Finding the Length of a List in Python (With Examples)
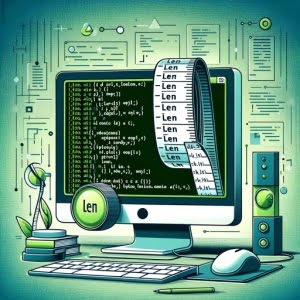
Determining the length of a list has many use cases when scripting data processing and analysis tasks, especially for us at IOFLOOD. As we have gotten familiar with the various available methods, we have created this article to share our techniques and best practices for finding the length of lists in Python, aiding our dedicated server hosting customers with their data processing needs.
In this guide, we will walk you through the process of finding the length of a list in Python. We will start with the basics and gradually delve into more advanced techniques. Whether you’re a novice coder or a seasoned developer, this guide has something for you.
Let’s dive in!
TL;DR: How Do I Find the Length of a List in Python?
You can use Python’s built-in
len()
function to find the length of a list with the syntax,listLength = len(my_list))
.
Here’s a simple example:
my_list = [1, 2, 3, 4, 5]
print(len(my_list))
# Output:
# 5
In this example, we created a list my_list
with five elements. Then, we used the len()
function, which returned the number of elements in the list, which is 5. The len()
function is a straightforward and efficient way to find the length of a list in Python.
Keep reading for a more in-depth explanation and to learn about more advanced usage scenarios of finding the length of a list in Python.
Table of Contents
Python’s len()
Function
Python’s built-in len()
function is a simple yet powerful tool that returns the number of items in a list or the length of a string. When used with a list, len()
provides the total count of elements in it. Let’s take a closer look at how it works:
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
print(len(fruits))
# Output:
# 5
In this example, we have a list named fruits
which contains five elements. When we pass this list to the len()
function, it returns the total count of elements in the list, which is 5.
The len()
function is straightforward to use and efficient. It can handle lists of any size and type, making it a versatile tool in Python.
It’s important to remember that
len()
only counts the top-level elements in a list. If you have a list with nested lists (a list within a list),len()
will count the nested list as a single element. Read below for a solution.
Nested Lists with len()
As you continue learning Python, you’ll come across more complex data structures like nested lists. A nested list is a list within a list. So, how does the len()
function handle these? Let’s explore:
nested_list = ['apple', ['banana', 'cherry'], 'date', ['elderberry', 'fig', 'grape']]
print(len(nested_list))
# Output:
# 4
In the example above, our nested_list
contains four elements. Two of these elements are themselves lists. However, when we use the len()
function, it counts only the top-level elements, returning a length of 4.
What if you want to find the total number of individual elements, including those within the nested lists? You’ll need to write a function to handle this. Here’s a simple example:
def total_elements(lst):
total = 0
for i in lst:
if type(i) == list:
total += total_elements(i)
else:
total += 1
return total
print(total_elements(nested_list))
# Output:
# 7
In the total_elements
function, we iterate over each item in the list. If an item is a list (checked using type(i) == list
), we recursively call total_elements
on it. If not, we increment the total
by 1. This function effectively counts all individual elements in a list, including those in nested lists.
Understanding how to handle nested lists is crucial for more advanced Python programming, including data analysis and machine learning.
Alternative Methods
While the len()
function is the most straightforward way to find the length of a list in Python, it’s not the only way. In this section, we will introduce two alternative methods: the reduce()
function from the functools
module and list comprehension. These methods can be particularly useful when dealing with more complex scenarios.
Using reduce()
from functools
The reduce()
function, found in Python’s functools
module, can also be used to calculate the length of a list. Here’s how:
from functools import reduce
my_list = ['apple', 'banana', 'cherry', 'date', 'elderberry']
length = reduce(lambda x, y: x + 1, my_list, 0)
print(length)
# Output:
# 5
In this example, we use reduce()
with a lambda function that increments a counter for each element in the list. The third argument to reduce()
is the initial value of the counter. This method can handle any iterable and can be customized with any function, making it a flexible tool. However, it can be less readable and efficient than len()
for simple list length calculations.
List Comprehension
List comprehension is a unique feature in Python that can be used to create and manipulate lists. We can use it to calculate the length of a list as follows:
my_list = ['apple', 'banana', 'cherry', 'date', 'elderberry']
length = sum([1 for _ in my_list])
print(length)
# Output:
# 5
In this example, we create a new list with a 1
for each element in my_list
, then use sum()
to add them up, effectively counting the elements. This method is very Pythonic and can be used for more complex tasks, but it may be overkill for simply finding the length of a list and could use more memory than len()
for large lists.
