the output ’30’.
This is just a basic way to create and use a nested dictionary in Python. There’s much more to learn about manipulating and accessing data in nested dictionaries. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Creating and Manipulating Nested Dictionaries
- Advanced Techniques with Nested Dictionaries
- Exploring Alternatives to Nested Dictionaries
- Troubleshooting Nested Dictionaries in Python
- Understanding Python’s Dictionary Data Type
- Python Nested Dictionaries in Real-World Applications
- Wrapping Up: Mastering Python Nested Dictionaries
Creating and Manipulating Nested Dictionaries
Nested dictionaries in Python allow us to store data in a structured and flexible way. Let’s explore how to create, access, modify, and add new elements to a nested dictionary.
Creating a Nested Dictionary
Creating a nested dictionary is as simple as creating a regular dictionary. The only difference is that the values in a nested dictionary can be dictionaries themselves. Here’s an example:
my_dict = {
'John': {'Age': 30, 'Job': 'Engineer'},
'Jane': {'Age': 28, 'Job': 'Doctor'}
}
print(my_dict)
# Output:
# {'John': {'Age': 30, 'Job': 'Engineer'}, 'Jane': {'Age': 28, 'Job': 'Doctor'}}
In this example, we’ve created a nested dictionary my_dict
with two keys: ‘John’ and ‘Jane’. Each key has its own dictionary as a value, containing further key-value pairs.
Accessing Elements in a Nested Dictionary
Accessing elements in a nested dictionary involves using the keys from the outer dictionary and the inner dictionary. Here’s how you can do it:
print(my_dict['John']['Age'])
# Output:
# 30
By chaining the keys ‘John’ and ‘Age’, we’re able to access the age of John, which is 30.
Modifying Elements in a Nested Dictionary
Modifying elements in a nested dictionary is done by accessing the specific element using its keys and then assigning a new value to it. Here’s how:
my_dict['John']['Age'] = 31
print(my_dict['John']['Age'])
# Output:
# 31
In this example, we’ve changed the age of John from 30 to 31.
Adding New Elements to a Nested Dictionary
Adding new elements to a nested dictionary involves creating a new key in the outer dictionary or in any of the inner dictionaries and assigning a value to it. Here’s an example:
my_dict['John']['Salary'] = 70000
print(my_dict['John'])
# Output:
# {'Age': 31, 'Job': 'Engineer', 'Salary': 70000}
Here, we’ve added a new key-value pair ‘Salary’: 70000 to the dictionary of ‘John’.
Working with nested dictionaries can be tricky, especially for beginners. However, with a bit of practice, you’ll find that they are an incredibly powerful tool for managing complex data structures in Python.
Advanced Techniques with Nested Dictionaries
As you become more comfortable with nested dictionaries, you can start to explore more advanced techniques. This includes looping through a nested dictionary, using dictionary comprehension, and applying dictionary methods. Let’s dive into each of these.
Looping Through a Nested Dictionary
Looping through a nested dictionary allows you to access and manipulate each key-value pair in the dictionary. Here’s an example:
for person, info in my_dict.items():
for key in info:
print(f'{person}'s {key} is {info[key]}')
# Output:
# John's Age is 31
# John's Job is Engineer
# John's Salary is 70000
# Jane's Age is 28
# Jane's Job is Doctor
In this example, we’re using a nested for loop to iterate over each person’s information in the my_dict
nested dictionary. The outer loop iterates over each person, and the inner loop iterates over each piece of information for that person.
Using Dictionary Comprehension with Nested Dictionaries
Dictionary comprehension is a powerful tool that allows you to create new dictionaries in a single, readable line of code. It can also be used with nested dictionaries. Here’s an example:
ages = {person: info['Age'] for person, info in my_dict.items()}
print(ages)
# Output:
# {'John': 31, 'Jane': 28}
In this example, we’re using dictionary comprehension to create a new dictionary that contains each person’s age. The expression person: info['Age']
is the key-value pair for the new dictionary, and for person, info in my_dict.items()
is the loop.
Using Dictionary Methods with Nested Dictionaries
Python’s dictionary methods can also be used with nested dictionaries. For example, you can use the get()
method to safely access the value of a key. If the key doesn’t exist, get()
returns None
(or a default value of your choice) instead of raising an error:
print(my_dict.get('John').get('Height', 'Not provided'))
# Output:
# Not provided
In this example, we’re trying to access John’s height, which doesn’t exist in the dictionary. Instead of raising a KeyError, the get()
method returns ‘Not provided’.
