Using Python to Filter a List: Targeted Guide
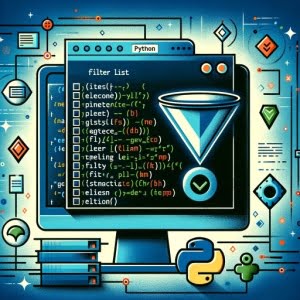
Filtering a list in Python is a a technique we often use when working to automate processes at IOFLOOD. In our experience, this process assists with consistent extraction of specific elements that meet defined criteria. Today’s article explains how to filter a list in Python, providing valuable insights and examples to assist our bare metal cloud server customers.
This comprehensive guide will cover everything from basic usage to sophisticated techniques. Whether you’re a Python novice or a seasoned programmer, you’ll find valuable insights into how to use the filter()
function more effectively to clean and analyze your data.
So let’s dive and and learn how to properly filter a python list!
TL;DR: How Do I Filter a List in Python?
You can utilize the
filter()
function in Python to filter a list. The syntax for this function is,sample_list = filter(filteringFunction)
.
Here’s a simple illustration:
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
print(list(even_numbers))
# Output:
# [2, 4]
In this example, we’ve used the filter()
function with a lambda function to filter out the even numbers from our list.
Keep reading for a deeper dive into Python list filtering, including more detailed examples and advanced usage scenarios.
Table of Contents
The Basics of Python Filter Function
The filter()
function in Python is a powerful tool for processing lists.
It takes in two arguments: a function and a list. The function is applied to each item in the list, and filter()
creates a new list with only the items for which the function returned True
. It’s like a gatekeeper, only letting through the items that meet a certain condition.
Here’s a basic example of the filter()
function in action:
# Here is a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# We define a function that checks if a number is greater than 5
def is_greater_than_five(x):
return x > 5
# We use the filter function to get a new list with only the numbers that are greater than 5
filtered_numbers = filter(is_greater_than_five, numbers)
# We convert the filter object to a list and print it
print(list(filtered_numbers))
# Output:
# [6, 7, 8, 9, 10]
In this example, we’ve defined a function is_greater_than_five(x)
, which checks if a number x
is greater than 5. We pass this function and our list of numbers to filter()
.
The filter()
function goes through each item in the list and applies the is_greater_than_five(x)
function to it. If the function returns True
, the item is added to the new list. If the function returns False
, the item is not included in the new list.
It’s important to note that the
filter()
function returns a filter object, not a list. To get a list, you need to convert the filter object to a list, as we did withlist(filtered_numbers)
in our example. If you forget to do this, you might run into unexpected behavior in your code.
Filtering Lists with Lambda Functions
As you become more comfortable with Python and its filter()
function, you can start to explore more complex uses. One such use is filtering with Python lambda functions.
Lambda functions are single line, anonymous functions that you can define in a single line. They’re useful when you need a small function for a short period of time and don’t want to define a full function for it.
Here’s an example of how you can use a lambda function with filter()
:
# Here is a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# We use a lambda function to check if a number is even
even_numbers = filter(lambda x: x % 2 == 0, numbers)
# We convert the filter object to a list and print it
print(list(even_numbers))
# Output:
# [2, 4, 6, 8, 10]
In this example, we’ve replaced the is_greater_than_five(x)
function from the previous example with a lambda function that checks if a number is even. The lambda function is defined right in the call to filter()
, making our code more concise.
Lambda functions can be a powerful tool when used with filter()
, allowing you to define complex filtering conditions without having to define a separate function. However, keep in mind that lambda functions can make your code harder to read if overused or used in complex scenarios.
Alternative Filtering Techniques
While the filter()
function is a powerful tool for filtering lists in Python, it’s not the only game in town. There are other techniques you can use to filter lists, such as list comprehension and the itertools
module.
Let’s dive into these alternatives and see how they compare to the filter()
function.
List Comprehension: A Pythonic Alternative
List comprehension is a concise way to create lists based on existing lists. In Python, list comprehension is considered more Pythonic than using functions like filter()
and map()
. Here’s how you can use list comprehension to filter a list:
# Here is a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# We use list comprehension to get a new list with only the even numbers
even_numbers = [x for x in numbers if x % 2 == 0]
# We print the new list
print(even_numbers)
# Output:
# [2, 4, 6, 8, 10]
In this example, we’ve used list comprehension to create a new list with only the even numbers from our original list. The syntax is a bit different from the filter()
function, but the result is the same.
List comprehension can be more readable than the filter()
function, especially for simple filtering conditions. However, for complex conditions, list comprehension can become hard to read.
To read further on the uses of Lambda functions, check out this helpful guide!
The itertools Module: Advanced Filtering
If you’re dealing with complex filtering conditions or large data sets, the itertools
module can be a powerful tool. The itertools
module contains a function filterfalse()
, which returns the items for which the function returns False
. Here’s an example:
import itertools
# Here is a list of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# We use itertools.filterfalse() to get a new list with only the odd numbers
odd_numbers = itertools.filterfalse(lambda x: x % 2 == 0, numbers)
# We convert the filter object to a list and print it
print(list(odd_numbers))
# Output:
# [1, 3, 5, 7, 9]
In this example, we’ve used itertools.filterfalse()
to create a new list with only the odd numbers from our original list. The filterfalse()
function is similar to the filter()
function, but it returns the items for which the function returns False
instead of True
. This can be useful in scenarios where you want to filter out certain items instead of keeping them.
