Python Empty List | Guide to Using Empty Lists in Python
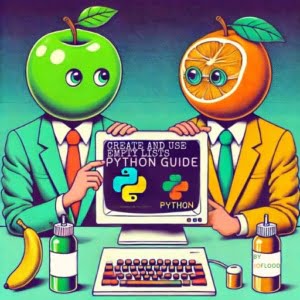
Creating and using empty lists in Python is a pivotal step we utilize when developing software at IOFLOOD. In our experience, empty lists serve as a starting point for data collection and manipulation, and aids in clean and concise code. This article shares our techniques and best practices for creating and using empty lists in Python, helping our bare metal hosting customers manage their data more effectively.
In this guide, we will focus on understanding and utilizing empty lists in Python. By the end of this post, you’ll know how to create, verify, and manipulate empty lists. You’ll also understand the common pitfalls and how to avoid them. So, whether you’re a beginner looking to expand your Python knowledge or an experienced programmer looking to brush up on the basics, this guide is for you.
Let’s embark on this journey!
TL;DR: How do I create and use empty lists in Python?
You can create an empty list in Python using either square brackets
[]
or thelist()
function. An empty list can be used as a placeholder for data that will be added later, or to represent a lack of data. To use an empty list, you can add items using methods like.append()
,.insert()
, and.extend()
. Read on for more advanced methods, background, tips and tricks.
Example:
# Creating an empty list
empty_list = []
# Adding an item to the list
empty_list.append('item')
Table of Contents
The Basics of Empty Lists in Python
In Python, there are two primary methods to create an empty list: using square brackets []
and using the list()
function. This section will provide a comprehensive examination of these methods, along with practical examples of creating, verifying, and manipulating empty lists.
The Square Brackets Method
The first and most common method to create an empty list is to use square brackets. Here’s how you do it:
empty_list = []
This method is typically faster because it involves fewer steps. Python directly interprets the square brackets as an empty list without any additional processing.
The list() Function Method
The second method to create an empty list is to use the list()
function, as shown below:
empty_list = list()
This method involves an extra step, as Python has to process the list()
function to create an empty list. Therefore, it’s slightly slower than using square brackets.
Comparing the Methods
Both methods will give you an empty list, but using square brackets is faster and more commonly used. However, the list()
function method can be more readable, especially for beginners. It clearly states that you’re creating a list, which can make your code easier to understand.
Verifying a Python List is Empty
Once you’ve created an empty list, you might want to verify it. You can do this by checking its length or its boolean value. Both should return False
or 0
for an empty list. Here’s how you can do it:
empty_list = []
print(len(empty_list) == 0) # Returns True
print(not empty_list) # Also returns True
In Python, an empty list is considered False
in a boolean context. Therefore, using not
with an empty list returns True
.
How to Manipulate an Empty List
Once you’ve created an empty list, you can add items to it. Python offers several methods to accomplish this, such as .append()
, .insert()
, and .extend()
. Let’s explore each of these methods.
The .append() Method
The .append()
method adds an item to the end of the list. Here’s how you can use it:
empty_list.append('apple')
print(empty_list) # Outputs: ['apple']
The .insert() Method
The .insert()
method adds an item at a specific position in the list. The first argument is the index position, and the second argument is the item. Here’s how you can use it:
empty_list.insert(0, 'banana')
print(empty_list) # Outputs: ['banana', 'apple']
The .extend() Method
The .extend()
method adds multiple items to the end of the list. The argument is a list of items. Here’s how you can use it:
empty_list.extend(['cherry', 'date'])
print(empty_list) # Outputs: ['banana', 'apple', 'cherry', 'date']
Using List Comprehension
List comprehension is a concise way to create lists based on existing lists. For example, you can use list comprehension to create a list of the first ten square numbers:
squares = [x**2 for x in range(1, 11)]
print(squares) # Outputs: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
In this line of code, x**2
is the expression for the items in the list, and for x in range(1, 11)
is the loop that generates the numbers 1 through 10.
Use Cases for Empty Lists
Empty lists in Python are more than just placeholders waiting for data. They are versatile tools that can be used in a variety of scenarios. Let’s discuss some of the most common use cases for empty lists and how they can enhance your Python programming journey.
Initializing a List
One of the most basic uses of an empty list is to initialize a list that will be populated later. This is like reserving seats on our train for passengers who will board later. For example, you might create an empty list to store user inputs or results from a loop. This is a common practice in Python programming.
Example of initializing a list and adding data to it later:
# Initializing a list
user_inputs = []
# Adding data to the list later in the code
user_inputs.append(input('Enter a value: '))
print(user_inputs) # Outputs: [value entered by the user]
user_inputs = []
# Later in the code
user_inputs.append(input('Enter a value: '))
Conditional Statements and Error Handling
Empty lists are also useful in conditional statements. Because an empty list is considered False
in a boolean context, you can use it to check if a list is empty. This can be useful for error handling, like checking if all passengers have disembarked before closing the train doors. For example:
if not my_list:
print('The list is empty.')
In this code, if not my_list
checks if my_list
is empty. If it is, the program prints ‘The list is empty.’
Placeholders for Future Inputs/Outputs
Empty lists can serve as placeholders for future inputs or outputs. For instance, you might create an empty list to store the results of a function that will be run multiple times. Each time the function is run, its results can be appended to the list, like adding passengers at each station.
results = []
# Later in the code
results.append(my_function())
Computational Mechanics and UI Design
In computational mechanics, empty lists are often used to store the results of calculations. For example, you might create an empty list to store the results of a series of mathematical operations.