These advanced techniques can help you to work more effectively with nested dictionaries in Python, allowing you to write more efficient and readable code.
Exploring Alternatives to Nested Dictionaries
While nested dictionaries are a powerful tool for handling complex data structures in Python, they are not the only solution. Alternatives such as classes or namedtuples can also be used, each with their own advantages and drawbacks. Let’s explore these alternatives.
Using Classes Instead of Nested Dictionaries
Classes in Python provide a way of bundling data and functionality together. They can be a more organized and readable alternative to nested dictionaries, especially when dealing with complex data structures:
class Person:
def __init__(self, name, age, job):
self.name = name
self.age = age
self.job = job
John = Person('John', 31, 'Engineer')
Jane = Person('Jane', 28, 'Doctor')
print(John.age)
# Output:
# 31
In this example, we’ve created a Person
class with attributes for name
, age
, and job
. We then create two instances of the class, John
and Jane
. We can access the age of John simply by using John.age
.
The advantage of using classes is that they provide a clear structure to the data and can bundle related functionality. However, they can be overkill for simple data structures and require more code to set up.
Using Namedtuples Instead of Nested Dictionaries
Namedtuples are a subclass of Python’s built-in tuple data type. They are immutable and can be a more memory-efficient alternative to nested dictionaries:
from collections import namedtuple
Person = namedtuple('Person', ['name', 'age', 'job'])
John = Person('John', 31, 'Engineer')
Jane = Person('Jane', 28, 'Doctor')
print(John.age)
# Output:
# 31
In this example, we’ve created a Person
namedtuple with fields for name
, age
, and job
. We then create two instances of the namedtuple, John
and Jane
. We can access the age of John simply by using John.age
.
The advantage of using namedtuples is that they are more memory-efficient and can be used as dictionary keys. However, they are immutable, meaning that once a namedtuple is created, it cannot be modified.
When deciding between nested dictionaries, classes, or namedtuples, consider the complexity of your data structure, the memory efficiency, and whether you need the data to be mutable or immutable.
Troubleshooting Nested Dictionaries in Python
Working with nested dictionaries in Python is not without its challenges. Let’s discuss some common issues you may encounter, such as KeyError, and how to resolve them.
Dealing with KeyError
One of the most common issues when working with nested dictionaries is a KeyError. This error occurs when you try to access a key that doesn’t exist in the dictionary. Here’s an example:
try:
print(my_dict['John']['Height'])
except KeyError:
print('Key does not exist.')
# Output:
# Key does not exist.
In this example, we’re trying to access the ‘Height’ of ‘John’, which doesn’t exist in the dictionary. This would normally raise a KeyError, but we’ve used a try-except block to catch the error and print a friendly message instead.
Tips for Working with Nested Dictionaries
Here are some tips to help you work more effectively with nested dictionaries in Python:
- Use the
get()
method: As we’ve seen earlier, theget()
method is a safe way to access a key’s value without raising an error if the key doesn’t exist. - Check if a key exists: You can check if a key exists in a dictionary before trying to access its value using the
in
keyword. - Use a default dictionary: Python’s
collections
module has adefaultdict
class that you can use to provide a default value for keys that don’t exist in the dictionary.
Working with nested dictionaries can be tricky, but with these tips and a bit of practice, you’ll be able to handle them effectively.
Understanding Python’s Dictionary Data Type
Before we dive deeper into nested dictionaries, it’s essential to understand the basics of Python’s dictionary data type and why it’s such a powerful tool for handling data.
Python’s Dictionary Data Type
A dictionary in Python is an unordered collection of items. Each item in a dictionary is stored as a key-value pair. Here’s a simple example:
simple_dict = {'Name': 'John', 'Age': 30, 'Job': 'Engineer'}
print(simple_dict['Name'])
# Output:
# John
In this example, we’ve created a dictionary simple_dict
with three key-value pairs. We can access the value of a key by using the key inside square brackets []
.
Why Dictionaries Are Useful
Dictionaries are incredibly versatile and efficient for data storage and retrieval. They allow you to store data in a way that’s easy to access and modify. Unlike lists or tuples, which use indices, dictionaries use keys, making it easier to retrieve values without needing to know their position.