When choosing a method to filter lists in Python, consider the complexity of your filtering condition and the size of your data. For simple conditions, the
filter()
function or list comprehension can be a good choice. For more complex conditions or large data sets, theitertools
module might be more suitable.
Troubleshooting Python List Filtering
While Python’s filter()
function is a powerful tool, you might encounter some common issues when using it. Let’s discuss these potential pitfalls and provide some solutions and workarounds.
Dealing with Empty Lists
One common issue arises when you’re dealing with empty lists. If the filter()
function is given an empty list, it will return an empty filter object. Here’s an example:
# Here is an empty list
numbers = []
# We try to filter the empty list
filtered_numbers = filter(lambda x: x > 5, numbers)
# We convert the filter object to a list and print it
print(list(filtered_numbers))
# Output:
# []
In this case, the filter()
function returns an empty list. If your code doesn’t account for this possibility, it might cause issues later on. Therefore, it’s a good practice to check if a list is empty before filtering it.
Filtering Based on Complex Conditions
Another issue can occur when you’re trying to filter a list based on complex conditions. The filter()
function can only handle conditions that can be expressed as a function that returns True
or False
.
If your condition is more complex, you might need to use a different technique, like list comprehension or the itertools
module.
For instance, suppose you have a list of dictionaries and you want to filter it based on the values of two different keys. Here’s how you can do it with list comprehension:
# Here is a list of dictionaries
data = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
# We use list comprehension to get a new list with only the dictionaries where the age is greater than 25
filtered_data = [d for d in data if d['age'] > 25]
# We print the new list
print(filtered_data)
# Output:
# [{'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
In this example, we’ve used list comprehension to filter our list of dictionaries based on the value of the ‘age’ key. This would be difficult to do with the filter()
function, as it can only handle conditions that can be expressed as a single function.
Real-World Uses of List Filterig
Python list filtering, as we’ve seen, is a powerful tool for data manipulation. But its relevance extends far beyond basic list operations.
In the fields of data analysis and machine learning, the ability to filter data effectively is crucial. It allows analysts and data scientists to focus on the data that matters, cleaning datasets and isolating key information for further processing.
Take, for example, a machine learning model being trained on a dataset. Not all data is equally useful – some might be irrelevant or even detrimental to the model’s performance. List filtering can help clean the dataset, removing unneeded data and improving the model’s accuracy.
# Suppose we have a list of data points for a machine learning model
data_points = [...]
# We can use list filtering to remove any data points that we know are not relevant
relevant_data_points = filter(is_relevant, data_points)
# We can then train our model on the relevant data points
model.train(relevant_data_points)
In this code snippet, is_relevant
could be a function that determines whether a data point is relevant for our machine learning model. By filtering out irrelevant data points, we can make our model more accurate and efficient.
Beyond list filtering, Python offers other powerful functions for data manipulation, such as map()
and reduce()
. The map()
function applies a function to every item of an iterable (like a list), while the reduce()
function applies a function to all items of an iterable in a cumulative way. These functions, combined with filter()
, form the cornerstone of functional programming in Python and are worth exploring further.
Further Resources for Python
If you’re interested in learning more about empty lists and adding elements to a list in Python, here are a few resources that you might find helpful:
- The Comprehensive Python Lists Resource: Dive into this comprehensive resource for a detailed exploration of Python lists, suitable for both beginners and advanced programmers.
Guide to Using Empty Lists in Python: An IOFlood overview of using empty lists in Python, including creating, checking, and appending elements to empty lists.
Tutorial: Adding Elements to a List in Python: This tutorial demonstrates various methods to add elements to a list in Python.
Official Python Documentation: Data Structures: Comprehensive documentation on data structures in Python.
Real Python: An online platform offering a wide range of Python tutorials, articles, and resources.
Python for Beginners: A website providing simplified Python tutorials and resources for beginners.
These resources will provide you with a comprehensive understanding of working with empty lists and adding elements to a list in Python.
A Recap: Python List Filtering
Throughout this guide, we’ve delved into the process of filtering lists in Python using the filter()
function. We’ve seen how it works, how to use it with lambda functions, and how to troubleshoot common issues. We’ve also explored alternative approaches like list comprehension and the itertools
module.
In essence, the filter()
function in Python allows us to create a new list from an existing one, keeping only elements that satisfy a certain condition.
We’ve also discussed potential issues you might encounter when using the filter()
function, such as dealing with empty lists and complex filtering conditions. Remember to check if a list is empty before filtering it, and consider other methods like list comprehension or the itertools
module for complex conditions.
Speaking of alternative methods, list comprehension is a more Pythonic way to create lists based on existing ones. It can be more readable than filter()
, especially for simple conditions. The itertools
module, on the other hand, offers advanced filtering functions for complex conditions or large data sets.
To wrap up, here’s a comparison table of the methods we’ve discussed:
Method | Complexity | Best for |
---|---|---|
filter() function | Simple to moderate | Simple conditions, small to medium-sized lists |
List comprehension | Simple to moderate | Simple conditions, readability |
itertools module | Moderate to high | Complex conditions, large data sets |
Each method has its strengths and weaknesses, so choose the one that best fits your needs. Whether you’re a beginner or an expert, understanding Python list filtering and its alternatives can help you manipulate and analyze data more effectively.