How To Read JSON Files in Python | Guide (With Examples)
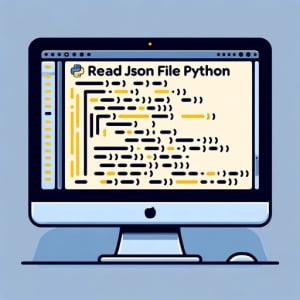
Struggling to read JSON files in Python? You’re not alone. JSON files, with their simple structure and universal format, are a common sight in the world of programming.
But when it comes to reading them in Python, things can get a bit tricky. Just like a librarian, Python can help you open the book of JSON and read its contents with ease.
This guide is designed to walk you through the process of reading JSON files in Python, from the basics to more advanced techniques. Whether you’re a beginner just starting out, or an experienced developer looking for a refresher, this guide has got you covered.
So let’s dive in and start decoding the mysteries of JSON files in Python.
TL;DR: How Do I Read a JSON File in Python?
To read a JSON file in Python, you use the
json
module’sload
function. Here’s a simple example:
import json
with open('file.json', 'r') as f:
data = json.load(f)
print(data)
# Output:
# Contents of 'file.json' printed here
This code block opens a JSON file named ‘file.json’, reads its contents using the json.load
function, and then prints the data. The json.load
function is a powerful tool in Python’s arsenal for handling JSON files, allowing you to read and parse JSON data with ease.
Intrigued? Keep reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding JSON in Python: The Basics
- Dealing with Complex JSON Structures
- Exploring Third-Party Libraries: Pandas
- Overcoming Common Issues in Reading JSON Files
- Unraveling JSON: The Backbone of Data Exchange
- The Power of JSON in Data Analysis and Web Scraping
- Extra Resources for JSON and More
- Mastering JSON Files in Python: A Recap
Understanding JSON in Python: The Basics
Python has a built-in module called json
for encoding and decoding JSON data. One of the most commonly used functions from this module is json.load()
. This function reads a file containing JSON object and returns a Python object. Let’s break it down.
import json
# Open the JSON file
with open('simple.json', 'r') as file:
# Load JSON data from file
data = json.load(file)
print(data)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In the above example, we first import the json
module. We then open a file named ‘simple.json’ in read mode. The with
statement is used here to ensure that the file is properly closed after it is no longer needed.
The json.load()
function is used to load the JSON file into a Python object. The result is a Python dictionary. If ‘simple.json’ contained the text {"name": "John", "age": 30, "city": "New York"}
, the output of the print statement would be {'name': 'John', 'age': 30, 'city': 'New York'}
.
This is a straightforward way to read a JSON file in Python, but it’s worth noting that the json.load()
function can only handle files that contain a single JSON object. If the file contains multiple JSON objects, you’ll need to use a different approach, which we’ll cover in the ‘Intermediate Level’ section.
One potential pitfall to be aware of when using json.load()
is that it can throw a JSONDecodeError
if the input file is not properly formatted as JSON. We’ll discuss how to handle this and other common issues in the ‘Troubleshooting and Considerations’ section.
Dealing with Complex JSON Structures
As we delve deeper into the world of JSON files, we come across more complex structures. These might include nested objects, arrays, or even multiple JSON objects in a single file. In such scenarios, the simple json.load()
function might not suffice.
Python’s json
module provides another function, json.loads()
, which can parse a JSON string. This function is particularly useful when dealing with JSON data received over a network, or stored as a string in a database.
Let’s take an example of a complex JSON structure:
import json
# A string containing JSON data
json_string = '{"employees":[{"firstName":"John", "lastName":"Doe"},{"firstName":"Anna", "lastName":"Smith"},{"firstName":"Peter", "lastName":"Jones"}]}'
# Parse the JSON string
data = json.loads(json_string)
print(data)
# Output:
# {'employees': [{'firstName': 'John', 'lastName': 'Doe'}, {'firstName': 'Anna', 'lastName': 'Smith'}, {'firstName': 'Peter', 'lastName': 'Jones'}]}
In the above example, we have a string json_string
that contains JSON data. We use the json.loads()
function to parse this string into a Python object. The result is a Python dictionary that mirrors the nested structure of the original JSON data.
This ability to parse JSON strings is incredibly powerful, as it allows you to work with JSON data in a variety of contexts, not just when reading from files.
However, similar to json.load()
, json.loads()
can also throw a JSONDecodeError
if the input string is not a valid JSON. We’ll cover how to handle these errors in the ‘Troubleshooting and Considerations’ section.
Exploring Third-Party Libraries: Pandas
Python offers a wealth of third-party libraries that can simplify and streamline the process of reading JSON files. One such library is pandas
, a powerful data manipulation and analysis tool. The pandas
library provides a function called read_json()
that can read a JSON file and convert it into a pandas DataFrame.
Let’s take a look at an example:
import pandas as pd
# Read JSON file
data = pd.read_json('file.json')
print(data)
# Output:
# DataFrame representation of 'file.json' contents
In the above example, we import the pandas
library and use its read_json()
function to read a JSON file. The result is a DataFrame, a two-dimensional labeled data structure that is one of pandas’ primary data structures.
The advantage of using pandas
to read JSON files is that it can handle more complex JSON structures, including nested objects and arrays, and it can also read multiple JSON objects from a single file. Additionally, once the JSON data is in a DataFrame, you can use all of pandas’ data analysis and manipulation capabilities on it.