While len()
is usually the best choice for finding the length of a list in Python, alternative methods like reduce()
and list comprehension can sometimes be preferable.
Troubleshooting Common Issues
While finding the length of a list in Python is relatively straightforward, you may encounter some common issues. Let’s discuss these problems and their solutions.
TypeError with len()
One common issue is encountering a TypeError when using len()
with a non-iterable data type, such as a float or an integer.
Here’s an example:
number = 12345
print(len(number))
# Output:
# TypeError: object of type 'int' has no len()
In this example, we are trying to find the length of an integer, which results in a TypeError. The len()
function can only be used with iterable objects like dictionaries, strings, and lists.
To solve this, ensure that you only use len()
with iterable objects. If you want to find the number of digits in an integer, you can convert it to a string first:
number = 12345
print(len(str(number)))
# Output:
# 5
Handling Empty Lists
Another issue you might face is handling empty lists. If you apply len()
to an empty list, it will return 0, which is the correct and expected behavior. However, in your code, you might want to handle this case differently. Here’s an example:
empty_list = []
print(len(empty_list))
# Output:
# 0
In this example, len(empty_list)
returns 0. Depending on your program’s requirements, you might want to check if a list is empty before finding its length.
What Are Python Lists?
Now, let’s take a step back and understand what a list in Python is. A list is a built-in Python data type that can hold different types of items. These items are ordered and changeable, and Python allows for duplicate members.
Here’s an example of a Python list:
my_list = ['apple', 'banana', 'cherry']
print(my_list)
# Output:
# ['apple', 'banana', 'cherry']
In this example, my_list
is a list that contains three elements. Each element is a string. Lists in Python are versatile and can hold any data type, including numbers, strings, and even other lists.
mixed_list = ['apple', 10, ['banana', 'cherry']]
print(mixed_list)
# Output:
# ['apple', 10, ['banana', 'cherry']]
In this mixed_list
, we have a string, an integer, and another list. This flexibility makes lists a powerful tool in Python.
When we talk about the length of a list, we refer to the number of top-level items or elements it contains. In the case of my_list
, the length is 3. For mixed_list
, the length is also 3, because it counts the nested list ['banana', 'cherry']
as a single item.
Real-World Uses
There are plenty of times you’d want to find the length of a list. For instance, in data analysis, you might need to know the length of a list to determine the number of data points you have. In machine learning, list lengths can be used to shape data sets for training models.
# Example of list length in data analysis
# List of sales data
sales_data = [150, 200, 175, 210, 250, 180, 220]
# Finding the length of the list
num_data_points = len(sales_data)
print(f'We have {num_data_points} data points.')
# Output:
# We have 7 data points.
In this example, we have a list sales_data
representing the number of sales made each day in a week. By finding the length of this list, we determine the number of data points we have, which is crucial for further data analysis.
Links and Further Resources
If you’re interested in learning more ways to utilize the Python language, here are a few resources that you might find helpful:
- Python Lists Uncovered: Simplifying Data Structures: Simplify your understanding of data structures by exploring Python lists, a fundamental tool for working with data in Python.
Guide on Reversing a List in Python: IOFlood’s guide demonstrates various approaches to reverse a list in Python, such as using the reversed() function, the reverse() method, and slicing with a negative step.
Tutorial on Flattening a Nested List in Python: IOFlood’s tutorial explores different methods to flatten a nested list in Python, discussing techniques like list comprehension, and recursion.
Find the Length of a List in Python: A tutorial on DigitalOcean that explains different methods to find the length of a list in Python.
Python List Length: A Beginner’s Guide: A beginner-friendly guide on Edureka that demonstrates how to find the length of a list in Python.
Python – Ways to Find the Length of List: An article on GeeksforGeeks that presents different approaches to determine the length of a list in Python.
Wrapping Up
In this comprehensive guide, we’ve explored how to find the length of a list in Python, a fundamental operation in Python programming. We learned that Python’s built-in len()
function is the simplest and most efficient way to measure list length.
We discussed common issues, such as encountering a TypeError when using len()
with a non-iterable data type, and handling empty lists. We also explored alternative methods to find list length, including the reduce()
function from the functools
module and list comprehension, and how to handle nested lists.
Keep exploring, keep practicing, and you’ll become a Python list expert!