In UI design, empty lists can be used to store user inputs or to create dynamic elements, like displaying the list of passengers on a digital board.
Boolean Contexts
As we’ve discussed, an empty list is considered False
in a boolean context. This can be useful in a variety of scenarios. For example, you might use an empty list in a while loop to continue the loop until the list is populated, like waiting for all passengers to board before the train departs.
while not my_list:
my_list.append(input('Enter a value: '))
In this code, while not my_list
continues the loop as long as my_list
is empty. Once a value is added to my_list
, the loop stops.
Avoiding Errors with Python Lists
Just like a train conductor must avoid common mistakes to ensure a smooth journey, Python programmers, especially beginners, need to be aware of common pitfalls when dealing with lists.
By understanding these, you can write cleaner, more efficient code. Let’s explore these mistakes and learn how to steer clear of them.
Unnecessary Use of the list() Constructor
While the list()
function is a valid way to create an empty list, it’s not always the best choice. As we discussed earlier, using square brackets []
is faster and more commonly used, like choosing a faster train route. Unless you have a specific reason to use the list()
function, stick with square brackets.
Example of the preferred method of creating a list:
# Preferred method
my_list = []
print(my_list) # Outputs: []
# Preferred method
my_list = []
# Not preferred unless necessary
my_list = list()
Creating Multiple References to the Same List
In Python, lists are mutable objects. This means that if you create a new reference to an existing list, any changes you make to one reference will affect all other references.
This can lead to unexpected behavior, like changing the destination of one train ticket affecting all tickets. Here’s an example:
list1 = []
list2 = list1
list2.append('apple')
print(list1) # Outputs: ['apple']
In this code, list1
and list2
are references to the same list. Therefore, when we append ‘apple’ to list2
, list1
is also affected.
Example of the problem and how to avoid it by creating a copy of the list:
# Creating a list
list1 = []
# Creating a new reference to the same list
list2 = list1
# Adding an item to list2
list2.append('apple')
print(list1) # Outputs: ['apple']
# Creating a copy of the list
list3 = list1.copy()
# Adding an item to list3
list3.append('banana')
print(list1) # Outputs: ['apple']
print(list3) # Outputs: ['apple', 'banana']
To avoid this, you can create a copy of the list using the .copy()
method or the slicing syntax [:]
, like issuing separate tickets to each passenger.
# Using .copy()
list2 = list1.copy()
# Using slicing
list2 = list1[:]
Shallow vs Deep Copy
When copying lists, it’s important to understand the difference between a shallow copy and a deep copy, like knowing the difference between a local and an express train. A shallow copy creates a new list with references to the same items, while a deep copy creates a new list with copies of the items.
This distinction is important when dealing with lists of mutable objects, such as other lists. A shallow copy of a list of lists will create a new outer list, but the inner lists will still be the same objects. Therefore, changes to the inner lists will affect both the original list and the copy.
To create a deep copy, you can use the copy.deepcopy()
function from the copy
module, like creating completely separate trains for different routes.
Example of the difference between a shallow copy and a deep copy:
# Creating a list of lists
list1 = [[1, 2], [3, 4]]
# Creating a shallow copy
list2 = list1.copy()
# Creating a deep copy
import copy
list3 = copy.deepcopy(list1)
# Modifying an inner list of list2
list2[0][0] = 'a'
print(list1) # Outputs: [['a', 2], [3, 4]]
print(list2) # Outputs: [['a', 2], [3, 4]]
print(list3) # Outputs: [[1, 2], [3, 4]]
import copy
list2 = copy.deepcopy(list1)
Further Resources for Lists in Python
If you’re interested in learning more about Python lists, here are a few resources that you might find helpful:
- Optimize Your Code with Python Lists: Master efficient list operations and methodologies in Python.
Check if a List is Empty in Python: Guide on various approaches to check if a list is empty in Python.
Filtering a List in Python: Tutorial showcasing different techniques to filter a list in Python based on specific conditions.
Comprehensive Python Syntax Guide: Extensive guide and cheat sheet covering Python syntax for programmers of all levels.
How to Declare an Empty List in Python: GeeksforGeeks article explaining different methods to declare an empty list in Python.
Python Empty List Tutorial: How to Create an Empty List in Python: A tutorial on FreeCodeCamp that demonstrates various ways to create an empty list in Python.
Empty List in Python: Tips and Tricks: Article on Scaler discussing tips and tricks related to empty lists in Python, including initializing an empty list, checking if a list is empty, and common use cases.
These resources will help you deepen your understanding of working with lists in Python and provide useful techniques for checking if a list is empty and filtering its elements.
Conclusion: Empty List Management
Empty lists, like our train at the start of its journey, serve as the starting point for storing and manipulating data in Python. We’ve seen how they can be created using either square brackets or the list()
function. We’ve also discussed how to verify them by checking their length or boolean context, ensuring that our train is ready for the journey ahead.
We’ve also explored how empty lists are not just placeholders for data. They are versatile tools that can be used in various scenarios, from initializing a list, to error handling, to computational mechanics and UI design.
We’ve discussed some common mistakes Python programmers make when working with lists, such as unnecessary use of the list() constructor and creating multiple references to the same mutable object. By being aware of these pitfalls, you can write cleaner, more efficient code.
For more advanced methods, background, tips and tricks on Python programming, continue exploring our blog. Practice and experiment with code, and you’ll soon be navigating Python like a seasoned programmer. Keep learning and keep growing!