However, there are a few things to consider before deciding to use pandas
. Firstly, it is a large library and can be overkill if you only need to read JSON files and do not require any of its data analysis features. Secondly, pandas
can be slower than the json
module for reading small files, although it can be faster for large files due to its optimized data structures.
In conclusion, the choice between the json
module and pandas
depends on your specific needs. If you need to read simple JSON files and do not require advanced data analysis capabilities, the json
module is probably sufficient. However, if you are dealing with complex JSON data or need to perform data analysis, pandas
might be the better choice.
Overcoming Common Issues in Reading JSON Files
In your journey to read JSON files in Python, you might encounter a few roadblocks. However, don’t worry! Most of these issues are common and can be resolved easily. Let’s go over a couple of these problems and their solutions.
Dealing with ‘FileNotFoundError’
This error occurs when Python can’t locate the JSON file you’re trying to read. Here’s an example:
import json
try:
with open('nonexistent_file.json', 'r') as file:
data = json.load(file)
except FileNotFoundError:
print('File not found.')
# Output:
# File not found.
In the code above, we attempt to open a file that doesn’t exist. Python throws a FileNotFoundError
, which we catch and print a simple error message. Always ensure that the file path is correct and the file exists.
Handling ‘json.decoder.JSONDecodeError’
This error is thrown when the json.load()
or json.loads()
function tries to parse a badly formatted JSON. Here’s how you can handle this:
import json
malformed_json = '"key": "value"}'
try:
data = json.loads(malformed_json)
except json.decoder.JSONDecodeError:
print('Bad JSON format.')
# Output:
# Bad JSON format.
In the code block above, we try to parse a malformed JSON string. The json.loads()
function throws a json.decoder.JSONDecodeError
, which we catch and print an error message. Always ensure your JSON data is correctly formatted.
These are just a couple of the common issues you might encounter when reading JSON files in Python. Remember, with a good understanding of the process and a bit of practice, you’ll be able to overcome these hurdles with ease.
Unraveling JSON: The Backbone of Data Exchange
Before we delve deeper into the Pythonic way of handling JSON files, let’s take a moment to understand what JSON is and why it’s so important.
JSON, or JavaScript Object Notation, is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It’s based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition – December 1999.
A JSON object looks something like this:
{
"firstName": "John",
"lastName": "Doe",
"age": 30,
"address": {
"streetAddress": "21 2nd Street",
"city": "New York",
"state": "NY",
"postalCode": "10021-3100"
}
}
JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
JSON is built on two structures:
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
In Python, JSON objects are translated into dictionaries, and JSON arrays are translated into lists. This makes JSON a very natural format to use in Python programs.
Python’s json
module is a powerful tool for working with JSON data. It provides functions like json.load()
, json.loads()
, json.dump()
, and json.dumps()
to read and write JSON data, allowing you to easily convert between JSON and Python objects.
The Power of JSON in Data Analysis and Web Scraping
Understanding how to read JSON files in Python can open up a world of possibilities. JSON is not just a simple data format, but a crucial player in various domains such as data analysis, web scraping, data storage, and more.
For instance, in data analysis, JSON files often serve as a source of data. With Python’s ability to read these files, you can easily import data from JSON files into your analysis workflows. The pandas
library, which we discussed earlier, is particularly useful in this context, as it can convert JSON data into a DataFrame, a format that is much easier to analyze.
In the field of web scraping, JSON plays a pivotal role too. Modern web applications frequently use JSON to send data from the server to the client. Therefore, when you’re scraping websites, you’re likely to encounter JSON data. Python’s json
module allows you to parse this data and extract the information you need.
Beyond just reading JSON files, there are several related concepts that might interest you. For example, you might want to learn how to write to a JSON file in Python, which is the reverse of what we’ve been discussing. The json
module provides the dump()
and dumps()
functions for this purpose.
Another important concept is handling JSON data in Python. This involves more than just reading and writing JSON data, but also manipulating it, such as adding, modifying, or deleting items from a JSON object.
Extra Resources for JSON and More
For a deeper understanding of these topics, we recommend checking out the following resources:
- Beginner’s Guide to Python JSON – Master the art of encoding and decoding JSON data in Python programs.
YAML Handling in Python – Explore Python’s YAML support for structured data representation.
Encoding URLs in Python – A quick guide on URL encoding and decoding using Python libraries.
Python JSON Formatting Guide – A helpful tutorial on how to effectively format JSON using Python.
Official Documentation for Python ‘json’ Module – Access the python.org official guide to mastering the Python ‘json’ module.
Pandas Read JSON Method Documentation – Learn how to use the ‘read_json’ method in pandas, as detailed in the pandas official documentation.
Mastering JSON Files in Python: A Recap
Throughout this guide, we’ve explored the ins and outs of reading JSON files in Python. We started with the basics, using Python’s built-in json
module and the load
function to read a simple JSON file.
We then delved into more complex scenarios, using the loads
function to parse JSON strings and handle more intricate JSON structures.
For those seeking alternative approaches, we introduced pandas
and its read_json
function, which can convert JSON data into a DataFrame for easy analysis and manipulation.
We also discussed some common issues you might encounter, such as ‘FileNotFoundError’ and ‘json.decoder.JSONDecodeError’, and how to handle them.
Finally, we explored the importance of JSON in data exchange and its relevance in fields like data analysis and web scraping. With this knowledge in your toolkit, you’re now equipped to tackle any JSON file that comes your way in Python.
Remember, practice is key, so don’t hesitate to experiment with different JSON structures and Python functions. Happy coding!