The Concept of Nesting in Python
Nesting is a concept in Python where a data structure is used as an element of another data structure. For instance, a list can have other lists as its elements, and a dictionary can have other dictionaries as its values. This concept allows you to create complex data structures.
Nested Dictionaries: A Powerful Tool
Nested dictionaries take the power of dictionaries to a whole new level. They allow you to create complex data structures within a single dictionary, making it easier to organize and access data. As we’ve seen in the previous sections, you can create, access, modify, and loop through a nested dictionary in much the same way as a regular dictionary.
Understanding the fundamentals of Python’s dictionary data type and the concept of nesting will help you better understand and utilize nested dictionaries.
Python Nested Dictionaries in Real-World Applications
Nested dictionaries in Python are not just a theoretical concept — they have practical applications in various fields such as data analysis and web development.
Nested Dictionaries in Data Analysis
In data analysis, nested dictionaries can be used to store and manipulate complex data structures. For example, you might have a dataset where each entry consists of several attributes, and each attribute can have its own set of sub-attributes. A nested dictionary can conveniently store this data in a structured manner.
Nested Dictionaries in Web Development
In web development, nested dictionaries are often used to handle JSON data. JSON (JavaScript Object Notation) is a popular data format for exchanging data between a server and a web application. Since JSON data is structured as key-value pairs, it maps directly to a dictionary in Python. If the JSON data is complex, it can be represented as a nested dictionary.
Handling JSON Data in Python
Python has built-in support for JSON data. You can convert a JSON string into a dictionary using the json.loads()
function, and convert a dictionary into a JSON string using the json.dumps()
function. Here’s an example:
import json
# a JSON string
json_string = '{"John": {"Age": 30, "Job": "Engineer"}, "Jane": {"Age": 28, "Job": "Doctor"}}'
# convert JSON string to dictionary
my_dict = json.loads(json_string)
print(my_dict['John']['Age'])
# Output:
# 30
# convert dictionary to JSON string
json_string = json.dumps(my_dict)
print(json_string)
# Output:
# {"John": {"Age": 30, "Job": "Engineer"}, "Jane": {"Age": 28, "Job": "Doctor"}}
In this example, we first convert a JSON string into a nested dictionary using json.loads()
. We then access the age of John, which is 30. Finally, we convert the dictionary back into a JSON string using json.dumps()
.
Further Resources for Mastering Python Nested Dictionaries
If you want to dive deeper into nested dictionaries in Python, here are some resources that you might find useful:
- Mastering Python Dictionary Operations with IOFlood – Master Python dictionary syntax and become proficient in using this essential data structure.
Keeping Your Dictionaries Current: Python Update Dictionary – Master the art of updating dictionaries in Python.
Sorting Python Dictionaries by Value: A Step-by-Step Tutorial – Learn how to sort a Python dictionary by its values.
Python Documentation: Data Structures – The official Python documentation provides a thorough overview of dictionaries, including nested dictionaries.
Real Python: Dictionaries in Python – This article from Real Python goes in-depth on dictionaries and includes several examples and exercises.
Python Course: Advanced Dictionaries – This tutorial covers advanced topics on dictionaries, including dictionary comprehensions and nested dictionaries.
Wrapping Up: Mastering Python Nested Dictionaries
In this comprehensive guide, we’ve delved deep into the world of Python nested dictionaries, a powerful tool for managing complex data structures.
We began with the basics, learning how to create, access, modify, and add new elements to a nested dictionary. We then ventured into more advanced territory, exploring techniques such as looping through a nested dictionary, using dictionary comprehension, and applying dictionary methods.
Along the way, we tackled common challenges you might face when working with nested dictionaries, such as KeyError, providing you with solutions and tips for each issue.
We also looked at alternative approaches to handling complex data structures, comparing nested dictionaries with other data structures like classes and namedtuples.
Here’s a quick comparison of these methods:
Method | Flexibility | Memory Efficiency | Ease of Use |
---|---|---|---|
Nested Dictionaries | High | Moderate | High |
Classes | High | Low | Moderate |
Namedtuples | Low | High | High |
Whether you’re just starting out with Python nested dictionaries or you’re looking to level up your data management skills, we hope this guide has given you a deeper understanding of nested dictionaries and their capabilities.
With their balance of flexibility, memory efficiency, and ease of use, nested dictionaries are a powerful tool for managing complex data structures in Python. Now, you’re well equipped to navigate the branches of Python nested dictionaries. Happy coding!